
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
cc3100_netapp.cpp
00001 /* 00002 * netapp.c - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 00039 /*****************************************************************************/ 00040 /* Include files */ 00041 /*****************************************************************************/ 00042 #include "cc3100_simplelink.h" 00043 #include "cc3100_protocol.h" 00044 #include "cc3100_driver.h" 00045 00046 #include "cc3100_netapp.h" 00047 #include "fPtr_func.h" 00048 #include "cli_uart.h" 00049 00050 namespace mbed_cc3100 { 00051 00052 /*****************************************************************************/ 00053 /* Macro declarations */ 00054 /*****************************************************************************/ 00055 const uint32_t NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT = ((uint32_t)0x1 << 31); 00056 00057 #ifdef SL_TINY 00058 const uint8_t NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH = 63; 00059 #else 00060 const uint8_t NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH = 255; 00061 #endif 00062 00063 cc3100_netapp::cc3100_netapp(cc3100_driver &driver, cc3100_nonos &nonos) 00064 : _driver(driver), _nonos(nonos) 00065 { 00066 00067 } 00068 00069 cc3100_netapp::~cc3100_netapp() 00070 { 00071 00072 } 00073 00074 00075 /*****************************************************************************/ 00076 /* API functions */ 00077 /*****************************************************************************/ 00078 00079 /***************************************************************************** 00080 sl_NetAppStart 00081 *****************************************************************************/ 00082 typedef union { 00083 _NetAppStartStopCommand_t Cmd; 00084 _NetAppStartStopResponse_t Rsp; 00085 } _SlNetAppStartStopMsg_u; 00086 00087 #if _SL_INCLUDE_FUNC(sl_NetAppStart) 00088 const _SlCmdCtrl_t _SlNetAppStartCtrl = { 00089 SL_OPCODE_NETAPP_START_COMMAND, 00090 sizeof(_NetAppStartStopCommand_t), 00091 sizeof(_NetAppStartStopResponse_t) 00092 }; 00093 00094 int16_t cc3100_netapp::sl_NetAppStart(const uint32_t AppBitMap) 00095 { 00096 _SlNetAppStartStopMsg_u Msg; 00097 Msg.Cmd.appId = AppBitMap; 00098 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppStartCtrl, &Msg, NULL)); 00099 00100 return Msg.Rsp.status; 00101 } 00102 #endif 00103 00104 /***************************************************************************** 00105 sl_NetAppStop 00106 *****************************************************************************/ 00107 #if _SL_INCLUDE_FUNC(sl_NetAppStop) 00108 const _SlCmdCtrl_t _SlNetAppStopCtrl = 00109 { 00110 SL_OPCODE_NETAPP_STOP_COMMAND, 00111 sizeof(_NetAppStartStopCommand_t), 00112 sizeof(_NetAppStartStopResponse_t) 00113 }; 00114 int16_t cc3100_netapp::sl_NetAppStop(const uint32_t AppBitMap) 00115 { 00116 _SlNetAppStartStopMsg_u Msg; 00117 Msg.Cmd.appId = AppBitMap; 00118 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppStopCtrl, &Msg, NULL)); 00119 00120 return Msg.Rsp.status; 00121 } 00122 #endif 00123 00124 00125 /******************************************************************************/ 00126 /* sl_NetAppGetServiceList */ 00127 /******************************************************************************/ 00128 typedef struct { 00129 uint8_t IndexOffest; 00130 uint8_t MaxServiceCount; 00131 uint8_t Flags; 00132 int8_t Padding; 00133 } NetappGetServiceListCMD_t; 00134 00135 typedef union { 00136 NetappGetServiceListCMD_t Cmd; 00137 _BasicResponse_t Rsp; 00138 } _SlNetappGetServiceListMsg_u; 00139 00140 #if _SL_INCLUDE_FUNC(sl_NetAppGetServiceList) 00141 const _SlCmdCtrl_t _SlGetServiceListeCtrl = { 00142 SL_OPCODE_NETAPP_NETAPP_MDNS_LOOKUP_SERVICE, 00143 sizeof(NetappGetServiceListCMD_t), 00144 sizeof(_BasicResponse_t) 00145 }; 00146 00147 int16_t cc3100_netapp::sl_NetAppGetServiceList(const uint8_t IndexOffest, 00148 const uint8_t MaxServiceCount, 00149 const uint8_t Flags, 00150 int8_t *pBuffer, 00151 const uint32_t RxBufferLength 00152 ) 00153 { 00154 int32_t retVal= 0; 00155 _SlNetappGetServiceListMsg_u Msg; 00156 _SlCmdExt_t CmdExt; 00157 uint16_t ServiceSize = 0; 00158 uint16_t BufferSize = 0; 00159 00160 /* 00161 Calculate RX pBuffer size 00162 WARNING: 00163 if this size is BufferSize than 1480 error should be returned because there 00164 is no place in the RX packet. 