
TI's CC3100 host driver and demo. Experimental and a work in progress.
Embed:
(wiki syntax)
Show/hide line numbers
cc3100_simplelink.h
00001 /* 00002 * simplelink.h - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 /*! 00039 \mainpage SimpleLink Driver 00040 00041 \section intro_sec Introduction 00042 00043 The SimpleLink CC31xx/CC2xx family allows to add Wi-Fi and networking capabilities 00044 to low-cost embedded products without having prior Wi-Fi, RF or networking expertise. 00045 The CC31xx/CC32xx is an ideal solution for microcontroller-based sensor and control 00046 applications such as home appliances, home automation and smart metering. 00047 The CC31xx/CC32xx has integrated a comprehensive TCP/IP network stack, Wi-Fi driver and 00048 security supplicant leading to easier portability to microcontrollers, to an 00049 ultra-low memory footprint, all without compromising the capabilities and robustness 00050 of the final application. 00051 00052 00053 00054 \section modules_sec Module Names 00055 To make it simple, TI's SimpleLink CC31xx/CC32xx platform capabilities were divided into modules by topic (Silo). 00056 These capabilities range from basic device management through wireless 00057 network configuration, standard BSD socket and much more. 00058 Listed below are the various modules in the SimpleLink CC31xx/CC32xx driver: 00059 -# \ref device - controls the behaviour of the CC31xx/CC32xx device (start/stop, events masking and obtaining specific device status) 00060 -# \ref wlan - controls the use of the WiFi WLAN module including: 00061 - Connection features, such as: profiles, policies, SmartConfig� 00062 - Advanced WLAN features, such as: scans, rx filters and rx statistics collection 00063 -# \ref socket - controls standard client/server sockets programming options and capabilities 00064 -# \ref netapp - activates networking applications, such as: HTTP Server, DHCP Server, Ping, DNS and mDNS. 00065 -# \ref netcfg - controls the configuration of the device addresses (i.e. IP and MAC addresses) 00066 -# \ref FileSystem - provides file system capabilities to TI's CC31XX that can be used by both the CC31XX device and the user. 00067 00068 00069 \section proting_sec Porting Guide 00070 00071 The porting of the SimpleLink driver to any new platform is based on few simple steps. 00072 This guide takes you through this process step by step. Please follow the instructions 00073 carefully to avoid any problems during this process and to enable efficient and proper 00074 work with the device. 00075 Please notice that all modifications and porting adjustments of the driver should be 00076 made in the user.h header file only. 00077 Keep making any of the changes only in this file will ensure smoothly transaction to 00078 new versions of the driver at the future! 00079 00080 00081 \subsection porting_step1 Step 1 - Create your own user.h file 00082 00083 The first step is to create a user.h file that will include your configurations and 00084 adjustments. You can use the empty template provided as part of this driver or 00085 you can choose to base your file on file from one of the wide range of examples 00086 applications provided by Texas Instruments 00087 00088 00089 \subsection porting_step2 Step 2 - Select the capabilities set required for your application 00090 00091 Texas Instruments made a lot of efforts to build set of predefined capability sets that would 00092 fit most of the target application. 00093 It is recommended to try and choose one of this predefined capabilities set before going to 00094 build your own customized set. If you find compatible set you can skip the rest of this step. 00095 00096 The available sets are: 00097 -# SL_TINY - Compatible to be used on platforms with very limited resources. Provides 00098 the best in class foot print in terms of Code and Data consumption. 00099 -# SL_SMALL - Compatible to most common networking applications. Provide the most 00100 common APIs with decent balance between code size, data size, functionality 00101 and performances 00102 -# SL_FULL - Provide access to all SimpleLink functionalities 00103 00104 00105 \subsection porting_step3 Step 3 - Bind the device enable/disable output line 00106 00107 The enable/disable line (nHib) provide mechanism to enter the device into the least current 00108 consumption mode. This mode could be used when no traffic is required (tx/rx). 