mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
build_api_test.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2016 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import unittest 00019 from collections import namedtuple 00020 from mock import patch, MagicMock 00021 from tools.build_api import prepare_toolchain, build_project, build_library,\ 00022 scan_resources 00023 00024 """ 00025 Tests for build_api.py 00026 """ 00027 00028 class BuildApiTests (unittest.TestCase): 00029 """ 00030 Test cases for Build Api 00031 """ 00032 00033 def setUp (self): 00034 """ 00035 Called before each test case 00036 00037 :return: 00038 """ 00039 self.target = "K64F" 00040 self.src_paths = ['.'] 00041 self.toolchain_name = "ARM" 00042 self.build_path = "build_path" 00043 00044 def tearDown (self): 00045 """ 00046 Called after each test case 00047 00048 :return: 00049 """ 00050 pass 00051 00052 @patch('tools.toolchains.arm.ARM_STD.parse_dependencies', 00053 return_value=["foo"]) 00054 @patch('tools.toolchains.mbedToolchain.need_update', 00055 side_effect=[i % 2 for i in range(3000)]) 00056 @patch('os.mkdir') 00057 @patch('tools.toolchains.exists', return_value=True) 00058 @patch('tools.utils.run_cmd', return_value=("", "", 0)) 00059 def test_always_complete_build(self, *_): 00060 with MagicMock() as notify: 00061 toolchain = prepare_toolchain(self.src_paths , self.build_path , self.target , 00062 self.toolchain_name , notify=notify) 00063 00064 res = scan_resources(self.src_paths , toolchain) 00065 00066 toolchain.RESPONSE_FILES=False 00067 toolchain.config_processed = True 00068 toolchain.config_file = "junk" 00069 toolchain.compile_sources(res) 00070 00071 assert any('percent' in msg[0] and msg[0]['percent'] == 100.0 00072 for _, msg, _ in notify.mock_calls if msg) 00073 00074 00075 @patch('tools.build_api.Config') 00076 def test_prepare_toolchain_app_config (self, mock_config_init): 00077 """ 00078 Test that prepare_toolchain uses app_config correctly 00079 00080 :param mock_config_init: mock of Config __init__ 00081 :return: 00082 """ 00083 app_config = "app_config" 00084 mock_target = namedtuple("Target", 00085 "init_hooks name features core")(lambda _, __ : None, 00086 "Junk", [], "Cortex-M3") 00087 mock_config_init.return_value = namedtuple("Config", 00088 "target has_regions")( 00089 mock_target, 00090 False) 00091 00092 prepare_toolchain(self.src_paths , None, self.target , self.toolchain_name , 00093 app_config=app_config) 00094 00095 mock_config_init.assert_called_once_with(self.target , self.src_paths , 00096 app_config=app_config) 00097 00098 @patch('tools.build_api.Config') 00099 def test_prepare_toolchain_no_app_config (self, mock_config_init): 00100 """ 00101 Test that prepare_toolchain correctly deals with no app_config 00102 00103 :param mock_config_init: mock of Config __init__ 00104 :return: 00105 """ 00106 mock_target = namedtuple("Target", 00107 "init_hooks name features core")(lambda _, __ : None, 00108 "Junk", [], "Cortex-M3") 00109 mock_config_init.return_value = namedtuple("Config", 00110 "target has_regions")( 00111 mock_target, 00112 False) 00113 00114 prepare_toolchain(self.src_paths , None, self.target , self.toolchain_name ) 00115 00116 mock_config_init.assert_called_once_with(self.target , self.src_paths , 00117 app_config=None) 00118 00119 @patch('tools.build_api.scan_resources') 00120 @patch('tools.build_api.mkdir') 00121 @patch('os.path.exists') 00122 @patch('tools.build_api.prepare_toolchain') 00123 def test_build_project_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00124 """ 00125 Test that build_project uses app_config correctly 00126 00127 :param mock_prepare_toolchain: mock of function prepare_toolchain 00128 :param mock_exists: mock of function os.path.exists 00129 :param _: mock of function mkdir (not tested) 00130 :param __: mock of function scan_resources (not tested) 00131 :return: 00132 """ 00133 app_config = "app_config" 00134 mock_exists.return_value = False 00135 