
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
main.cpp
00001 /* 00002 * mqtt_client.c - Sample application to connect to a MQTT broker and 00003 * exercise functionalities like subscribe, publish etc. 00004 * 00005 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00006 * 00007 * 00008 * All rights reserved. Property of Texas Instruments Incorporated. 00009 * Restricted rights to use, duplicate or disclose this code are 00010 * granted through contract. 00011 * 00012 * The program may not be used without the written permission of 00013 * Texas Instruments Incorporated or against the terms and conditions 00014 * stipulated in the agreement under which this program has been supplied, 00015 * and under no circumstances can it be used with non-TI connectivity device. 00016 * 00017 * 00018 * Application Name - MQTT Client 00019 * Application Overview - This application acts as a MQTT client and connects 00020 * to the IBM MQTT broker, simultaneously we can 00021 * connect a web client from a web browser. Both 00022 * clients can inter-communicate using appropriate 00023 * topic names. 00024 * 00025 * Application Details - http://processors.wiki.ti.com/index.php/CC31xx_MQTT_Client 00026 * docs\examples\mqtt_client.pdf 00027 * 00028 */ 00029 00030 /* 00031 * 00032 *! \addtogroup mqtt_client 00033 *! @{ 00034 * 00035 */ 00036 00037 /* Standard includes */ 00038 #include "mbed.h" 00039 00040 #include "simplelink_V2/cc3100_simplelink.h" 00041 #include "simplelink_V2/cc3100_sl_common.h" 00042 #include "simplelink_V2/G_functions/fPtr_func.h" 00043 #include "simplelink_V2/cc3100.h" 00044 #include "cli_uart.h" 00045 #include "myBoardInit.h" 00046 00047 /* Free-RTOS includes */ 00048 //#include "FreeRTOS.h" 00049 //#include "task.h" 00050 //#include "semphr.h" 00051 //#include "portmacro.h" 00052 00053 #include "osi.h" 00054 00055 //#include <stdio.h> 00056 //#include <stdlib.h> 00057 00058 #include "mqtt_client.h" 00059 #include "sl_mqtt_client.h" 00060 #include "mqtt_config.h" 00061 00062 using namespace mbed_cc3100; 00063 using namespace mbed_mqtt; 00064 00065 int32_t demo = 0; 00066 00067 #if (THIS_BOARD == MBED_BOARD_LPC1768) 00068 //Serial pc(USBTX, USBRX);//lpc1768 00069 DigitalOut led1(LED1); 00070 DigitalOut led2(LED2); 00071 // DigitalOut led1(P1_28);/* For LPC1769 */ 00072 // DigitalOut led2(P1_29);/* For LPC1769 */ 00073 cc3100 _cc3100(p16, p17, p9, p10, p8, SPI(p5, p6, p7));//LPC1768 irq, nHib, cs, mosi, miso, sck 00074 //cc3100 _cc3100(p14, p15, p9, p10, p8, SPI(p11, p12, p13));//LPC1768 irq, nHib, cs, mosi, miso, sck 00075 #elif (THIS_BOARD == ST_MBED_NUCLEOF411) 00076 Serial pc(SERIAL_TX, SERIAL_RX);//nucleoF411 00077 cc3100 _cc3100(PA_9, PC_7, PB_6, SPI(PA_7, PA_6, PA_5));//nucleoF411 irq, nHib, cs, mosi, miso, sck 00078 #elif (THIS_BOARD == ST_MBED_NUCLEOF401) 00079 Serial pc(SERIAL_TX, SERIAL_RX);//nucleoF401 00080 DigitalOut led1(LED1); 00081 DigitalOut led2(LED2); 00082 cc3100 _cc3100(PA_8, PA_9, PC_7, PB_6, SPI(PA_7, PA_6, PA_5));//nucleoF401 irq, nHib, cs, mosi, miso, sck 00083 #elif (THIS_BOARD == EA_MBED_LPC4088) 00084 //Serial pc(USBTX, USBRX);//EA_lpc4088 00085 DigitalOut led1(LED1); 00086 DigitalOut led2(LED2); 