
TI's CC3100 host driver and demo. Experimental and a work in progress.
Embed:
(wiki syntax)
Show/hide line numbers
cc3100_protocol.h
00001 /* 00002 * protocol.h - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 /*******************************************************************************\ 00038 * 00039 * FILE NAME: protocol.h 00040 * 00041 * DESCRIPTION: Constant and data structure definitions and function 00042 * prototypes for the SL protocol module, which implements 00043 * processing of SimpleLink Commands. 00044 * 00045 * AUTHOR: 00046 * 00047 \*******************************************************************************/ 00048 00049 #ifndef _SL_PROTOCOL_TYPES_H_ 00050 #define _SL_PROTOCOL_TYPES_H_ 00051 00052 #ifdef __cplusplus 00053 extern "C" { 00054 #endif 00055 00056 /**************************************************************************** 00057 ** 00058 ** User I/F pools definitions 00059 ** 00060 ****************************************************************************/ 00061 00062 /**************************************************************************** 00063 ** 00064 ** Definitions for SimpleLink Commands 00065 ** 00066 ****************************************************************************/ 00067 00068 00069 /* pattern for LE 8/16/32 or BE*/ 00070 #define H2N_SYNC_PATTERN {0xBBDDEEFF,0x4321,0x34,0x12} 00071 #define H2N_CNYS_PATTERN {0xBBDDEEFF,0x8765,0x78,0x56} 00072 00073 #define H2N_DUMMY_PATTERN (_u32)0xFFFFFFFF 00074 #define N2H_SYNC_PATTERN (_u32)0xABCDDCBA 00075 #define SYNC_PATTERN_LEN (_u32)sizeof(_u32) 00076 #define UART_SET_MODE_MAGIC_CODE (_u32)0xAA55AA55 00077 #define SPI_16BITS_BUG(pattern) (_u32)((_u32)pattern & (_u32)0xFFFF7FFF) 00078 #define SPI_8BITS_BUG(pattern) (_u32)((_u32)pattern & (_u32)0xFFFFFF7F) 00079 00080 00081 00082 typedef struct 00083 { 00084 _u16 Opcode; 00085 _u16 Len; 00086 }_SlGenericHeader_t; 00087 00088 00089 typedef struct 00090 { 00091 _u32 Long; 00092 _u16 Short; 00093 _u8 Byte1; 00094 _u8 Byte2; 00095 }_SlSyncPattern_t; 00096 00097 typedef _SlGenericHeader_t _SlCommandHeader_t; 00098 00099 typedef struct 00100 { 00101 _SlGenericHeader_t GenHeader; 00102 _u8 TxPoolCnt; 00103 _u8 DevStatus; 00104 _u8 SocketTXFailure; 00105 _u8 SocketNonBlocking; 00106 }_SlResponseHeader_t; 00107 00108 #define _SL_RESP_SPEC_HDR_SIZE (sizeof(_SlResponseHeader_t) - sizeof(_SlGenericHeader_t)) 00109 #define _SL_RESP_HDR_SIZE sizeof(_SlResponseHeader_t) 00110 #define _SL_CMD_HDR_SIZE sizeof(_SlCommandHeader_t) 00111 00112 #define _SL_RESP_ARGS_START(_pMsg) (((_SlResponseHeader_t *)(_pMsg)) + 1) 00113 00114 /* Used only in NWP! */ 00115 typedef struct 00116 { 00117 _SlCommandHeader_t sl_hdr; 00118 _u8 func_args_start; 00119 } T_SCMD; 00120 00121 00122 #define WLAN_CONN_STATUS_BIT 0x01 00123 #define EVENTS_Q_STATUS_BIT 0x02 00124 #define PENDING_RCV_CMD_BIT 0x04 00125 #define FW_BUSY_PACKETS_BIT 0x08 00126 00127 #define INIT_STA_OK 0x11111111 00128 #define INIT_STA_ERR 0x22222222 00129 #define INIT_AP_OK 0x33333333 00130 #define INIT_AP_ERR 0x44444444 00131 #define INIT_P2P_OK 0x55555555 00132 #define INIT_P2P_ERR 0x66666666 00133 00134 /**************************************************************************** 00135 ** OPCODES 00136 ****************************************************************************/ 00137 #define SL_IPV4_IPV6_OFFSET ( 9 ) 00138 #define SL_OPCODE_IPV4 ( 0x0 << SL_IPV4_IPV6_OFFSET ) 00139 #define SL_OPCODE_IPV6 ( 0x1 << SL_IPV4_IPV6_OFFSET ) 00140 00141 #define SL_SYNC_ASYNC_OFFSET ( 10 ) 00142 #define SL_OPCODE_SYNC (0x1 << SL_SYNC_ASYNC_OFFSET ) 00143 #define SL_OPCODE_SILO_OFFSET ( 11 ) 00144 #define SL_OPCODE_SILO_MASK ( 0xF << SL_OPCODE_SILO_OFFSET ) 00145 #define SL_OPCODE_SILO_DEVICE ( 0x0 << SL_OPCODE_SILO_OFFSET ) 00146 #define SL_OPCODE_SILO_WLAN ( 0x1 << SL_OPCODE_SILO_OFFSET ) 00147 #define SL_OPCODE_SILO_SOCKET ( 0x2 << SL_OPCODE_SILO_OFFSET ) 00148 #define SL_OPCODE_SILO_NETAPP ( 0x3 << SL_OPCODE_SILO_OFFSET ) 00149 #define SL_OPCODE_SILO_NVMEM ( 0x4 << SL_OPCODE_SILO_OFFSET ) 00150 #define SL_OPCODE_SILO_NETCFG ( 0x5 << SL_OPCODE_SILO_OFFSET ) 00151 00152 #define SL_FAMILY_SHIFT (0x4) 00153 #define SL_FLAGS_MASK (0xF) 00154 00155 #define SL_OPCODE_DEVICE_INITCOMPLETE 0x0008 00156 #define SL_OPCODE_DEVICE_STOP_COMMAND 0x8473 00157 #define