
TI's MQTT Demo with freertos CM4F
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * mqtt_client.c - Sample application to connect to a MQTT broker and 00003 * exercise functionalities like subscribe, publish etc. 00004 * 00005 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00006 * 00007 * 00008 * All rights reserved. Property of Texas Instruments Incorporated. 00009 * Restricted rights to use, duplicate or disclose this code are 00010 * granted through contract. 00011 * 00012 * The program may not be used without the written permission of 00013 * Texas Instruments Incorporated or against the terms and conditions 00014 * stipulated in the agreement under which this program has been supplied, 00015 * and under no circumstances can it be used with non-TI connectivity device. 00016 * 00017 * 00018 * Application Name - MQTT Client 00019 * Application Overview - This application acts as a MQTT client and connects 00020 * to the IBM MQTT broker, simultaneously we can 00021 * connect a web client from a web browser. Both 00022 * clients can inter-communicate using appropriate 00023 * topic names. 00024 * 00025 * Application Details - http://processors.wiki.ti.com/index.php/CC31xx_MQTT_Client 00026 * docs\examples\mqtt_client.pdf 00027 * 00028 */ 00029 00030 /* 00031 * 00032 *! \addtogroup mqtt_client 00033 *! @{ 00034 * 00035 */ 00036 00037 /* Standard includes */ 00038 #include "mbed.h" 00039 00040 #include "simplelink/cc3100_simplelink.h" 00041 #include "simplelink/cc3100_sl_common.h" 00042 #include "simplelink/G_functions/fPtr_func.h" 00043 #include "simplelink/cc3100.h" 00044 00045 #include "myBoardInit.h" 00046 00047 /* Free-RTOS includes */ 00048 #include "FreeRTOS.h" 00049 #include "task.h" 00050 #include "semphr.h" 00051 #include "portmacro.h" 00052 00053 #include "osi.h" 00054 00055 #include <stdio.h> 00056 #include <stdlib.h> 00057 00058 #include "mqtt_client.h" 00059 #include "sl_mqtt_client.h" 00060 #include "mqtt_config.h" 00061 00062 #include "cli_uart.h" 00063 00064 using namespace mbed_cc3100; 00065 using namespace mbed_mqtt; 00066 00067 int32_t demo = 0; 00068 00069 /* Warning Changing pins below you will need the same changes in the following files: 00070 * 00071 * sl_mqtt_client.cpp 00072 * cc31xx_sl_net.cpp 00073 * fPtr_func.cpp 00074 */ 00075 00076 #if (THIS_BOARD == EA_MBED_LPC4088) 00077 //Serial pc(USBTX, USBRX);//EA_lpc4088 00078 DigitalOut led1(LED1); 00079 DigitalOut led2(LED2); 00080 DigitalOut led3(LED3); 00081 DigitalOut led4(LED4); 00082 cc3100 _cc3100(p14, p15, p9, p10, p8, SPI(p5, p6, p7));//LPC4088 irq, nHib, cs, mosi, miso, sck 00083 #elif (THIS_BOARD == Seeed_Arch_Max) 00084 /* Off board leds */ 00085 DigitalOut led1(PB_15); 00086 DigitalOut led2(PB_14); 00087 cc3100 _cc3100(PB_9, PB_8, PD_12, PD_13, PD_11, SPI(PB_5, PB_4, PB_3));//Seeed_Arch_Max irq, nHib, cs, mosi, miso, sck 00088 #elif (THIS_BOARD == LPCXpresso4337) 00089 Serial pc(UART0_TX, UART0_RX); 00090 cc3100 _cc3100(P2_2, P3_5, P1_2, SPI(P1_4, P1_3, PF_4));//LPCXpresso4337 irq, nHib, cs, mosi, miso, sck 00091 #else 00092 00093 #endif 00094 00095 #define APPLICATION_VERSION "1.1.0" 00096 #define SL_STOP_TIMEOUT 0xFF 00097 00098 #undef LOOP_FOREVER 00099 #define LOOP_FOREVER() \ 00100 {\ 00101 while(1) \ 00102 { \ 00103 osi_Sleep(10); \ 00104 } \ 00105 } 00106 00107 00108 #define PRINT_BUF_LEN 128 00109 int8_t print_buf[PRINT_BUF_LEN]; 00110 00111 /* Application