mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
aesni.h
00001 /** 00002 * \file aesni.h 00003 * 00004 * \brief AES-NI for hardware AES acceleration on some Intel processors 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_AESNI_H 00024 #define MBEDTLS_AESNI_H 00025 00026 #include "aes.h" 00027 00028 #define MBEDTLS_AESNI_AES 0x02000000u 00029 #define MBEDTLS_AESNI_CLMUL 0x00000002u 00030 00031 #if defined(MBEDTLS_HAVE_ASM) && defined(__GNUC__) && \ 00032 ( defined(__amd64__) || defined(__x86_64__) ) && \ 00033 ! defined(MBEDTLS_HAVE_X86_64) 00034 #define MBEDTLS_HAVE_X86_64 00035 #endif 00036 00037 #if defined(MBEDTLS_HAVE_X86_64) 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /** 00044 * \brief AES-NI features detection routine 00045 * 00046 * \param what The feature to detect 00047 * (MBEDTLS_AESNI_AES or MBEDTLS_AESNI_CLMUL) 00048 * 00049 * \return 1 if CPU has support for the feature, 0 otherwise 00050 */ 00051 int mbedtls_aesni_has_support( unsigned int what ); 00052 00053 /** 00054 * \brief AES-NI AES-ECB block en(de)cryption 00055 * 00056 * \param ctx AES context 00057 * \param mode MBEDTLS_AES_ENCRYPT or MBEDTLS_AES_DECRYPT 00058 * \param input 16-byte input block 00059 * \param output 16-byte output block 00060 * 00061 * \return 0 on success (cannot fail) 00062 */ 00063 int mbedtls_aesni_crypt_ecb( mbedtls_aes_context *ctx, 00064 int mode, 00065 const unsigned char input[16], 00066 unsigned char output[16] ); 00067 00068 /** 00069 * \brief GCM multiplication: c = a * b in GF(2^128) 00070 * 00071 * \param c Result 00072 * \param a First operand 00073 * \param b Second operand 00074 * 00075 * \note Both operands and result are bit strings interpreted as 00076 * elements of GF(2^128) as per the GCM spec. 00077 */ 00078 void mbedtls_aesni_gcm_mult( unsigned char c[16], 00079 const unsigned char a[16], 00080 const unsigned char b[16] ); 00081 00082 /** 00083 * \brief Compute decryption round keys from encryption round keys 00084 * 00085 * \param invkey Round keys for the equivalent inverse cipher 00086 * \param fwdkey Original round keys (for encryption) 00087 * \param nr Number of rounds (that is, number of round keys minus one) 00088 */ 00089 void mbedtls_aesni_inverse_key( unsigned char *invkey, 00090 const unsigned char *fwdkey, int nr ); 00091 00092 /** 00093 * \brief Perform key expansion (for encryption) 00094 * 00095 * \param rk Destination buffer where the round keys are written 00096 * \param key Encryption key 00097 * \param bits Key size in bits (must be 128, 192 or 256) 00098 * 00099 * \return 0 if successful, or MBEDTLS_ERR_AES_INVALID_KEY_LENGTH 00100 */ 00101 int mbedtls_aesni_setkey_enc( unsigned char *rk, 00102 const unsigned char *key, 00103 size_t bits ); 00104 00105 #ifdef __cplusplus 00106 } 00107 #endif 00108 00109 #endif /* MBEDTLS_HAVE_X86_64 */ 00110 00111 #endif /* MBEDTLS_AESNI_H */
Generated on Tue Jul 12 2022 11:02:21 by
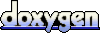