00165 */ 00166 switch(Flags) { 00167 case SL_NET_APP_FULL_SERVICE_WITH_TEXT_IPV4_TYPE: 00168 ServiceSize = sizeof(SlNetAppGetFullServiceWithTextIpv4List_t); 00169 break; 00170 00171 case SL_NET_APP_FULL_SERVICE_IPV4_TYPE: 00172 ServiceSize = sizeof(SlNetAppGetFullServiceIpv4List_t); 00173 break; 00174 00175 case SL_NET_APP_SHORT_SERVICE_IPV4_TYPE: 00176 ServiceSize = sizeof(SlNetAppGetShortServiceIpv4List_t); 00177 break; 00178 00179 default: 00180 ServiceSize = sizeof(_BasicResponse_t); 00181 break; 00182 } 00183 00184 00185 00186 BufferSize = MaxServiceCount * ServiceSize; 00187 00188 /*Check the size of the requested services is smaller than size of the user buffer. 00189 If not an error is returned in order to avoid overwriting memory. */ 00190 if(RxBufferLength <= BufferSize) { 00191 return SL_ERROR_NETAPP_RX_BUFFER_LENGTH_ERROR; 00192 } 00193 00194 _driver._SlDrvResetCmdExt(&CmdExt); 00195 CmdExt.RxPayloadLen = BufferSize; 00196 00197 CmdExt.pRxPayload = (uint8_t *)pBuffer; 00198 00199 Msg.Cmd.IndexOffest = IndexOffest; 00200 Msg.Cmd.MaxServiceCount = MaxServiceCount; 00201 Msg.Cmd.Flags = Flags; 00202 Msg.Cmd.Padding = 0; 00203 00204 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetServiceListeCtrl, &Msg, &CmdExt)); 00205 retVal = Msg.Rsp.status; 00206 00207 return (int16_t)retVal; 00208 } 00209 00210 #endif 00211 00212 /*****************************************************************************/ 00213 /* sl_mDNSRegisterService */ 00214 /*****************************************************************************/ 00215 /* 00216 * The below struct depicts the constant parameters of the command/API RegisterService. 00217 * 00218 1. ServiceLen - The length of the service should be smaller than NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00219 2. TextLen - The length of the text should be smaller than NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00220 3. port - The port on this target host. 00221 4. TTL - The TTL of the service 00222 5. Options - bitwise parameters: 00223 bit 0 - is unique (means if the service needs to be unique) 00224 bit 31 - for internal use if the service should be added or deleted (set means ADD). 00225 bit 1-30 for future. 00226 00227 NOTE: 00228 00229 1. There are another variable parameter is this API which is the service name and the text. 00230 2. According to now there is no warning and Async event to user on if the service is a unique. 00231 * 00232 */ 00233 00234 typedef struct { 00235 uint8_t ServiceNameLen; 00236 uint8_t TextLen; 00237 uint16_t Port; 00238 uint32_t TTL; 00239 uint32_t Options; 00240 } NetappMdnsSetService_t; 00241 00242 typedef union { 00243 NetappMdnsSetService_t Cmd; 00244 _BasicResponse_t Rsp; 00245 } _SlNetappMdnsRegisterServiceMsg_u; 00246 00247 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSRegisterUnregisterService) 00248 const _SlCmdCtrl_t _SlRegisterServiceCtrl = { 00249 SL_OPCODE_NETAPP_MDNSREGISTERSERVICE, 00250 sizeof(NetappMdnsSetService_t), 00251 sizeof(_BasicResponse_t) 00252 }; 00253 00254 /****************************************************************************** 00255 00256 sl_NetAppMDNSRegisterService 00257 00258 CALLER user from its host 00259 00260 00261 DESCRIPTION: 00262 Add/delete service 00263 The function manipulates the command that register the service and call 00264 to the NWP in order to add/delete the service to/from the mDNS package and to/from the DB. 00265 00266 This register service is a service offered by the application. 00267 This unregister service is a service offered by the application before. 00268 00269 The service name should be full service name according to RFC 00270 of the DNS-SD - means the value in name field in SRV answer. 00271 00272 Example for service name: 00273 1. PC1._ipp._tcp.local 00274 2. PC2_server._ftp._tcp.local 00275 00276 If the option is_unique is set, mDNS probes the service name to make sure 00277 it is unique before starting to announce the service on the network. 