SL_OPCODE_DEVICE_STOP_RESPONSE 0x0473 00158 #define SL_OPCODE_DEVICE_STOP_ASYNC_RESPONSE 0x0073 00159 #define SL_OPCODE_DEVICE_DEVICEASYNCDUMMY 0x0063 00160 00161 #define SL_OPCODE_DEVICE_VERSIONREADCOMMAND 0x8470 00162 #define SL_OPCODE_DEVICE_VERSIONREADRESPONSE 0x0470 00163 #define SL_OPCODE_DEVICE_DEVICEASYNCFATALERROR 0x0078 00164 #define SL_OPCODE_WLAN_WLANCONNECTCOMMAND 0x8C80 00165 #define SL_OPCODE_WLAN_WLANCONNECTRESPONSE 0x0C80 00166 #define SL_OPCODE_WLAN_WLANASYNCCONNECTEDRESPONSE 0x0880 00167 #define SL_OPCODE_WLAN_P2P_DEV_FOUND 0x0830 00168 #define SL_OPCODE_WLAN_CONNECTION_FAILED 0x0831 00169 #define SL_OPCODE_WLAN_P2P_NEG_REQ_RECEIVED 0x0832 00170 00171 #define SL_OPCODE_WLAN_WLANDISCONNECTCOMMAND 0x8C81 00172 #define SL_OPCODE_WLAN_WLANDISCONNECTRESPONSE 0x0C81 00173 #define SL_OPCODE_WLAN_WLANASYNCDISCONNECTEDRESPONSE 0x0881 00174 #define SL_OPCODE_WLAN_WLANCONNECTEAPCOMMAND 0x8C82 00175 #define SL_OPCODE_WLAN_WLANCONNECTEAPCRESPONSE 0x0C82 00176 #define SL_OPCODE_WLAN_PROFILEADDCOMMAND 0x8C83 00177 #define SL_OPCODE_WLAN_PROFILEADDRESPONSE 0x0C83 00178 #define SL_OPCODE_WLAN_PROFILEGETCOMMAND 0x8C84 00179 #define SL_OPCODE_WLAN_PROFILEGETRESPONSE 0x0C84 00180 #define SL_OPCODE_WLAN_PROFILEDELCOMMAND 0x8C85 00181 #define SL_OPCODE_WLAN_PROFILEDELRESPONSE 0x0C85 00182 #define SL_OPCODE_WLAN_POLICYSETCOMMAND 0x8C86 00183 #define SL_OPCODE_WLAN_POLICYSETRESPONSE 0x0C86 00184 #define SL_OPCODE_WLAN_POLICYGETCOMMAND 0x8C87 00185 #define SL_OPCODE_WLAN_POLICYGETRESPONSE 0x0C87 00186 #define SL_OPCODE_WLAN_FILTERADD 0x8C88 00187 #define SL_OPCODE_WLAN_FILTERADDRESPONSE 0x0C88 00188 #define SL_OPCODE_WLAN_FILTERGET 0x8C89 00189 #define SL_OPCODE_WLAN_FILTERGETRESPONSE 0x0C89 00190 #define SL_OPCODE_WLAN_FILTERDELETE 0x8C8A 00191 #define SL_OPCODE_WLAN_FILTERDELETERESPOSNE 0x0C8A 00192 #define SL_OPCODE_WLAN_WLANGETSTATUSCOMMAND 0x8C8F 00193 #define SL_OPCODE_WLAN_WLANGETSTATUSRESPONSE 0x0C8F 00194 #define SL_OPCODE_WLAN_STARTTXCONTINUESCOMMAND 0x8CAA 00195 #define SL_OPCODE_WLAN_STARTTXCONTINUESRESPONSE 0x0CAA 00196 #define SL_OPCODE_WLAN_STOPTXCONTINUESCOMMAND 0x8CAB 00197 #define SL_OPCODE_WLAN_STOPTXCONTINUESRESPONSE 0x0CAB 00198 #define SL_OPCODE_WLAN_STARTRXSTATCOMMAND 0x8CAC 00199 #define SL_OPCODE_WLAN_STARTRXSTATRESPONSE 0x0CAC 00200 #define SL_OPCODE_WLAN_STOPRXSTATCOMMAND 0x8CAD 00201 #define SL_OPCODE_WLAN_STOPRXSTATRESPONSE 0x0CAD 00202 #define SL_OPCODE_WLAN_GETRXSTATCOMMAND 0x8CAF 00203 #define SL_OPCODE_WLAN_GETRXSTATRESPONSE 0x0CAF 00204 #define SL_OPCODE_WLAN_POLICYSETCOMMANDNEW 0x8CB0 00205 #define SL_OPCODE_WLAN_POLICYSETRESPONSENEW 0x0CB0 00206 #define SL_OPCODE_WLAN_POLICYGETCOMMANDNEW 0x8CB1 00207 #define SL_OPCODE_WLAN_POLICYGETRESPONSENEW 0x0CB1 00208 00209 #define SL_OPCODE_WLAN_SMART_CONFIG_START_COMMAND 0x8CB2 00210 #define SL_OPCODE_WLAN_SMART_CONFIG_START_RESPONSE 0x0CB2 00211 #define SL_OPCODE_WLAN_SMART_CONFIG_START_ASYNC_RESPONSE 0x08B2 00212 #define SL_OPCODE_WLAN_SMART_CONFIG_STOP_COMMAND 0x8CB3 00213 #define SL_OPCODE_WLAN_SMART_CONFIG_STOP_RESPONSE 0x0CB3 00214 #define SL_OPCODE_WLAN_SMART_CONFIG_STOP_ASYNC_RESPONSE 0x08B3 00215 #define SL_OPCODE_WLAN_SET_MODE 0x8CB4 00216 #define SL_OPCODE_WLAN_SET_MODE_RESPONSE 0x0CB4 00217 #define SL_OPCODE_WLAN_CFG_SET 0x8CB5 00218 #define SL_OPCODE_WLAN_CFG_SET_RESPONSE 0x0CB5 00219 #define SL_OPCODE_WLAN_CFG_GET 0x8CB6 00220 #define SL_OPCODE_WLAN_CFG_GET_RESPONSE 0x0CB6 00221 #define SL_OPCODE_WLAN_STA_CONNECTED 0x082E 00222 #define SL_OPCODE_WLAN_STA_DISCONNECTED 0x082F 00223 #define SL_OPCODE_WLAN_EAP_PROFILEADDCOMMAND 0x8C67 00224 #define SL_OPCODE_WLAN_EAP_PROFILEADDCOMMAND_RESPONSE 0x0C67 00225 00226 #define SL_OPCODE_SOCKET_SOCKET 0x9401 00227 #define SL_OPCODE_SOCKET_SOCKETRESPONSE 0x1401 00228 #define SL_OPCODE_SOCKET_CLOSE 0x9402 00229 #define SL_OPCODE_SOCKET_CLOSERESPONSE 0x1402 00230 #define SL_OPCODE_SOCKET_ACCEPT 0x9403 00231 #define SL_OPCODE_SOCKET_ACCEPTRESPONSE 0x1403 00232 #define SL_OPCODE_SOCKET_ACCEPTASYNCRESPONSE 0x1003 00233 #define SL_OPCODE_SOCKET_ACCEPTASYNCRESPONSE_V6 0x1203 00234 #define SL_OPCODE_SOCKET_BIND 0x9404 00235 #define SL_OPCODE_SOCKET_BIND_V6 0x9604 00236 #define SL_OPCODE_SOCKET_BINDRESPONSE 0x1404 00237 #define SL_OPCODE_SOCKET_LISTEN 0x9405 00238 #define SL_OPCODE_SOCKET_LISTENRESPONSE 0x1405 00239 #define SL_OPCODE_SOCKET_CONNECT 0x9406 00240 #define SL_OPCODE_SOCKET_CONNECT_V6 0x9606 00241 #define SL_OPCODE_SOCKET_CONNECTRESPONSE 0x1406 00242 #define SL_OPCODE_SOCKET_CONNECTASYNCRESPONSE 0x1006 00243 #define SL_OPCODE_SOCKET_SELECT 0x9407 00244 #define SL_OPCODE_SOCKET_SELECTRESPONSE 0x1407 00245 #define SL_OPCODE_SOCKET_SELECTASYNCRESPONSE 0x1007 00246 #define SL_OPCODE_SOCKET_SETSOCKOPT 0x9408 00247 #define SL_OPCODE_SOCKET_SETSOCKOPTRESPONSE 0x1408 00248 #define SL_OPCODE_SOCKET_GETSOCKOPT 0x9409 00249 #define SL_OPCODE_SOCKET_GETSOCKOPTRESPONSE 0x1409 00250 #define SL_OPCODE_SOCKET_RECV 0x940A 00251 #define SL_OPCODE_SOCKET_RECVASYNCRESPONSE 0x100A 00252 #define SL_OPCODE_SOCKET_RECVFROM 0x940B 00253 #define SL_OPCODE_SOCKET_RECVFROMASYNCRESPONSE 0x100B 00254 #define SL_OPCODE_SOCKET_RECVFROMASYNCRESPONSE_V6 0x120B 00255 #define SL_OPCODE_SOCKET_SEND 0x940C 00256 #define SL_OPCODE_SOCKET_SENDTO 0x940D 00257 #define SL_OPCODE_SOCKET_SENDTO_V6 0x960D 00258 #define SL_OPCODE_SOCKET_TXFAILEDASYNCRESPONSE 0x100E 00259 #define SL_OPCODE_SOCKET_SOCKETASYNCEVENT 0x100F 00260 #define SL_OPCODE_NETAPP_START_COMMAND 0x9C0A 00261 #define SL_OPCODE_NETAPP_START_RESPONSE 0x1C0A 00262 #define SL_OPCODE_NETAPP_NETAPPSTARTRESPONSE 0x1C0A 00263 #define SL_OPCODE_NETAPP_STOP_COMMAND 0x9C61 00264 #define SL_OPCODE_NETAPP_STOP_RESPONSE 0x1C61 00265 #define SL_OPCODE_NETAPP_NETAPPSET 0x9C0B 00266 #define SL_OPCODE_NETAPP_NETAPPSETRESPONSE 0x1C0B 00267 #define SL_OPCODE_NETAPP_NETAPPGET 0x9C27 00268 #define SL_OPCODE_NETAPP_NETAPPGETRESPONSE 0x1C27 00269 #define SL_OPCODE_NETAPP_DNSGETHOSTBYNAME 0x9C20 00270 #define SL_OPCODE_NETAPP_DNSGETHOSTBYNAMERESPONSE 0x1C20 00271 #define SL_OPCODE_NETAPP_DNSGETHOSTBYNAMEASYNCRESPONSE 0x1820 00272 #define SL_OPCODE_NETAPP_DNSGETHOSTBYNAMEASYNCRESPONSE_V6 0x1A20 00273 #define SL_OPCODE_NETAPP_NETAPP_MDNS_LOOKUP_SERVICE 0x9C71 00274 #define SL_OPCODE_NETAPP_NETAPP_MDNS_LOOKUP_SERVICE_RESPONSE 0x1C72 00275 #define SL_OPCODE_NETAPP_MDNSREGISTERSERVICE 0x9C34 00276 #define SL_OPCODE_NETAPP_MDNSREGISTERSERVICERESPONSE 0x1C34 00277 #define SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICE 0x9C35 00278 #define SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICERESPONSE 0x1C35 00279 #define SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICEASYNCRESPONSE 0x1835 00280 #define SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICEASYNCRESPONSE_V6 0x1A35 00281 #define SL_OPCODE_NETAPP_DNSGETHOSTBYADDR 0x9C26 00282 #define SL_OPCODE_NETAPP_DNSGETHOSTBYADDR_V6 0x9E26 00283 #define SL_OPCODE_NETAPP_DNSGETHOSTBYADDRRESPONSE 0x1C26 00284 #define SL_OPCODE_NETAPP_DNSGETHOSTBYADDRASYNCRESPONSE 0x1826 00285 #define SL_OPCODE_NETAPP_PINGSTART 0x9C21 00286 #define SL_OPCODE_NETAPP_PINGSTART_V6 0x9E21 00287 #define SL_OPCODE_NETAPP_PINGSTARTRESPONSE 0x1C21 00288 #define SL_OPCODE_NETAPP_PINGREPORTREQUEST 0x9C22 00289 #define SL_OPCODE_NETAPP_PINGREPORTREQUESTRESPONSE 0x1822 00290 #define SL_OPCODE_NETAPP_PINGSTOP 0x9C23 00291 #define SL_OPCODE_NETAPP_PINGSTOPRESPONSE 0x1C23 00292 #define SL_OPCODE_NETAPP_ARPFLUSH 0x9C24 00293 #define SL_OPCODE_NETAPP_ARPFLUSHRESPONSE 0x1C24 00294 #define SL_OPCODE_NETAPP_IPACQUIRED 0x1825 00295 #define SL_OPCODE_NETAPP_IPV4_LOST 0x1832 00296 #define SL_OPCODE_NETAPP_DHCP_IPV4_ACQUIRE_TIMEOUT 0x1833 00297 #define SL_OPCODE_NETAPP_IPACQUIRED_V6 0x1A25 00298 #define SL_OPCODE_NETAPP_IPERFSTARTCOMMAND 0x9C28 00299 #define SL_OPCODE_NETAPP_IPERFSTARTRESPONSE 0x1C28 00300 #define SL_OPCODE_NETAPP_IPERFSTOPCOMMAND 0x9C29 00301 #define SL_OPCODE_NETAPP_IPERFSTOPRESPONSE 0x1C29 00302 #define SL_OPCODE_NETAPP_CTESTSTARTCOMMAND 0x9C2A 00303 #define SL_OPCODE_NETAPP_CTESTSTARTRESPONSE 0x1C2A 00304 #define SL_OPCODE_NETAPP_CTESTASYNCRESPONSE 0x182A 00305 #define SL_OPCODE_NETAPP_CTESTSTOPCOMMAND 0x9C2B 00306 #define SL_OPCODE_NETAPP_CTESTSTOPRESPONSE 0x1C2B 00307 #define SL_OPCODE_NETAPP_IP_LEASED 0x182C 00308 #define SL_OPCODE_NETAPP_IP_RELEASED 0x182D 00309 #define SL_OPCODE_NETAPP_HTTPGETTOKENVALUE 0x182E 00310 #define SL_OPCODE_NETAPP_HTTPSENDTOKENVALUE 0x9C2F 00311 #define SL_OPCODE_NETAPP_HTTPPOSTTOKENVALUE 0x1830 00312 #define SL_OPCODE_NVMEM_FILEOPEN 0xA43C 00313 #define SL_OPCODE_NVMEM_FILEOPENRESPONSE 0x243C 00314 #define SL_OPCODE_NVMEM_FILECLOSE 0xA43D 00315 #define