
This is a sample using ESP32 AT command for BLE.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ESP32.h" 00003 00004 #define BLE_NOTIFICATION_CHAR 6 00005 #define BLE_INDICATION_CHAR 7 00006 00007 BufferedSerial esp32(P7_1, P0_1, 1024); 00008 ATParser_os esp_parser(esp32); 00009 Serial pc(USBTX, USBRX); // tx, rx 00010 DigitalOut esp_en(P5_3); 00011 DigitalOut esp_io0(P3_14); 00012 DigitalOut led_green(LED1); 00013 DigitalOut led_yellow(LED2); 00014 DigitalOut led_orange(LED3); 00015 DigitalOut led_red(LED4); 00016 InterruptIn button0(USER_BUTTON0); 00017 InterruptIn button1(USER_BUTTON1); 00018 Semaphore event_sem(0); 00019 00020 const char ble_name[] = "GR-LYCHEE"; 00021 bool ble_advertizing; 00022 bool ble_connected; 00023 uint8_t ble_conn_index; 00024 uint8_t ble_srv_index; 00025 00026 bool button0_flag = false; 00027 bool button1_flag = false; 00028 bool ble_notification_on = false; 00029 bool ble_indication_on = false; 00030 00031 void esp32_event() { 00032 event_sem.release(); 00033 } 00034 00035 void ble_client_read() { 00036 uint8_t mac[6] = {0}; 00037 00038 led_yellow = 1; 00039 esp_parser.recv("%hhd,\"%hhx:%hhx:%hhx:%hhx:%hhx:%hhx\"", 00040 &ble_conn_index, &mac[0], &mac[1], &mac[2], &mac[3], &mac[4], &mac[5]); 00041 printf("conn_index=%d, mac[%x:%x:%x:%x:%x:%x]\r\n", 00042 ble_conn_index, mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]); 00043 led_yellow = 0; 00044 } 00045 00046 void ble_client_write() { 00047 uint8_t char_index, desc_index = 0; 00048 uint16_t len; 00049 00050 led_orange = 1; 00051 esp_parser.recv("%hhd,%hhd,%hhd,", &ble_conn_index, &ble_srv_index, &char_index); 00052 printf("conn=%d srv=%d char=%d\r\n", ble_conn_index, ble_srv_index, char_index); 00053 00054 char c = esp_parser.getc(); 00055 if (c != ',') { 00056 desc_index = c; 00057 esp_parser.getc(); // to read ',' after desc_index. 00058 printf("desc=%d\r\n", desc_index); 00059 } 00060 00061 esp_parser.recv("%hhd,", &len); 00062 printf("length=%d\r\n", len); 00063 00064 uint8_t *data = (uint8_t *)malloc(len * sizeof(uint8_t)); 00065 for (int i = 0; i < len; i++) { 00066 data[i] = esp_parser.getc(); 00067 printf("%x\r\n", data[i]); 00068 } 00069 00070 if ((desc_index == 49) && (char_index == BLE_NOTIFICATION_CHAR)) { 00071 if (data[0] == 1) { 00072 printf("Notification On\r\n"); 00073 ble_notification_on = true; 00074 } else { 00075 printf("Notification Off\r\n"); 00076 ble_notification_on = false; 00077 } 00078 } else if ((desc_index == 49) && (char_index == BLE_INDICATION_CHAR)) { 00079 if (data[0] == 2) { 00080 printf("Indication On\r\n"); 00081 ble_indication_on = true; 00082 } else { 00083 printf("Indication Off\r\n"); 00084 ble_indication_on = false; 00085 } 00086 } 00087 led_orange = 0; 00088 } 00089 00090 void ble_client_disconn() { 00091 led_green = 0; 00092 printf("disconnected client\r\n"); 00093 ble_connected = false; 00094 } 00095 00096 void ble_client_conn() { 00097 uint8_t mac[6] = {0}; 00098 esp_parser.recv("%hhd,\"%hhx:%hhx:%hhx:%hhx:%hhx:%hhx\"", 00099 &ble_conn_index, &mac[0], &mac[1], &mac[2], &mac[3], &mac[4], &mac[5]); 00100 printf("connected client conn_index is %d, mac[%x:%x:%x:%x:%x:%x]\r\n", 00101 ble_conn_index, mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]); 00102 00103 led_green = 1; 00104 ble_advertizing = false; 00105 ble_connected = true; 00106 } 00107 00108 void ub0_interrupt() { 00109 if (button0_flag == false) { 00110 button0_flag = true; 00111 event_sem.release(); 00112 } 00113 } 00114 00115 void ub1_interrupt() { 00116 if (button1_flag == false) { 00117 button1_flag = true; 00118 event_sem.release(); 00119 } 00120 } 00121 00122 int main() { 00123 