specific data types */ 00112 typedef struct connection_config 00113 { 00114 SlMqttClientCtxCfg_t broker_config; 00115 void *clt_ctx; 00116 const char *client_id; 00117 uint8_t *usr_name; 00118 uint8_t *usr_pwd; 00119 bool is_clean; 00120 uint32_t keep_alive_time; 00121 SlMqttClientCbs_t CallBAcks; 00122 int32_t num_topics; 00123 char *topic[SUB_TOPIC_COUNT]; 00124 uint8_t qos[SUB_TOPIC_COUNT]; 00125 SlMqttWill_t will_params; 00126 bool is_connected; 00127 } connect_config; 00128 00129 /* 00130 * GLOBAL VARIABLES -- Start 00131 */ 00132 uint32_t g_publishCount = 0; 00133 OsiMsgQ_t g_PBQueue; /*Message Queue*/ 00134 00135 /* 00136 * GLOBAL VARIABLES -- End 00137 */ 00138 00139 /* 00140 * STATIC FUNCTION DEFINITIONS -- Start 00141 */ 00142 static void displayBanner(void); 00143 static void Mqtt_Recv(void *application_hndl, const char *topstr, int32_t top_len, 00144 const void *payload, int32_t pay_len, bool dup,uint8_t qos, bool retain); 00145 static void sl_MqttEvt(void *application_hndl,int32_t evt, const void *buf, uint32_t len); 00146 static void sl_MqttDisconnect(void *application_hndl); 00147 static int32_t Dummy(const char *inBuff, ...); 00148 static void MqttClient(void *pvParameters); 00149 void buttonHandler_1(void); 00150 void buttonHandler_2(void); 00151 void toggleLed(int ind); 00152 void initLEDs(void); 00153 namespace mbed_cc3100 { 00154 void stackDump(uint32_t stack[]); 00155 } 00156 void printErrorMsg(const char *errMsg); 00157 00158 /* 00159 * STATIC FUNCTION DEFINITIONS -- End 00160 */ 00161 00162 /* 00163 * APPLICATION SPECIFIC DATA -- Start 00164 */ 00165 /* Connection configuration */ 00166 connect_config usr_connect_config[] = 00167 { 00168 { 00169 { 00170 { 00171 SL_MQTT_NETCONN_URL, 00172 SERVER_ADDRESS, 00173 PORT_NUMBER, 00174 0, 00175 0, 00176 0, 00177 NULL 00178 }, 00179 SERVER_MODE, 00180 true, 00181 }, 00182 NULL, 00183 "user007", 00184 // CLIENT_ID, /* Must be unique */ 00185 NULL, 00186 NULL, 00187 true, 00188 KEEP_ALIVE_TIMER, 00189 {&Mqtt_Recv, &sl_MqttEvt, &sl_MqttDisconnect}, 00190 SUB_TOPIC_COUNT, 00191 {SUB_TOPIC1, SUB_TOPIC2}, 00192 {QOS2, QOS2}, 00193 {WILL_TOPIC, WILL_MSG, WILL_QOS, WILL_RETAIN}, 00194 false 00195 } 00196 }; 00197 00198 /* library configuration */ 00199 SlMqttClientLibCfg_t Mqtt_Client = 00200 { 00201 LOOPBACK_PORT_NUMBER, 00202 TASK_PRIORITY, 00203 RCV_TIMEOUT, 00204 true, 00205 (int32_t(*)(const char *, ...))Dummy 00206 }; 00207 00208 void initLEDs(void){ 00209 00210 #if (THIS_BOARD == EA_MBED_LPC4088) 00211 led1 = 1; 00212 led2 = 1; 00213 led3 = 0; 00214 led4 = 0; 00215 #elif (THIS_BOARD == Seeed_Arch_Max) 00216 /* Off board leds */ 00217 led1 = 0; 00218 led2 = 0; 00219 #endif 00220 00221 } 00222 00223 void toggleLed(int ind){ 00224 00225 if(ind == 1){ 00226 led1 = !led1; 00227 } 00228 if(ind == 2){ 00229 led2 = !led2; 00230 } 00231 00232 } 00233 00234 /*Publishing topics and messages*/ 00235 const char *pub_topic_1 = PUB_TOPIC_1; 00236 const char *pub_topic_2 = PUB_TOPIC_2; 00237 const char *data_1={"Push button s1 is pressed: Data 1"}; 00238 const char *data_2={"Push button s2 is pressed: Data 2"}; 00239 void *g_application_hndl = (void*)usr_connect_config; 00240 /* 00241 * APPLICATION SPECIFIC DATA -- End 00242 */ 00243 00244 /*Dummy print message*/ 00245 static int32_t Dummy(const char *inBuff, ...) 00246 { 00247 return 0; 00248 } 00249 00250 /*! 00251 \brief Defines Mqtt_Pub_Message_Receive event handler. 