00087 DigitalOut led3(LED3); 00088 DigitalOut led4(LED4); 00089 cc3100 _cc3100(p14, p15, p9, p10, p8, SPI(p5, p6, p7));//LPC4088 irq, nHib, cs, mosi, miso, sck 00090 #elif (THIS_BOARD == ST_MBED_NUCLEOF103) 00091 Serial pc(SERIAL_TX, SERIAL_RX); 00092 cc3100 _cc3100(PA_9, PC_7, PB_6, SPI(PA_7, PA_6, PA_5));//nucleoF103 irq, nHib, cs, mosi, miso, sck 00093 #elif (THIS_BOARD == Seeed_Arch_Max) 00094 /* Off board leds */ 00095 DigitalOut led1(PB_15); 00096 DigitalOut led2(PB_14); 00097 cc3100 _cc3100(PC_13, PC_6, PE_5, PE_4, PE_6, SPI(PB_5, PB_4, PB_3));//Seeed_Arch_Max irq, nHib, cs, mosi, miso, sck 00098 //SDFileSystem sd(PE_2, PD_11, SPI(PC_3, PC_2, PB_10), "sd", SDFileSystem::SWITCH_NEG_NC, 25000000); 00099 #elif (THIS_BOARD == LPCXpresso4337) 00100 /* LPCXpresso4337 not working due to mbed lib */ 00101 Serial pc(UART0_TX, UART0_RX); 00102 DigitalOut led1(LED1); 00103 DigitalOut led2(LED2); 00104 DigitalOut led3(LED3); 00105 cc3100 _cc3100(P2_12, P2_9, P2_2, P3_5, P1_2, SPI(P1_4, P1_3, PF_4));//LPCXpresso4337 irq, nHib, cs, mosi, miso, sck 00106 #else 00107 00108 #endif 00109 00110 #define APPLICATION_VERSION "1.1.0" 00111 #define SL_STOP_TIMEOUT 0xFF 00112 00113 #undef LOOP_FOREVER 00114 #define LOOP_FOREVER() \ 00115 {\ 00116 while(1) \ 00117 { \ 00118 osi_Sleep(10); \ 00119 } \ 00120 } 00121 00122 00123 #define PRINT_BUF_LEN 128 00124 int8_t print_buf[PRINT_BUF_LEN]; 00125 00126 /* Application specific data types */ 00127 typedef struct connection_config 00128 { 00129 SlMqttClientCtxCfg_t broker_config; 00130 void *clt_ctx; 00131 const char *client_id; 00132 uint8_t *usr_name; 00133 uint8_t *usr_pwd; 00134 bool is_clean; 00135 uint32_t keep_alive_time; 00136 SlMqttClientCbs_t CallBAcks; 00137 int32_t num_topics; 00138 char *topic[SUB_TOPIC_COUNT]; 00139 uint8_t qos[SUB_TOPIC_COUNT]; 00140 SlMqttWill_t will_params; 00141 bool is_connected; 00142 } connect_config; 00143 00144 /* 00145 * GLOBAL VARIABLES -- Start 00146 */ 00147 uint32_t g_publishCount = 0; 00148 OsiMsgQ_t g_PBQueue; /*Message Queue*/ 00149 00150 /* 00151 * GLOBAL VARIABLES -- End 00152 */ 00153 00154 /* 00155 * STATIC FUNCTION DEFINITIONS -- Start 00156 */ 00157 static void displayBanner(void); 00158 static void Mqtt_Recv(void *application_hndl, const char *topstr, int32_t top_len, 00159 const void *payload, int32_t pay_len, bool dup,uint8_t qos, bool retain); 00160 static void sl_MqttEvt(void *application_hndl,int32_t evt, const void *buf, uint32_t len); 00161 static void sl_MqttDisconnect(void *application_hndl); 00162 static int32_t Dummy(const char *inBuff, ...); 00163 static void MqttClient(void *pvParameters); 00164 void toggleLed(int ind); 00165 void initLEDs(void); 00166 00167 /* 00168 * STATIC FUNCTION DEFINITIONS -- End 00169 */ 00170 00171 /* 00172 * APPLICATION SPECIFIC DATA -- Start 00173 */ 00174 /* Connection configuration */ 00175 connect_config usr_connect_config[] = 00176 { 00177 { 00178 { 00179 { 00180 SL_MQTT_NETCONN_URL, 00181 SERVER_ADDRESS, 00182 PORT_NUMBER, 00183 0, 00184 0, 00185 0, 00186 NULL 00187 }, 00188 SERVER_MODE, 00189 true, 00190 }, 00191 NULL, 00192 "user1", 00193 // CLIENT_ID, /* Must be unique */ 00194 NULL, 00195 NULL, 00196 