
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
cli_uart.cpp
00001 00002 #include "mbed.h" 00003 #include "myBoardInit.h" 00004 #include "cli_uart.h" 00005 #include "osi.h" 00006 #include "stdio.h" 00007 00008 OsiLockObj_t g_printLock; 00009 #if (THIS_BOARD == Seeed_Arch_Max) 00010 Serial uart(PA_9, PA_10); 00011 #elif (THIS_BOARD == EA_MBED_LPC4088) 00012 Serial uart(p37, p31); 00013 #elif (THIS_BOARD == MBED_BOARD_LPC1768) 00014 Serial uart(p13, p14); 00015 #elif (THIS_BOARD == LPCXpresso4337) 00016 Serial uart(P3_4, P1_14); 00017 Serial uart0(UART0_TX, UART0_RX); 00018 #endif 00019 00020 int Uart_Write(unsigned char *inBuff) 00021 { 00022 uint16_t ret, ecount, usLength = strlen((const char *)inBuff); 00023 ecount = 0; 00024 ret = 0; 00025 00026 while(!(uart.writeable())){ecount++;if(ecount>3000)break;}; 00027 00028 if(uart.writeable()) { 00029 00030 if(inBuff == NULL) { 00031 printf("Uart Write buffer empty\r\n"); 00032 return -1; 00033 } 00034 00035 RTOS_MUTEX_ACQUIRE(&g_printLock); 00036 ret = usLength; 00037 00038 while (usLength) { 00039 uart.putc(*inBuff); 00040 usLength--; 00041 inBuff++; 00042 } 00043 00044 RTOS_MUTEX_RELEASE(&g_printLock); 00045 } else { 00046 // printf("Uart Write failed [uart not writeable] now trying printf\r\n"); 00047 while (usLength) { 00048 printf("%c",*inBuff); 00049 usLength--; 00050 inBuff++; 00051 } 00052 return -1; 00053 } 00054 00055 return (int)ret; 00056 00057 } 00058 00059 void CLI_Configure(void) 00060 { 00061 uart.baud(115200); 00062 // uart0.baud(115200); 00063 00064 RTOS_MUTEX_CREATE(&g_printLock); 00065 00066 }
Generated on Tue Jul 12 2022 18:55:10 by
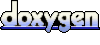