
A dedictaed demo for ra8875
Dependencies: DmTftLibrary mbed
main.cpp
00001 /********************************************************************************************** 00002 Copyright (c) 2014 DisplayModule. All rights reserved. 00003 00004 Redistribution and use of this source code, part of this source code or any compiled binary 00005 based on this source code is permitted as long as the above copyright notice and following 00006 disclaimer is retained. 00007 00008 DISCLAIMER: 00009 THIS SOFTWARE IS SUPPLIED "AS IS" WITHOUT ANY WARRANTIES AND SUPPORT. DISPLAYMODULE ASSUMES 00010 NO RESPONSIBILITY OR LIABILITY FOR THE USE OF THE SOFTWARE. 00011 00012 The GFX_TEST function is based on UTFT_Demo (http://www.henningkarlsen.com/electronics/) 00013 ********************************************************************************************/ 00014 00015 /****************************************************************************** 00016 * Includes 00017 *****************************************************************************/ 00018 00019 #include "mbed.h" 00020 00021 00022 #include "DmTftRa8875.h" 00023 #include "DmTouch.h" 00024 00025 /****************************************************************************** 00026 * Typedefs and defines 00027 *****************************************************************************/ 00028 00029 /* Note that there are restrictions on which platforms that can use printf 00030 in combinations with the DmTftLibrary. Some platforms (e.g. LPC1549 LPCXpresso) 00031 use the same pins for USBRX/USBTX and display control. Printing will 00032 cause the display to not work. Read more about this on the display's notebook 00033 page. */ 00034 //#define log(...) printf(__VA_ARGS__) 00035 #define log(...) 00036 00037 #if 1 00038 /* Displays without adapter */ 00039 #define DM_PIN_SPI_MOSI D11 00040 #define DM_PIN_SPI_MISO D12 00041 #define DM_PIN_SPI_SCLK D13 00042 #define DM_PIN_CS_TOUCH D4 00043 #define DM_PIN_CS_TFT D10 00044 #define DM_PIN_CS_SDCARD D8 00045 #define DM_PIN_CS_FLASH D6 00046 #else 00047 /* Displays with adapter */ 00048 #define DM_PIN_SPI_MOSI A0 00049 #define DM_PIN_SPI_MISO D9 00050 #define DM_PIN_SPI_SCLK A1 00051 #define DM_PIN_CS_TOUCH D8 00052 #define DM_PIN_CS_TFT A3 00053 #define DM_PIN_CS_SDCARD D10 00054 #endif 00055 00056 /****************************************************************************** 00057 * Local variables 00058 *****************************************************************************/ 00059 DmTftRa8875 tft; /* DM_TFT50_111 */ 00060 00061 //For DmTftRa8875 driver, The panel resolution should be config in DmTftRa8875::init() function on the DmTftRa8875.cpp file. 00062 DmTouch touch(DmTouch::DM_TFT50_111); 00063 00064 DigitalInOut csTouch(DM_PIN_CS_TOUCH, PIN_OUTPUT, PullUp, 1); 00065 DigitalInOut csDisplay(DM_PIN_CS_TFT, PIN_OUTPUT, PullUp, 1); 00066 DigitalInOut csSDCard(DM_PIN_CS_SDCARD, PIN_OUTPUT, PullUp, 1); 00067 #ifdef DM_PIN_CS_FLASH 00068 DigitalInOut csFlash(DM_PIN_CS_FLASH, PIN_OUTPUT, PullUp, 