
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
sl_mqtt_server.cpp
00001 /****************************************************************************** 00002 * 00003 * Copyright (C) 2014 Texas Instruments Incorporated 00004 * 00005 * All rights reserved. Property of Texas Instruments Incorporated. 00006 * Restricted rights to use, duplicate or disclose this code are 00007 * granted through contract. 00008 * 00009 * The program may not be used without the written permission of 00010 * Texas Instruments Incorporated or against the terms and conditions 00011 * stipulated in the agreement under which this program has been supplied, 00012 * and under no circumstances can it be used with non-TI connectivity device. 00013 * 00014 ******************************************************************************/ 00015 #include "mbed.h" 00016 #include "mqtt_common.h" 00017 #include "sl_mqtt_server.h" 00018 #include "server_core.h" 00019 #include "client_mgmt.h" 00020 #include "server_util.h" 00021 #include "cc31xx_sl_net.h" 00022 #include "FreeRTOS.h" 00023 #include "osi.h" 00024 00025 namespace mbed_mqtt { 00026 00027 /*------------------------------------------------------------------------- 00028 * MQTT Routines 00029 *------------------------------------------------------------------------- 00030 */ 00031 OsiLockObj_t MutexLockObj; 00032 00033 // wrapper function 00034 static void mutex_lock(void* mutex_hndl) 00035 { 00036 osi_LockObjLock((OsiLockObj_t*)mutex_hndl, OSI_WAIT_FOREVER); 00037 } 00038 00039 // wrapper function 00040 static void mutex_unlock(void* mutex_hndl) 00041 { 00042 osi_LockObjUnlock((OsiLockObj_t*)mutex_hndl); 00043 } 00044 00045 struct mqtt_server_lib_cfg server_cfg = { 00046 0, 00047 0, 00048 &MutexLockObj, 00049 mutex_lock, 00050 mutex_unlock, 00051 NULL /*Debug print*/ 00052 }; 00053 00054 struct mqtt_server_app_cfg app_config = { 00055 NULL 00056 }; 00057 00058 00059 /*Task Priority and Response Time*/ 00060 uint32_t g_srvr_wait_secs,g_srvr_task_priority; 00061 struct device_net_services net_ops = {comm_open,tcp_send,tcp_recv, 00062 send_dest,recv_from,comm_close, 00063 tcp_listen,tcp_accept,tcp_select,rtc_secs}; 00064 void VMqttServerRunTask(const signed char *pvParams); 00065 00066 SlMqttServerCbs_t cbs_obj,*cbs_ptr=NULL; 00067 struct ctrl_struct{ 00068 const void* plugin_hndl; 00069 }app_hndl; 00070 00071 00072 uint16_t sl_server_connect_cb (const struct utf8_string *clientId, 00073 const struct utf8_string *username, 00074 const struct utf8_string *password, 00075 void **app_usr) 00076 { 00077 return( cbs_obj.sl_ExtLib_MqttConn((const char*)MQ_CONN_UTF8_BUF(clientId), 00078 MQ_CONN_UTF8_LEN(clientId), (const char*)MQ_CONN_UTF8_BUF(username), 00079 MQ_CONN_UTF8_LEN(username), (const char*)MQ_CONN_UTF8_BUF(password), 00080 MQ_CONN_UTF8_LEN(password), app_usr)); 00081 } 00082 00083 void sl_server_publish_cb(const struct utf8_string *topic, 00084 const uint8_t *payload, uint32_t pay_len, 00085 bool dup, uint8_t qos, bool retain) 00086 { 00087 cbs_obj.sl_ExtLib_MqttRecv((const char*)MQ_CONN_UTF8_BUF(topic), 00088 MQ_CONN_UTF8_LEN(topic), payload, pay_len, dup, qos, retain); 00089 } 00090 00091 void sl_server_disconn_cb(const void *app_usr, bool due2err) 00092 { 00093 cbs_obj.sl_ExtLib_MqttDisconn((void*)app_usr, due2err); 00094 } 00095 00096 int32_t sl_ExtLib_MqttServerSend(const char *topic, const void *data, int32_t len, 00097 uint8_t qos, bool retain, uint32_t flags) 00098 { 00099 struct utf8_string topic_utf8={NULL}; 00100 topic_utf8.buffer = (char*)topic; 00101 topic_utf8.length = strlen(topic); 00102 return( mqtt_server_app_pub_send(&topic_utf8,(const uint8_t*)data, len, 00103 (enum mqtt_qos)qos, retain)); 00104 } 00105 00106 int32_t sl_ExtLib_MqttTopicEnroll(const char *topic) 00107 { 00108 struct utf8_string topic_utf8={NULL}; 00109 topic_utf8.buffer = (char*)topic; 00110 topic_utf8.length = strlen(topic); 00111 return( mqtt_server_topic_enroll((void*)(app_hndl.plugin_hndl), 00112 &topic_utf8,MQTT_QOS2)); 00113 00114 } 00115 00116 int32_t sl_ExtLib_MqttTopicDisenroll(const char *topic) 00117 { 00118 struct utf8_string topic_utf8={NULL}; 00119 topic_utf8.buffer = (char*)topic; 00120 topic_utf8.length = strlen(topic); 00121 return( mqtt_server_topic_disenroll((void*)&(app_hndl.plugin_hndl), 00122 &topic_utf8)); 00123 } 00124 00125 struct mqtt_server_app_cbs server_appcallbacks = 00126 { 00127 sl_server_connect_cb, 00128 sl_server_publish_cb, 00129 sl_server_disconn_cb 00130 }; 00131 00132 int32_t sl_ExtLib_MqttServerInit(const SlMqttServerCfg_t *cfg, 00133 const SlMqttServerCbs_t *app_cbs) 00134 { 00135 00136 int32_t ret; 00137 00138 //valid loopback port has to be specified for correct operations 00139 if(cfg->loopback_port == 0) 00140 { 00141 return -1; 00142 } 00143 server_cfg.listener_port=cfg->server_info.port_number; 00144 server_cfg.debug_printf = cfg->dbg_print; 00145 cbs_ptr=&cbs_obj; 00146 memcpy(cbs_ptr,app_cbs,sizeof(SlMqttServerCbs_t )); 00147 00148 g_srvr_task_priority=(uint32_t)cfg->rx_tsk_priority; 00149 g_srvr_wait_secs=(uint32_t)cfg->resp_time; 00150 ret = osi_LockObjCreate(&MutexLockObj); 00151 if(!ret) 00152 { 00153 ret=mqtt_server_init(&server_cfg, &app_config); 00154 } 00155 else 00156 { 00157 ret = osi_LockObjDelete(&MutexLockObj); 00158 return(-1); 00159 } 00160 /* registering the device specific implementations for net operations */ 00161 mqtt_server_register_net_svc(&net_ops); 00162 00163 /* registering the apps callbacks */ 00164 app_hndl.plugin_hndl = mqtt_server_app_register(&server_appcallbacks, "temp"); 00165 00166 /* start the Server Run task */ 00167 00168 // osi_TaskCreate(VMqttServerRunTask, (const signed char *) "MQTTServerRun", 2048, NULL, g_srvr_task_priority, NULL ); 00169 00170 return ret; 00171 } 00172 00173 void VMqttServerRunTask(void *pvParams) 00174 { 00175 mqtt_server_run(g_srvr_wait_secs); 00176 } 00177 00178 }//namespace mbed_mqtt 00179 00180
Generated on Tue Jul 12 2022 18:55:10 by
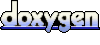