SL_OPCODE_NVMEM_FILECLOSERESPONSE 0x243D 00316 #define SL_OPCODE_NVMEM_FILEREADCOMMAND 0xA440 00317 #define SL_OPCODE_NVMEM_FILEREADRESPONSE 0x2440 00318 #define SL_OPCODE_NVMEM_FILEWRITECOMMAND 0xA441 00319 #define SL_OPCODE_NVMEM_FILEWRITERESPONSE 0x2441 00320 #define SL_OPCODE_NVMEM_FILEGETINFOCOMMAND 0xA442 00321 #define SL_OPCODE_NVMEM_FILEGETINFORESPONSE 0x2442 00322 #define SL_OPCODE_NVMEM_FILEDELCOMMAND 0xA443 00323 #define SL_OPCODE_NVMEM_FILEDELRESPONSE 0x2443 00324 #define SL_OPCODE_NVMEM_NVMEMFORMATCOMMAND 0xA444 00325 #define SL_OPCODE_NVMEM_NVMEMFORMATRESPONSE 0x2444 00326 00327 #define SL_OPCODE_DEVICE_SETDEBUGLEVELCOMMAND 0x846A 00328 #define SL_OPCODE_DEVICE_SETDEBUGLEVELRESPONSE 0x046A 00329 00330 #define SL_OPCODE_DEVICE_NETCFG_SET_COMMAND 0x8432 00331 #define SL_OPCODE_DEVICE_NETCFG_SET_RESPONSE 0x0432 00332 #define SL_OPCODE_DEVICE_NETCFG_GET_COMMAND 0x8433 00333 #define SL_OPCODE_DEVICE_NETCFG_GET_RESPONSE 0x0433 00334 /* */ 00335 #define SL_OPCODE_DEVICE_SETUARTMODECOMMAND 0x846B 00336 #define SL_OPCODE_DEVICE_SETUARTMODERESPONSE 0x046B 00337 #define SL_OPCODE_DEVICE_SSISIZESETCOMMAND 0x846B 00338 #define SL_OPCODE_DEVICE_SSISIZESETRESPONSE 0x046B 00339 00340 /* */ 00341 #define SL_OPCODE_DEVICE_EVENTMASKSET 0x8464 00342 #define SL_OPCODE_DEVICE_EVENTMASKSETRESPONSE 0x0464 00343 #define SL_OPCODE_DEVICE_EVENTMASKGET 0x8465 00344 #define SL_OPCODE_DEVICE_EVENTMASKGETRESPONSE 0x0465 00345 00346 #define SL_OPCODE_DEVICE_DEVICEGET 0x8466 00347 #define SL_OPCODE_DEVICE_DEVICEGETRESPONSE 0x0466 00348 #define SL_OPCODE_DEVICE_DEVICESET 0x84B7 00349 #define SL_OPCODE_DEVICE_DEVICESETRESPONSE 0x04B7 00350 00351 #define SL_OPCODE_WLAN_SCANRESULTSGETCOMMAND 0x8C8C 00352 #define SL_OPCODE_WLAN_SCANRESULTSGETRESPONSE 0x0C8C 00353 #define SL_OPCODE_WLAN_SMARTCONFIGOPTSET 0x8C8D 00354 #define SL_OPCODE_WLAN_SMARTCONFIGOPTSETRESPONSE 0x0C8D 00355 #define SL_OPCODE_WLAN_SMARTCONFIGOPTGET 0x8C8E 00356 #define SL_OPCODE_WLAN_SMARTCONFIGOPTGETRESPONSE 0x0C8E 00357 00358 #define SL_OPCODE_FREE_BSD_RECV_BUFFER 0xCCCB 00359 #define SL_OPCODE_FREE_NON_BSD_READ_BUFFER 0xCCCD 00360 00361 00362 /* Rx Filters opcodes */ 00363 #define SL_OPCODE_WLAN_WLANRXFILTERADDCOMMAND 0x8C6C 00364 #define SL_OPCODE_WLAN_WLANRXFILTERADDRESPONSE 0x0C6C 00365 #define SL_OPCODE_WLAN_WLANRXFILTERSETCOMMAND 0x8C6D 00366 #define SL_OPCODE_WLAN_WLANRXFILTERSETRESPONSE 0x0C6D 00367 #define SL_OPCODE_WLAN_WLANRXFILTERGETSTATISTICSINFOCOMMAND 0x8C6E 00368 #define SL_OPCODE_WLAN_WLANRXFILTERGETSTATISTICSINFORESPONSE 0x0C6E 00369 #define SL_OPCODE_WLAN_WLANRXFILTERGETCOMMAND 0x8C6F 00370 #define SL_OPCODE_WLAN_WLANRXFILTERGETRESPONSE 0x0C6F 00371 #define SL_OPCODE_WLAN_WLANRXFILTERGETINFO 0x8C70 00372 #define SL_OPCODE_WLAN_WLANRXFILTERGETINFORESPONSE 0x0C70 00373 00374 00375 /******************************************************************************************/ 00376 /* Device structs */ 00377 /******************************************************************************************/ 00378 typedef _u32 InitStatus_t; 00379 00380 00381 typedef struct 00382 { 00383 _i32 Status; 00384 }InitComplete_t; 00385 00386 typedef struct 00387 { 00388 _i16 status; 00389 _u16 padding; 00390 00391 }_BasicResponse_t; 00392 00393 typedef struct 00394 { 00395 _u16 Timeout; 00396 _u16 padding; 00397 }_DevStopCommand_t; 00398 00399 typedef struct 00400 { 00401 _u32 group; 00402 _u32 mask; 00403 }_DevMaskEventSetCommand_t; 00404 00405 typedef _BasicResponse_t _DevMaskEventSetResponse_t; 00406 00407 00408 typedef struct 00409 { 00410 _u32 group; 00411 }_DevMaskEventGetCommand_t; 00412 00413 00414 typedef struct 00415 { 00416 _u32 group; 00417 _u32 mask; 00418 }_DevMaskEventGetResponse_t; 00419 00420 00421 typedef struct 00422 { 00423 _u32 group; 00424 }_DevStatusGetCommand_t; 00425 00426 00427 typedef struct 00428 { 00429 _u32 group; 00430 _u32 status; 00431 }_DevStatusGetResponse_t; 00432 00433 typedef struct 00434 { 00435 _u32 ChipId; 00436 _u32 FwVersion[4]; 00437 _u8 PhyVersion[4]; 00438 }_Device_VersionReadResponsePart_t; 00439 00440 typedef struct 00441 { 00442 _Device_VersionReadResponsePart_t part; 00443 _u32 NwpVersion[4]; 00444 _u16 RomVersion; 00445 _u16 Padding; 00446 }_Device_VersionReadResponseFull_t; 00447 00448 00449 typedef struct 00450 { 00451 _u32 BaudRate; 00452 _u8 