00252 Client App needs to register this event handler with sl_ExtLib_mqtt_Init 00253 API. Background receive task invokes this handler whenever MQTT Client 00254 receives a Publish Message from the broker. 00255 00256 \param[out] topstr => pointer to topic of the message 00257 \param[out] top_len => topic length 00258 \param[out] payload => pointer to payload 00259 \param[out] pay_len => payload length 00260 \param[out] retain => Tells whether its a Retained message or not 00261 \param[out] dup => Tells whether its a duplicate message or not 00262 \param[out] qos => Tells the Qos level 00263 00264 \return none 00265 */ 00266 static void 00267 Mqtt_Recv(void *application_hndl, const char *topstr, int32_t top_len, const void *payload, 00268 int32_t pay_len, bool dup,uint8_t qos, bool retain) 00269 { 00270 int8_t *output_str=(int8_t*)malloc(top_len+1); 00271 memset(output_str,'\0',top_len+1); 00272 strncpy((char*)output_str, topstr, top_len); 00273 output_str[top_len]='\0'; 00274 00275 if(strncmp((char*)output_str, SUB_TOPIC1, top_len) == 0) 00276 { 00277 toggleLed(1); 00278 } 00279 else if(strncmp((char*)output_str, SUB_TOPIC2, top_len) == 0) 00280 { 00281 toggleLed(2); 00282 } 00283 00284 Uart_Write((uint8_t*)"\n\r Publish Message Received\r\n"); 00285 00286 memset(print_buf, 0x00, PRINT_BUF_LEN); 00287 sprintf((char*) print_buf, "\n\r Topic: %s", output_str); 00288 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00289 free(output_str); 00290 00291 memset(print_buf, 0x00, PRINT_BUF_LEN); 00292 sprintf((char*) print_buf, " [Qos: %d] ", qos); 00293 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00294 00295 if(retain){ 00296 Uart_Write((uint8_t*)" [Retained]\r\n"); 00297 } 00298 if(dup){ 00299 Uart_Write((uint8_t*)" [Duplicate]\r\n"); 00300 } 00301 output_str=(int8_t*)malloc(pay_len+1); 00302 memset(output_str,'\0',pay_len+1); 00303 strncpy((char*)output_str, (const char*)payload, pay_len); 00304 output_str[pay_len]='\0'; 00305 00306 memset(print_buf, 0x00, PRINT_BUF_LEN); 00307 sprintf((char*) print_buf, " \n\r Data is: %s\n\r", (char*)output_str); 00308 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00309 00310 free(output_str); 00311 00312 return; 00313 } 00314 00315 /*! 00316 \brief Defines sl_MqttEvt event handler. 00317 Client App needs to register this event handler with sl_ExtLib_mqtt_Init 00318 API. Background receive task invokes this handler whenever MQTT Client 00319 receives an ack(whenever user is in non-blocking mode) or encounters an error. 00320 00321 \param[out] evt => Event that invokes the handler. Event can be of the 00322 following types: 00323 MQTT_ACK - Ack Received 00324 MQTT_ERROR - unknown error 00325 00326 00327 \param[out] buf => points to buffer 00328 \param[out] len => buffer length 00329 00330 \return none 00331 */ 00332 static void 00333 sl_MqttEvt(void *application_hndl,int32_t evt, const void *buf,uint32_t len) 00334 { 00335 int32_t i; 00336 00337 switch(evt) 00338 { 00339 case SL_MQTT_CL_EVT_PUBACK: 00340 { 00341 Uart_Write((uint8_t*)" PubAck:\n\r"); 00342 } 00343 break; 00344 00345 case SL_MQTT_CL_EVT_SUBACK: 00346 { 00347 Uart_Write((uint8_t*)"\n\r Granted QoS Levels\n\r"); 00348 00349 for(i=0;i<len;i++) 00350 { 00351 memset(print_buf, 0x00, PRINT_BUF_LEN); 00352 sprintf((char*) print_buf, " QoS %d\n\r",((uint8_t*)buf)[i]); 00353 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00354 } 00355 } 00356 break; 00357 00358 case SL_MQTT_CL_EVT_UNSUBACK: 00359 { 00360 Uart_Write((uint8_t*)" UnSub Ack:\n\r"); 00361 } 00362 break; 00363 00364 default: 00365 { 00366 Uart_Write((uint8_t*)" [MQTT EVENT] Unexpected event \n\r"); 00367 } 00368 break; 00369 } 00370 } 00371 00372 /*! 