
TI's CC3100 host driver and demo. Experimental and a work in progress.
Embed:
(wiki syntax)
Show/hide line numbers
cc3100_netcfg.cpp
00001 /* 00002 * netcfg.c - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 00039 /*****************************************************************************/ 00040 /* Include files */ 00041 /*****************************************************************************/ 00042 #include "cc3100_simplelink.h" 00043 #include "cc3100_protocol.h" 00044 #include "cc3100_driver.h" 00045 00046 /*****************************************************************************/ 00047 /* sl_NetCfgSet */ 00048 /*****************************************************************************/ 00049 typedef union 00050 { 00051 _NetCfgSetGet_t Cmd; 00052 _BasicResponse_t Rsp; 00053 }_SlNetCfgMsgSet_u; 00054 00055 const _SlCmdCtrl_t _SlNetCfgSetCmdCtrl = 00056 { 00057 SL_OPCODE_DEVICE_NETCFG_SET_COMMAND, 00058 sizeof(_NetCfgSetGet_t), 00059 sizeof(_BasicResponse_t) 00060 }; 00061 00062 #if _SL_INCLUDE_FUNC(sl_NetCfgSet) 00063 _i32 sl_NetCfgSet(_u8 ConfigId ,_u8 ConfigOpt,_u8 ConfigLen, _u8 *pValues) 00064 { 00065 _SlNetCfgMsgSet_u Msg; 00066 _SlCmdExt_t CmdExt; 00067 00068 CmdExt.TxPayloadLen = (ConfigLen+3) & (~3); 00069 CmdExt.RxPayloadLen = 0; 00070 CmdExt.pTxPayload = (_u8 *)pValues; 00071 CmdExt.pRxPayload = NULL; 00072 00073 00074 Msg.Cmd.ConfigId = ConfigId; 00075 Msg.Cmd.ConfigLen = ConfigLen; 00076 Msg.Cmd.ConfigOpt = ConfigOpt; 00077 00078 VERIFY_RET_OK(_SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetCfgSetCmdCtrl, &Msg, &CmdExt)); 00079 00080 return (_i16)Msg.Rsp.status; 00081 } 00082 #endif 00083 00084 00085 /*****************************************************************************/ 00086 /* sl_NetCfgGet */ 00087 /*****************************************************************************/ 00088 typedef union 00089 { 00090 _NetCfgSetGet_t Cmd; 00091 _NetCfgSetGet_t Rsp; 00092 }_SlNetCfgMsgGet_u; 00093 00094 const _SlCmdCtrl_t _SlNetCfgGetCmdCtrl = 00095 { 00096 SL_OPCODE_DEVICE_NETCFG_GET_COMMAND, 00097 sizeof(_NetCfgSetGet_t), 00098 sizeof(_NetCfgSetGet_t) 00099 }; 00100 00101 #if _SL_INCLUDE_FUNC(sl_NetCfgGet) 00102 _i32 sl_NetCfgGet(_u8 ConfigId, _u8 *pConfigOpt,_u8 *pConfigLen, _u8 *pValues) 00103 { 00104 _SlNetCfgMsgGet_u Msg; 00105 _SlCmdExt_t CmdExt; 00106 00107 if (*pConfigLen == 0) 00108 { 00109 return SL_EZEROLEN; 00110 } 00111 CmdExt.TxPayloadLen = 0; 00112 CmdExt.RxPayloadLen = *pConfigLen; 00113 CmdExt.pTxPayload = NULL; 00114 CmdExt.pRxPayload = (_u8 *)pValues; 00115 CmdExt.ActualRxPayloadLen = 0; 00116 Msg.Cmd.ConfigLen = *pConfigLen; 00117 Msg.Cmd.ConfigId = ConfigId; 00118 00119 if( pConfigOpt ) 00120 { 00121 Msg.Cmd.ConfigOpt = (_u16)*pConfigOpt; 00122 } 00123 VERIFY_RET_OK(_SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetCfgGetCmdCtrl, &Msg, &CmdExt)); 00124 00125 if( pConfigOpt ) 00126 { 00127 *pConfigOpt = (_u8)Msg.Rsp.ConfigOpt; 00128 } 00129 if (CmdExt.RxPayloadLen < CmdExt.ActualRxPayloadLen) 00130 { 00131 *pConfigLen = (_u8)CmdExt.RxPayloadLen; 00132 return SL_ESMALLBUF; 00133 } 00134 else 00135 { 00136 *pConfigLen = (_u8)CmdExt.ActualRxPayloadLen; 00137 } 00138 00139 return (_i16)Msg.Rsp.Status; 00140 } 00141 #endif 00142 00143
Generated on Tue Jul 12 2022 22:55:20 by
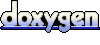