FlowControlEnable; 00453 }_DevUartSetModeCommand_t; 00454 00455 typedef _BasicResponse_t _DevUartSetModeResponse_t; 00456 00457 /******************************************************/ 00458 00459 typedef struct 00460 { 00461 _u8 SsiSizeInBytes; 00462 _u8 Padding[3]; 00463 }_StellarisSsiSizeSet_t; 00464 00465 /*****************************************************************************************/ 00466 /* WLAN structs */ 00467 /*****************************************************************************************/ 00468 #define MAXIMAL_PASSWORD_LENGTH (64) 00469 00470 typedef struct{ 00471 _u8 SecType; 00472 _u8 SsidLen; 00473 _u8 Bssid[6]; 00474 _u8 PasswordLen; 00475 }_WlanConnectCommon_t; 00476 00477 #define SSID_STRING(pCmd) (_i8 *)((_WlanConnectCommon_t *)(pCmd) + 1) 00478 #define PASSWORD_STRING(pCmd) (SSID_STRING(pCmd) + ((_WlanConnectCommon_t *)(pCmd))->SsidLen) 00479 00480 typedef struct{ 00481 _WlanConnectCommon_t Common; 00482 _u8 UserLen; 00483 _u8 AnonUserLen; 00484 _u8 CertIndex; 00485 _u32 EapBitmask; 00486 }_WlanConnectEapCommand_t; 00487 00488 #define EAP_SSID_STRING(pCmd) (_i8 *)((_WlanConnectEapCommand_t *)(pCmd) + 1) 00489 #define EAP_PASSWORD_STRING(pCmd) (EAP_SSID_STRING(pCmd) + ((_WlanConnectEapCommand_t *)(pCmd))->Common.SsidLen) 00490 #define EAP_USER_STRING(pCmd) (EAP_PASSWORD_STRING(pCmd) + ((_WlanConnectEapCommand_t *)(pCmd))->Common.PasswordLen) 00491 #define EAP_ANON_USER_STRING(pCmd) (EAP_USER_STRING(pCmd) + ((_WlanConnectEapCommand_t *)(pCmd))->UserLen) 00492 00493 00494 typedef struct 00495 { 00496 _u8 PolicyType; 00497 _u8 Padding; 00498 _u8 PolicyOption; 00499 _u8 PolicyOptionLen; 00500 }_WlanPoliciySetGet_t; 00501 00502 00503 typedef struct{ 00504 _u32 minDwellTime; 00505 _u32 maxDwellTime; 00506 _u32 numProbeResponse; 00507 _u32 G_Channels_mask; 00508 _i32 rssiThershold; 00509 _i32 snrThershold; 00510 _i32 defaultTXPower; 00511 _u16 intervalList[16]; 00512 }_WlanScanParamSetCommand_t; 00513 00514 00515 typedef struct{ 00516 _i8 SecType; 00517 _u8 SsidLen; 00518 _u8 Priority; 00519 _u8 Bssid[6]; 00520 _u8 PasswordLen; 00521 _u8 WepKeyId; 00522 }_WlanAddGetProfile_t; 00523 00524 00525 typedef struct{ 00526 _WlanAddGetProfile_t Common; 00527 _u8 UserLen; 00528 _u8 AnonUserLen; 00529 _u8 CertIndex; 00530 _u16 padding; 00531 _u32 EapBitmask; 00532 }_WlanAddGetEapProfile_t; 00533 00534 00535 00536 00537 #define PROFILE_SSID_STRING(pCmd) ((_i8 *)((_WlanAddGetProfile_t *)(pCmd) + 1)) 00538 #define PROFILE_PASSWORD_STRING(pCmd) (PROFILE_SSID_STRING(pCmd) + ((_WlanAddGetProfile_t *)(pCmd))->SsidLen) 00539 00540 #define EAP_PROFILE_SSID_STRING(pCmd) (_i8 *)((_WlanAddGetEapProfile_t *)(pCmd) + 1) 00541 #define EAP_PROFILE_PASSWORD_STRING(pCmd) (EAP_PROFILE_SSID_STRING(pCmd) + ((_WlanAddGetEapProfile_t *)(pCmd))->Common.SsidLen) 00542 #define EAP_PROFILE_USER_STRING(pCmd) (EAP_PROFILE_PASSWORD_STRING(pCmd) + ((_WlanAddGetEapProfile_t *)(pCmd))->Common.PasswordLen) 00543 #define EAP_PROFILE_ANON_USER_STRING(pCmd) (EAP_PROFILE_USER_STRING(pCmd) + ((_WlanAddGetEapProfile_t *)(pCmd))->UserLen) 00544 00545 00546 00547 typedef struct 00548 { 00549 _u8 index; 00550 _u8 padding[3]; 00551 }_WlanProfileDelGetCommand_t; 00552 00553 typedef _BasicResponse_t _WlanGetNetworkListResponse_t; 00554 00555 typedef struct 00556 { 00557 _u8 index; 00558 _u8 count; 00559 _i8 padding[2]; 00560 }_WlanGetNetworkListCommand_t; 00561 00562 00563 00564 00565 typedef struct 00566 { 00567 _u32 groupIdBitmask; 00568 _u8 cipher; 00569 _u8 publicKeyLen; 00570 _u8 group1KeyLen; 00571 _u8 group2KeyLen; 00572 }_WlanSmartConfigStartCommand_t; 00573 00574 #define SMART_CONFIG_START_PUBLIC_KEY_STRING(pCmd) ((_i8 *)((_WlanSmartConfigStartCommand_t *)(pCmd) + 1)) 00575 #define SMART_CONFIG_START_GROUP1_KEY_STRING(pCmd) ((_i8 *) (SMART_CONFIG_START_PUBLIC_KEY_STRING(pCmd) + ((_WlanSmartConfigStartCommand_t *)(pCmd))->publicKeyLen)) 00576 #define SMART_CONFIG_START_GROUP2_KEY_STRING(pCmd) ((_i8 *) (SMART_CONFIG_START_GROUP1_KEY_STRING(pCmd) + ((_WlanSmartConfigStartCommand_t *)(pCmd))->group1KeyLen)) 00577 00578 00579 00580 typedef struct 00581 { 00582 _u8 mode; 00583 _u8 padding[3]; 00584 }_WlanSetMode_t; 00585 00586 00587 00588 00589 typedef struct 00590 { 00591 _u16 Status; 00592 _u16 ConfigId; 00593 _u16 ConfigOpt; 00594 _u16 ConfigLen; 