The easy-connect library for GR-PEACH.
Dependencies: LWIPBP3595Interface_STA_for_mbed-os
Dependents: GR-PEACH_HVC-P2_sample_client GR-PEACH_HVC-P2_IoTPlatform_http GR-PEACH_IoT_Platform_HTTP_sample
easy-connect.h
00001 #ifndef __MAGIC_CONNECT_H__ 00002 #define __MAGIC_CONNECT_H__ 00003 00004 #include "mbed.h" 00005 00006 #define ETHERNET 1 00007 #define WIFI_ESP8266 2 00008 #define WIFI_BP3595 3 00009 00010 #if MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ESP8266 00011 #include "ESP8266Interface.h" 00012 00013 #ifdef MBED_CONF_APP_ESP8266_DEBUG 00014 ESP8266Interface wifi(MBED_CONF_APP_ESP8266_TX, MBED_CONF_APP_ESP8266_RX, MBED_CONF_APP_ESP8266_DEBUG); 00015 #else 00016 ESP8266Interface wifi(MBED_CONF_APP_ESP8266_TX, MBED_CONF_APP_ESP8266_RX); 00017 #endif 00018 00019 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_BP3595 00020 #include "LWIPBP3595Interface.h" 00021 LWIPBP3595Interface wifi; 00022 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00023 #include "EthernetInterface.h" 00024 EthernetInterface eth; 00025 #else 00026 #error "No connectivity method chosen. Please add 'config.network-interfaces.value' to your mbed_app.json (see README.md for more information)." 00027 #endif 00028 00029 // This is address to mbed Device Connector 00030 #define MBED_SERVER_ADDRESS "coap://api.connector.mbed.com:5684" 00031 00032 #ifdef MBED_CONF_APP_ESP8266_SSID 00033 #define MBED_CONF_APP_WIFI_SSID MBED_CONF_APP_ESP8266_SSID 00034 #endif 00035 00036 #ifdef MBED_CONF_APP_ESP8266_PASSWORD 00037 #define MBED_CONF_APP_WIFI_PASSWORD MBED_CONF_APP_ESP8266_PASSWORD 00038 #endif 00039 00040 #ifndef MBED_CONF_APP_WIFI_SECURITY 00041 #define MBED_CONF_APP_WIFI_SECURITY NSAPI_SECURITY_WPA_WPA2 00042 #endif 00043 00044 NetworkInterface* easy_connect(bool log_messages = false) { 00045 NetworkInterface* network_interface = 0; 00046 int connect_success = -1; 00047 #if MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ESP8266 00048 if (log_messages) { 00049 printf("[EasyConnect] Using WiFi (ESP8266) \n"); 00050 printf("[EasyConnect] Connecting to WiFi %s\n", MBED_CONF_APP_WIFI_SSID); 00051 } 00052 connect_success = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, MBED_CONF_APP_WIFI_SECURITY); 00053 network_interface = &wifi; 00054 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_BP3595 00055 if (log_messages) { 00056 printf("[EasyConnect] Using WiFi (BP3595) \n"); 00057 printf("[EasyConnect] Connecting to WiFi %s\n", MBED_CONF_APP_WIFI_SSID); 00058 } 00059 connect_success = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, MBED_CONF_APP_WIFI_SECURITY); 00060 network_interface = &wifi; 00061 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00062 if (log_messages) { 00063 printf("[EasyConnect] Using Ethernet\n"); 00064 } 00065 connect_success = eth.connect(); 00066 network_interface = ð 00067 #endif 00068 if(connect_success == 0) { 00069 if (log_messages) { 00070 printf("[EasyConnect] Connected to Network successfully\n"); 00071 } 00072 } else { 00073 if (log_messages) { 00074 printf("[EasyConnect] Connection to Network Failed %d!\n", connect_success); 00075 } 00076 return NULL; 00077 } 00078 const char *ip_addr = network_interface->get_ip_address(); 00079 const char *mac_addr = network_interface->get_mac_address(); 00080 if (ip_addr == NULL) { 00081 if (log_messages) { 00082 printf("[EasyConnect] ERROR - No IP address\n"); 00083 } 00084 return NULL; 00085 } 00086 if (mac_addr == NULL) { 00087 if (log_messages) { 00088 printf("[EasyConnect] ERROR - No MAC address\n"); 00089 } 00090 return NULL; 00091 } 00092 if (log_messages) { 00093 printf("[EasyConnect] IP address %s\n", ip_addr); 00094 printf("[EasyConnect] MAC address %s\n", mac_addr); 00095 } 00096 return network_interface; 00097 } 00098 00099 #endif // __MAGIC_CONNECT_H__
Generated on Tue Jul 12 2022 21:31:45 by
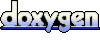