00373 00374 \brief callback event in case of MQTT disconnection 00375 00376 \param application_hndl is the handle for the disconnected connection 00377 00378 \return none 00379 00380 */ 00381 static void 00382 sl_MqttDisconnect(void *application_hndl) 00383 { 00384 connect_config *local_con_conf; 00385 osi_messages var = BROKER_DISCONNECTION; 00386 local_con_conf = (connect_config*)application_hndl; 00387 sl_ExtLib_MqttClientUnsub(local_con_conf->clt_ctx, local_con_conf->topic, 00388 SUB_TOPIC_COUNT); 00389 00390 memset(print_buf, 0x00, PRINT_BUF_LEN); 00391 sprintf((char*) print_buf, " Disconnect from broker %s\r\n", 00392 (local_con_conf->broker_config).server_info.server_addr); 00393 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00394 00395 local_con_conf->is_connected = false; 00396 sl_ExtLib_MqttClientCtxDelete(local_con_conf->clt_ctx); 00397 00398 /* 00399 * Write message indicating publish message 00400 */ 00401 osi_MsgQWrite(&g_PBQueue, &var, OSI_NO_WAIT); 00402 } 00403 00404 /* 00405 \brief Application defined hook (or callback) function - assert 00406 00407 \param[in] pcFile - Pointer to the File Name 00408 \param[in] ulLine - Line Number 00409 00410 \return none 00411 */ 00412 void vAssertCalled(const int8_t *pcFile, uint32_t ulLine) 00413 { 00414 /* Handle Assert here */ 00415 while(1) 00416 { 00417 } 00418 } 00419 00420 /* 00421 00422 \brief Application defined idle task hook 00423 00424 \param none 00425 00426 \return none 00427 00428 */ 00429 void vApplicationIdleHook(void) 00430 { 00431 /* Handle Idle Hook for Profiling, Power Management etc */ 00432 } 00433 00434 /* 00435 00436 \brief Application defined malloc failed hook 00437 00438 \param none 00439 00440 \return none 00441 00442 */ 00443 void vApplicationMallocFailedHook(void) 00444 { 00445 /* Handle Memory Allocation Errors */ 00446 while(1) 00447 { 00448 } 00449 } 00450 00451 /* 00452 00453 \brief Application defined stack overflow hook 00454 00455 \param none 00456 00457 \return none 00458 00459 */ 00460 void vApplicationStackOverflowHook( OsiTaskHandle *pxTask, 00461 int8_t *pcTaskName ) 00462 { 00463 /* Handle FreeRTOS Stack Overflow */ 00464 while(1) 00465 { 00466 } 00467 } 00468 00469 /*! 00470 \brief Publishes the message on a topic. 00471 00472 \param[in] clt_ctx - Client context 00473 \param[in] publish_topic - Topic on which the message will be published 00474 \param[in] publish_data - The message that will be published 00475 00476 \return none 00477 */ 00478 static void 00479 publishData(void *clt_ctx, const char *publish_topic, uint8_t *publish_data) 00480 { 00481 // printf("publishData\n\r"); 00482 int32_t retVal = -1; 00483 00484 /* 00485 * Send the publish message 00486 */ 00487 retVal = sl_ExtLib_MqttClientSend((void*)clt_ctx, 00488 publish_topic ,publish_data, strlen((const char*)publish_data), QOS2, RETAIN); 00489 if(retVal < 0) 00490 { 00491 Uart_Write((uint8_t*)"\n\r CC3100 failed to publish the message\n\r"); 00492 return; 00493 } 00494 00495 Uart_Write((uint8_t*)"\n\r CC3100 Publishes the following message \n\r"); 00496 00497 memset(print_buf, 0x00, PRINT_BUF_LEN); 00498 sprintf((char*) print_buf, " Topic: %s\n\r", publish_topic); 00499 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00500 00501 memset(print_buf, 0x00, PRINT_BUF_LEN); 00502 sprintf((char*) print_buf, " Data: %s\n\r", publish_data); 00503 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00504 } 00505 00506 /*! 