
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
cc3100_wlan.cpp
00001 /* 00002 * wlan.c - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 00039 /*****************************************************************************/ 00040 /* Include files */ 00041 /*****************************************************************************/ 00042 #include "cc3100_simplelink.h" 00043 #include "cc3100_protocol.h" 00044 #include "cc3100_driver.h" 00045 00046 #include "cc3100_wlan.h" 00047 #include "cc3100_wlan_rx_filters.h" 00048 00049 /*****************************************************************************/ 00050 /* Macro declarations */ 00051 /*****************************************************************************/ 00052 #define MAX_SSID_LEN (32) 00053 #define MAX_KEY_LEN (63) 00054 #define MAX_USER_LEN (32) 00055 #define MAX_ANON_USER_LEN (32) 00056 #define MAX_SMART_CONFIG_KEY (16) 00057 00058 namespace mbed_cc3100 { 00059 00060 cc3100_wlan::cc3100_wlan(cc3100_driver &driver, cc3100_wlan_rx_filters &wlan_filters) 00061 : _driver(driver), _wlan_filters(wlan_filters) 00062 { 00063 00064 } 00065 00066 cc3100_wlan::~cc3100_wlan() 00067 { 00068 00069 } 00070 00071 /***************************************************************************** 00072 sl_WlanConnect 00073 *****************************************************************************/ 00074 typedef struct { 00075 _WlanConnectEapCommand_t Args; 00076 int8_t Strings[MAX_SSID_LEN + MAX_KEY_LEN + MAX_USER_LEN + MAX_ANON_USER_LEN]; 00077 } _WlanConnectCmd_t; 00078 00079 typedef union { 00080 _WlanConnectCmd_t Cmd; 00081 _BasicResponse_t Rsp; 00082 } _SlWlanConnectMsg_u; 00083 00084 00085 #if _SL_INCLUDE_FUNC(sl_WlanConnect) 00086 int16_t cc3100_wlan::sl_WlanConnect(const signed char* pName, const int16_t NameLen, const uint8_t *pMacAddr, const SlSecParams_t* pSecParams , const SlSecParamsExt_t* pSecExtParams) 00087 { 00088 _SlWlanConnectMsg_u Msg = {0}; 00089 _SlCmdCtrl_t CmdCtrl = {0}; 00090 00091 CmdCtrl.TxDescLen = 0;/* init */ 00092 CmdCtrl.RxDescLen = sizeof(_BasicResponse_t); 00093 00094 /* verify SSID length */ 00095 VERIFY_PROTOCOL(NameLen <= MAX_SSID_LEN); 00096 /* verify SSID is not NULL */ 00097 if( NULL == pName ) 00098 { 00099 return SL_INVALPARAM; 00100 } 00101 00102 /* update SSID length */ 00103 Msg.Cmd.Args.Common.SsidLen = (uint8_t)NameLen; 00104 /* Profile with no security */ 00105 /* Enterprise security profile */ 00106 if (NULL != pSecExtParams) { 00107 /* Update command opcode */ 00108 CmdCtrl.Opcode = SL_OPCODE_WLAN_WLANCONNECTEAPCOMMAND; 00109 CmdCtrl.TxDescLen += sizeof(_WlanConnectEapCommand_t); 00110 /* copy SSID */ 00111 memcpy(EAP_SSID_STRING(&Msg), pName, NameLen); 00112 CmdCtrl.TxDescLen += NameLen; 00113 /* Copy password if supplied */ 00114 if ((NULL != pSecParams) && (pSecParams->KeyLen > 0)) { 00115 /* update security type */ 00116 Msg.Cmd.Args.Common.SecType = pSecParams->Type; 00117 /* verify key length */ 00118 if (pSecParams->KeyLen > MAX_KEY_LEN) { 00119 return SL_INVALPARAM; 00120 } 00121 /* update key length */ 00122 Msg.Cmd.Args.Common.PasswordLen = pSecParams->KeyLen; 00123 ARG_CHECK_PTR(pSecParams->Key); 00124 /* copy key */ 00125 memcpy(EAP_PASSWORD_STRING(&Msg), pSecParams->Key, pSecParams->KeyLen); 00126 CmdCtrl.TxDescLen += pSecParams->KeyLen; 00127 } else { 00128 Msg.Cmd.Args.Common.PasswordLen = 0; 00129 } 00130 ARG_CHECK_PTR(pSecExtParams); 00131 /* Update Eap bitmask */ 00132 Msg.Cmd.Args.EapBitmask = pSecExtParams->EapMethod; 00133 /* Update Certificate file ID index - currently not supported */ 00134 Msg.Cmd.Args.CertIndex = pSecExtParams->CertIndex; 00135 /* verify user length */ 00136 if (pSecExtParams->UserLen > MAX_USER_LEN) { 00137 return SL_INVALPARAM; 00138 } 00139 Msg.Cmd.Args.UserLen = pSecExtParams->UserLen; 00140 /* copy user name (identity) */ 00141 if(pSecExtParams->UserLen > 0) { 00142 memcpy(EAP_USER_STRING(&Msg), pSecExtParams->User, pSecExtParams->UserLen); 00143 CmdCtrl.TxDescLen += pSecExtParams->UserLen; 00144 } 00145 /* verify Anonymous user length */ 00146 if (pSecExtParams->AnonUserLen > MAX_ANON_USER_LEN) { 00147 return SL_INVALPARAM; 00148 } 00149 Msg.Cmd.Args.AnonUserLen = pSecExtParams->AnonUserLen; 00150 /* copy Anonymous user */ 00151 if(pSecExtParams->AnonUserLen > 0) { 00152 memcpy(EAP_ANON_USER_STRING(&Msg), pSecExtParams->AnonUser, pSecExtParams->AnonUserLen); 00153 CmdCtrl.TxDescLen += pSecExtParams->AnonUserLen; 00154 } 00155 00156 } 00157 00158 /* Regular or open security profile */ 00159 else { 00160 /* Update command opcode */ 00161 CmdCtrl.Opcode = SL_OPCODE_WLAN_WLANCONNECTCOMMAND; 00162 CmdCtrl.TxDescLen += sizeof(_WlanConnectCommon_t); 00163 /* copy SSID */ 00164 memcpy(SSID_STRING(&Msg), pName, NameLen); 00165 CmdCtrl.TxDescLen += NameLen; 00166 /* Copy password if supplied */ 00167 if( NULL != pSecParams ) { 00168 /* update security type */ 00169 Msg.Cmd.Args.Common.SecType = pSecParams->Type; 00170 /* verify key length is valid */ 00171 if (pSecParams->KeyLen > MAX_KEY_LEN) { 00172 return SL_INVALPARAM; 00173 } 00174 /* update key length */ 00175 Msg.Cmd.Args.Common.PasswordLen = pSecParams->KeyLen; 00176 CmdCtrl.TxDescLen += pSecParams->KeyLen; 00177 /* copy key (could be no key in case of WPS pin) */ 00178 if( NULL != pSecParams->Key ) { 00179 memcpy(PASSWORD_STRING(&Msg), pSecParams->Key, pSecParams->KeyLen); 00180 } 00181 } 00182 /* Profile with no security */ 00183 else { 00184 Msg.Cmd.Args.Common.PasswordLen = 0; 00185 Msg.Cmd.Args.Common.SecType = SL_SEC_TYPE_OPEN; 00186 } 00187 } 00188 /* If BSSID is not null, copy to buffer, otherwise set to 0 */ 00189 if(NULL != pMacAddr) { 00190 memcpy(Msg.Cmd.Args.Common.Bssid, pMacAddr, sizeof(Msg.Cmd.Args.Common.Bssid)); 00191 } else { 00192 _driver._SlDrvMemZero(Msg.Cmd.Args.Common.Bssid, sizeof(Msg.Cmd.Args.Common.Bssid)); 00193 } 00194 00195 VERIFY_RET_OK ( _driver._SlDrvCmdOp(&CmdCtrl, &Msg, NULL)); 00196 return (int16_t)Msg.Rsp.status; 00197 } 00198 #endif 00199 00200 /*******************************************************************************/ 00201 /* sl_Disconnect */ 00202 /* ******************************************************************************/ 00203 #if _SL_INCLUDE_FUNC(sl_WlanDisconnect) 00204 int16_t cc3100_wlan::sl_WlanDisconnect(void) 00205 { 00206 return _driver._SlDrvBasicCmd(SL_OPCODE_WLAN_WLANDISCONNECTCOMMAND); 00207 } 00208 #endif 00209 00210 /******************************************************************************/ 00211 /* sl_PolicySet */ 00212 /******************************************************************************/ 00213 typedef union { 00214 _WlanPoliciySetGet_t Cmd; 00215 _BasicResponse_t Rsp; 00216 } _SlPolicyMsg_u; 00217 00218 #if _SL_INCLUDE_FUNC(sl_WlanPolicySet) 00219 const _SlCmdCtrl_t _SlPolicySetCmdCtrl = { 00220 SL_OPCODE_WLAN_POLICYSETCOMMAND, 00221 sizeof(_WlanPoliciySetGet_t), 00222 sizeof(_BasicResponse_t) 00223 }; 00224 00225 int16_t cc3100_wlan::sl_WlanPolicySet(const uint8_t Type , const uint8_t Policy, uint8_t *pVal, const uint8_t ValLen) 00226 { 00227 00228 _SlPolicyMsg_u Msg; 00229 _SlCmdExt_t CmdExt; 00230 00231 _driver._SlDrvResetCmdExt(&CmdExt); 00232 CmdExt.TxPayloadLen = ValLen; 00233 CmdExt.pTxPayload = (uint8_t *)pVal; 00234 00235 Msg.Cmd.PolicyType = Type; 00236 Msg.Cmd.PolicyOption = Policy; 00237 Msg.Cmd.PolicyOptionLen = ValLen; 00238 00239 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlPolicySetCmdCtrl, &Msg, &CmdExt)); 00240 00241 return (int16_t)Msg.Rsp.status; 00242 } 00243 #endif 00244 00245 00246 /******************************************************************************/ 00247 /* sl_PolicyGet */ 00248 /******************************************************************************/ 00249 typedef union { 00250 _WlanPoliciySetGet_t Cmd; 00251 _WlanPoliciySetGet_t Rsp; 00252 } _SlPolicyGetMsg_u; 00253 00254 #if _SL_INCLUDE_FUNC(sl_WlanPolicyGet) 00255 const _SlCmdCtrl_t _SlPolicyGetCmdCtrl = { 00256 SL_OPCODE_WLAN_POLICYGETCOMMAND, 00257 sizeof(_WlanPoliciySetGet_t), 00258 sizeof(_WlanPoliciySetGet_t) 00259 }; 00260 00261 int16_t cc3100_wlan::sl_WlanPolicyGet(const uint8_t Type , uint8_t Policy,uint8_t *pVal,uint8_t *pValLen) 00262 { 00263 _SlPolicyGetMsg_u Msg; 00264 _SlCmdExt_t CmdExt; 00265 00266 if (*pValLen == 0) { 00267 return SL_EZEROLEN; 00268 } 00269 _driver._SlDrvResetCmdExt(&CmdExt); 00270 CmdExt.RxPayloadLen = *pValLen; 00271 CmdExt.pRxPayload = pVal; 00272 00273 Msg.Cmd.PolicyType = Type; 00274 Msg.Cmd.PolicyOption = Policy; 00275 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlPolicyGetCmdCtrl, &Msg, &CmdExt)); 00276 00277 00278 if (CmdExt.RxPayloadLen < CmdExt.ActualRxPayloadLen) { 00279 *pValLen = Msg.Rsp.PolicyOptionLen; 00280 return SL_ESMALLBUF; 00281 } else { 00282 /* no pointer valus, fill the results into int8_t */ 00283 *pValLen = (uint8_t)CmdExt.ActualRxPayloadLen; 00284 if( 0 == CmdExt.ActualRxPayloadLen ) { 00285 *pValLen = 1; 00286 pVal[0] = Msg.Rsp.PolicyOption; 00287 } 00288 } 00289 return (int16_t)SL_OS_RET_CODE_OK; 00290 } 00291 #endif 00292 00293 00294 /*******************************************************************************/ 00295 /* sl_ProfileAdd */ 00296 /*******************************************************************************/ 00297 typedef struct { 00298 _WlanAddGetEapProfile_t Args; 00299 int8_t Strings[MAX_SSID_LEN + MAX_KEY_LEN + MAX_USER_LEN + MAX_ANON_USER_LEN]; 00300 } _SlProfileParams_t; 00301 00302 typedef union { 00303 _SlProfileParams_t Cmd; 00304 _BasicResponse_t Rsp; 00305 } _SlProfileAddMsg_u; 00306 00307 00308 00309 #if _SL_INCLUDE_FUNC(sl_WlanProfileAdd) 00310 int16_t cc3100_wlan::sl_WlanProfileAdd(const int8_t* pName, 00311 const int16_t NameLen, 00312 const uint8_t *pMacAddr, 00313 const SlSecParams_t* pSecParams , 00314 const SlSecParamsExt_t* pSecExtParams, 00315 const uint32_t Priority, 00316 const uint32_t Options) 00317 { 00318 _SlProfileAddMsg_u Msg; 00319 _SlCmdCtrl_t CmdCtrl = {0}; 00320 CmdCtrl.TxDescLen = 0;/* init */ 00321 CmdCtrl.RxDescLen = sizeof(_BasicResponse_t); 00322 00323 /* update priority */ 00324 Msg.Cmd.Args.Common.Priority = (uint8_t)Priority; 00325 /* verify SSID is not NULL */ 00326 if( NULL == pName ) 00327 { 00328 return SL_INVALPARAM; 00329 } 00330 00331 /* verify SSID length */ 00332 VERIFY_PROTOCOL(NameLen <= MAX_SSID_LEN); 00333 /* update SSID length */ 00334 Msg.Cmd.Args.Common.SsidLen = (uint8_t)NameLen; 00335 00336 00337 /* Enterprise security profile */ 00338 if (NULL != pSecExtParams) { 00339 /* Update command opcode */ 00340 CmdCtrl.Opcode = SL_OPCODE_WLAN_EAP_PROFILEADDCOMMAND; 00341 CmdCtrl.TxDescLen += sizeof(_WlanAddGetEapProfile_t); 00342 00343 /* copy SSID */ 00344 memcpy(EAP_PROFILE_SSID_STRING(&Msg), pName, NameLen); 00345 CmdCtrl.TxDescLen += NameLen; 00346 00347 /* Copy password if supplied */ 00348 if ((NULL != pSecParams) && (pSecParams->KeyLen > 0)) { 00349 /* update security type */ 00350 Msg.Cmd.Args.Common.SecType = pSecParams->Type; 00351 00352 if( SL_SEC_TYPE_WEP == Msg.Cmd.Args.Common.SecType ) { 00353 Msg.Cmd.Args.Common.WepKeyId = 0; 00354 } 00355 00356 /* verify key length */ 00357 if (pSecParams->KeyLen > MAX_KEY_LEN) { 00358 return SL_INVALPARAM; 00359 } 00360 VERIFY_PROTOCOL(pSecParams->KeyLen <= MAX_KEY_LEN); 00361 /* update key length */ 00362 Msg.Cmd.Args.Common.PasswordLen = pSecParams->KeyLen; 00363 CmdCtrl.TxDescLen += pSecParams->KeyLen; 00364 ARG_CHECK_PTR(pSecParams->Key); 00365 /* copy key */ 00366 