00278 Instance is the instance portion of the service name. 00279 00280 00281 00282 00283 PARAMETERS: 00284 00285 The command is from constant parameters and variables parameters. 00286 00287 Constant parameters are: 00288 00289 ServiceLen - The length of the service. 00290 TextLen - The length of the service should be smaller than 64. 00291 port - The port on this target host. 00292 TTL - The TTL of the service 00293 Options - bitwise parameters: 00294 bit 0 - is unique (means if the service needs to be unique) 00295 bit 31 - for internal use if the service should be added or deleted (set means ADD). 00296 bit 1-30 for future. 00297 00298 The variables parameters are: 00299 00300 Service name(full service name) - The service name. 00301 Example for service name: 00302 1. PC1._ipp._tcp.local 00303 2. PC2_server._ftp._tcp.local 00304 00305 Text - The description of the service. 00306 should be as mentioned in the RFC 00307 (according to type of the service IPP,FTP...) 00308 00309 NOTE - pay attention 00310 00311 1. Temporary - there is an allocation on stack of internal buffer. 00312 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00313 It means that the sum of the text length and service name length cannot be bigger than 00314 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00315 If it is - An error is returned. 00316 00317 2. According to now from certain constraints the variables parameters are set in the 00318 attribute part (contain constant parameters) 00319 00320 00321 00322 RETURNS: Status - the immediate response of the command status. 00323 0 means success. 00324 00325 00326 00327 ******************************************************************************/ 00328 int16_t cc3100_netapp::sl_NetAppMDNSRegisterUnregisterService(const char* pServiceName, 00329 const uint8_t ServiceNameLen, 00330 const char* pText, 00331 const uint8_t TextLen, 00332 const uint16_t Port, 00333 const uint32_t TTL, 00334 const uint32_t Options) 00335 { 00336 00337 _SlNetappMdnsRegisterServiceMsg_u Msg; 00338 _SlCmdExt_t CmdExt ; 00339 unsigned char ServiceNameAndTextBuffer[NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH]; 00340 unsigned char *TextPtr; 00341 00342 /* 00343 00344 NOTE - pay attention 00345 00346 1. Temporary - there is an allocation on stack of internal buffer. 00347 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00348 It means that the sum of the text length and service name length cannot be bigger than 00349 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00350 If it is - An error is returned. 00351 00352 2. According to now from certain constraints the variables parameters are set in the 00353 attribute part (contain constant parameters) 00354 00355 00356 */ 00357 00358 /*build the attribute part of the command. 00359 It contains the constant parameters of the command*/ 00360 00361 Msg.Cmd.ServiceNameLen = ServiceNameLen; 00362 Msg.Cmd.Options = Options; 00363 Msg.Cmd.Port = Port; 00364 Msg.Cmd.TextLen = TextLen; 00365 Msg.Cmd.TTL = TTL; 00366 00367 /*Build the payload part of the command 00368 Copy the service name and text to one buffer. 00369 NOTE - pay attention 00370 The size of the service length + the text length should be smaller than 255, 00371 Until the simplelink drive supports to variable length through SPI command. */ 00372 if(TextLen + ServiceNameLen > (NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH - 1 )) { /*-1 is for giving a place to set null termination at the end of the text*/ 00373 return -1; 00374 } 00375 00376 _driver._SlDrvMemZero(ServiceNameAndTextBuffer, NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH); 00377 00378 00379 /*Copy the service name*/ 00380 memcpy(ServiceNameAndTextBuffer, 00381 pServiceName, 00382 ServiceNameLen); 00383 00384 if(TextLen > 0 ) { 00385 00386 TextPtr = &ServiceNameAndTextBuffer[ServiceNameLen]; 00387 /*Copy the text just after the service name*/ 00388 memcpy(TextPtr, pText, TextLen); 00389 } 00390 00391 _driver._SlDrvResetCmdExt(&CmdExt); 