1); 00069 #endif 00070 00071 /****************************************************************************** 00072 * Global variables 00073 *****************************************************************************/ 00074 unsigned int m_z=12434,m_w=33254; 00075 /****************************************************************************** 00076 * Local functions 00077 *****************************************************************************/ 00078 unsigned int rnd() 00079 { 00080 m_z = 36969 * (m_z & 65535) + (m_z >>16); 00081 m_w = 18000 * (m_w & 65535) + (m_w >>16); 00082 return ((m_z <<16) + m_w); 00083 } 00084 00085 void GFX_TEST() 00086 { 00087 uint16_t x = 0; 00088 uint16_t y = 0; 00089 uint16_t w = tft.width(); 00090 uint16_t h = tft.height(); 00091 int buf[798]; 00092 00093 int x0, x1; 00094 int y0, y1; 00095 int r; 00096 uint16_t color; 00097 00098 tft.clearScreen(BLACK); 00099 tft.fillRectangle(0, 0, 799, 19, RED); 00100 tft.fillRectangle(0, 460, 799, 479, GRAY2); 00101 tft.setFontColor(RED, WHITE); 00102 tft.drawStringCentered(0, 0, 800, 20, "*** Ra8875 demo ***"); 00103 tft.setFontColor(GRAY2, YELLOW); 00104 tft.drawStringCentered(0, 460, 800, 20, "www.displaymodule.com"); 00105 00106 tft.drawRectangle(0, 20, 799, 459, BLUE); 00107 tft.drawLine(399, 21, 399, 458, BLUE); 00108 tft.drawLine(1, 239, 798, 239, BLUE); 00109 for (int i=9; i<790; i+=10) 00110 tft.drawVerticalLine(i, 234, 10, BLUE); 00111 for (int i=29; i<450; i+=10) 00112 tft.drawHorizontalLine(395, i, 10, BLUE); 00113 00114 tft.setFontColor(BLACK, RED); 00115 tft.drawString(5, 25, "Sin"); 00116 00117 for (int i=1; i<798; i++) { 00118 tft.drawPoint(i,239+(sin(((i*1.13)*3.14)/90)*95)); 00119 wait_ms(1); 00120 } 00121 wait(1); 00122 tft.setFontColor(BLACK, YELLOW); 00123 tft.drawString(5, 45, "Cos"); 00124 00125 for (int i=1; i<798; i++) { 00126 tft.drawPoint(i,239+(cos(((i*1.13)*3.14)/90)*95)); 00127 wait_ms(1); 00128 } 00129 wait(1.0); 00130 tft.setFontColor(BLACK, GREEN); 00131 tft.drawString(5, 65, "Tan"); 00132 00133 for (int i=1; i<798; i++) { 00134 tft.drawPoint(i,239+(tan(((i*1.13)*3.14)/90))); 00135 wait_ms(1); 00136 } 00137 00138 tft.fillRectangle(1, 21, 798, 458, BLACK); 00139 tft.drawLine(399, 22, 399, 458, BLUE); 00140 tft.drawLine(1, 239, 798, 239, BLUE); 00141 00142 // Draw a moving sinewave 00143 x=1; 00144 for (int i=1; i<(798*20); i++) { 00145 x++; 00146 if (x==799) 00147 x=1; 00148 if (i>799) { 00149 if ((x==399)||(buf[x-1]==239)) 00150 tft.setFontColor(BLACK, BLUE); 00151 else 00152 tft.setFontColor(BLACK, BLACK); 00153 tft.drawPoint(x,buf[x-1]); 00154 } 00155 tft.setFontColor(BLACK, 0x07FF); 00156 y=239+(sin(((i*1.13)*3.14)/90)*(90-(i / 100))); 00157 tft.drawPoint(x,y); 00158 wait_ms(1); 00159 buf[x-1]=y; 00160 } 00161 00162 00163 wait(1.0); 00164 tft.fillRectangle(1, 21, 798, 458, BLACK); 00165 // Draw some filled rectangles 00166 for (int i=1; i<6; i++) { 00167 wait_ms(20); 00168 switch (i) { 00169 case 1: 00170 tft.fillRectangle(70+(i*50), 30+(i*50), 