mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
UDPSocket.h
00001 00002 /** \addtogroup netsocket */ 00003 /** @{*/ 00004 /* UDPSocket 00005 * Copyright (c) 2015 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 00020 #ifndef UDPSOCKET_H 00021 #define UDPSOCKET_H 00022 00023 #include "netsocket/Socket.h" 00024 #include "netsocket/NetworkStack.h" 00025 #include "netsocket/NetworkInterface.h" 00026 #include "rtos/Semaphore.h" 00027 00028 00029 /** UDP socket 00030 */ 00031 class UDPSocket : public Socket { 00032 public: 00033 /** Create an uninitialized socket 00034 * 00035 * Must call open to initialize the socket on a network stack. 00036 */ 00037 UDPSocket(); 00038 00039 /** Create a socket on a network interface 00040 * 00041 * Creates and opens a socket on the network stack of the given 00042 * network interface. 00043 * 00044 * @param stack Network stack as target for socket 00045 */ 00046 template <typename S> 00047 UDPSocket(S *stack) 00048 : _pending(0), _read_sem(0), _write_sem(0) 00049 { 00050 open(stack); 00051 } 00052 00053 /** Destroy a socket 00054 * 00055 * Closes socket if the socket is still open 00056 */ 00057 virtual ~UDPSocket(); 00058 00059 /** Send a packet over a UDP socket 00060 * 00061 * Sends data to the specified address specified by either a domain name 00062 * or an IP address and port. Returns the number of bytes sent from the 00063 * buffer. 00064 * 00065 * By default, sendto blocks until data is sent. If socket is set to 00066 * non-blocking or times out, NSAPI_ERROR_WOULD_BLOCK is returned 00067 * immediately. 00068 * 00069 * @param host Hostname of the remote host 00070 * @param port Port of the remote host 00071 * @param data Buffer of data to send to the host 00072 * @param size Size of the buffer in bytes 00073 * @return Number of sent bytes on success, negative error 00074 * code on failure 00075 */ 00076 nsapi_size_or_error_t sendto(const char *host, uint16_t port, 00077 const void *data, nsapi_size_t size); 00078 00079 /** Send a packet over a UDP socket 00080 * 00081 * Sends data to the specified address. Returns the number of bytes 00082 * sent from the buffer. 00083 * 00084 * By default, sendto blocks until data is sent. If socket is set to 00085 * non-blocking or times out, NSAPI_ERROR_WOULD_BLOCK is returned 00086 * immediately. 00087 * 00088 * @param address The SocketAddress of the remote host 00089 * @param data Buffer of data to send to the host 00090 * @param size Size of the buffer in bytes 00091 * @return Number of sent bytes on success, negative error 00092 * code on failure 00093 */ 00094 nsapi_size_or_error_t sendto(const SocketAddress &address, 00095 const void *data, nsapi_size_t size); 00096 00097 /** Receive a packet over a UDP socket 00098 * 00099 * Receives data and stores the source address in address if address 00100 * is not NULL. Returns the number of bytes received into the buffer. 00101 * 00102 * By default, recvfrom blocks until data is sent. If socket is set to 00103 * non-blocking or times out, NSAPI_ERROR_WOULD_BLOCK is returned 00104 * immediately. 00105 * 00106 * @param address Destination for the source address or NULL 00107 * @param data Destination buffer for data received from the host 00108 * @param size Size of the buffer in bytes 00109 * @return Number of received bytes on success, negative error 00110 * code on failure 00111 */ 00112 nsapi_size_or_error_t recvfrom(SocketAddress *address, 00113 void *data, nsapi_size_t size); 00114 00115 protected: 00116 virtual nsapi_protocol_t get_proto(); 00117 virtual void event(); 00118 00119 volatile unsigned _pending; 00120 rtos::Semaphore _read_sem; 00121 rtos::Semaphore _write_sem; 00122 }; 00123 00124 00125 #endif 00126 00127 /** @}*/
Generated on Tue Jul 12 2022 11:02:34 by
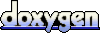