00392 CmdExt.TxPayloadLen = (TextLen + ServiceNameLen); 00393 CmdExt.pTxPayload = (uint8_t *)ServiceNameAndTextBuffer; 00394 00395 00396 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlRegisterServiceCtrl, &Msg, &CmdExt)); 00397 00398 return (int16_t)Msg.Rsp.status; 00399 00400 00401 } 00402 #endif 00403 00404 /**********************************************************************************************/ 00405 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSRegisterService) 00406 int16_t cc3100_netapp::sl_NetAppMDNSRegisterService(const char* pServiceName, 00407 const uint8_t ServiceNameLen, 00408 const char* pText, 00409 const uint8_t TextLen, 00410 const uint16_t Port, 00411 const uint32_t TTL, 00412 uint32_t Options) 00413 { 00414 00415 /* 00416 00417 NOTE - pay attention 00418 00419 1. Temporary - there is an allocation on stack of internal buffer. 00420 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00421 It means that the sum of the text length and service name length cannot be bigger than 00422 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00423 If it is - An error is returned. 00424 00425 2. According to now from certain constraints the variables parameters are set in the 00426 attribute part (contain constant parameters) 00427 00428 */ 00429 00430 /*Set the add service bit in the options parameter. 00431 In order not use different opcodes for the register service and unregister service 00432 bit 31 in option is taken for this purpose. if it is set it means in NWP that the service should be added 00433 if it is cleared it means that the service should be deleted and there is only meaning to pServiceName 00434 and ServiceNameLen values. */ 00435 Options |= NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT; 00436 00437 return (sl_NetAppMDNSRegisterUnregisterService( pServiceName, ServiceNameLen, pText, TextLen, Port, TTL, Options)); 00438 00439 00440 } 00441 #endif 00442 /**********************************************************************************************/ 00443 00444 00445 00446 /**********************************************************************************************/ 00447 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSUnRegisterService) 00448 int16_t cc3100_netapp::sl_NetAppMDNSUnRegisterService(const char* pServiceName, const uint8_t ServiceNameLen) 00449 { 00450 uint32_t Options = 0; 00451 00452 /* 00453 00454 NOTE - pay attention 00455 00456 The size of the service length should be smaller than 255, 00457 Until the simplelink drive supports to variable length through SPI command. 00458 00459 00460 */ 00461 00462 /*Clear the add service bit in the options parameter. 00463 In order not use different opcodes for the register service and unregister service 00464 bit 31 in option is taken for this purpose. if it is set it means in NWP that the service should be added 00465 if it is cleared it means that the service should be deleted and there is only meaning to pServiceName 00466 and ServiceNameLen values.*/ 00467 00468 Options &= (~NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT); 00469 00470 return (sl_NetAppMDNSRegisterUnregisterService(pServiceName, ServiceNameLen, NULL, 0, 0, 0, Options)); 00471 00472 00473 } 00474 #endif 00475 /**********************************************************************************************/ 00476 00477 00478 00479 /*****************************************************************************/ 00480 /* sl_DnsGetHostByService */ 00481 /*****************************************************************************/ 00482 /* 00483 * The below struct depicts the constant parameters of the command/API sl_DnsGetHostByService. 00484 * 00485 1. ServiceLen - The length of the service should be smaller than 255. 00486 2. AddrLen - TIPv4 or IPv6 (SL_AF_INET , SL_AF_INET6). 00487 * 00488 */ 00489 00490 typedef struct { 00491 uint8_t ServiceLen; 00492 uint8_t AddrLen; 00493 uint16_t Padding; 00494 } _GetHostByServiceCommand_t; 00495 00496 00497 00498 /* 00499 * The below structure depict the constant parameters that are returned in the Async event answer 00500 * according to command/API sl_DnsGetHostByService for IPv4 and IPv6. 