
TI's MQTT Demo with freertos CM4F
Embed:
(wiki syntax)
Show/hide line numbers
server_util.h
00001 /****************************************************************************** 00002 * 00003 * Copyright (C) 2014 Texas Instruments Incorporated 00004 * 00005 * All rights reserved. Property of Texas Instruments Incorporated. 00006 * Restricted rights to use, duplicate or disclose this code are 00007 * granted through contract. 00008 * 00009 * The program may not be used without the written permission of 00010 * Texas Instruments Incorporated or against the terms and conditions 00011 * stipulated in the agreement under which this program has been supplied, 00012 * and under no circumstances can it be used with non-TI connectivity device. 00013 * 00014 ******************************************************************************/ 00015 00016 #ifndef __SERVER_UTIL_H__ 00017 #define __SERVER_UTIL_H__ 00018 00019 #include "mqtt_common.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" 00023 { 00024 #endif 00025 00026 namespace mbed_mqtt { 00027 00028 #define my_malloc malloc 00029 #define my_free free 00030 00031 #define MQTT_SERVER_VERSTR "1.0.1" 00032 00033 #define MIN(a,b) ((a > b)? b : a) 00034 00035 uint16_t mqp_new_id_server(void); 00036 struct mqtt_packet *mqp_server_alloc(uint8_t msg_type, uint32_t buf_sz); 00037 00038 struct mqtt_packet *mqp_server_copy(const struct mqtt_packet *mqp); 00039 00040 extern int32_t (*util_dbg_prn)(const char *fmt, ...); 00041 extern bool util_prn_aux; 00042 00043 void util_mutex_lockin(void); 00044 void util_mutex_unlock(void); 00045 00046 #define MUTEX_LOCKIN() util_mutex_lockin() 00047 #define MUTEX_UNLOCK() util_mutex_unlock() 00048 00049 #define USR_INFO(FMT, ...) if(util_dbg_prn) util_dbg_prn(FMT, ##__VA_ARGS__) 00050 00051 #define DBG_INFO(FMT, ...) \ 00052 if(util_prn_aux && util_dbg_prn) \ 00053 util_dbg_prn(FMT, ##__VA_ARGS__) 00054 00055 void util_params_set(int32_t (*dbg_prn)(const char *fmt, ...), 00056 void *mutex, 00057 void (*mutex_lockin)(void*), 00058 void (*mutex_unlock)(void*)); 00059 00060 }//namespace mbed_mqtt { 00061 00062 #ifdef __cplusplus 00063 } 00064 #endif 00065 00066 #endif 00067
Generated on Wed Jul 13 2022 09:55:39 by
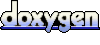