00595 }_WlanCfgSetGet_t; 00596 00597 00598 /* ******************************************************************************/ 00599 /* RX filters - Start */ 00600 /* ******************************************************************************/ 00601 /* -- 80 bytes */ 00602 typedef struct _WlanRxFilterAddCommand_t 00603 { 00604 /* -- 1 byte */ 00605 SlrxFilterRuleType_t RuleType; 00606 /* -- 1 byte */ 00607 SlrxFilterFlags_t FilterFlags; 00608 /* -- 1 byte */ 00609 SlrxFilterID_t FilterId; 00610 /* -- 1 byte */ 00611 _u8 Padding; 00612 /* -- 56 byte */ 00613 SlrxFilterRule_t Rule; 00614 /* -- 12 byte ( 3 padding ) */ 00615 SlrxFilterTrigger_t Trigger; 00616 /* -- 8 byte */ 00617 SlrxFilterAction_t Action; 00618 }_WlanRxFilterAddCommand_t; 00619 00620 00621 00622 /* -- 4 bytes */ 00623 typedef struct l_WlanRxFilterAddCommandReponse_t 00624 { 00625 /* -- 1 byte */ 00626 SlrxFilterID_t FilterId; 00627 /* -- 1 Byte */ 00628 _u8 Status; 00629 /* -- 2 byte */ 00630 _u8 Padding[2]; 00631 00632 }_WlanRxFilterAddCommandReponse_t; 00633 00634 00635 00636 /* 00637 * \struct _WlanRxFilterSetCommand_t 00638 */ 00639 typedef struct _WlanRxFilterSetCommand_t 00640 { 00641 _u16 InputBufferLength; 00642 /* 1 byte */ 00643 SLrxFilterOperation_t RxFilterOperation; 00644 _u8 Padding[1]; 00645 }_WlanRxFilterSetCommand_t; 00646 00647 /** 00648 * \struct _WlanRxFilterSetCommandReponse_t 00649 */ 00650 typedef struct _WlanRxFilterSetCommandReponse_t 00651 { 00652 /* 1 byte */ 00653 _u8 Status; 00654 /* 3 bytes */ 00655 _u8 Padding[3]; 00656 00657 }_WlanRxFilterSetCommandReponse_t; 00658 00659 /** 00660 * \struct _WlanRxFilterGetCommand_t 00661 */ 00662 typedef struct _WlanRxFilterGetCommand_t 00663 { 00664 _u16 OutputBufferLength; 00665 /* 1 byte */ 00666 SLrxFilterOperation_t RxFilterOperation; 00667 _u8 Padding[1]; 00668 }_WlanRxFilterGetCommand_t; 00669 00670 /** 00671 * \struct _WlanRxFilterGetCommandReponse_t 00672 */ 00673 typedef struct _WlanRxFilterGetCommandReponse_t 00674 { 00675 /* 1 byte */ 00676 _u8 Status; 00677 /* 1 bytes */ 00678 _u8 Padding; 00679 /* 2 byte */ 00680 _u16 OutputBufferLength; 00681 00682 }_WlanRxFilterGetCommandReponse_t; 00683 00684 00685 00686 /* ******************************************************************************/ 00687 /* RX filters -- End */ 00688 /* ******************************************************************************/ 00689 00690 typedef struct 00691 { 00692 _u16 status; 00693 _u8 WlanRole; /* 0 = station, 2 = AP */ 00694 _u8 Ipv6Enabled; 00695 _u8 Ipv6DhcpEnabled; 00696 00697 _u32 ipV6Global[4]; 00698 _u32 ipV6Local[4]; 00699 _u32 ipV6DnsServer[4]; 00700 _u8 Ipv6DhcpState; 00701 00702 }_NetappIpV6configRetArgs_t; 00703 00704 00705 typedef struct 00706 { 00707 _u8 ipV4[4]; 00708 _u8 ipV4Mask[4]; 00709 _u8 ipV4Gateway[4]; 00710 _u8 ipV4DnsServer[4]; 00711 _u8 ipV4Start[4]; 00712 _u8 ipV4End[4]; 00713 }_NetCfgIpV4AP_Args_t; 00714 00715 00716 00717 typedef struct 00718 { 00719 _u16 status; 00720 _u8 MacAddr[6]; 00721 } _MAC_Address_SetGet_t; 00722 00723 00724 typedef struct 00725 { 00726 _u16 Status; 00727 _u16 ConfigId; 00728 _u16 ConfigOpt; 00729 _u16 ConfigLen; 00730 }_NetCfgSetGet_t; 00731 00732 typedef struct 00733 { 00734 _u16 Status; 00735 _u16 DeviceSetId; 00736 _u16 Option; 00737 _u16 ConfigLen; 00738 }_DeviceSetGet_t; 00739 00740 00741 00742 00743 /******************************************************************************************/ 00744 /* Socket structs */ 00745 /******************************************************************************************/ 00746 00747 typedef struct 00748 { 00749 _u8 Domain; 00750 _u8 Type; 00751 _u8 Protocol; 00752 _u8 Padding; 00753 }_SocketCommand_t; 00754 00755 00756 typedef struct 00757 { 00758 _i16 statusOrLen; 00759 _u8 sd; 00760 _u8 padding; 00761 }_SocketResponse_t; 00762 00763 typedef struct 00764 { 00765 _u8 sd; 00766 _u8 family; 00767 _u8 padding1; 00768 _u8 padding2; 00769 }_AcceptCommand_t; 00770 00771 00772 typedef struct 00773 { 00774 _i16 statusOrLen; 00775 _u8 sd; 00776 _u8 family; 00777 _u16 port; 00778 _u16 paddingOrAddr; 00779 _u32 address; 00780 }_SocketAddrAsyncIPv4Response_t; 00781 00782 typedef struct 00783 { 00784 _i16 statusOrLen; 00785 _u8 sd; 00786 _u8 family; 00787 _u16 port; 00788 _u8 address[6]; 00789 }_SocketAddrAsyncIPv6EUI48Response_t; 