00501 * 00502 1Status - The status of the response. 00503 2.Address - Contains the IP address of the service. 00504 3.Port - Contains the port of the service. 00505 4.TextLen - Contains the max length of the text that the user wants to get. 00506 it means that if the test of service is bigger that its value than 00507 the text is cut to inout_TextLen value. 00508 Output: Contain the length of the text that is returned. Can be full text or part 00509 of the text (see above). 00510 00511 * 00512 00513 typedef struct { 00514 uint16_t Status; 00515 uint16_t TextLen; 00516 uint32_t Port; 00517 uint32_t Address; 00518 } _GetHostByServiceIPv4AsyncResponse_t; 00519 */ 00520 00521 typedef struct { 00522 uint16_t Status; 00523 uint16_t TextLen; 00524 uint32_t Port; 00525 uint32_t Address[4]; 00526 } _GetHostByServiceIPv6AsyncResponse_t; 00527 00528 00529 typedef union { 00530 _GetHostByServiceIPv4AsyncResponse_t IpV4; 00531 _GetHostByServiceIPv6AsyncResponse_t IpV6; 00532 } _GetHostByServiceAsyncResponseAttribute_u; 00533 00534 typedef union { 00535 _GetHostByServiceCommand_t Cmd; 00536 _BasicResponse_t Rsp; 00537 } _SlGetHostByServiceMsg_u; 00538 00539 #if _SL_INCLUDE_FUNC(sl_NetAppDnsGetHostByService) 00540 const _SlCmdCtrl_t _SlGetHostByServiceCtrl = { 00541 SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICE, 00542 sizeof(_GetHostByServiceCommand_t), 00543 sizeof(_BasicResponse_t) 00544 }; 00545 00546 int32_t cc3100_netapp::sl_NetAppDnsGetHostByService(unsigned char *pServiceName, /* string containing all (or only part): name + subtype + service */ 00547 const uint8_t ServiceLen, 00548 const uint8_t Family, /* 4-IPv4 , 16-IPv6 */ 00549 uint32_t pAddr[], 00550 uint32_t *pPort, 00551 uint16_t *pTextLen, /* in: max len , out: actual len */ 00552 unsigned char *pText) 00553 { 00554 00555 _SlGetHostByServiceMsg_u Msg; 00556 _SlCmdExt_t CmdExt ; 00557 _GetHostByServiceAsyncResponse_t AsyncRsp; 00558 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00559 00560 /* 00561 Note: 00562 1. The return's attributes are belonged to first service that is found. 00563 It can be other services with the same service name will response to 00564 the query. The results of these responses are saved in the peer cache of the NWP, and 00565 should be read by another API. 00566 00567 2. Text length can be 120 bytes only - not more 00568 It is because of constraints in the NWP on the buffer that is allocated for the Async event. 00569 00570 3.The API waits to Async event by blocking. It means that the API is finished only after an Async event 00571 is sent by the NWP. 00572 00573 4.No rolling option!!! - only PTR type is sent. 00574 00575 00576 */ 00577 /*build the attribute part of the command. 00578 It contains the constant parameters of the command */ 00579 00580 Msg.Cmd.ServiceLen = ServiceLen; 00581 Msg.Cmd.AddrLen = Family; 00582 00583 /*Build the payload part of the command 00584 Copy the service name and text to one buffer.*/ 00585 _driver._SlDrvResetCmdExt(&CmdExt); 00586 CmdExt.TxPayloadLen = ServiceLen; 00587 00588 CmdExt.pTxPayload = (uint8_t *)pServiceName; 00589 00590 00591 /*set pointers to the output parameters (the returned parameters). 00592 This pointers are belonged to local struct that is set to global Async response parameter. 00593 It is done in order not to run more than one sl_DnsGetHostByService at the same time. 00594 The API should be run only if global parameter is pointed to NULL. */ 00595 AsyncRsp.out_pText = pText; 00596 AsyncRsp.inout_TextLen = (uint16_t* )pTextLen; 00597 AsyncRsp.out_pPort = pPort; 00598 AsyncRsp.out_pAddr = (uint32_t *)pAddr; 00599 00600 00601 ObjIdx = _driver._SlDrvProtectAsyncRespSetting((uint8_t*)&AsyncRsp, GETHOSYBYSERVICE_ID, SL_MAX_SOCKETS); 00602 00603 if (MAX_CONCURRENT_ACTIONS == ObjIdx) 00604 { 00605 return SL_POOL_IS_EMPTY; 00606 } 00607 00608 if (SL_AF_INET6 == Family) { 00609 g_pCB->ObjPool[ObjIdx].AdditionalData |= SL_NETAPP_FAMILY_MASK; 00610 } 00611 /* Send the command */ 00612 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetHostByServiceCtrl, &Msg, &CmdExt)); 