00507 00508 \brief Task implementing MQTT client communication to other web client through 00509 a broker 00510 00511 \param none 00512 00513 This function 00514 1. Initializes network driver and connects to the default AP 00515 2. Initializes the mqtt library and set up MQTT connection configurations 00516 3. set up the button events and their callbacks(for publishing) 00517 4. handles the callback signals 00518 00519 \return none 00520 00521 */ 00522 static void MqttClient(void *pvParameters) 00523 { 00524 Uart_Write((uint8_t*)"MqttClient\r\n"); 00525 int32_t retVal = -1; 00526 int32_t iCount = 0; 00527 int32_t iNumBroker = 0; 00528 int32_t iConnBroker = 0; 00529 osi_messages RecvQue; 00530 00531 connect_config *local_con_conf = (connect_config *)g_application_hndl; 00532 00533 /* Configure LED */ 00534 initLEDs(); 00535 _cc3100._spi.button1_InterruptEnable(); 00536 _cc3100._spi.button2_InterruptEnable(); 00537 00538 // registerButtonIrqHandler(buttonHandler, NULL); 00539 00540 /* 00541 * Following function configures the device to default state by cleaning 00542 * the persistent settings stored in NVMEM (viz. connection profiles & 00543 * policies, power policy etc) 00544 * 00545 * Applications may choose to skip this step if the developer is sure 00546 * that the device is in its default state at start of application 00547 * 00548 * Note that all profiles and persistent settings that were done on the 00549 * device will be lost 00550 */ 00551 retVal = _cc3100.configureSimpleLinkToDefaultState(); 00552 if(retVal < 0) 00553 { 00554 if(DEVICE_NOT_IN_STATION_MODE == retVal) 00555 Uart_Write((uint8_t*)" Failed to configure the device in its default state \n\r"); 00556 00557 LOOP_FOREVER(); 00558 } 00559 00560 Uart_Write((uint8_t*)" Device is configured in default state \n\r"); 00561 00562 /* 00563 * Assumption is that the device is configured in station mode already 00564 * and it is in its default state 00565 */ 00566 retVal = _cc3100.sl_Start(0, 0, 0); 00567 if ((retVal < 0) || 00568 (ROLE_STA != retVal) ) 00569 { 00570 Uart_Write((uint8_t*)" Failed to start the device \n\r"); 00571 LOOP_FOREVER(); 00572 } 00573 00574 Uart_Write((uint8_t*)" Device started as STATION \n\r"); 00575 00576 /* Connecting to WLAN AP */ 00577 retVal = _cc3100.establishConnectionWithAP(); 00578 if(retVal < 0) 00579 { 00580 Uart_Write((uint8_t*)" Failed to establish connection w/ an AP \n\r"); 00581 LOOP_FOREVER(); 00582 } 00583 00584 Uart_Write((uint8_t*)"\r\n Connection established with AP\n\r"); 00585 00586 /* Initialize MQTT client lib */ 00587 retVal = sl_ExtLib_MqttClientInit(&Mqtt_Client); 00588 if(retVal != 0) 00589 { 00590 /* lib initialization failed */ 00591 Uart_Write((uint8_t*)"MQTT Client lib initialization failed\n\r"); 00592 LOOP_FOREVER(); 00593 } 00594 // Uart_Write((uint8_t*)"MQTT Client lib initialized\n\r"); 00595 00596 /* 00597 * Connection to the broker 00598 */ 00599 iNumBroker = sizeof(usr_connect_config)/sizeof(connect_config); 00600 if(iNumBroker > MAX_BROKER_CONN) 00601 { 00602 Uart_Write((uint8_t*)"Num of brokers are more then max num