memcpy(EAP_PROFILE_PASSWORD_STRING(&Msg), pSecParams->Key, pSecParams->KeyLen); 00367 } else { 00368 Msg.Cmd.Args.Common.PasswordLen = 0; 00369 } 00370 00371 ARG_CHECK_PTR(pSecExtParams); 00372 /* Update Eap bitmask */ 00373 Msg.Cmd.Args.EapBitmask = pSecExtParams->EapMethod; 00374 /* Update Certificate file ID index - currently not supported */ 00375 Msg.Cmd.Args.CertIndex = pSecExtParams->CertIndex; 00376 /* verify user length */ 00377 if (pSecExtParams->UserLen > MAX_USER_LEN) { 00378 return SL_INVALPARAM; 00379 } 00380 Msg.Cmd.Args.UserLen = pSecExtParams->UserLen; 00381 /* copy user name (identity) */ 00382 if(pSecExtParams->UserLen > 0) { 00383 memcpy(EAP_PROFILE_USER_STRING(&Msg), pSecExtParams->User, pSecExtParams->UserLen); 00384 CmdCtrl.TxDescLen += pSecExtParams->UserLen; 00385 } 00386 00387 /* verify Anonymous user length (for tunneled) */ 00388 if (pSecExtParams->AnonUserLen > MAX_ANON_USER_LEN) { 00389 return SL_INVALPARAM; 00390 } 00391 Msg.Cmd.Args.AnonUserLen = pSecExtParams->AnonUserLen; 00392 00393 /* copy Anonymous user */ 00394 if(pSecExtParams->AnonUserLen > 0) { 00395 memcpy(EAP_PROFILE_ANON_USER_STRING(&Msg), pSecExtParams->AnonUser, pSecExtParams->AnonUserLen); 00396 CmdCtrl.TxDescLen += pSecExtParams->AnonUserLen; 00397 } 00398 00399 } 00400 /* Regular or open security profile */ 00401 else { 00402 /* Update command opcode */ 00403 CmdCtrl.Opcode = SL_OPCODE_WLAN_PROFILEADDCOMMAND; 00404 /* update commnad length */ 00405 CmdCtrl.TxDescLen += sizeof(_WlanAddGetProfile_t); 00406 00407 if (NULL != pName) { 00408 /* copy SSID */ 00409 memcpy(PROFILE_SSID_STRING(&Msg), pName, NameLen); 00410 CmdCtrl.TxDescLen += NameLen; 00411 } 00412 00413 /* Copy password if supplied */ 00414 if( NULL != pSecParams ) { 00415 /* update security type */ 00416 Msg.Cmd.Args.Common.SecType = pSecParams->Type; 00417 00418 if( SL_SEC_TYPE_WEP == Msg.Cmd.Args.Common.SecType ) { 00419 Msg.Cmd.Args.Common.WepKeyId = 0; 00420 } 00421 00422 /* verify key length */ 00423 if (pSecParams->KeyLen > MAX_KEY_LEN) { 00424 return SL_INVALPARAM; 00425 } 00426 /* update key length */ 00427 Msg.Cmd.Args.Common.PasswordLen = pSecParams->KeyLen; 00428 CmdCtrl.TxDescLen += pSecParams->KeyLen; 00429 /* copy key (could be no key in case of WPS pin) */ 00430 if( NULL != pSecParams->Key ) { 00431 memcpy(PROFILE_PASSWORD_STRING(&Msg), pSecParams->Key, pSecParams->KeyLen); 00432 } 00433 } else { 00434 Msg.Cmd.Args.Common.SecType = SL_SEC_TYPE_OPEN; 00435 Msg.Cmd.Args.Common.PasswordLen = 0; 00436 } 00437 00438 } 00439 00440 00441 /* If BSSID is not null, copy to buffer, otherwise set to 0 */ 00442 if(NULL != pMacAddr) { 00443 memcpy(Msg.Cmd.Args.Common.Bssid, pMacAddr, sizeof(Msg.Cmd.Args.Common.Bssid)); 00444 } else { 00445 _driver._SlDrvMemZero(Msg.Cmd.Args.Common.Bssid, sizeof(Msg.Cmd.Args.Common.Bssid)); 00446 } 00447 00448 VERIFY_RET_OK(_driver._SlDrvCmdOp(&CmdCtrl, &Msg, NULL)); 00449 00450 return (int16_t)Msg.Rsp.status; 00451 } 00452 #endif 00453 00454 /*******************************************************************************/ 00455 /* sl_ProfileGet */ 00456 /*******************************************************************************/ 00457 typedef union { 00458 _WlanProfileDelGetCommand_t Cmd; 00459 _SlProfileParams_t Rsp; 00460 } _SlProfileGetMsg_u; 00461 00462 #if _SL_INCLUDE_FUNC(sl_WlanProfileGet) 00463 const _SlCmdCtrl_t _SlProfileGetCmdCtrl = { 00464 SL_OPCODE_WLAN_PROFILEGETCOMMAND, 00465 sizeof(_WlanProfileDelGetCommand_t), 00466 sizeof(_SlProfileParams_t) 00467 }; 00468 00469 int16_t cc3100_wlan::sl_WlanProfileGet(const int16_t Index,int8_t* pName, int16_t *pNameLen, uint8_t *pMacAddr, SlSecParams_t* pSecParams, SlGetSecParamsExt_t* pEntParams, uint32_t *pPriority) 00470 { 00471 _SlProfileGetMsg_u Msg; 00472 Msg.Cmd.index = (uint8_t)Index; 00473 00474 