Part of TI's mqtt
Dependents: mqtt_V1 cc3100_Test_mqtt_CM3
mqtt_client.h File Reference
This C library provisions the interface / API(s) for the MQTT Client. More...
Go to the source code of this file.
Data Structures | |
struct | mqtt_client_ctx_cbs |
Callbacks to be invoked by MQTT Client library onto Client application. More... | |
struct | mqtt_client_lib_cfg |
Contruct / Data to initialize MQTT Client Library. More... | |
Functions | |
uint16_t | mqtt_client_new_msg_id (void) |
Provides a new MSG Identifier for a packet dispatch to server. | |
bool | mqtt_client_is_connected (void *ctx) |
Ascertain whether connection / session with the server is active or not. | |
int32_t | mqtt_connect_msg_send (void *ctx, bool clean_session, uint16_t ka_secs) |
Send the CONNECT message to the server (and don't wait for CONNACK). | |
int32_t | mqtt_client_pub_msg_send (void *ctx, const struct utf8_string *topic, const uint8_t *data_buf, uint32_t data_len, enum mqtt_qos qos, bool retain) |
Send a PUBLISH message to the server (don't wait for PUBACK / PUBREC). | |
int32_t | mqtt_client_pub_dispatch (void *ctx, struct mqtt_packet *mqp, enum mqtt_qos qos, bool retain) |
Dispatch application constructed PUBLISH message to the server. | |
int32_t | mqtt_sub_msg_send (void *ctx, const struct utf8_strqos *qos_topics, uint32_t count) |
Send a SUBSCRIBE message to the server (and don't wait for SUBACK). | |
int32_t | mqtt_sub_dispatch (void *ctx, struct mqtt_packet *mqp) |
Dispatch application constructed SUSBSCRIBE message to the server. | |
int32_t | mqtt_unsub_msg_send (void *ctx, const struct utf8_string *topics, uint32_t count) |
Send an UNSUBSCRIBE message to the server (and don't wait for UNSUBACK). | |
int32_t | mqtt_unsub_dispatch (void *ctx, struct mqtt_packet *mqp) |
Dispatch application constructed UNSUSBSCRIBE message to the server. | |
int32_t | mqtt_pingreq_send (void *ctx) |
Send a PINGREQ message to the server (and don't wait for PINGRSP). | |
int32_t | mqtt_disconn_send (void *ctx) |
Send a DISCONNECT message to the server. | |
int32_t | mqtt_client_send_progress (void *ctx) |
Send remaining data or contents of the scheduled message to the server. | |
int32_t | mqtt_client_ctx_await_msg (void *ctx, uint8_t msg_type, struct mqtt_packet *mqp, uint32_t wait_secs) |
Block on the 'context' to receive a message type with-in specified wait time. | |
static int32_t | mqtt_client_ctx_recv (void *ctx, struct mqtt_packet *mqp, uint32_t wait_secs) |
Helper function to receive any message from the server. | |
int32_t | mqtt_client_ctx_run (void *ctx, uint32_t wait_secs) |
Run the context for the specificed wait time. | |
int32_t | mqtt_client_await_msg (struct mqtt_packet *mqp, uint32_t wait_secs, void **app) |
Block to receive any message for the grouped contexts within specified time. | |
int32_t | mqtt_client_run (uint32_t wait_secs) |
Run the LIB for the specified wait time. | |
struct mqtt_packet * | mqp_client_alloc (uint8_t msg_type, uint8_t offset) |
Allocates a free MQTT Packet Buffer. | |
static struct mqtt_packet * | mqp_client_send_alloc (uint8_t msg_type) |
Helper function to allocate a MQTT Packet Buffer for a message to be dispatched to server. | |
static struct mqtt_packet * | mqp_client_recv_alloc (uint8_t msg_type) |
Helper function to allocate a MQTT Packet Buffer for a message to be received from the server. | |
int32_t | mqtt_client_buffers_register (uint32_t num_mqp, struct mqtt_packet *mqp_vec, uint32_t buf_len, uint8_t *buf_vec) |
Create a pool of MQTT Packet Buffers for the client library. | |
int32_t | mqtt_client_ctx_info_register (void *ctx, const struct utf8_string *client_id, const struct utf8_string *user_name, const struct utf8_string *pass_word) |
Register application info and its credentials with the client library. | |
int32_t | mqtt_client_ctx_will_register (void *ctx, const struct utf8_string *will_top, const struct utf8_string *will_msg, enum mqtt_qos will_qos, bool retain) |
Register WILL information of the client application. | |
int32_t | mqtt_client_net_svc_register (const struct device_net_services *net) |
Abstraction for the device specific network services. | |
int32_t | mqtt_client_ctx_create (const struct mqtt_client_ctx_cfg *ctx_cfg, const struct mqtt_client_ctx_cbs *ctx_cbs, void *app, void **ctx) |
Create a Network Connection Context. | |
int32_t | mqtt_client_ctx_delete (void *ctx) |
Delete a Network Connection Context. | |
int32_t | mqtt_client_lib_init (const struct mqtt_client_lib_cfg *cfg) |
Initialize the MQTT client library. | |
int32_t | mqtt_client_lib_exit (void) |
Exit the MQTT client library. |
Detailed Description
This C library provisions the interface / API(s) for the MQTT Client.
This is a light-weight library to enable the services of the MQTT protocol for one or more client applications (that would typically run on a resource constrained system). The key consideration in the design of small footprint library has been the abstraction of the low level details of the message transactions with the server and yet, provide rudimentary API(s), such that, the capabilities and features of the protocol are availalbe to be utilized by existing and new applications in an un-restrictive manner.
The library is targeted to conform to MQTT 3.1.1 specification.
The MQTT Client library is a highly portable software and implies a very limited set of dependencies on a platform. Importantly, these limited dependencies are the commonly used features used in the embedded and the networking world, and can be easily adapted to the target platforms.
The services of the library are multi-task safe. Platform specific atomicity constructs are used, through abstractions, by the library to maintain data coherency and synchronization. In addition, the library can be configured to support several in-flight messages.
The client library supports multiple and simultaneous MQTT connections to one or more servers. In this client LIB, the reference to an individual connection in conjunction with the associated configuration parameters has been termed as a 'context'. Therefore, the client library supports multiple 'contexts'. An application that intends to make use of the client library must set-up or create a 'context' prior to establishing a connection with the server. The application can choose to destroy the 'context' after the connection with the server has been terminated. The client LIB can only support a finite set of 'contexts' and the number can be configured by using a compiler line option / flag -DCFG_CL_MQTT_CTXS .
Once, the 'context' is set-up, the application can send and receive the MQTT packets to / from the server. Among several features supported by the client LIB, the 'context' manages the keep-alive mechanism by automatically sending PING request to the server, if there has been no other packet send to the server with the keep-alive duration.
- Note:
- Any future extensions & development must follow the following guidelines. A new API or an extension to the existing API a) must be rudimentary b) must not imply a rule or policy (including a state machine) b) must ensure simple design and implementation
Definition in file mqtt_client.h.
Generated on Fri Jul 15 2022 07:38:03 by
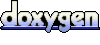