00790 typedef struct 00791 { 00792 _i16 statusOrLen; 00793 _u8 sd; 00794 _u8 family; 00795 _u16 port; 00796 _u16 paddingOrAddr; 00797 _u32 address[4]; 00798 }_SocketAddrAsyncIPv6Response_t; 00799 00800 00801 typedef struct 00802 { 00803 _i16 lenOrPadding; 00804 _u8 sd; 00805 _u8 FamilyAndFlags; 00806 _u16 port; 00807 _u16 paddingOrAddr; 00808 _u32 address; 00809 }_SocketAddrIPv4Command_t; 00810 00811 typedef struct 00812 { 00813 _i16 lenOrPadding; 00814 _u8 sd; 00815 _u8 FamilyAndFlags; 00816 _u16 port; 00817 _u8 address[6]; 00818 }_SocketAddrIPv6EUI48Command_t; 00819 typedef struct 00820 { 00821 _i16 lenOrPadding; 00822 _u8 sd; 00823 _u8 FamilyAndFlags; 00824 _u16 port; 00825 _u16 paddingOrAddr; 00826 _u32 address[4]; 00827 }_SocketAddrIPv6Command_t; 00828 00829 typedef union { 00830 _SocketAddrIPv4Command_t IpV4; 00831 _SocketAddrIPv6EUI48Command_t IpV6EUI48; 00832 #ifdef SL_SUPPORT_IPV6 00833 _SocketAddrIPv6Command_t IpV6; 00834 #endif 00835 } _SocketAddrCommand_u; 00836 00837 typedef union { 00838 _SocketAddrAsyncIPv4Response_t IpV4; 00839 _SocketAddrAsyncIPv6EUI48Response_t IpV6EUI48; 00840 #ifdef SL_SUPPORT_IPV6 00841 _SocketAddrAsyncIPv6Response_t IpV6; 00842 #endif 00843 } _SocketAddrResponse_u; 00844 00845 typedef struct 00846 { 00847 _u8 sd; 00848 _u8 backlog; 00849 _u8 padding1; 00850 _u8 padding2; 00851 }_ListenCommand_t; 00852 00853 typedef struct 00854 { 00855 _u8 sd; 00856 _u8 padding0; 00857 _u8 padding1; 00858 _u8 padding2; 00859 }_CloseCommand_t; 00860 00861 00862 typedef struct 00863 { 00864 _u8 nfds; 00865 _u8 readFdsCount; 00866 _u8 writeFdsCount; 00867 _u8 padding; 00868 _u16 readFds; 00869 _u16 writeFds; 00870 _u16 tv_usec; 00871 _u16 tv_sec; 00872 }_SelectCommand_t; 00873 00874 00875 typedef struct 00876 { 00877 _u16 status; 00878 _u8 readFdsCount; 00879 _u8 writeFdsCount; 00880 _u16 readFds; 00881 _u16 writeFds; 00882 }_SelectAsyncResponse_t; 00883 00884 typedef struct 00885 { 00886 _u8 sd; 00887 _u8 level; 00888 _u8 optionName; 00889 _u8 optionLen; 00890 }_setSockOptCommand_t; 00891 00892 typedef struct 00893 { 00894 _u8 sd; 00895 _u8 level; 00896 _u8 optionName; 00897 _u8 optionLen; 00898 }_getSockOptCommand_t; 00899 00900 typedef struct 00901 { 00902 _i16 status; 00903 _u8 sd; 00904 _u8 optionLen; 00905 }_getSockOptResponse_t; 00906 00907 00908 typedef struct 00909 { 00910 _u16 StatusOrLen; 00911 _u8 sd; 00912 _u8 FamilyAndFlags; 00913 }_sendRecvCommand_t; 00914 00915 /***************************************************************************************** 00916 * NETAPP structs 00917 ******************************************************************************************/ 00918 00919 00920 typedef _BasicResponse_t _NetAppStartStopResponse_t; 00921 00922 typedef struct 00923 { 00924 _u32 appId; 00925 }_NetAppStartStopCommand_t; 00926 00927 typedef struct 00928 { 00929 _u16 Status; 00930 _u16 AppId; 00931 _u16 ConfigOpt; 00932 _u16 ConfigLen; 00933 }_NetAppSetGet_t; 00934 typedef struct 00935 { 00936 _u16 port_number; 00937 } _NetAppHttpServerGetSet_port_num_t; 00938 00939 typedef struct 00940 { 00941 _u8 auth_enable; 00942 }_NetAppHttpServerGetSet_auth_enable_t; 00943 00944 typedef struct _sl_NetAppHttpServerGetToken_t 00945 { 00946 _u8 token_name_len; 00947 _u8 padd1; 00948 _u16 padd2; 00949 }sl_NetAppHttpServerGetToken_t; 00950 00951 typedef struct _sl_NetAppHttpServerSendToken_t 00952 { 00953 _u8 token_value_len; 00954 _u8 token_name_len; 00955 _u8 token_name[MAX_TOKEN_NAME_LEN]; 00956 _u16 padd; 00957 }sl_NetAppHttpServerSendToken_t; 00958 00959 typedef struct _sl_NetAppHttpServerPostToken_t 00960 { 00961 _u8 post_action_len; 00962 _u8 token_name_len; 00963 _u8 token_value_len; 00964 _u8 padding; 00965 }sl_NetAppHttpServerPostToken_t; 00966 00967 00968 typedef struct 00969 { 00970 _u16 Len; 00971 _u8 family; 00972 _u8 padding; 00973 }_GetHostByNameCommand_t; 00974 00975 typedef struct 00976 { 00977 _u16 status; 00978 _u16 padding; 00979 _u32 ip0; 00980 _u32 ip1; 00981 _u32 ip2; 00982 _u32 ip3; 00983 }_GetHostByNameIPv6AsyncResponse_t; 00984 00985 typedef struct 00986 { 00987 _u16 status; 00988 _u8 padding1; 00989 _u8 padding2; 00990 _u32 ip0; 00991 }_GetHostByNameIPv4AsyncResponse_t; 00992 00993 00994 00995 00996 typedef enum 00997 { 00998 CTST_BSD_UDP_TX, 00999 CTST_BSD_UDP_RX, 