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlProfileGetCmdCtrl, &Msg, NULL)); 00475 00476 pSecParams->Type = Msg.Rsp.Args.Common.SecType; 00477 /* since password is not transferred in getprofile, password length should always be zero */ 00478 pSecParams->KeyLen = Msg.Rsp.Args.Common.PasswordLen; 00479 if (NULL != pEntParams) { 00480 pEntParams->UserLen = Msg.Rsp.Args.UserLen; 00481 /* copy user name */ 00482 if (pEntParams->UserLen > 0) { 00483 memcpy(pEntParams->User, EAP_PROFILE_USER_STRING(&Msg), pEntParams->UserLen); 00484 } 00485 pEntParams->AnonUserLen = Msg.Rsp.Args.AnonUserLen; 00486 /* copy anonymous user name */ 00487 if (pEntParams->AnonUserLen > 0) { 00488 memcpy(pEntParams->AnonUser, EAP_PROFILE_ANON_USER_STRING(&Msg), pEntParams->AnonUserLen); 00489 } 00490 } 00491 00492 *pNameLen = Msg.Rsp.Args.Common.SsidLen; 00493 *pPriority = Msg.Rsp.Args.Common.Priority; 00494 00495 if (NULL != Msg.Rsp.Args.Common.Bssid) { 00496 memcpy(pMacAddr, Msg.Rsp.Args.Common.Bssid, sizeof(Msg.Rsp.Args.Common.Bssid)); 00497 } 00498 00499 memcpy(pName, EAP_PROFILE_SSID_STRING(&Msg), *pNameLen); 00500 00501 return (int16_t)Msg.Rsp.Args.Common.SecType; 00502 00503 } 00504 #endif 00505 00506 /*******************************************************************************/ 00507 /* sl_ProfileDel */ 00508 /*******************************************************************************/ 00509 typedef union { 00510 _WlanProfileDelGetCommand_t Cmd; 00511 _BasicResponse_t Rsp; 00512 } _SlProfileDelMsg_u; 00513 00514 #if _SL_INCLUDE_FUNC(sl_WlanProfileDel) 00515 const _SlCmdCtrl_t _SlProfileDelCmdCtrl = { 00516 SL_OPCODE_WLAN_PROFILEDELCOMMAND, 00517 sizeof(_WlanProfileDelGetCommand_t), 00518 sizeof(_BasicResponse_t) 00519 }; 00520 00521 int16_t cc3100_wlan::sl_WlanProfileDel(const int16_t Index) 00522 { 00523 _SlProfileDelMsg_u Msg; 00524 00525 Msg.Cmd.index = (uint8_t)Index; 00526 00527 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlProfileDelCmdCtrl, &Msg, NULL)); 00528 00529 return (int16_t)Msg.Rsp.status; 00530 } 00531 #endif 00532 00533 /******************************************************************************/ 00534 /* sl_WlanGetNetworkList */ 00535 /******************************************************************************/ 00536 typedef union { 00537 _WlanGetNetworkListCommand_t Cmd; 00538 _WlanGetNetworkListResponse_t Rsp; 00539 } _SlWlanGetNetworkListMsg_u; 00540 00541 #if _SL_INCLUDE_FUNC(sl_WlanGetNetworkList) 00542 const _SlCmdCtrl_t _SlWlanGetNetworkListCtrl = { 00543 SL_OPCODE_WLAN_SCANRESULTSGETCOMMAND, 00544 sizeof(_WlanGetNetworkListCommand_t), 00545 sizeof(_WlanGetNetworkListResponse_t) 00546 }; 00547 00548 int16_t cc3100_wlan::sl_WlanGetNetworkList(const uint8_t Index, const uint8_t Count, Sl_WlanNetworkEntry_t *pEntries) 00549 { 00550 int16_t retVal = 0; 00551 _SlWlanGetNetworkListMsg_u Msg; 00552 _SlCmdExt_t CmdExt; 00553 00554 if (Count == 0) { 00555 return SL_EZEROLEN; 00556 } 00557 00558 _driver._SlDrvResetCmdExt(&CmdExt); 00559 CmdExt.RxPayloadLen = sizeof(Sl_WlanNetworkEntry_t)*(Count); 00560 CmdExt.pRxPayload = (uint8_t *)pEntries; 00561 00562 Msg.Cmd.index = Index; 00563 Msg.Cmd.count = Count; 00564 00565 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlWlanGetNetworkListCtrl, &Msg, &CmdExt)); 00566 retVal = Msg.Rsp.status; 00567 00568 return (int16_t)retVal; 00569 } 00570 #endif 00571 00572 /*******************************************************************************/ 00573 /* sl_WlanRxStatStart */ 00574 /*******************************************************************************/ 00575 #if _SL_INCLUDE_FUNC(sl_WlanRxStatStart) 00576 int16_t cc3100_wlan::sl_WlanRxStatStart(void) 00577 { 00578 return _driver._SlDrvBasicCmd(SL_OPCODE_WLAN_STARTRXSTATCOMMAND); 00579 } 00580 #endif 00581 00582 #if _SL_INCLUDE_FUNC(sl_WlanRxStatStop) 00583 int16_t