00109 when this line is not connected to any IO of the host this define should be left empty. 00110 Not connecting this line results in ability to start the driver only once. 00111 00112 00113 \subsection porting_step4 Step 4 - Writing your interface communication driver 00114 00115 The SimpleLink device support several standard communication protocol among SPI and 00116 UART. Depending on your needs and your hardware design, you should choose the 00117 communication channel type. 00118 The interface for this communication channel should include 4 simple access functions: 00119 -# open 00120 -# close 00121 -# read 00122 -# write 00123 00124 The way this driver would be implemented is directly effecting the efficiency and 00125 the performances of the SimpleLink device on this platform. 00126 If your system has DMA you should consider to use it in order to increase the utilization 00127 of the communication channel 00128 If you have enough memory resources you should consider using a buffer to increase the 00129 efficiency of the write operations. 00130 00131 00132 \subsection porting_step5 Step 5 - Choose your memory management model 00133 00134 The SimpleLink driver support two memory models: 00135 -# Static (default) 00136 -# Dynamic 00137 00138 If you choose to work in dynamic model you will have to provide alloc and free functions 00139 to be used by the Simple Link driver otherwise nothing need to be done. 00140 00141 00142 \subsection porting_step6 Step 6 - OS adaptation 00143 00144 The SimpleLink driver could run on two kind of platforms: 00145 -# Non-Os / Single Threaded (default) 00146 -# Multi-Threaded 00147 00148 If you choose to work in multi-threaded environment under operating system you will have to 00149 provide some basic adaptation routines to allow the driver to protect access to resources 00150 for different threads (locking object) and to allow synchronization between threads (sync objects). 00151 In additional the driver support running without dedicated thread allocated solely to the simple 00152 link driver. If you choose to work in this mode, you should also supply a spawn method that 00153 will enable to run function on a temporary context. 00154 00155 00156 \subsection porting_step7 Step 7 - Set your asynchronous event handlers routines 00157 00158 The SimpleLink device generate asynchronous events in several situations. 00159 These asynchronous events could be masked. 00160 In order to catch these events you have to provide handler routines. 00161 Please notice that if you not provide a handler routine and the event is received, 00162 the driver will drop this event without any indication of this drop. 00163 00164 00165 \subsection porting_step8 Step 8 - Run diagnostic tools to validate the correctness of your porting 00166 00167 The driver is delivered with some porting diagnostic tools to simplify the porting validation process 00168 and to reduce issues latter. It is very important to follow carefully this process. 00169 00170 The diagnostic process include: 00171 -# Validating Interface Communication Driver 00172 -# Validating OS adaptation layer 00173 -# Validating HW integrity 00174 -# Validating basic work with the device 00175 00176 00177 \section sw_license License 00178 00179 * 00180 * 00181 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00182 * 00183 * 00184 * Redistribution and use in source and binary forms, with or without 00185 * modification, are permitted provided that the following conditions 00186 * are met: 00187 * 00188 * Redistributions of source code must retain the above copyright 00189 * notice, this list of conditions and the following disclaimer. 00190 * 00191 * Redistributions in binary form must reproduce the above copyright 00192 * notice, this list of conditions and the following disclaimer in the 00193 * documentation and/or other materials provided with the 00194 * distribution. 