01000 CTST_BSD_TCP_TX, 01001 CTST_BSD_TCP_RX, 01002 CTST_BSD_TCP_SERVER_BI_DIR, 01003 CTST_BSD_TCP_CLIENT_BI_DIR, 01004 CTST_BSD_UDP_BI_DIR, 01005 CTST_BSD_RAW_TX, 01006 CTST_BSD_RAW_RX, 01007 CTST_BSD_RAW_BI_DIR, 01008 CTST_BSD_SECURED_TCP_TX, 01009 CTST_BSD_SECURED_TCP_RX, 01010 CTST_BSD_SECURED_TCP_SERVER_BI_DIR, 01011 CTST_BSD_SECURED_TCP_CLIENT_BI_DIR 01012 }CommTest_e; 01013 01014 typedef struct _sl_protocol_CtestStartCommand_t 01015 { 01016 _u32 Test; 01017 _u16 DestPort; 01018 _u16 SrcPort; 01019 _u32 DestAddr[4]; 01020 _u32 PayloadSize; 01021 _u32 timeout; 01022 _u32 csEnabled; 01023 _u32 secure; 01024 _u32 rawProtocol; 01025 _u8 reserved1[4]; 01026 }_CtestStartCommand_t; 01027 01028 typedef struct 01029 { 01030 _u8 test; 01031 _u8 socket; 01032 _i16 status; 01033 _u32 startTime; 01034 _u32 endTime; 01035 _u16 txKbitsSec; 01036 _u16 rxKbitsSec; 01037 _u32 outOfOrderPackets; 01038 _u32 missedPackets; 01039 }_CtestAsyncResponse_t; 01040 01041 typedef struct 01042 { 01043 _u32 pingIntervalTime; 01044 _u16 PingSize; 01045 _u16 pingRequestTimeout; 01046 _u32 totalNumberOfAttempts; 01047 _u32 flags; 01048 _u32 ip0; 01049 _u32 ip1OrPaadding; 01050 _u32 ip2OrPaadding; 01051 _u32 ip3OrPaadding; 01052 }_PingStartCommand_t; 01053 01054 typedef struct 01055 { 01056 _u16 status; 01057 _u16 rttMin; 01058 _u16 rttMax; 01059 _u16 rttAvg; 01060 _u32 numSuccsessPings; 01061 _u32 numSendsPings; 01062 _u32 testTime; 01063 }_PingReportResponse_t; 01064 01065 01066 typedef struct 01067 { 01068 _u32 ip; 01069 _u32 gateway; 01070 _u32 dns; 01071 }_IpV4AcquiredAsync_t; 01072 01073 01074 typedef enum 01075 { 01076 ACQUIRED_IPV6_LOCAL = 1, 01077 ACQUIRED_IPV6_GLOBAL 01078 }IpV6AcquiredType_e; 01079 01080 01081 typedef struct 01082 { 01083 _u32 type; 01084 _u32 ip[4]; 01085 _u32 gateway[4]; 01086 _u32 dns[4]; 01087 }_IpV6AcquiredAsync_t; 01088 01089 01090 typedef union 01091 { 01092 _SocketCommand_t EventMask; 01093 _sendRecvCommand_t DeviceInit; 01094 }_device_commands_t; 01095 01096 /***************************************************************************************** 01097 * FS structs 01098 ******************************************************************************************/ 01099 01100 typedef struct 01101 { 01102 _u32 FileHandle; 01103 _u32 Offset; 01104 _u16 Len; 01105 _u16 Padding; 01106 }_FsReadCommand_t; 01107 01108 typedef struct 01109 { 01110 _u32 Mode; 01111 _u32 Token; 01112 }_FsOpenCommand_t; 01113 01114 typedef struct 01115 { 01116 _u32 FileHandle; 01117 _u32 Token; 01118 }_FsOpenResponse_t; 01119 01120 01121 typedef struct 01122 { 01123 _u32 FileHandle; 01124 _u32 CertificFileNameLength; 01125 _u32 SignatureLen; 01126 }_FsCloseCommand_t; 01127 01128 01129 typedef _BasicResponse_t _FsReadResponse_t; 01130 typedef _BasicResponse_t _FsDeleteResponse_t; 01131 typedef _BasicResponse_t _FsCloseResponse_t; 01132 01133 typedef struct 01134 { 01135 _u16 Status; 01136 _u16 flags; 01137 _u32 FileLen; 01138 _u32 AllocatedLen; 01139 _u32 Token[4]; 01140 }_FsGetInfoResponse_t; 01141 01142 typedef struct 01143 { 01144 _u8 DeviceID; 01145 _u8 Padding[3]; 01146 }_FsFormatCommand_t; 01147 01148 typedef _BasicResponse_t _FsFormatResponse_t; 01149 01150 typedef struct 01151 { 01152 _u32 Token; 01153 }_FsDeleteCommand_t; 01154 01155 typedef _FsDeleteCommand_t _FsGetInfoCommand_t; 01156 01157 typedef struct 01158 { 01159 _u32 FileHandle; 01160 _u32 Offset; 01161 _u16 Len; 01162 _u16 Padding; 01163 }_FsWriteCommand_t; 01164 01165 typedef _BasicResponse_t _FsWriteResponse_t; 01166 01167 01168 01169 /* Set Max Async Payload length depending on flavor (Tiny, Small, etc.) */ 01170 #define SL_ASYNC_MAX_PAYLOAD_LEN 160 /* size must be aligned to 4 */ 01171 #define SL_ASYNC_MAX_MSG_LEN (_SL_RESP_HDR_SIZE + SL_ASYNC_MAX_PAYLOAD_LEN) 01172 01173 #define RECV_ARGS_SIZE (sizeof(_SocketResponse_t)) 01174 #define RECVFROM_IPV4_ARGS_SIZE (sizeof(_SocketAddrAsyncIPv4Response_t)) 01175 #define RECVFROM_IPV6_ARGS_SIZE (sizeof(_SocketAddrAsyncIPv6Response_t)) 01176 01177 #define SL_IPV4_ADDRESS_SIZE (sizeof(_u32)) 01178 #define SL_IPV6_ADDRESS_SIZE (4 * sizeof(_u32)) 01179 01180 #ifdef __cplusplus 01181 } 01182 #endif /* __cplusplus */ 01183 01184 #endif /* _SL_PROTOCOL_TYPES_H_ */ 01185
Generated on Tue Jul 12 2022 22:55:20 by
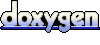