of brokers\n\r"); 00603 LOOP_FOREVER(); 00604 } 00605 00606 while(iCount < iNumBroker) 00607 { 00608 /* 00609 * create client context 00610 */ 00611 00612 local_con_conf[iCount].clt_ctx = 00613 sl_ExtLib_MqttClientCtxCreate(&local_con_conf[iCount].broker_config, 00614 &local_con_conf[iCount].CallBAcks, 00615 &(local_con_conf[iCount])); 00616 00617 /* 00618 * Set Client ID 00619 */ 00620 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00621 SL_MQTT_PARAM_CLIENT_ID, 00622 local_con_conf[iCount].client_id, 00623 strlen((local_con_conf[iCount].client_id))); 00624 00625 /* 00626 * Set will Params 00627 */ 00628 if(local_con_conf[iCount].will_params.will_topic != NULL) 00629 { 00630 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00631 SL_MQTT_PARAM_WILL_PARAM, 00632 &(local_con_conf[iCount].will_params), 00633 sizeof(SlMqttWill_t)); 00634 } 00635 00636 /* 00637 * Setting user name and password 00638 */ 00639 if(local_con_conf[iCount].usr_name != NULL) 00640 { 00641 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00642 SL_MQTT_PARAM_USER_NAME, 00643 local_con_conf[iCount].usr_name, 00644 strlen((const char*)local_con_conf[iCount].usr_name)); 00645 00646 if(local_con_conf[iCount].usr_pwd != NULL) 00647 { 00648 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00649 SL_MQTT_PARAM_PASS_WORD, 00650 local_con_conf[iCount].usr_pwd, 00651 strlen((const char*)local_con_conf[iCount].usr_pwd)); 00652 } 00653 } 00654 00655 /* 00656 * Connecting to the broker 00657 */ 00658 if((sl_ExtLib_MqttClientConnect((void*)local_con_conf[iCount].clt_ctx, 00659 local_con_conf[iCount].is_clean, 00660 local_con_conf[iCount].keep_alive_time) & 0xFF) != 0) 00661 { 00662 memset(print_buf, 0x00, PRINT_BUF_LEN); 00663 sprintf((char*) print_buf, "\n\r Broker connect failed for conn no. %d \n\r", (int16_t)iCount+1); 00664 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00665 00666 /* 00667 * Delete the context for this connection 00668 */ 00669 sl_ExtLib_MqttClientCtxDelete(local_con_conf[iCount].clt_ctx); 00670 00671 break; 00672 } 00673 else 00674 { 00675 memset(print_buf, 0x00, PRINT_BUF_LEN); 00676 sprintf((char*) print_buf, "\n\r Success: conn to Broker no. %d \n\r ", (int16_t)iCount+1); 00677 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00678 00679 local_con_conf[iCount].is_connected = true; 00680 iConnBroker++; 00681 } 00682 00683 /* 00684 * Subscribe to topics 00685 */ 00686 if(sl_ExtLib_MqttClientSub((void*)local_con_conf[iCount].clt_ctx, 00687 local_con_conf[iCount].topic, 00688 local_con_conf[iCount].qos, SUB_TOPIC_COUNT) < 0) 00689 { 00690 memset(print_buf, 0x00, PRINT_BUF_LEN); 00691 sprintf((char*) print_buf, "\n\r Subscription Error for conn no. %d \n\r", (int16_t)iCount+1); 00692 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00693 00694 Uart_Write((uint8_t*)"Disconnecting from the broker\r\n"); 00695 00696 sl_ExtLib_MqttClientDisconnect(local_con_conf[iCount].clt_ctx); 00697 local_con_conf[iCount].is_connected = false; 00698 00699 /* 00700 * Delete the context for this connection 00701 */ 00702 sl_ExtLib_MqttClientCtxDelete(local_con_conf[iCount].clt_ctx); 00703 iConnBroker--; 00704 break; 00705 } 00706 else 00707 { 00708 int32_t iSub; 00709 00710 Uart_Write((uint8_t*)"Client subscribed on following topics:\n\r"); 00711 for(iSub = 0; iSub < local_con_conf[iCount].num_topics; iSub++) 00712 { 00713 memset(print_buf, 0x00, PRINT_BUF_LEN); 