220+(i*50), 180+(i*50), 0xF81F); 00171 break; 00172 case 2: 00173 tft.fillRectangle(70+(i*50), 30+(i*50), 220+(i*50), 180+(i*50), RED); 00174 break; 00175 case 3: 00176 tft.fillRectangle(70+(i*50), 30+(i*50), 220+(i*50), 180+(i*50),GREEN); 00177 break; 00178 case 4: 00179 tft.fillRectangle(70+(i*50), 30+(i*50), 220+(i*50), 180+(i*50), BLUE); 00180 break; 00181 case 5: 00182 tft.fillRectangle(70+(i*50), 30+(i*50), 220+(i*50), 180+(i*50),YELLOW); 00183 break; 00184 } 00185 00186 } 00187 00188 wait(1.0); 00189 tft.fillRectangle(1, 21, 798, 458, BLACK); 00190 // Draw some filled, rounded rectangles 00191 for (int i=1; i<6; i++) { 00192 wait_ms(20); 00193 switch (i) { 00194 case 1: 00195 tft.fillRoundRectangle(570-(i*50), 30+(i*50),720-(i*50), 180+(i*50), 10, 10, 0xF81F); 00196 break; 00197 case 2: 00198 tft.fillRoundRectangle(570-(i*50), 30+(i*50), 720-(i*50), 180+(i*50), 10, 10, RED); 00199 break; 00200 case 3: 00201 tft.fillRoundRectangle(570-(i*50), 30+(i*50), 720-(i*50), 180+(i*50), 10, 10, GREEN); 00202 break; 00203 case 4: 00204 tft.fillRoundRectangle(570-(i*50), 30+(i*50), 720-(i*50), 180+(i*50), 10, 10, BLUE); 00205 break; 00206 case 5: 00207 tft.fillRoundRectangle(570-(i*50), 30+(i*50), 720-(i*50), 180+(i*50), 10, 10, YELLOW); 00208 break; 00209 } 00210 } 00211 00212 wait(1.0); 00213 tft.fillRectangle(1, 21, 798, 458, BLACK); 00214 // Draw some filled, circles 00215 for (int i=1; i<6; i++) { 00216 wait_ms(20); 00217 switch (i) { 00218 case 1: 00219 tft.fillCircle(70+(i*50), 70+(i*50), 75, 0xF81F); 00220 break; 00221 case 2: 00222 tft.fillCircle(70+(i*50), 70+(i*50), 75, RED); 00223 break; 00224 case 3: 00225 tft.fillCircle(70+(i*50), 70+(i*50), 75, GREEN); 00226 break; 00227 case 4: 00228 tft.fillCircle(70+(i*50), 70+(i*50), 75, BLUE); 00229 break; 00230 case 5: 00231 tft.fillCircle(70+(i*50), 70+(i*50), 75, YELLOW); 00232 break; 00233 } 00234 } 00235 00236 wait(1.0); 00237 tft.fillRectangle(1, 21, 798, 458, BLACK); 00238 // Draw some filled, ellipses 00239 for (int i=1; i<6; i++) { 00240 wait_ms(20); 00241 switch (i) { 00242 case 1: 00243 tft.fillEllipse(700-(i*50), 70+(i*50), 75, 40, 0xF81F); 00244 break; 00245 case 2: 00246 tft.fillEllipse(700-(i*50), 70+(i*50), 75, 40, RED); 00247 break; 00248 case 3: 00249 tft.fillEllipse(700-(i*50), 70+(i*50), 75, 40, GREEN); 00250 break; 00251 case 4: 00252 tft.fillEllipse(700-(i*50), 70+(i*50), 75, 40, BLUE); 00253 break; 00254 case 5: 00255 tft.fillEllipse(700-(i*50), 70+(i*50), 75, 40, YELLOW); 00256 break; 00257 } 00258 } 00259 00260 wait(1.0); 00261 tft.fillRectangle(1, 21, 798, 458, BLACK); 00262 // Draw some filled, triangles 00263 for (int i=1; i<6; i++) { 00264 wait_ms(20); 00265 switch (i) { 00266 case 1: 00267 tft.fillTriangle(399, 50+(i*50), 249, 150+(i*50), 549, 150+(i*50), 0xF81F); 00268 break; 00269 case 2: 00270 tft.fillTriangle(399, 50+(i*50), 249, 150+(i*50), 549, 150+(i*50), RED); 00271 break; 00272 case 3: 00273 tft.fillTriangle(399, 50+(i*50), 249, 150+(i*50), 549, 150+(i*50), GREEN); 00274 break; 00275 case 