00613 00614 00615 00616 /* If the immediate reponse is O.K. than wait for aSYNC event response. */ 00617 if(SL_RET_CODE_OK == Msg.Rsp.status) { 00618 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00619 00620 /* If we are - it means that Async event was sent. 00621 The results are copied in the Async handle return functions */ 00622 00623 Msg.Rsp.status = AsyncRsp.Status; 00624 } 00625 00626 _driver._SlDrvReleasePoolObj(ObjIdx); 00627 return Msg.Rsp.status; 00628 } 00629 #endif 00630 00631 /*****************************************************************************/ 00632 /* _sl_HandleAsync_DnsGetHostByAddr */ 00633 /*****************************************************************************/ 00634 #ifndef SL_TINY_EXT 00635 void cc3100_netapp::_sl_HandleAsync_DnsGetHostByAddr(void *pVoidBuf) 00636 { 00637 SL_TRACE0(DBG_MSG, MSG_303, "STUB: _sl_HandleAsync_DnsGetHostByAddr not implemented yet!"); 00638 return; 00639 } 00640 #endif 00641 /*****************************************************************************/ 00642 /* sl_DnsGetHostByName */ 00643 /*****************************************************************************/ 00644 typedef union { 00645 _GetHostByNameIPv4AsyncResponse_t IpV4; 00646 _GetHostByNameIPv6AsyncResponse_t IpV6; 00647 } _GetHostByNameAsyncResponse_u; 00648 00649 typedef union { 00650 _GetHostByNameCommand_t Cmd; 00651 _BasicResponse_t Rsp; 00652 } _SlGetHostByNameMsg_u; 00653 00654 #if _SL_INCLUDE_FUNC(sl_NetAppDnsGetHostByName) 00655 const _SlCmdCtrl_t _SlGetHostByNameCtrl = { 00656 SL_OPCODE_NETAPP_DNSGETHOSTBYNAME, 00657 sizeof(_GetHostByNameCommand_t), 00658 sizeof(_BasicResponse_t) 00659 }; 00660 00661 int16_t cc3100_netapp::sl_NetAppDnsGetHostByName(unsigned char * hostname, const uint16_t usNameLen, uint32_t* out_ip_addr, const uint8_t family) 00662 { 00663 _SlGetHostByNameMsg_u Msg; 00664 _SlCmdExt_t ExtCtrl; 00665 _GetHostByNameAsyncResponse_u AsyncRsp; 00666 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00667 00668 _driver._SlDrvResetCmdExt(&ExtCtrl); 00669 ExtCtrl.TxPayloadLen = usNameLen; 00670 00671 ExtCtrl.pTxPayload = (unsigned char *)hostname; 00672 00673 00674 Msg.Cmd.Len = usNameLen; 00675 Msg.Cmd.family = family; 00676 00677 /*Use Obj to issue the command, if not available try later */ 00678 ObjIdx = (uint8_t)_driver._SlDrvWaitForPoolObj(GETHOSYBYNAME_ID,SL_MAX_SOCKETS); 00679 if (MAX_CONCURRENT_ACTIONS == ObjIdx) { 00680 Uart_Write((uint8_t*)"SL_POOL_IS_EMPTY \r\n"); 00681 return SL_POOL_IS_EMPTY; 00682 } 00683 00684 _driver._SlDrvProtectionObjLockWaitForever(); 00685 00686 g_pCB->ObjPool[ObjIdx].pRespArgs = (uint8_t *)&AsyncRsp; 00687 /*set bit to indicate IPv6 address is expected */ 00688 if (SL_AF_INET6 == family) { 00689 g_pCB->ObjPool[ObjIdx].AdditionalData |= SL_NETAPP_FAMILY_MASK; 00690 } 00691 00692 _driver._SlDrvProtectionObjUnLock(); 00693 00694 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetHostByNameCtrl, &Msg, &ExtCtrl)); 00695 00696 if(SL_RET_CODE_OK == Msg.Rsp.status) { 00697 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00698 Msg.Rsp.status = AsyncRsp.IpV4.status; 00699 00700 if(SL_OS_RET_CODE_OK == (int16_t)Msg.Rsp.status) { 00701 memcpy((int8_t *)out_ip_addr, (signed char *)&AsyncRsp.IpV4.ip0, (SL_AF_INET == family) ? SL_IPV4_ADDRESS_SIZE : SL_IPV6_ADDRESS_SIZE); 00702 } 00703 } 00704 _driver._SlDrvReleasePoolObj(ObjIdx); 00705 return Msg.Rsp.status; 00706 } 00707 #endif 00708 00709 #ifndef SL_TINY_EXT 00710 void cc3100_netapp::CopyPingResultsToReport(_PingReportResponse_t *pResults,SlPingReport_t *pReport) 00711 { 00712 pReport->PacketsSent = pResults->numSendsPings; 00713 pReport->PacketsReceived = pResults->numSuccsessPings; 00714 pReport->MinRoundTime = pResults->rttMin; 00715 pReport->MaxRoundTime = pResults->rttMax; 00716 pReport->AvgRoundTime = pResults->rttAvg; 00717 pReport->TestTime = pResults->testTime; 00718 } 00719 #endif 00720 /*****************************************************************************/ 00721 /* sl_PingStart */ 00722 /*****************************************************************************/ 00723 