00195 * 00196 * Neither the name of Texas Instruments Incorporated nor the names of 00197 * its contributors may be used to endorse or promote products derived 00198 * from this software without specific prior written permission. 00199 * 00200 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00201 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00202 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00203 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00204 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00205 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00206 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00207 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00208 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00209 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00210 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00211 * 00212 */ 00213 00214 00215 00216 #ifndef __SIMPLELINK_H__ 00217 #define __SIMPLELINK_H__ 00218 00219 #include "cc3100_user.h" 00220 00221 #ifdef __cplusplus 00222 extern "C" 00223 { 00224 #endif 00225 00226 00227 /*! \attention Async event activation notes 00228 Function prototypes for event callback handlers 00229 Event handler function names should be defined in the user.h file 00230 e.g. 00231 "#define sl_WlanEvtHdlr SLWlanEventHandler" 00232 Indicates all WLAN events are handled by User func "SLWlanEventHandler" 00233 Important notes: 00234 1. Event handlers cannot activate another SimpleLink API from the event's context 00235 2. Event's data is valid during event's context. Any application data 00236 which is required for the user application should be copied or marked 00237 into user's variables 00238 3. It is not recommended to delay the execution of the event callback handler 00239 00240 */ 00241 00242 /*! 00243 00244 \addtogroup UserEvents 00245 @{ 00246 00247 */ 00248 00249 00250 /*****************************************************************************/ 00251 /* Macro declarations for Host Driver version */ 00252 /*****************************************************************************/ 00253 #define SL_DRIVER_VERSION "1.0.0.1" 00254 #define SL_MAJOR_VERSION_NUM 1L 00255 #define SL_MINOR_VERSION_NUM 0L 00256 #define SL_VERSION_NUM 0L 00257 #define SL_SUB_VERSION_NUM 1L 00258 00259 00260 /*****************************************************************************/ 00261 /* Macro declarations for predefined configurations */ 00262 /*****************************************************************************/ 00263 00264 #ifdef SL_TINY 00265 #undef SL_INC_ARG_CHECK 00266 #undef SL_INC_EXT_API 00267 #undef SL_INC_SOCK_CLIENT_SIDE_API 00268 #undef SL_INC_SOCK_SEND_API 00269 #undef SL_INC_WLAN_PKG 00270 #undef SL_INC_NET_APP_PKG 00271 #undef SL_INC_NET_CFG_PKG 00272 #undef SL_INC_FS_PKG 00273 #define SL_INC_SOCK_SERVER_SIDE_API 00274 #define SL_INC_SOCK_RECV_API 00275 #define SL_INC_SOCKET_PKG 00276 #endif 00277 00278 #ifdef SL_SMALL 00279 #undef SL_INC_EXT_API 00280 #undef SL_INC_NET_APP_PKG 00281 #undef SL_INC_NET_CFG_PKG 00282 #undef SL_INC_FS_PKG 00283 #define SL_INC_ARG_CHECK 00284 #define SL_INC_WLAN_PKG 00285 #define SL_INC_SOCKET_PKG 00286 #define SL_INC_SOCK_CLIENT_SIDE_API 00287 #define SL_INC_SOCK_SERVER_SIDE_API 00288 #define SL_INC_SOCK_RECV_API 00289 #define SL_INC_SOCK_SEND_API 00290 #endif 00291 00292 #ifdef SL_FULL 00293 #define SL_INC_EXT_API 00294 #define SL_INC_NET_APP_PKG 00295 #define SL_INC_NET_CFG_PKG 00296 #define SL_INC_FS_PKG 00297 #define SL_INC_ARG_CHECK 00298 #define SL_INC_WLAN_PKG 00299 #define SL_INC_SOCKET_PKG 00300 #define SL_INC_SOCK_CLIENT_SIDE_API 00301 #define SL_INC_SOCK_SERVER_SIDE_API 00302 #define SL_INC_SOCK_RECV_API 00303 #define SL_INC_SOCK_SEND_API 00304 #endif 00305 00306 #define SL_RET_CODE_OK (0) 00307 #define SL_RET_CODE_INVALID_INPUT (-2) 00308 #define SL_RET_CODE_SELF_ERROR (-3) 00309 #define SL_RET_CODE_NWP_IF_ERROR (-4) 00310 #define SL_RET_CODE_MALLOC_ERROR (-5) 00311 00312 #define sl_Memcpy memcpy 00313 #define sl_Memset memset 00314 00315 #define sl_SyncObjClear(pObj) sl_SyncObjWait(pObj,SL_OS_NO_WAIT) 00316 00317 #define SL_MAX_SOCKETS (8) 00318 00319 00320 /*****************************************************************************/ 