4: 00276 tft.fillTriangle(399, 50+(i*50), 249, 150+(i*50), 549, 150+(i*50), BLUE); 00277 break; 00278 case 5: 00279 tft.fillTriangle(399, 50+(i*50), 249, 150+(i*50), 549, 150+(i*50), YELLOW); 00280 break; 00281 } 00282 } 00283 00284 wait(1.0); 00285 tft.fillRectangle(1, 21, 798, 458, BLACK); 00286 // Draw some lines in a pattern 00287 00288 for (int i=21; i<458; i+=5) { 00289 tft.drawLine(1, i, (i*1.82)-31, 458, RED); 00290 wait_ms(20); 00291 } 00292 for (int i=458; i>21; i-=5) { 00293 tft.drawLine(798, i, (i*1.82)-35, 21, RED); 00294 wait_ms(20); 00295 } 00296 00297 for (int i=458; i>21; i-=5) { 00298 tft.drawLine(1, i, 835-(i*1.82), 21,CYAN); 00299 wait_ms(20); 00300 } 00301 for (int i=21; i<458; i+=5) { 00302 tft.drawLine(798, i, 831-(i*1.82), 458,CYAN); 00303 wait_ms(20); 00304 } 00305 00306 wait(1.0); 00307 tft.fillRectangle(1, 21, 798, 458, BLACK); 00308 00309 00310 // Draw some random circles 00311 for (int i=0; i<100; i++) { 00312 color = ((rnd()%255 >> 3) << 11) | ((rnd()%255 >> 2) << 5) | ((rnd()%255 >> 3)); 00313 x0=62+rnd()%650; 00314 y0=85+rnd()%300; 00315 r=1+ rnd()%60; 00316 tft.fillCircle(x0, y0, r, color); 00317 wait_ms(40); 00318 } 00319 00320 wait(1.0); 00321 tft.fillRectangle(1, 21, 798, 458, BLACK); 00322 // Draw some random rectangles 00323 for (int i=0; i<100; i++) { 00324 color = ((rnd()%255 >> 3) << 11) | ((rnd()%255 >> 2) << 5) | ((rnd()%255 >> 3)); 00325 x0=2+rnd()%796; 00326 y0=25+rnd()%420; 00327 x1=2+rnd()%796; 00328 y1=25+rnd()%420; 00329 tft.drawRectangle(x0, y0, x1, y1, color); 00330 wait_ms(40); 00331 } 00332 00333 wait(1.0); 00334 tft.fillRectangle(1, 21, 798, 458, BLACK); 00335 // Draw some random lines 00336 for (int i=0; i<100; i++) { 00337 color = ((rnd()%255 >> 3) << 11) | ((rnd()%255 >> 2) << 5) | ((rnd()%255 >> 3)); 00338 x0=2+rnd()%796; 00339 y0=25+rnd()%420; 00340 x1=2+rnd()%796; 00341 y1=25+rnd()%420; 00342 tft.drawLine(x0, y0, x1, y1, color); 00343 wait_ms(40); 00344 } 00345 00346 00347 wait(1.0); 00348 tft.fillRectangle(1, 21, 798, 458, BLACK); 00349 // Draw some random pixels 00350 for (int i=0; i<5000; i++) { 00351 color = ((rnd()%255 >> 3) << 11) | ((rnd()%255 >> 2) << 5) | ((rnd()%255 >> 3)); 00352 tft.setFontColor(BLACK, color); 00353 x0=2+rnd()%796; 00354 y0=25+rnd()%420; 00355 tft.drawPoint(x0, y0); 00356 } 00357 00358 wait(1.0); 00359 tft.fillRectangle(0, 0, 799, 479, BLUE); 00360 tft.fillRoundRectangle(200, 140, 600, 340, 10, 10, RED); 00361 wait(0.2); 00362 tft.setFontColor(RED, WHITE); 00363 tft.drawStringCentered(200, 140, 400, 200, "Restarting in a few seconds..."); 00364 } 00365 00366 /****************************************************************************** 00367 * Main 00368 *****************************************************************************/ 00369 00370 00371 int main() 00372 { 00373 log("init tft \r\n"); 00374 tft.init(); 00375 touch.init(); 00376 while(1) { 00377 GFX_TEST(); 00378 wait(3); 00379 } 00380 00381 }
Generated on Wed Jul 13 2022 09:50:25 by
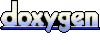