
For RevB MBED Hardware, with the new boot sequence. This example establishes a transparent link between the mbed USB CDC port and the modem (LISA) on the C027. You can use it to use the standard u-blox tools such as m-center or any terminal program. These tools can then connect to the serial port and talk directly to the modem. Baudrate should be set to 115200 baud and is fixed. m-center can be downloaded from u-blox website following this link: http://www.u-blox.com/en/evaluation-tools-a-software/u-center/m-center.html
Dependencies: C027-REVB UbloxUSBModem mbed
main.cpp
00001 #include "mbed.h" 00002 #include "C027.h" 00003 #include "WANDongle.h" 00004 #include "USBSerialStream.h" 00005 #include "UbloxCDMAModemInitializer.h" 00006 #include "UbloxGSMModemInitializer.h" 00007 00008 DigitalOut mdm_activity(LED); 00009 00010 int main() 00011 { 00012 int led_toggle_count = 5; 00013 00014 // the instantiation of the type, calls C027::C027 00015 C027 c027; 00016 00017 c027.mdmPower(true,false); 00018 00019 // enable the GPS, and connect it 00020 // to the m3. 00021 c027.gpsPower(true, false); 00022 00023 #if 0 00024 while(1) { 00025 mdm_activity = !mdm_activity; 00026 wait(0.2); 00027 } 00028 #else 00029 while( led_toggle_count-- > 0 ) 00030 { 00031 mdm_activity = !mdm_activity; 00032 wait(0.2); 00033 } 00034 #endif 00035 00036 // open the mdm USB port 00037 WANDongle mdmDongle; 00038 USBSerialStream mdmStream(mdmDongle.getSerial(1/* the CDC usually 0 or 1, LISA-C requires CDC1*/)); 00039 USBHost* host = USBHost::getHostInst(); 00040 mdmDongle.addInitializer(new UbloxCDMAModemInitializer(host)); 00041 mdmDongle.addInitializer(new UbloxGSMModemInitializer(host)); 00042 00043 // open the PC serial port. 00044 Serial pc(USBTX, USBRX); 00045 pc.baud(MDMBAUD); 00046 00047 pc.printf( "M3->USB CDC connection ready\r\n"); 00048 00049 while (1) 00050 { 00051 uint8_t buf[64]; 00052 size_t len; 00053 int i; 00054 00055 if (!mdmDongle.connected()) 00056 { 00057 mdmDongle.tryConnect(); 00058 } 00059 else 00060 { 00061 00062 // transfer data from pc to modem 00063 len = mdmStream.space(); 00064 if (len>0) 00065 { 00066 if (len > sizeof(buf)) 00067 len = sizeof(buf); 00068 for (i = 0; (i < len) && pc.readable(); ) 00069 buf[i++] = pc.getc(); 00070 if (OK == mdmStream.write(buf, i)) 00071 /* do something? */; 00072 } 00073 // transfer data from modem to pc 00074 len = mdmStream.available(); 00075 if ((len>0) && pc.writeable()) 00076 { 00077 if (len > sizeof(buf)) 00078 len = sizeof(buf); 00079 if (OK == mdmStream.read(buf, &len, len)) 00080 { 00081 for (i = 0; (i < len); ) 00082 { 00083 mdm_activity=!mdm_activity; 00084 pc.putc(buf[i++]); 00085 } 00086 00087 // default the led to off 00088 mdm_activity=0; 00089 } 00090 } 00091 } 00092 } 00093 }
Generated on Thu Jul 14 2022 10:31:13 by
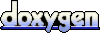