00321 /* Types definitions */ 00322 /*****************************************************************************/ 00323 typedef void (*_SlSpawnEntryFunc_t)(void* pValue); 00324 00325 #ifndef NULL 00326 #define NULL (0) 00327 #endif 00328 00329 #ifndef FALSE 00330 #define FALSE (0) 00331 #endif 00332 00333 #ifndef TRUE 00334 #define TRUE (!FALSE) 00335 #endif 00336 00337 #ifndef OK 00338 #define OK (0) 00339 #endif 00340 00341 #ifndef _SL_USER_TYPES 00342 #define _u8 unsigned char 00343 #define _i8 signed char 00344 00345 #define _u16 unsigned short 00346 #define _i16 signed short 00347 00348 #define _u32 unsigned long 00349 #define _i32 signed long 00350 #define _volatile volatile 00351 #define _const const 00352 #endif 00353 00354 typedef _u16 _SlOpcode_t; 00355 typedef _u8 _SlArgSize_t; 00356 typedef _i16 _SlDataSize_t; 00357 typedef _i16 _SlReturnVal_t; 00358 00359 #ifdef __cplusplus 00360 } 00361 #endif /* __cplusplus */ 00362 00363 00364 00365 /*****************************************************************************/ 00366 /* Include files */ 00367 /*****************************************************************************/ 00368 00369 #ifdef SL_PLATFORM_MULTI_THREADED 00370 #include "cc3100_spawn.h" 00371 #else 00372 #include "cc3100_nonos.h" 00373 #endif 00374 00375 00376 /* 00377 objInclusion.h and user.h must be included before all api header files 00378 objInclusion.h must be the last arrangement just before including the API header files 00379 since it based on the other configurations to decide which object should be included 00380 */ 00381 #include "cc3100_objInclusion.h" 00382 #include "cc3100_trace.h" 00383 #include "cc3100_fs.h" 00384 #include "cc3100_socket.h" 00385 #include "cc3100_netapp.h" 00386 #include "cc3100_wlan.h" 00387 #include "cc3100_device.h" 00388 #include "cc3100_netcfg.h" 00389 #include "cc3100_wlan_rx_filters.h" 00390 00391 #ifdef __cplusplus 00392 extern "C" { 00393 #endif 00394 00395 00396 00397 /* Async functions description*/ 00398 00399 /*! 00400 \brief General async event for inspecting general events 00401 00402 \param[out] pSlDeviceEvent pointer to SlDeviceEvent_t 00403 00404 \par 00405 Parameters: \n 00406 <b>pSlDeviceEvent->Event = SL_DEVICE_FATAL_ERROR_EVENT </b> 00407 - pSlDeviceEvent->EventData.deviceEvent fields: 00408 - status: An error code indication from the device 00409 - sender: The sender originator which is based on SlErrorSender_e enum 00410 00411 \par Example: 00412 \code 00413 printf(General Event Handler - ID=%d Sender=%d\n\n", 00414 pSlDeviceEvent->EventData.deviceEvent.status, // status of the general event 00415 pSlDeviceEvent->EventData.deviceEvent.sender); // sender type 00416 \endcode 00417 */ 00418 #if (defined(sl_GeneralEvtHdlr)) 00419 extern void sl_GeneralEvtHdlr(SlDeviceEvent_t *pSlDeviceEvent); 00420 #endif 00421 00422 00423 /*! 00424 \brief WLAN Async event handler 00425 00426 \param[out] pSlWlanEvent pointer to SlWlanEvent_t data 00427 00428 \par 00429 Parameters: 00430 00431 - <b>pSlWlanEvent->Event = SL_WLAN_CONNECT_EVENT </b>, STA or P2P client connection indication event 00432 - pSlWlanEvent->EventData.STAandP2PModeWlanConnected main fields: 00433 - ssid_name 00434 - ssid_len 00435 - bssid 00436 - go_peer_device_name 00437 - go_peer_device_name_len 00438 00439 - <b>pSlWlanEvent->Event = SL_WLAN_DISCONNECT_EVENT </b>, STA or P2P client disconnection event 00440 - pSlWlanEvent->EventData.STAandP2PModeDisconnected main fields: 00441 - ssid_name 00442 - ssid_len 00443 - reason_code 00444 00445 - <b>pSlWlanEvent->Event = SL_WLAN_STA_CONNECTED_EVENT </b>, AP/P2P(Go) connected STA/P2P(Client) 00446 - pSlWlanEvent->EventData.APModeStaConnected fields: 00447 - go_peer_device_name 00448 - mac 00449 - go_peer_device_name_len 00450 - wps_dev_password_id 00451 - own_ssid: relevant for event sta-connected only 00452 - own_ssid_len: relevant for event sta-connected only 00453 00454 - <b>pSlWlanEvent->Event = SL_WLAN_STA_DISCONNECTED_EVENT </b>, AP/P2P(Go) disconnected STA/P2P(Client) 00455 - pSlWlanEvent->EventData.APModestaDisconnected fields: 00456 - go_peer_device_name 00457 - mac 00458 - go_peer_device_name_len 00459 - wps_dev_password_id 