00124 printf("Bluetooth sample started\r\n"); 00125 led_green = 0; 00126 led_yellow = 0; 00127 led_orange = 0; 00128 led_red = 0; 00129 ble_advertizing = false; 00130 ble_connected = false; 00131 button0.fall(ub0_interrupt); 00132 button1.fall(ub1_interrupt); 00133 00134 // Initializing esp32 access 00135 esp32.baud(115200); 00136 esp32.attach(Callback<void()>(esp32_event)); 00137 esp_io0 = 1; 00138 esp_en = 0; 00139 Thread::wait(10); 00140 esp_en = 1; 00141 esp_parser.setTimeout(1500); 00142 if (esp_parser.recv("ready")) { 00143 printf("ESP32 ready\r\n"); 00144 } else { 00145 printf("ESP32 error\r\n"); 00146 led_red = 1; 00147 while (1); 00148 } 00149 00150 // Initializing esp32 as a server with GATT service 00151 esp_parser.setTimeout(5000); 00152 if (esp_parser.send("AT+BLEINIT=2") && esp_parser.recv("OK") 00153 && esp_parser.send("AT+BLENAME=\"%s\"", ble_name) && esp_parser.recv("OK") 00154 && esp_parser.send("AT+BLEGATTSSRVCRE") && esp_parser.recv("OK") 00155 && esp_parser.send("AT+BLEGATTSSRVSTART") && esp_parser.recv("OK")) { 00156 printf("GATT initialized\r\n"); 00157 } else { 00158 printf("fail to initialize\r\n"); 00159 led_red = 1; 00160 while (1); 00161 } 00162 00163 uint8_t start, type; 00164 uint16_t uuid; 00165 esp_parser.send("AT+BLEGATTSSRV?"); 00166 if (esp_parser.recv("+BLEGATTSSRV:%hhd,%hhd,%hhx,%hhd\r\nOK", &ble_srv_index, &start, &uuid, &type)) { 00167 printf("srv_index is %d\r\n", ble_srv_index); 00168 } else { 00169 printf("fail to get service\r\n"); 00170 led_red = 1; 00171 } 00172 00173 esp_parser.oob("+READ:", ble_client_read); 00174 esp_parser.oob("+WRITE:", ble_client_write); 00175 esp_parser.oob("+BLEDISCONN:", ble_client_disconn); 00176 esp_parser.oob("+BLECONN:", ble_client_conn); 00177 00178 while (1) { 00179 esp_parser.setTimeout(5000); 00180 if (esp_parser.send("AT+BLEADVSTART") && esp_parser.recv("OK")) { 00181 printf("Advertising started. Please connect to any client\r\n"); 00182 ble_advertizing = true; 00183 } else { 00184 printf("fail to start advertising\r\n"); 00185 led_red = 1; 00186 while (1); 00187 } 00188 00189 while (ble_connected || ble_advertizing) { 00190 event_sem.wait(); 00191 esp_parser.setTimeout(5); 00192 esp_parser.recv(" "); //dummy read for parser callback 00193 00194 // Set attribute of C300 00195 if (button0_flag) { 00196 static uint8_t data = 1; // write data 00197 esp_parser.setTimeout(5000); 00198 // AT+BLEGATTSSETATTR=<srv_index>,<char_index>[,<desc_index>],<length> 00199 if (esp_parser.send("AT+BLEGATTSSETATTR=%d,1,,1", ble_srv_index) && esp_parser.recv(">")) { 00200 if (esp_parser.putc(data) && esp_parser.recv("OK")) { 00201 printf("success to send\r\n"); 00202 } else { 00203 printf("fail to send\r\n"); 00204 } 00205 } else { 00206 printf("fail to command AT\r\n"); 00207 } 00208 esp_parser.flush(); 00209 button0_flag = false; 00210 data++; 00211 } 00212 00213 // Set notification of C305 00214 if (button1_flag && ble_notification_on) { 00215 static uint8_t data = 0xff; // write data 00216 esp_parser.setTimeout(5000); 00217 // AT+BLEGATTSNTFY=<conn_index>,<srv_index>,<char_index>,<length> 00218 if (esp_parser.send("AT+BLEGATTSNTFY=%d,%d,%d,1", ble_conn_index, ble_srv_index, BLE_NOTIFICATION_CHAR) 00219 && esp_parser.recv(">")) { 00220 if (esp_parser.putc(data) && esp_parser.recv("OK")) { 00221 printf("success to notify\r\n"); 00222 } else { 00223 printf("fail to notify\r\n"); 00224 } 00225 } else { 00226 printf("fail to command AT\r\n"); 00227 } 00228 esp_parser.flush(); 00229 button1_flag = false; 00230 data--; 00231 } 00232 } 00233 } 00234 }
Generated on Sun Jul 17 2022 03:48:06 by
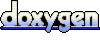