cc3100_wlan::sl_WlanRxStatStop(void) 00584 { 00585 return _driver._SlDrvBasicCmd(SL_OPCODE_WLAN_STOPRXSTATCOMMAND); 00586 } 00587 #endif 00588 00589 #if _SL_INCLUDE_FUNC(sl_WlanRxStatGet) 00590 int16_t cc3100_wlan::sl_WlanRxStatGet(SlGetRxStatResponse_t *pRxStat, const uint32_t Flags) 00591 { 00592 _SlCmdCtrl_t CmdCtrl = {SL_OPCODE_WLAN_GETRXSTATCOMMAND, 0, sizeof(SlGetRxStatResponse_t)}; 00593 _driver._SlDrvMemZero(pRxStat, sizeof(SlGetRxStatResponse_t)); 00594 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&CmdCtrl, pRxStat, NULL)); 00595 00596 return 0; 00597 } 00598 #endif 00599 00600 00601 00602 /******************************************************************************/ 00603 /* sl_WlanSmartConfigStop */ 00604 /******************************************************************************/ 00605 #if _SL_INCLUDE_FUNC(sl_WlanSmartConfigStop) 00606 int16_t cc3100_wlan::sl_WlanSmartConfigStop(void) 00607 { 00608 return _driver._SlDrvBasicCmd(SL_OPCODE_WLAN_SMART_CONFIG_STOP_COMMAND); 00609 } 00610 #endif 00611 00612 00613 /******************************************************************************/ 00614 /* sl_WlanSmartConfigStart */ 00615 /******************************************************************************/ 00616 00617 00618 typedef struct { 00619 _WlanSmartConfigStartCommand_t Args; 00620 int8_t Strings[3 * MAX_SMART_CONFIG_KEY]; /* public key + groupId1 key + groupId2 key */ 00621 } _SlSmartConfigStart_t; 00622 00623 typedef union { 00624 _SlSmartConfigStart_t Cmd; 00625 _BasicResponse_t Rsp; 00626 } _SlSmartConfigStartMsg_u; 00627 00628 const _SlCmdCtrl_t _SlSmartConfigStartCmdCtrl = { 00629 SL_OPCODE_WLAN_SMART_CONFIG_START_COMMAND, 00630 sizeof(_SlSmartConfigStart_t), 00631 sizeof(_BasicResponse_t) 00632 }; 00633 00634 #if _SL_INCLUDE_FUNC(sl_WlanSmartConfigStart) 00635 int16_t cc3100_wlan::sl_WlanSmartConfigStart( const uint32_t groupIdBitmask, 00636 const uint8_t cipher, 00637 const uint8_t publicKeyLen, 00638 const uint8_t group1KeyLen, 00639 const uint8_t group2KeyLen, 00640 const uint8_t* pPublicKey, 00641 const uint8_t* pGroup1Key, 00642 const uint8_t* pGroup2Key) 00643 { 00644 _SlSmartConfigStartMsg_u Msg; 00645 00646 Msg.Cmd.Args.groupIdBitmask = (uint8_t)groupIdBitmask; 00647 Msg.Cmd.Args.cipher = (uint8_t)cipher; 00648 Msg.Cmd.Args.publicKeyLen = (uint8_t)publicKeyLen; 00649 Msg.Cmd.Args.group1KeyLen = (uint8_t)group1KeyLen; 00650 Msg.Cmd.Args.group2KeyLen = (uint8_t)group2KeyLen; 00651 00652 /* copy keys (if exist) after command (one after another) */ 00653 memcpy(SMART_CONFIG_START_PUBLIC_KEY_STRING(&Msg), pPublicKey, publicKeyLen); 00654 memcpy(SMART_CONFIG_START_GROUP1_KEY_STRING(&Msg), pGroup1Key, group1KeyLen); 00655 memcpy(SMART_CONFIG_START_GROUP2_KEY_STRING(&Msg), pGroup2Key, group2KeyLen); 00656 00657 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlSmartConfigStartCmdCtrl , &Msg, NULL)); 00658 00659 return (int16_t)Msg.Rsp.status; 00660 00661 00662 } 00663 #endif 00664 00665 /*******************************************************************************/ 00666 /* sl_WlanSetMode */ 00667 /*******************************************************************************/ 00668 typedef union { 00669 _WlanSetMode_t Cmd; 00670 _BasicResponse_t Rsp; 00671 } _SlwlanSetModeMsg_u; 00672 00673 const _SlCmdCtrl_t _SlWlanSetModeCmdCtrl = { 00674 SL_OPCODE_WLAN_SET_MODE, 00675 sizeof(_WlanSetMode_t), 00676 sizeof(_BasicResponse_t) 00677 }; 00678 00679 /* possible values are: 00680 WLAN_SET_STA_MODE = 1 00681 WLAN_SET_AP_MODE = 2 00682 WLAN_SET_P2P_MODE = 3 */ 00683 00684 #if _SL_INCLUDE_FUNC(sl_WlanSetMode) 00685 int16_t cc3100_wlan::sl_WlanSetMode(const uint8_t mode) 00686 { 00687 _SlwlanSetModeMsg_u Msg; 00688 00689 Msg.Cmd.mode = mode; 00690 00691 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlWlanSetModeCmdCtrl , &Msg, NULL)); 