00460 - own_ssid: relevant for event sta-connected only 00461 - own_ssid_len: relevant for event sta-connected only 00462 00463 - <b>pSlWlanEvent->Event = SL_WLAN_SMART_CONFIG_COMPLETE_EVENT </b> 00464 - pSlWlanEvent->EventData.smartConfigStartResponse fields: 00465 - status 00466 - ssid_len 00467 - ssid 00468 - private_token_len 00469 - private_token 00470 00471 - <b>pSlWlanEvent->Event = SL_WLAN_SMART_CONFIG_STOP_EVENT </b> 00472 - pSlWlanEvent->EventData.smartConfigStopResponse fields: 00473 - status 00474 00475 - <b>pSlWlanEvent->Event = SL_WLAN_P2P_DEV_FOUND_EVENT </b> 00476 - pSlWlanEvent->EventData.P2PModeDevFound fields: 00477 - go_peer_device_name 00478 - mac 00479 - go_peer_device_name_len 00480 - wps_dev_password_id 00481 - own_ssid: relevant for event sta-connected only 00482 - own_ssid_len: relevant for event sta-connected only 00483 00484 - <b>pSlWlanEvent->Event = SL_WLAN_P2P_NEG_REQ_RECEIVED_EVENT </b> 00485 - pSlWlanEvent->EventData.P2PModeNegReqReceived fields 00486 - go_peer_device_name 00487 - mac 00488 - go_peer_device_name_len 00489 - wps_dev_password_id 00490 - own_ssid: relevant for event sta-connected only 00491 00492 - <b>pSlWlanEvent->Event = SL_WLAN_CONNECTION_FAILED_EVENT </b>, P2P only 00493 - pSlWlanEvent->EventData.P2PModewlanConnectionFailure fields: 00494 - status 00495 */ 00496 #if (defined(sl_WlanEvtHdlr)) 00497 extern void sl_WlanEvtHdlr(SlWlanEvent_t *pSlWlanEvent); 00498 #endif 00499 00500 00501 /*! 00502 \brief NETAPP Async event handler 00503 00504 \param[out] pSlNetApp pointer to SlNetAppEvent_t data 00505 00506 \par 00507 Parameters: 00508 - <b>pSlWlanEvent->Event = SL_NETAPP_IPV4_IPACQUIRED_EVENT</b>, IPV4 acquired event 00509 - pSlWlanEvent->EventData.ipAcquiredV4 fields: 00510 - ip 00511 - gateway 00512 - dns 00513 00514 - <b>pSlWlanEvent->Event = SL_NETAPP_IP_LEASED_EVENT</b>, AP or P2P go dhcp lease event 00515 - pSlWlanEvent->EventData.ipLeased fields: 00516 - ip_address 00517 - lease_time 00518 - mac 00519 00520 - <b>pSlWlanEvent->Event = SL_NETAPP_IP_RELEASED_EVENT</b>, AP or P2P go dhcp ip release event 00521 - pSlWlanEvent->EventData.ipReleased fields 00522 - ip_address 00523 - mac 00524 - reason 00525 00526 */ 00527 #if (defined(sl_NetAppEvtHdlr)) 00528 extern void sl_NetAppEvtHdlr(SlNetAppEvent_t *pSlNetApp); 00529 #endif 00530 00531 /*! 00532 \brief Socket Async event handler 00533 00534 \param[out] pSlSockEvent pointer to SlSockEvent_t data 00535 00536 \par 00537 Parameters:\n 00538 - <b>pSlSockEvent->Event = SL_SOCKET_TX_FAILED_EVENT</b> 00539 - pSlSockEvent->EventData fields: 00540 - sd 00541 - status 00542 - <b>pSlSockEvent->Event = SL_SOCKET_ASYNC_EVENT</b> 00543 - pSlSockEvent->EventData fields: 00544 - sd 00545 - type: SSL_ACCEPT or RX_FRAGMENTATION_TOO_BIG or OTHER_SIDE_CLOSE_SSL_DATA_NOT_ENCRYPTED 00546 - val 00547 00548 */ 00549 #if (defined(sl_SockEvtHdlr)) 00550 extern void sl_SockEvtHdlr(SlSockEvent_t *pSlSockEvent); 00551 #endif 00552 00553 /*! 00554 \brief HTTP server async event 00555 00556 \param[out] pSlHttpServerEvent pointer to SlHttpServerEvent_t 00557 \param[in] pSlHttpServerResponse pointer to SlHttpServerResponse_t 00558 00559 \par 00560 Parameters: \n 00561 00562 - <b>pSlHttpServerEvent->Event = SL_NETAPP_HTTPGETTOKENVALUE_EVENT</b> 00563 - pSlHttpServerEvent->EventData fields: 00564 - httpTokenName 00565 - data 00566 - len 00567 - pSlHttpServerResponse->ResponseData fields: 00568 - data 00569 - len 00570 00571 - <b>pSlHttpServerEvent->Event = SL_NETAPP_HTTPPOSTTOKENVALUE_EVENT</b> 00572 - pSlHttpServerEvent->EventData.httpPostData fields: 00573 - action 00574 - token_name 00575 - token_value 00576 - pSlHttpServerResponse->ResponseData fields: 00577 - data 00578 - len 00579 00580 */ 00581 #if (defined(sl_HttpServerCallback)) 00582 extern void sl_HttpServerCallback(SlHttpServerEvent_t *pSlHttpServerEvent, SlHttpServerResponse_t *pSlHttpServerResponse); 00583 #endif 00584 /*! 00585 00586 Close the Doxygen group. 00587 @} 00588 00589 */ 00590 00591 #ifdef __cplusplus 00592 } 00593 #endif /* __cplusplus */ 00594 00595 #endif /* __SIMPLELINK_H__ */ 00596 00597
Generated on Tue Jul 12 2022 22:55:20 by
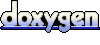