typedef union { 00724 _PingStartCommand_t Cmd; 00725 _PingReportResponse_t Rsp; 00726 } _SlPingStartMsg_u; 00727 00728 00729 typedef enum { 00730 CMD_PING_TEST_RUNNING = 0, 00731 CMD_PING_TEST_STOPPED 00732 } _SlPingStatus_e; 00733 00734 P_SL_DEV_PING_CALLBACK pPingCallBackFunc; 00735 00736 #if _SL_INCLUDE_FUNC(sl_NetAppPingStart) 00737 int16_t cc3100_netapp::sl_NetAppPingStart(const SlPingStartCommand_t* pPingParams, const uint8_t family, SlPingReport_t *pReport, const P_SL_DEV_PING_CALLBACK pPingCallback) 00738 { 00739 00740 _SlCmdCtrl_t CmdCtrl = {0, sizeof(_PingStartCommand_t), sizeof(_BasicResponse_t)}; 00741 _SlPingStartMsg_u Msg; 00742 _PingReportResponse_t PingRsp; 00743 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00744 00745 if( 0 == pPingParams->Ip ) 00746 { /* stop any ongoing ping */ 00747 return _driver._SlDrvBasicCmd(SL_OPCODE_NETAPP_PINGSTOP); 00748 } 00749 00750 if(SL_AF_INET == family) { 00751 CmdCtrl.Opcode = SL_OPCODE_NETAPP_PINGSTART; 00752 memcpy(&Msg.Cmd.ip0, &pPingParams->Ip, SL_IPV4_ADDRESS_SIZE); 00753 } else { 00754 CmdCtrl.Opcode = SL_OPCODE_NETAPP_PINGSTART_V6; 00755 memcpy(&Msg.Cmd.ip0, &pPingParams->Ip, SL_IPV6_ADDRESS_SIZE); 00756 } 00757 00758 Msg.Cmd.pingIntervalTime = pPingParams->PingIntervalTime; 00759 Msg.Cmd.PingSize = pPingParams->PingSize; 00760 Msg.Cmd.pingRequestTimeout = pPingParams->PingRequestTimeout; 00761 Msg.Cmd.totalNumberOfAttempts = pPingParams->TotalNumberOfAttempts; 00762 Msg.Cmd.flags = pPingParams->Flags; 00763 00764 if( pPingCallback ) { 00765 pPingCallBackFunc = pPingCallback; 00766 } else { 00767 /*Use Obj to issue the command, if not available try later */ 00768 ObjIdx = (uint8_t)_driver._SlDrvWaitForPoolObj(PING_ID,SL_MAX_SOCKETS); 00769 if (MAX_CONCURRENT_ACTIONS == ObjIdx) { 00770 return SL_POOL_IS_EMPTY; 00771 } 00772 OSI_RET_OK_CHECK(_nonos.sl_LockObjLock(&g_pCB->ProtectionLockObj, NON_OS_LOCK_OBJ_UNLOCK_VALUE, NON_OS_LOCK_OBJ_LOCK_VALUE, SL_OS_WAIT_FOREVER)); 00773 /* async response handler for non callback mode */ 00774 g_pCB->ObjPool[ObjIdx].pRespArgs = (uint8_t *)&PingRsp; 00775 pPingCallBackFunc = NULL; 00776 OSI_RET_OK_CHECK(_nonos.sl_LockObjUnlock(&g_pCB->ProtectionLockObj, NON_OS_LOCK_OBJ_UNLOCK_VALUE)); 00777 } 00778 00779 00780 VERIFY_RET_OK(_driver._SlDrvCmdOp(&CmdCtrl, &Msg, NULL)); 00781 /*send the command*/ 00782 if(CMD_PING_TEST_RUNNING == (int16_t)Msg.Rsp.status || CMD_PING_TEST_STOPPED == (int16_t)Msg.Rsp.status ) { 00783 /* block waiting for results if no callback function is used */ 00784 if( NULL == pPingCallback ) { 00785 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00786 if( SL_OS_RET_CODE_OK == (int16_t)PingRsp.status ) { 00787 CopyPingResultsToReport(&PingRsp,pReport); 00788 } 00789 _driver._SlDrvReleasePoolObj(ObjIdx); 00790 } 00791 } else { 00792 /* ping failure, no async response */ 00793 if( NULL == pPingCallback ) { 00794 _driver._SlDrvReleasePoolObj(ObjIdx); 00795 } 00796 } 00797 00798 return Msg.Rsp.status; 00799 } 00800 #endif 00801 00802 /*****************************************************************************/ 00803 /* sl_NetAppSet */ 00804 /*****************************************************************************/ 00805 typedef union { 00806 _NetAppSetGet_t Cmd; 00807 _BasicResponse_t Rsp; 00808 } _SlNetAppMsgSet_u; 00809 00810 #if _SL_INCLUDE_FUNC(sl_NetAppSet) 00811 const _SlCmdCtrl_t _SlNetAppSetCmdCtrl = { 00812 SL_OPCODE_NETAPP_NETAPPSET, 00813 sizeof(_NetAppSetGet_t), 00814 sizeof(_BasicResponse_t) 00815 }; 00816 00817 int32_t cc3100_netapp::sl_NetAppSet(const uint8_t AppId ,const uint8_t Option, const uint8_t OptionLen, const uint8_t *pOptionValue) 00818 { 00819 00820 _SlNetAppMsgSet_u Msg; 00821 _SlCmdExt_t CmdExt; 00822 00823 _driver._SlDrvResetCmdExt(&CmdExt); 00824 CmdExt.TxPayloadLen = (OptionLen+3) & (~3); 00825 00826 CmdExt.pTxPayload = (uint8_t *)pOptionValue; 00827 00828 Msg.Cmd.AppId = AppId; 00829 Msg.Cmd.ConfigLen = OptionLen; 00830 Msg.Cmd.ConfigOpt = Option; 00831 00832 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppSetCmdCtrl, &Msg, &CmdExt)); 00833 00834 return (int16_t)Msg.Rsp.status; 00835 } 00836 #endif 