
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
cc31xx_sl_net.h
00001 /****************************************************************************** 00002 * 00003 * Copyright (C) 2014 Texas Instruments Incorporated 00004 * 00005 * All rights reserved. Property of Texas Instruments Incorporated. 00006 * Restricted rights to use, duplicate or disclose this code are 00007 * granted through contract. 00008 * 00009 * The program may not be used without the written permission of 00010 * Texas Instruments Incorporated or against the terms and conditions 00011 * stipulated in the agreement under which this program has been supplied, 00012 * and under no circumstances can it be used with non-TI connectivity device. 00013 * 00014 ******************************************************************************/ 00015 00016 /* 00017 Network Services for General Purpose Linux environment 00018 */ 00019 00020 #ifndef __CC31XX_SL_NET_H__ 00021 #define __CC31XX_SL_NET_H__ 00022 00023 #include "cc3100_simplelink.h" 00024 #include "mqtt_client.h" /* conn options */ 00025 00026 #ifdef __cplusplus 00027 extern "C" 00028 { 00029 #endif 00030 00031 namespace mbed_mqtt { 00032 00033 //***************************************************************************** 00034 // MACROS 00035 //***************************************************************************** 00036 00037 // MACRO to include receive time out feature 00038 #define SOC_RCV_TIMEOUT_OPT 1 00039 00040 00041 /*----------------------------------------------------------------------------- 00042 definitions needed for the functions in this file 00043 -----------------------------------------------------------------------------*/ 00044 00045 00046 /*----------------------------------------------------------------------------- 00047 prototypes of functions 00048 -----------------------------------------------------------------------------*/ 00049 00050 int32_t comm_open(uint32_t nwconn_opts, const char *server_addr, uint16_t port_number, 00051 const struct secure_conn *nw_security); 00052 int32_t tcp_send(int32_t comm, const uint8_t *buf, uint32_t len, void *ctx); 00053 int32_t tcp_recv(int32_t comm, uint8_t *buf, uint32_t len, uint32_t wait_secs, bool *timed_out, 00054 void *ctx); 00055 int32_t comm_close(int32_t comm); 00056 uint32_t rtc_secs(void); 00057 00058 /*-----------------functions added for server -----------------------------*/ 00059 00060 int32_t tcp_listen(uint32_t nwconn_info, uint16_t port_number, 00061 const struct secure_conn *nw_security); 00062 00063 int32_t tcp_select(int32_t *recv_cvec, int32_t *send_cvec, int32_t *rsvd_cvec, 00064 uint32_t wait_secs); 00065 00066 00067 int32_t tcp_accept(int32_t listen_hnd, uint8_t *client_ip, 00068 uint32_t *ip_length); 00069 00070 /*----------------- adding functions for udp functionalities -------------------*/ 00071 00072 /** Send a UDP packet 00073 00074 @param[in] comm communication entity handle; socket handle in this case 00075 @param[in] buf buf to be sent in the udp packet 00076 @param[in] len length of the buffer buf 00077 @param[in] dest_port destination port number 00078 @param[in] dest_ip ip address of the destination in dot notation, interpretted as a string; 00079 Only IPV4 is supported currently. 00080 @param[in] ip_len length of string dest_ip; currently not used 00081 @return number of bytes sent or error returned by sl_SendTo call 00082 */ 00083 int32_t send_dest(int32_t, const uint8_t *buf, uint32_t len, uint16_t dest_port, const uint8_t *dest_ip, uint32_t ip_len); 00084 00085 /** Recieve a UDP packet 00086 00087 @param[in] comm communication entity handle; socket handle in this case 00088 @param[out] buf buf into which received UDP packet is written 00089 @param[in] maximum len length of the buffer buf 00090 @param[out] from_port port number of UDP packet source 00091 @param[out] from_ip ip address of the UDP packet source. 00092 The ip address is to be interpreted as a uint32_t number in network byte ordering 00093 Only IPV4 is supported currently. 00094 @param[out] ip_len length of string from_ip; 00095 currently always populated with 4 as the address is a IPV4 address 00096 @return number of bytes received or error returned by sl_RecvFrom call 00097 */ 00098 int32_t recv_from(int32_t comm, uint8_t *buf, uint32_t len, uint16_t *from_port, uint8_t *from_ip, uint32_t *ip_len); 00099 00100 }//namespace mbed_mqtt 00101 00102 #ifdef __cplusplus 00103 } 00104 #endif 00105 00106 #endif 00107
Generated on Tue Jul 12 2022 18:55:10 by
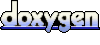