00692 00693 return (int16_t)Msg.Rsp.status; 00694 00695 } 00696 #endif 00697 00698 /*******************************************************************************/ 00699 /* sl_WlanSet */ 00700 /* ******************************************************************************/ 00701 typedef union { 00702 _WlanCfgSetGet_t Cmd; 00703 _BasicResponse_t Rsp; 00704 } _SlWlanCfgSetMsg_u; 00705 00706 #if _SL_INCLUDE_FUNC(sl_WlanSet) 00707 const _SlCmdCtrl_t _SlWlanCfgSetCmdCtrl = { 00708 SL_OPCODE_WLAN_CFG_SET, 00709 sizeof(_WlanCfgSetGet_t), 00710 sizeof(_BasicResponse_t) 00711 }; 00712 00713 int16_t cc3100_wlan::sl_WlanSet(const uint16_t ConfigId, const uint16_t ConfigOpt, const uint16_t ConfigLen, const uint8_t *pValues) 00714 { 00715 _SlWlanCfgSetMsg_u Msg; 00716 _SlCmdExt_t CmdExt; 00717 00718 _driver._SlDrvResetCmdExt(&CmdExt); 00719 CmdExt.TxPayloadLen = (ConfigLen+3) & (~3); 00720 CmdExt.pTxPayload = (uint8_t *)pValues; 00721 00722 00723 Msg.Cmd.ConfigId = ConfigId; 00724 Msg.Cmd.ConfigLen = ConfigLen; 00725 Msg.Cmd.ConfigOpt = ConfigOpt; 00726 00727 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlWlanCfgSetCmdCtrl, &Msg, &CmdExt)); 00728 00729 return (int16_t)Msg.Rsp.status; 00730 } 00731 #endif 00732 00733 00734 /******************************************************************************/ 00735 /* sl_WlanGet */ 00736 /******************************************************************************/ 00737 typedef union { 00738 _WlanCfgSetGet_t Cmd; 00739 _WlanCfgSetGet_t Rsp; 00740 } _SlWlanCfgMsgGet_u; 00741 00742 #if _SL_INCLUDE_FUNC(sl_WlanGet) 00743 const _SlCmdCtrl_t _SlWlanCfgGetCmdCtrl = { 00744 SL_OPCODE_WLAN_CFG_GET, 00745 sizeof(_WlanCfgSetGet_t), 00746 sizeof(_WlanCfgSetGet_t) 00747 }; 00748 00749 int16_t cc3100_wlan::sl_WlanGet(const uint16_t ConfigId, uint16_t *pConfigOpt,uint16_t *pConfigLen, uint8_t *pValues) 00750 { 00751 _SlWlanCfgMsgGet_u Msg; 00752 _SlCmdExt_t CmdExt; 00753 00754 if (*pConfigLen == 0) { 00755 return SL_EZEROLEN; 00756 } 00757 00758 _driver._SlDrvResetCmdExt(&CmdExt); 00759 CmdExt.RxPayloadLen = *pConfigLen; 00760 CmdExt.pRxPayload = (uint8_t *)pValues; 00761 00762 Msg.Cmd.ConfigId = ConfigId; 00763 if( pConfigOpt ) { 00764 Msg.Cmd.ConfigOpt = (uint16_t)*pConfigOpt; 00765 } 00766 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlWlanCfgGetCmdCtrl, &Msg, &CmdExt)); 00767 00768 if( pConfigOpt ) { 00769 *pConfigOpt = (uint8_t)Msg.Rsp.ConfigOpt; 00770 } 00771 if (CmdExt.RxPayloadLen < CmdExt.ActualRxPayloadLen) { 00772 *pConfigLen = (uint8_t)CmdExt.RxPayloadLen; 00773 return SL_ESMALLBUF; 00774 } else { 00775 *pConfigLen = (uint8_t)CmdExt.ActualRxPayloadLen; 00776 } 00777 00778 00779 return (int16_t)Msg.Rsp.Status; 00780 } 00781 #endif 00782 00783 cc3100_wlan_rx_filters::cc3100_wlan_rx_filters(cc3100_driver &driver) 00784 : _driver(driver) 00785 { 00786 00787 } 00788 00789 cc3100_wlan_rx_filters::~cc3100_wlan_rx_filters() 00790 { 00791 00792 } 00793 00794 /******************************************************************************/ 00795 /* RX filters message command response structures */ 00796 /******************************************************************************/ 00797 00798 /* Set command */ 00799 typedef union { 00800 _WlanRxFilterAddCommand_t Cmd; 00801 _WlanRxFilterAddCommandReponse_t Rsp; 00802 } _SlrxFilterAddMsg_u; 00803 00804 /* Set command */ 00805 typedef union _SlRxFilterSetMsg_u { 00806 _WlanRxFilterSetCommand_t Cmd; 00807 _WlanRxFilterSetCommandReponse_t Rsp; 00808 } _SlRxFilterSetMsg_u; 00809 00810 /* Get command */ 00811 typedef union _SlRxFilterGetMsg_u { 00812 _WlanRxFilterGetCommand_t Cmd; 00813 _WlanRxFilterGetCommandReponse_t Rsp; 00814 } _SlRxFilterGetMsg_u; 00815 00816 00817 #if _SL_INCLUDE_FUNC(sl_WlanRxFilterAdd) 00818 00819 const _SlCmdCtrl_t _SlRxFilterAddtCmdCtrl = 00820 { 