mock_prepare_toolchain().link_program.return_value = 1, 2 00136 mock_prepare_toolchain().config = namedtuple("Config", "has_regions")(None) 00137 00138 build_project(self.src_paths , self.build_path , self.target , 00139 self.toolchain_name , app_config=app_config) 00140 00141 args = mock_prepare_toolchain.call_args 00142 self.assertTrue('app_config' in args[1], 00143 "prepare_toolchain was not called with app_config") 00144 self.assertEqual(args[1]['app_config'], app_config, 00145 "prepare_toolchain was called with an incorrect app_config") 00146 00147 @patch('tools.build_api.scan_resources') 00148 @patch('tools.build_api.mkdir') 00149 @patch('os.path.exists') 00150 @patch('tools.build_api.prepare_toolchain') 00151 def test_build_project_no_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00152 """ 00153 Test that build_project correctly deals with no app_config 00154 00155 :param mock_prepare_toolchain: mock of function prepare_toolchain 00156 :param mock_exists: mock of function os.path.exists 00157 :param _: mock of function mkdir (not tested) 00158 :param __: mock of function scan_resources (not tested) 00159 :return: 00160 """ 00161 mock_exists.return_value = False 00162 # Needed for the unpacking of the returned value 00163 mock_prepare_toolchain().link_program.return_value = 1, 2 00164 mock_prepare_toolchain().config = namedtuple("Config", "has_regions")(None) 00165 00166 build_project(self.src_paths , self.build_path , self.target , 00167 self.toolchain_name ) 00168 00169 args = mock_prepare_toolchain.call_args 00170 self.assertTrue('app_config' in args[1], 00171 "prepare_toolchain was not called with app_config") 00172 self.assertEqual(args[1]['app_config'], None, 00173 "prepare_toolchain was called with an incorrect app_config") 00174 00175 @patch('tools.build_api.scan_resources') 00176 @patch('tools.build_api.mkdir') 00177 @patch('os.path.exists') 00178 @patch('tools.build_api.prepare_toolchain') 00179 def test_build_library_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00180 """ 00181 Test that build_library uses app_config correctly 00182 00183 :param mock_prepare_toolchain: mock of function prepare_toolchain 00184 :param mock_exists: mock of function os.path.exists 00185 :param _: mock of function mkdir (not tested) 00186 :param __: mock of function scan_resources (not tested) 00187 :return: 00188 """ 00189 app_config = "app_config" 00190 mock_exists.return_value = False 00191 00192 build_library(self.src_paths , self.build_path , self.target , 00193 self.toolchain_name , app_config=app_config) 00194 00195 args = mock_prepare_toolchain.call_args 00196 self.assertTrue('app_config' in args[1], 00197 "prepare_toolchain was not called with app_config") 00198 self.assertEqual(args[1]['app_config'], app_config, 00199 "prepare_toolchain was called with an incorrect app_config") 00200 00201 @patch('tools.build_api.scan_resources') 00202 @patch('tools.build_api.mkdir') 00203 @patch('os.path.exists') 00204 @patch('tools.build_api.prepare_toolchain') 00205 def test_build_library_no_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00206 """ 00207 Test that build_library correctly deals with no app_config 00208 00209 :param mock_prepare_toolchain: mock of function prepare_toolchain 00210 :param mock_exists: mock of function os.path.exists 00211 :param _: mock of function mkdir (not tested) 00212 :param __: mock of function scan_resources (not tested) 00213 :return: 00214 """ 00215 mock_exists.return_value = False 00216 00217 build_library(self.src_paths , self.build_path , self.target , 00218 self.toolchain_name ) 00219 00220 args = mock_prepare_toolchain.call_args 00221 self.assertTrue('app_config' in args[1], 00222 "prepare_toolchain was not called with app_config") 00223 self.assertEqual(args[1]['app_config'], None, 00224 "prepare_toolchain was called with an incorrect app_config") 00225 00226 if __name__ == '__main__': 00227 unittest.main()
Generated on Tue Jul 12 2022 11:02:21 by
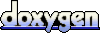