00714 sprintf((char*) print_buf, " %s\n\r", local_con_conf[iCount].topic[iSub]); 00715 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00716 } 00717 } 00718 iCount++; 00719 } 00720 00721 if(iConnBroker < 1) 00722 { 00723 /* 00724 * No successful connection to broker 00725 00726 */ 00727 Uart_Write((uint8_t*)"No successful connections to a broker\r\n"); 00728 goto end; 00729 } 00730 00731 iCount = 0; 00732 00733 for(;;) 00734 { 00735 Uart_Write((uint8_t*)"Waiting for button push event\r\n"); 00736 osi_MsgQRead( &g_PBQueue, &RecvQue, OSI_WAIT_FOREVER); 00737 00738 if(PUSH_BUTTON_1_PRESSED == RecvQue) 00739 { 00740 publishData((void*)local_con_conf[iCount].clt_ctx, pub_topic_1, (uint8_t*)data_1); 00741 _cc3100._spi.button1_InterruptEnable(); 00742 } 00743 else if(PUSH_BUTTON_2_PRESSED == RecvQue) 00744 { 00745 publishData((void*)local_con_conf[iCount].clt_ctx, pub_topic_2, (uint8_t*)data_2); 00746 _cc3100._spi.button2_InterruptEnable(); 00747 } 00748 else if(BROKER_DISCONNECTION == RecvQue) 00749 { 00750 iConnBroker--; 00751 if(iConnBroker < 1) 00752 { 00753 /* 00754 * Device not connected to any broker 00755 */ 00756 goto end; 00757 } 00758 } 00759 } 00760 00761 end: 00762 /* 00763 * De-initializing the client library 00764 */ 00765 sl_ExtLib_MqttClientExit(); 00766 00767 Uart_Write((uint8_t*)"\n\r Exiting the Application\n\r"); 00768 00769 LOOP_FOREVER(); 00770 } 00771 00772 00773 /* 00774 * Application's entry point 00775 */ 00776 int main(int argc, char** argv) 00777 { 00778 int rv = 0; 00779 00780 CLI_Configure(); 00781 00782 memset(print_buf, 0x00, PRINT_BUF_LEN); 00783 sprintf((char*) print_buf, " \r\nSystemCoreClock = %dMHz\r\n ", SystemCoreClock /1000000); 00784 rv = Uart_Write((uint8_t *) print_buf); 00785 if(rv < 0){ 00786 while(1){ 00787 toggleLed(1); 00788 wait(0.1); 00789 } 00790 } 00791 00792 int32_t retVal = -1; 00793 00794 // 00795 // Display Application Banner 00796 // 00797 displayBanner(); 00798 00799 createMutex(); 00800 00801 /* 00802 * Start the SimpleLink Host 00803 */ 00804 retVal = VStartSimpleLinkSpawnTask(SPAWN_TASK_PRIORITY); 00805 if(retVal < 0) 00806 { 00807 Uart_Write((uint8_t*)"VStartSimpleLinkSpawnTask Failed\r\n"); 00808 LOOP_FOREVER(); 00809 } 00810 toggleLed(1); 00811 00812 /* 00813 * Start the MQTT Client task 00814 */ 00815 osi_MsgQCreate(&g_PBQueue,"PBQueue",sizeof(osi_messages),MAX_QUEUE_MSG); 00816 retVal = osi_TaskCreate(MqttClient, 00817 (const int8_t *)"Mqtt Client App", 00818 OSI_STACK_SIZE, NULL, MQTT_APP_TASK_PRIORITY, NULL ); 00819 00820 if(retVal < 0) 00821 { 00822 Uart_Write((uint8_t*)"osi_TaskCreate Failed\r\n"); 00823 LOOP_FOREVER(); 00824 } 00825 00826 /* 00827 * Start the task scheduler 00828 */ 00829 Uart_Write((uint8_t*)"Start the task scheduler\r\n"); 00830 00831 osi_start(); 00832 00833 return 0; 00834 } 00835 00836 /*! 00837 \brief This function displays the application's banner 00838 00839 \param None 00840 00841 \return None 00842 */ 00843 static void displayBanner() 00844 { 00845 Uart_Write((uint8_t*)"\r\n"); 00846 Uart_Write((uint8_t*)" *************************************************\r\n"); 00847 Uart_Write((uint8_t*)" MQTT Client Application - Version\r\n"); 00848 Uart_Write((uint8_t*)" *************************************************\r\n"); 00849 Uart_Write((uint8_t*)"\r\n"); 00850 } 00851 00852 00853 00854
Generated on Wed Jul 13 2022 09:55:38 by
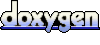