
working version
Fork of foc-ed_in_the_bot_compact by
Embed:
(wiki syntax)
Show/hide line numbers
pwm_in.cpp
00001 #include "pwm_in.h" 00002 #include "mbed.h" 00003 00004 float map(float x, float in_min, float in_max, float out_min, float out_max) 00005 { 00006 return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min; 00007 } 00008 00009 float constrain(float in, float min, float max) 00010 { 00011 if(in > max) return max; 00012 if(in < min) return min; 00013 return in; 00014 } 00015 00016 PWM_IN::PWM_IN(PinName pin, int usec_min, int usec_max) 00017 { 00018 int_in = new InterruptIn(pin); 00019 dig_in = new DigitalIn(pin); 00020 int_in->rise(this, &PWM_IN::handle_rise); 00021 int_in->fall(this, &PWM_IN::handle_fall); 00022 this->usec_min = usec_min; 00023 this->usec_max = usec_max; 00024 } 00025 00026 00027 bool PWM_IN::get_enabled() 00028 { 00029 return enabled; 00030 } 00031 00032 void PWM_IN::handle_rise() 00033 { 00034 enabled = true; 00035 timer.stop(); 00036 timer.reset(); 00037 timer.start(); 00038 was_on = true; 00039 } 00040 00041 void PWM_IN::handle_fall() 00042 { 00043 was_on = false; 00044 usecs = timer.read_us(); 00045 timer.stop(); 00046 timer.reset(); 00047 timer.start(); 00048 } 00049 00050 float PWM_IN::get_throttle() 00051 { 00052 if(timer.read_us() > 40000) enabled = false; 00053 if(!enabled) return -1; 00054 return constrain(map((float)usecs, usec_min, usec_max, 0, 1), 0, 1); 00055 } 00056
Generated on Fri Jul 15 2022 19:19:55 by
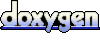