mqtt - potistiriProject
Embed:
(wiki syntax)
Show/hide line numbers
MQTTNetwork.h
00001 00002 #ifndef _MQTTNETWORK_H_ 00003 #define _MQTTNETWORK_H_ 00004 00005 00006 #include "EthernetInterface.h" 00007 00008 class MQTTNetwork { 00009 public: 00010 MQTTNetwork(NetworkInterface* aNetwork) : network(aNetwork) { 00011 socket = new TCPSocket(); 00012 } 00013 00014 ~MQTTNetwork() { 00015 delete socket; 00016 } 00017 00018 int read(unsigned char* buffer, int len, int timeout) { 00019 return socket->recv(buffer, len); 00020 } 00021 00022 int write(unsigned char* buffer, int len, int timeout) { 00023 return socket->send(buffer, len); 00024 } 00025 00026 int connect(const char* hostname, int port) { 00027 /* 00028 socket->open(network); 00029 return socket->connect(hostname, port); 00030 */ 00031 socket->open(network); 00032 network->gethostbyname(hostname,&a); 00033 a.set_port(port); 00034 return socket->connect(a); 00035 } 00036 00037 int disconnect() { 00038 return socket->close(); 00039 } 00040 00041 private: 00042 NetworkInterface* network; 00043 TCPSocket* socket; 00044 SocketAddress a; 00045 }; 00046 00047 00048 #endif // _MQTTNETWORK_H_
Generated on Mon Jul 18 2022 09:22:00 by
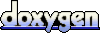