true, 00197 KEEP_ALIVE_TIMER, 00198 {&Mqtt_Recv, &sl_MqttEvt, &sl_MqttDisconnect}, 00199 SUB_TOPIC_COUNT, 00200 {SUB_TOPIC1, SUB_TOPIC2}, 00201 {QOS2, QOS2}, 00202 {WILL_TOPIC, WILL_MSG, WILL_QOS, WILL_RETAIN}, 00203 false 00204 } 00205 }; 00206 00207 /* library configuration */ 00208 SlMqttClientLibCfg_t Mqtt_Client = 00209 { 00210 LOOPBACK_PORT_NUMBER, 00211 TASK_PRIORITY, 00212 RCV_TIMEOUT, 00213 true, 00214 (int32_t(*)(const char *, ...))Dummy 00215 }; 00216 00217 void initLEDs(void){ 00218 00219 #if (THIS_BOARD == EA_MBED_LPC4088) 00220 led1 = 1; 00221 led2 = 1; 00222 led3 = 0; 00223 led4 = 0; 00224 #elif (THIS_BOARD == LPCXpresso4337) 00225 led1 = 0; 00226 led2 = 0; 00227 led3 = 0; 00228 #else 00229 led1 = 0; 00230 led2 = 0; 00231 #endif 00232 00233 } 00234 00235 void toggleLed(int ind){ 00236 00237 if(ind == 1){ 00238 led1 = !led1; 00239 } 00240 if(ind == 2){ 00241 led2 = !led2; 00242 } 00243 00244 } 00245 00246 /*Publishing topics and messages*/ 00247 const char *pub_topic_1 = PUB_TOPIC_1; 00248 const char *pub_topic_2 = PUB_TOPIC_2; 00249 const char *data_1={"Push button sw1 is pressed: Data 1"}; 00250 const char *data_2={"Push button sw2 is pressed: Data 2"}; 00251 void *g_application_hndl = (void*)usr_connect_config; 00252 /* 00253 * APPLICATION SPECIFIC DATA -- End 00254 */ 00255 00256 /*Dummy print message*/ 00257 static int32_t Dummy(const char *inBuff, ...) 00258 { 00259 return 0; 00260 } 00261 00262 /*! 00263 \brief Defines Mqtt_Pub_Message_Receive event handler. 00264 Client App needs to register this event handler with sl_ExtLib_mqtt_Init 00265 API. Background receive task invokes this handler whenever MQTT Client 00266 receives a Publish Message from the broker. 00267 00268 \param[out] topstr => pointer to topic of the message 00269 \param[out] top_len => topic length 00270 \param[out] payload => pointer to payload 00271 \param[out] pay_len => payload length 00272 \param[out] retain => Tells whether its a Retained message or not 00273 \param[out] dup => Tells whether its a duplicate message or not 00274 \param[out] qos => Tells the Qos level 00275 00276 \return none 00277 */ 00278 static void 00279 Mqtt_Recv(void *application_hndl, const char *topstr, int32_t top_len, const void *payload, 00280 int32_t pay_len, bool dup,uint8_t qos, bool retain) 00281 { 00282 int8_t *output_str=(int8_t*)malloc(top_len+1); 00283 memset(output_str,'\0',top_len+1); 00284 strncpy((char*)output_str, topstr, top_len); 00285 output_str[top_len]='\0'; 00286 00287 if(strncmp((char*)output_str, SUB_TOPIC1, top_len) == 0) 00288 { 00289 toggleLed(1); 00290 } 00291 else if(strncmp((char*)output_str, SUB_TOPIC2, top_len) == 0) 00292 { 00293 toggleLed(2); 00294 } 00295 00296 Uart_Write((uint8_t*)"\n\r Publish Message Received\r\n"); 00297 00298 memset(print_buf, 0x00, PRINT_BUF_LEN); 00299 sprintf((char*) print_buf, "\n\r Topic: %s", output_str); 00300 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00301 free(output_str); 00302 00303 memset(print_buf, 0x00, PRINT_BUF_LEN); 00304 sprintf((char*) print_buf, " [Qos: %d] ", qos); 00305 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00306 00307 if(retain){ 00308 Uart_Write((uint8_t*)" [Retained]\r\n"); 