00821 SL_OPCODE_WLAN_WLANRXFILTERADDCOMMAND, 00822 sizeof(_WlanRxFilterAddCommand_t), 00823 sizeof(_WlanRxFilterAddCommandReponse_t) 00824 }; 00825 00826 00827 /*******************************************************************************/ 00828 /* RX filters */ 00829 /*******************************************************************************/ 00830 SlrxFilterID_t cc3100_wlan_rx_filters::sl_WlanRxFilterAdd( SlrxFilterRuleType_t RuleType, 00831 SlrxFilterFlags_t FilterFlags, 00832 const SlrxFilterRule_t* const Rule, 00833 const SlrxFilterTrigger_t* const Trigger, 00834 const SlrxFilterAction_t* const Action, 00835 SlrxFilterID_t* pFilterId) 00836 { 00837 00838 00839 _SlrxFilterAddMsg_u Msg; 00840 Msg.Cmd.RuleType = RuleType; 00841 /* filterId is zero */ 00842 Msg.Cmd.FilterId = 0; 00843 Msg.Cmd.FilterFlags = FilterFlags; 00844 memcpy( &(Msg.Cmd.Rule), Rule, sizeof(SlrxFilterRule_t) ); 00845 memcpy( &(Msg.Cmd.Trigger), Trigger, sizeof(SlrxFilterTrigger_t) ); 00846 memcpy( &(Msg.Cmd.Action), Action, sizeof(SlrxFilterAction_t) ); 00847 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlRxFilterAddtCmdCtrl, &Msg, NULL) ); 00848 *pFilterId = Msg.Rsp.FilterId; 00849 return (int16_t)Msg.Rsp.Status; 00850 00851 } 00852 #endif 00853 00854 00855 00856 /*******************************************************************************/ 00857 /* RX filters */ 00858 /*******************************************************************************/ 00859 #if _SL_INCLUDE_FUNC(sl_WlanRxFilterSet) 00860 const _SlCmdCtrl_t _SlRxFilterSetCmdCtrl = 00861 { 00862 SL_OPCODE_WLAN_WLANRXFILTERSETCOMMAND, 00863 sizeof(_WlanRxFilterSetCommand_t), 00864 sizeof(_WlanRxFilterSetCommandReponse_t) 00865 }; 00866 00867 int16_t cc3100_wlan_rx_filters::sl_WlanRxFilterSet(const SLrxFilterOperation_t RxFilterOperation, 00868 const uint8_t* const pInputBuffer, 00869 uint16_t InputbufferLength) 00870 { 00871 _SlRxFilterSetMsg_u Msg; 00872 _SlCmdExt_t CmdExt; 00873 00874 _driver._SlDrvResetCmdExt(&CmdExt); 00875 CmdExt.TxPayloadLen = InputbufferLength; 00876 CmdExt.pTxPayload = (uint8_t *)pInputBuffer; 00877 00878 Msg.Cmd.RxFilterOperation = RxFilterOperation; 00879 Msg.Cmd.InputBufferLength = InputbufferLength; 00880 00881 00882 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlRxFilterSetCmdCtrl, &Msg, &CmdExt) ); 00883 00884 00885 return (int16_t)Msg.Rsp.Status; 00886 } 00887 #endif 00888 00889 /******************************************************************************/ 00890 /* RX filters */ 00891 /******************************************************************************/ 00892 #if _SL_INCLUDE_FUNC(sl_WlanRxFilterGet) 00893 const _SlCmdCtrl_t _SlRxFilterGetCmdCtrl = 00894 { 00895 SL_OPCODE_WLAN_WLANRXFILTERGETCOMMAND, 00896 sizeof(_WlanRxFilterGetCommand_t), 00897 sizeof(_WlanRxFilterGetCommandReponse_t) 00898 }; 00899 00900 int16_t cc3100_wlan_rx_filters::sl_WlanRxFilterGet(const SLrxFilterOperation_t RxFilterOperation, 00901 uint8_t* pOutputBuffer, 00902 uint16_t OutputbufferLength) 00903 { 00904 _SlRxFilterGetMsg_u Msg; 00905 _SlCmdExt_t CmdExt; 00906 00907 if (OutputbufferLength == 0) { 00908 return SL_EZEROLEN; 00909 } 00910 00911 _driver._SlDrvResetCmdExt(&CmdExt); 00912 CmdExt.RxPayloadLen = OutputbufferLength; 00913 CmdExt.pRxPayload = (uint8_t *)pOutputBuffer; 00914 00915 Msg.Cmd.RxFilterOperation = RxFilterOperation; 00916 Msg.Cmd.OutputBufferLength = OutputbufferLength; 00917 00918 00919 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlRxFilterGetCmdCtrl, &Msg, &CmdExt) ); 00920 00921 if (CmdExt.RxPayloadLen < CmdExt.ActualRxPayloadLen) { 00922 return SL_ESMALLBUF; 00923 } 00924 00925 return (int16_t)Msg.Rsp.Status; 00926 } 00927 #endif 00928 00929 }//namespace mbed_cc3100 00930 00931
Generated on Tue Jul 12 2022 18:55:10 by
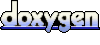