00837 00838 /*****************************************************************************/ 00839 /* sl_NetAppSendTokenValue */ 00840 /*****************************************************************************/ 00841 typedef union { 00842 sl_NetAppHttpServerSendToken_t Cmd; 00843 _BasicResponse_t Rsp; 00844 } _SlNetAppMsgSendTokenValue_u; 00845 00846 #if defined(sl_HttpServerCallback) || defined(EXT_LIB_REGISTERED_HTTP_SERVER_EVENTS) 00847 const _SlCmdCtrl_t _SlNetAppSendTokenValueCmdCtrl = { 00848 SL_OPCODE_NETAPP_HTTPSENDTOKENVALUE, 00849 sizeof(sl_NetAppHttpServerSendToken_t), 00850 sizeof(_BasicResponse_t) 00851 }; 00852 00853 uint16_t cc3100_netapp::sl_NetAppSendTokenValue(slHttpServerData_t * Token_value) 00854 { 00855 00856 _SlNetAppMsgSendTokenValue_u Msg; 00857 _SlCmdExt_t CmdExt; 00858 00859 CmdExt.TxPayloadLen = (Token_value->value_len+3) & (~3); 00860 CmdExt.RxPayloadLen = 0; 00861 CmdExt.pTxPayload = (uint8_t *) Token_value->token_value; 00862 CmdExt.pRxPayload = NULL; 00863 00864 Msg.Cmd.token_value_len = Token_value->value_len; 00865 Msg.Cmd.token_name_len = Token_value->name_len; 00866 memcpy(&Msg.Cmd.token_name[0], Token_value->token_name, Token_value->name_len); 00867 00868 00869 VERIFY_RET_OK(_driver._SlDrvCmdSend((_SlCmdCtrl_t *)&_SlNetAppSendTokenValueCmdCtrl, &Msg, &CmdExt)); 00870 00871 return Msg.Rsp.status; 00872 } 00873 #endif 00874 00875 /*****************************************************************************/ 00876 /* sl_NetAppGet */ 00877 /*****************************************************************************/ 00878 typedef union { 00879 _NetAppSetGet_t Cmd; 00880 _NetAppSetGet_t Rsp; 00881 } _SlNetAppMsgGet_u; 00882 00883 #if _SL_INCLUDE_FUNC(sl_NetAppGet) 00884 const _SlCmdCtrl_t _SlNetAppGetCmdCtrl = { 00885 SL_OPCODE_NETAPP_NETAPPGET, 00886 sizeof(_NetAppSetGet_t), 00887 sizeof(_NetAppSetGet_t) 00888 }; 00889 00890 int32_t cc3100_netapp::sl_NetAppGet(const uint8_t AppId, const uint8_t Option, uint8_t *pOptionLen, uint8_t *pOptionValue) 00891 { 00892 _SlNetAppMsgGet_u Msg; 00893 _SlCmdExt_t CmdExt; 00894 00895 if (*pOptionLen == 0) { 00896 return SL_EZEROLEN; 00897 } 00898 00899 _driver._SlDrvResetCmdExt(&CmdExt); 00900 CmdExt.RxPayloadLen = *pOptionLen; 00901 00902 CmdExt.pRxPayload = (uint8_t *)pOptionValue; 00903 00904 Msg.Cmd.AppId = AppId; 00905 Msg.Cmd.ConfigOpt = Option; 00906 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppGetCmdCtrl, &Msg, &CmdExt)); 00907 00908 00909 if (CmdExt.RxPayloadLen < CmdExt.ActualRxPayloadLen) { 00910 *pOptionLen = (uint8_t)CmdExt.RxPayloadLen; 00911 return SL_ESMALLBUF; 00912 } else { 00913 *pOptionLen = (uint8_t)CmdExt.ActualRxPayloadLen; 00914 } 00915 00916 return (int16_t)Msg.Rsp.Status; 00917 } 00918 #endif 00919 00920 cc3100_flowcont::cc3100_flowcont(cc3100_driver &driver, cc3100_nonos &nonos) 00921 : _driver(driver), _nonos(nonos) 00922 { 00923 00924 } 00925 00926 cc3100_flowcont::~cc3100_flowcont() 00927 { 00928 00929 } 00930 #if 0 00931 /*****************************************************************************/ 00932 /* _SlDrvFlowContInit */ 00933 /*****************************************************************************/ 00934 void cc3100_flowcont::_SlDrvFlowContInit(void) 00935 { 00936 g_pCB->FlowContCB.TxPoolCnt = FLOW_CONT_MIN; 00937 00938 OSI_RET_OK_CHECK(_nonos.sl_LockObjCreate(&g_pCB->FlowContCB.TxLockObj, "TxLockObj")); 00939 00940 OSI_RET_OK_CHECK(_nonos.sl_SyncObjCreate(&g_pCB->FlowContCB.TxSyncObj, "TxSyncObj")); 00941 } 00942 00943 /*****************************************************************************/ 00944 /* _SlDrvFlowContDeinit */ 00945 /*****************************************************************************/ 00946 void cc3100_flowcont::_SlDrvFlowContDeinit(void) 00947 { 00948 g_pCB->FlowContCB.TxPoolCnt = 0; 00949 00950 OSI_RET_OK_CHECK(_nonos.sl_LockObjDelete(&g_pCB->FlowContCB.TxLockObj, 0)); 00951 00952 OSI_RET_OK_CHECK(_nonos.sl_SyncObjDelete(&g_pCB->FlowContCB.TxSyncObj, 0)); 00953 } 00954 #endif 00955 }//namespace mbed_cc3100 00956 00957
Generated on Tue Jul 12 2022 18:55:10 by
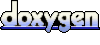