00309 } 00310 if(dup){ 00311 Uart_Write((uint8_t*)" [Duplicate]\r\n"); 00312 } 00313 output_str=(int8_t*)malloc(pay_len+1); 00314 memset(output_str,'\0',pay_len+1); 00315 strncpy((char*)output_str, (const char*)payload, pay_len); 00316 output_str[pay_len]='\0'; 00317 00318 memset(print_buf, 0x00, PRINT_BUF_LEN); 00319 sprintf((char*) print_buf, " \n\r Data is: %s\n\r", (char*)output_str); 00320 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00321 00322 free(output_str); 00323 00324 return; 00325 } 00326 00327 /*! 00328 \brief Defines sl_MqttEvt event handler. 00329 Client App needs to register this event handler with sl_ExtLib_mqtt_Init 00330 API. Background receive task invokes this handler whenever MQTT Client 00331 receives an ack(whenever user is in non-blocking mode) or encounters an error. 00332 00333 \param[out] evt => Event that invokes the handler. Event can be of the 00334 following types: 00335 MQTT_ACK - Ack Received 00336 MQTT_ERROR - unknown error 00337 00338 00339 \param[out] buf => points to buffer 00340 \param[out] len => buffer length 00341 00342 \return none 00343 */ 00344 static void 00345 sl_MqttEvt(void *application_hndl,int32_t evt, const void *buf,uint32_t len) 00346 { 00347 int32_t i; 00348 00349 switch(evt) 00350 { 00351 case SL_MQTT_CL_EVT_PUBACK: 00352 { 00353 Uart_Write((uint8_t*)" PubAck:\n\r"); 00354 } 00355 break; 00356 00357 case SL_MQTT_CL_EVT_SUBACK: 00358 { 00359 Uart_Write((uint8_t*)"\n\r Granted QoS Levels\n\r"); 00360 00361 for(i=0;i<len;i++) 00362 { 00363 memset(print_buf, 0x00, PRINT_BUF_LEN); 00364 sprintf((char*) print_buf, " QoS %d\n\r",((uint8_t*)buf)[i]); 00365 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00366 } 00367 } 00368 break; 00369 00370 case SL_MQTT_CL_EVT_UNSUBACK: 00371 { 00372 Uart_Write((uint8_t*)" UnSub Ack:\n\r"); 00373 } 00374 break; 00375 00376 default: 00377 { 00378 Uart_Write((uint8_t*)" [MQTT EVENT] Unexpected event \n\r"); 00379 } 00380 break; 00381 } 00382 } 00383 00384 /*! 00385 00386 \brief callback event in case of MQTT disconnection 00387 00388 \param application_hndl is the handle for the disconnected connection 00389 00390 \return none 00391 00392 */ 00393 static void 00394 sl_MqttDisconnect(void *application_hndl) 00395 { 00396 connect_config *local_con_conf; 00397 osi_messages var = BROKER_DISCONNECTION; 00398 local_con_conf = (connect_config*)application_hndl; 00399 sl_ExtLib_MqttClientUnsub(local_con_conf->clt_ctx, local_con_conf->topic, 00400 SUB_TOPIC_COUNT); 00401 00402 memset(print_buf, 0x00, PRINT_BUF_LEN); 00403 sprintf((char*) print_buf, " Disconnect from broker %s\r\n", 00404 (local_con_conf->broker_config).server_info.server_addr); 00405 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00406 00407 local_con_conf->is_connected = false; 00408 sl_ExtLib_MqttClientCtxDelete(local_con_conf->clt_ctx); 00409 00410 /* 00411 * Write message indicating publish message 00412 */ 00413 osi_MsgQWrite(&g_PBQueue, &var, OSI_NO_WAIT); 00414 } 00415 00416 /* 00417 \brief Application defined hook (or callback) function - assert 00418 00419 \param[in] pcFile - Pointer to the File Name 00420 \param[in] ulLine - Line Number 00421 00422 \return none 00423 */ 00424 void vAssertCalled(const int8_t *pcFile, uint32_t ulLine) 00425 { 00426 /* Handle Assert here */ 00427 while(1) 00428 { 00429 } 00430 } 00431 00432 /* 00433 00434 \brief Application defined idle task hook 00435 00436 \param none 00437 00438 \return none 00439 00440 */ 00441 void vApplicationIdleHook(void) 00442 { 00443 /* Handle Idle Hook for Profiling, Power Management etc */ 00444 } 00445 00446 /* 00447 00448 \brief Application defined malloc failed hook 00449 00450 \param none 00451 00452 \return none 00453 00454 */ 00455 void vApplicationMallocFailedHook(void) 00456 { 00457 /* Handle Memory Allocation Errors */ 00458 while(1) 00459 { 00460 } 00461 } 00462 00463 /* 00464 00465 \brief Application defined stack overflow hook 00466 00467 \param none 00468 00469 \return none 00470 00471 */ 00472 void vApplicationStackOverflowHook( OsiTaskHandle *pxTask, 00473 int8_t *pcTaskName ) 00474 { 00475 /* Handle FreeRTOS Stack Overflow */ 00476 while(1) 00477 { 00478 } 00479 } 00480 00481 /*! 00482 \brief Publishes the message on a topic. 00483 00484 \param[in] clt_ctx - Client context 00485 \param[in] publish_topic - Topic on which the message will be published 00486 \param[in] publish_data - The message that will be published 00487 00488 \return none 00489 */ 00490 static void 00491 publishData(void *clt_ctx, const char *publish_topic, uint8_t *publish_data) 00492 { 00493 // printf("publishData\n\r"); 00494 int32_t retVal = -1; 00495 00496 /* 00497 * Send the publish message 00498 */ 00499 retVal = sl_ExtLib_MqttClientSend((void*)clt_ctx, 00500 publish_topic ,publish_data, strlen((const char*)publish_data), QOS2, RETAIN); 00501 if(retVal < 0) 00502 { 00503 Uart_Write((uint8_t*)"\n\r CC3100 failed to publish the message\n\r"); 00504 return; 00505 } 00506 00507 Uart_Write((uint8_t*)"\n\r CC3100 Publishes the following message \n\r"); 00508 00509 memset(print_buf, 0x00, PRINT_BUF_LEN); 00510 sprintf((char*) print_buf, " Topic: %s\n\r", publish_topic); 00511 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00512 00513 memset(print_buf, 0x00, PRINT_BUF_LEN); 00514 sprintf((char*) print_buf, " Data: %s\n\r", publish_data); 00515 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00516 } 00517 00518 /*! 00519 00520 \brief Task implementing MQTT client communication to other web client through 00521 a broker 00522 00523 \param none 00524 00525 This function 00526 1. Initializes network driver and connects to the default AP 00527 2. Initializes the mqtt library and set up MQTT connection configurations 00528 3. set up the button events and their callbacks(for publishing) 00529 4. handles the callback signals 00530 00531 \return none 00532 00533 */ 00534 static void MqttClient(void *pvParameters) 00535 { 00536 //Uart_Write((uint8_t*)"MqttClient\r\n"); 00537 int32_t retVal = -1; 00538 int32_t iCount = 0; 00539 int32_t iNumBroker = 0; 00540 int32_t iConnBroker = 0; 00541 osi_messages RecvQue; 00542 00543 connect_config *local_con_conf = (connect_config *)g_application_hndl; 00544 00545 /* Configure LED */ 00546 initLEDs(); 00547 _cc3100._spi.button1_InterruptEnable(); 00548 _cc3100._spi.button2_InterruptEnable(); 00549 // registerButtonIrqHandler(buttonHandler, NULL); 00550 00551 /* 00552 * Following function configures the device to default state by cleaning 00553 * the persistent settings stored in NVMEM (viz. connection profiles & 00554 * policies, power policy etc) 00555 * 00556 * Applications may choose to skip this step if the developer is sure 00557 * that the device is in its default state at start of application 00558 * 00559 * Note that all profiles and persistent settings that were done on the 00560 * device will be lost 00561 */ 00562 retVal = _cc3100.configureSimpleLinkToDefaultState(); 00563 if(retVal < 0) 00564 { 00565 if(DEVICE_NOT_IN_STATION_MODE == retVal) 00566 Uart_Write((uint8_t*)" Failed to configure the device in its default state \n\r"); 00567 00568 LOOP_FOREVER(); 00569 } 00570 00571 Uart_Write((uint8_t*)" Device is configured in default state \n\r"); 00572 00573 /* 00574 * Assumption is that the device is configured in station mode already 00575 * and it is in its default state 00576 */ 00577 retVal = _cc3100.sl_Start(0, 0, 0); 00578 if ((retVal < 0) || 00579 (ROLE_STA != retVal) ) 00580 { 00581 Uart_Write((uint8_t*)" Failed to start the device \n\r"); 00582 LOOP_FOREVER(); 00583 } 00584 00585 Uart_Write((uint8_t*)" Device started as STATION \n\r"); 00586 00587 /* Connecting to WLAN AP */ 00588 retVal = _cc3100.establishConnectionWithAP(); 00589 if(retVal < 0) 00590 { 00591 Uart_Write((uint8_t*)" Failed to establish connection w/ an AP \n\r"); 00592 LOOP_FOREVER(); 00593 } 00594 00595 Uart_Write((uint8_t*)"\r\n Connection established with AP\n\r"); 00596 00597 /* Initialize MQTT client lib */ 00598 retVal = sl_ExtLib_MqttClientInit(&Mqtt_Client); 00599 if(retVal != 0) 00600 { 00601 /* lib initialization failed */ 00602 Uart_Write((uint8_t*)"MQTT Client lib initialization failed\n\r"); 00603 LOOP_FOREVER(); 00604 } 00605 // Uart_Write((uint8_t*)"MQTT Client lib initialized\n\r"); 00606 00607 /* 00608 * Connection to the broker 00609 */ 00610 iNumBroker = sizeof(usr_connect_config)/sizeof(connect_config); 00611 if(iNumBroker > MAX_BROKER_CONN) 00612 { 00613 Uart_Write((uint8_t*)"Num of brokers are more then max num of brokers\n\r"); 00614 LOOP_FOREVER(); 00615 } 00616 // Uart_Write((uint8_t*)"Num of brokers %i\n\r",iNumBroker); 00617 00618 while(iCount < iNumBroker) 00619 { 00620 /* 00621 * create client context 00622 */ 00623 00624 local_con_conf[iCount].clt_ctx = 00625 sl_ExtLib_MqttClientCtxCreate(&local_con_conf[iCount].broker_config, 00626 &local_con_conf[iCount].CallBAcks, 00627 &(local_con_conf[iCount])); 00628 00629 /* 00630 * Set Client ID 00631 */ 00632 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00633 SL_MQTT_PARAM_CLIENT_ID, 00634 local_con_conf[iCount].client_id, 00635 strlen((local_con_conf[iCount].client_id))); 00636 00637 /* 00638 * Set will Params 00639 */ 00640 if(local_con_conf[iCount].will_params.will_topic != NULL) 00641 { 00642 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00643 SL_MQTT_PARAM_WILL_PARAM, 00644 &(local_con_conf[iCount].will_params), 00645 sizeof(SlMqttWill_t)); 00646 } 00647 00648 /* 00649 * Setting user name and password 00650 */ 00651 if(local_con_conf[iCount].usr_name != NULL) 00652 { 00653 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00654 SL_MQTT_PARAM_USER_NAME, 00655 local_con_conf[iCount].usr_name, 00656 strlen((const char*)local_con_conf[iCount].usr_name)); 00657 00658 if(local_con_conf[iCount].usr_pwd != NULL) 00659 { 00660 sl_ExtLib_MqttClientSet((void*)local_con_conf[iCount].clt_ctx, 00661 SL_MQTT_PARAM_PASS_WORD, 00662 local_con_conf[iCount].usr_pwd, 00663 strlen((const char*)local_con_conf[iCount].usr_pwd)); 00664 } 00665 } 00666 00667 /* 00668 * Connecting to the broker 00669 */ 00670 if((sl_ExtLib_MqttClientConnect((void*)local_con_conf[iCount].clt_ctx, 00671 local_con_conf[iCount].is_clean, 00672 local_con_conf[iCount].keep_alive_time) & 0xFF) != 0) 00673 { 00674 memset(print_buf, 0x00, PRINT_BUF_LEN); 00675 sprintf((char*) print_buf, "\n\r Broker connect failed for conn no. %d \n\r", (int16_t)iCount+1); 00676 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00677 00678 /* 00679 * Delete the context for this connection 00680 */ 00681 sl_ExtLib_MqttClientCtxDelete(local_con_conf[iCount].clt_ctx); 00682 00683 break; 00684 } 00685 else 00686 { 00687 memset(print_buf, 0x00, PRINT_BUF_LEN); 00688 sprintf((char*) print_buf, "\n\r Success: conn to Broker no. %d \n\r ", (int16_t)iCount+1); 00689 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00690 00691 local_con_conf[iCount].is_connected = true; 00692 iConnBroker++; 00693 } 00694 00695 /* 00696 * Subscribe to topics 00697 */ 00698 if(sl_ExtLib_MqttClientSub((void*)local_con_conf[iCount].clt_ctx, 00699 local_con_conf[iCount].topic, 00700 local_con_conf[iCount].qos, SUB_TOPIC_COUNT) < 0) 00701 { 00702 memset(print_buf, 0x00, PRINT_BUF_LEN); 00703 sprintf((char*) print_buf, "\n\r Subscription Error for conn no. %d \n\r", (int16_t)iCount+1); 00704 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00705 00706 Uart_Write((uint8_t*)"Disconnecting from the broker\r\n"); 00707 00708 sl_ExtLib_MqttClientDisconnect(local_con_conf[iCount].clt_ctx); 00709 local_con_conf[iCount].is_connected = false; 00710 00711 /* 00712 * Delete the context for this connection 00713 */ 00714 sl_ExtLib_MqttClientCtxDelete(local_con_conf[iCount].clt_ctx); 00715 iConnBroker--; 00716 break; 00717 } 00718 else 00719 { 00720 int32_t iSub; 00721 00722 Uart_Write((uint8_t*)"Client subscribed on following topics:\n\r"); 00723 for(iSub = 0; iSub < local_con_conf[iCount].num_topics; iSub++) 00724 { 00725 memset(print_buf, 0x00, PRINT_BUF_LEN); 00726 sprintf((char*) print_buf, " %s\n\r", local_con_conf[iCount].topic[iSub]); 00727 Uart_Write((uint8_t*)print_buf); Uart_Write((uint8_t*)"\r\n"); 00728 } 00729 } 00730 iCount++; 00731 } 00732 00733 if(iConnBroker < 1) 00734 { 00735 /* 00736 * No successful connection to broker 00737 00738 */ 00739 Uart_Write((uint8_t*)"No successful connections to a broker\r\n"); 00740 goto end; 00741 } 00742 00743 iCount = 0; 00744 00745 for(;;) 00746 { 00747 Uart_Write((uint8_t*)"Waiting for button push event\r\n"); 00748 osi_MsgQRead( &g_PBQueue, &RecvQue, OSI_WAIT_FOREVER); 00749 00750 if(PUSH_BUTTON_1_PRESSED == RecvQue) 00751 { 00752 publishData((void*)local_con_conf[iCount].clt_ctx, pub_topic_1, (uint8_t*)data_1); 00753 _cc3100._spi.button1_InterruptEnable(); 00754 } 00755 else if(PUSH_BUTTON_2_PRESSED == RecvQue) 00756 { 00757 publishData((void*)local_con_conf[iCount].clt_ctx, pub_topic_2, (uint8_t*)data_2); 00758 _cc3100._spi.button2_InterruptEnable(); 00759 } 00760 else if(BROKER_DISCONNECTION == RecvQue) 00761 { 00762 iConnBroker--; 00763 if(iConnBroker < 1) 00764 { 00765 /* 00766 * Device not connected to any broker 00767 */ 00768 Uart_Write((uint8_t*)"\n\r Device not connected to any broker\n\r"); 00769 goto end; 00770 } 00771 } 00772 //Uart_Write((uint8_t*)"\n\r Unknown event occured\n\r"); 00773 } 00774 00775 end: 00776 /* 00777 * De-initializing the client library 00778 */ 00779 sl_ExtLib_MqttClientExit(); 00780 00781 Uart_Write((uint8_t*)"\n\r Exiting the Application\n\r"); 00782 00783 LOOP_FOREVER(); 00784 } 00785 00786 00787 /* 00788 * Application's entry point 00789 */ 00790 int main(int argc, char** argv) 00791 { 00792 int rv = 0; 00793 // SCB->SHCSR |= 0x00070000;//Enable fault handler. 00794 // pc.baud(115200); 00795 initLEDs(); 00796 00797 CLI_Configure(); 00798 00799 00800 printf(" \r\nSystemCoreClock = %dMHz\r\n ", SystemCoreClock /1000000); 00801 00802 memset(print_buf, 0x00, PRINT_BUF_LEN); 00803 sprintf((char*) print_buf, " \r\nSystemCoreClock = %dMHz\r\n ", SystemCoreClock /1000000); 00804 rv = Uart_Write((uint8_t *) print_buf); 00805 if(rv < 0){ 00806 while(1){ 00807 toggleLed(1); 00808 wait(0.1); 00809 } 00810 } 00811 00812 int32_t retVal = -1; 00813 00814 // 00815 // Display Application Banner 00816 // 00817 displayBanner(); 00818 00819 createMutex(); 00820 00821 /* 00822 * Start the SimpleLink Host 00823 */ 00824 retVal = VStartSimpleLinkSpawnTask(SPAWN_TASK_PRIORITY); 00825 if(retVal < 0) 00826 { 00827 Uart_Write((uint8_t*)"VStartSimpleLinkSpawnTask Failed\r\n"); 00828 LOOP_FOREVER(); 00829 } 00830 00831 // retVal = _cc3100.initializeAppVariables(); 00832 // ASSERT_ON_ERROR(retVal); 00833 00834 // _cc3100.CLR_STATUS_BIT(g_Status, STATUS_BIT_PING_DONE); 00835 // g_PingPacketsRecv = 0; 00836 00837 /* 00838 * Start the MQTT Client task 00839 */ 00840 osi_MsgQCreate(&g_PBQueue,"PBQueue",sizeof(osi_messages),MAX_QUEUE_MSG); 00841 retVal = osi_TaskCreate(MqttClient, 00842 (const int8_t *)"Mqtt Client App", 00843 OSI_STACK_SIZE, NULL, MQTT_APP_TASK_PRIORITY, NULL ); 00844 00845 if(retVal < 0) 00846 { 00847 Uart_Write((uint8_t*)"osi_TaskCreate Failed\r\n"); 00848 LOOP_FOREVER(); 00849 } 00850 00851 /* 00852 * Start the task scheduler 00853 */ 00854 Uart_Write((uint8_t*)"Start the task scheduler\r\n"); 00855 osi_start(); 00856 00857 return 0; 00858 } 00859 00860 /*! 00861 \brief This function displays the application's banner 00862 00863 \param None 00864 00865 \return None 00866 */ 00867 static void displayBanner() 00868 { 00869 Uart_Write((uint8_t*)"\r\n"); 00870 Uart_Write((uint8_t*)" *************************************************\r\n"); 00871 Uart_Write((uint8_t*)" MQTT Client Application - Version\r\n"); 00872 Uart_Write((uint8_t*)" *************************************************\r\n"); 00873 Uart_Write((uint8_t*)"\r\n"); 00874 } 00875 00876 00877
Generated on Tue Jul 12 2022 18:55:10 by
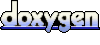