
TI's CC3100. A test demo with very little testing done!
Embed:
(wiki syntax)
Show/hide line numbers
cc3100_spi.h
00001 /* 00002 * spi.h - mbed 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 #ifndef SPI_H_ 00039 #define SPI_H_ 00040 00041 #include "mbed.h" 00042 00043 00044 /*! 00045 \brief type definition for the spi channel file descriptor 00046 00047 \note On each porting or platform the type could be whatever is needed 00048 - integer, pointer to structure etc. 00049 */ 00050 typedef unsigned int Fd_t; 00051 00052 typedef void (*P_EVENT_HANDLER)(void* pValue); 00053 00054 namespace mbed_cc3100 { 00055 00056 class cc3100_driver; 00057 00058 class cc3100_spi 00059 { 00060 public: 00061 00062 cc3100_spi(PinName cc3100_irq, PinName cc3100_nHIB, PinName cc3100_cs, SPI cc3100_spi, cc3100_driver &driver); 00063 00064 ~cc3100_spi(); 00065 00066 00067 /*! 00068 \brief Enables the CC3100 00069 00070 \param[in] none 00071 00072 \return none 00073 00074 \note 00075 00076 \warning 00077 */ 00078 void CC3100_enable(); 00079 00080 /*! 00081 \brief Disables the CC3100 00082 00083 \param[in] none 00084 00085 \return none 00086 00087 \note 00088 00089 \warning 00090 */ 00091 void CC3100_disable(); 00092 00093 /*! 00094 \brief Enables the interrupt from the CC3100 00095 00096 \param[in] none 00097 00098 \return none 00099 00100 \note 00101 00102 \warning 00103 */ 00104 void cc3100_InterruptEnable(); 00105 00106 /*! 00107 \brief Disables the interrupt from the CC3100 00108 00109 \param[in] none 00110 00111 \return none 00112 00113 \note 00114 00115 \warning 00116 */ 00117 void cc3100_InterruptDisable(); 00118 00119 /*! 00120 \brief open spi communication port to be used for communicating with a 00121 SimpleLink device 00122 00123 Given an interface name and option flags, this function opens the spi 00124 communication port and creates a file descriptor. This file descriptor can 00125 be used afterwards to read and write data from and to this specific spi 00126 channel. 00127 The SPI speed, clock polarity, clock phase, chip select and all other 00128 attributes are all set to hardcoded values in this function. 00129 00130 \param[in] ifName - points to the interface name/path. The 00131 interface name is an optional attributes that the simple 00132 link driver receives on opening the device. in systems that 00133 the spi channel is not implemented as part of the os device 00134 drivers, this parameter could be NULL. 00135 \param[in] flags - option flags 00136 00137 \return upon successful completion, the function shall open the spi 00138 channel and return a non-negative integer representing the 00139 file descriptor. Otherwise, -1 shall be returned 00140 00141 \sa spi_Close , spi_Read , spi_Write 00142 \note 00143 \warning 00144 */ 00145 00146 Fd_t spi_Open(int8_t *ifName, uint32_t flags); 00147 00148 /*! 00149 \brief closes an opened spi communication port 00150 00151 \param[in] fd - file descriptor of an opened SPI channel 00152 00153 \return upon successful completion, the function shall return 0. 00154 Otherwise, -1 shall be returned 00155 00156 \sa spi_Open 00157 \note 00158 \warning 00159 */ 00160 int spi_Close(Fd_t fd); 00161 00162 /*! 00163 \brief attempts to read up to len bytes from SPI channel into a buffer 00164 starting at pBuff. 00165 00166 \param[in] fd - file descriptor of an opened SPI channel 00167 00168 \param[in] pBuff - points to first location to start writing the 00169 data 00170 00171 \param[in] len - number of bytes to read from the SPI channel 00172 00173 \return upon successful completion, the function shall return 0. 00174 Otherwise, -1 shall be returned 00175 00176 \sa spi_Open , spi_Write 00177 \note 00178 \warning 00179 */ 00180 int spi_Read(Fd_t fd, uint8_t *pBuff, int len); 00181 00182 /*! 00183 \brief attempts to write up to len bytes to the SPI channel 00184 00185 \param[in] fd - file descriptor of an opened SPI channel 00186 00187 \param[in] pBuff - points to first location to start getting the 00188 data from 00189 00190 \param[in] len - number of bytes to write to the SPI channel 00191 00192 \return upon successful completion, the function shall return 0. 00193 Otherwise, -1 shall be returned 00194 00195 \sa spi_Open , spi_Read 00196 \note This function could be implemented as zero copy and return 00197 only upon successful completion of writing the whole buffer, 00198 but in cases that memory allocation is not too tight, the 00199 function could copy the data to internal buffer, return 00200 back and complete the write in parallel to other activities 00201 as long as the other SPI activities would be blocked untill 00202 the entire buffer write would be completed 00203 \warning 00204 */ 00205 int spi_Write(Fd_t fd, uint8_t *pBuff, int len); 00206 00207 /*! 00208 \brief The IntSpiGPIOHandler interrupt handler 00209 00210 \param[in] none 00211 00212 \return none 00213 00214 \note 00215 00216 \warning 00217 */ 00218 void IntSpiGPIOHandler(void); 00219 00220 /*! 00221 \brief register an interrupt handler for the host IRQ 00222 00223 \param[in] InterruptHdl - pointer to interrupt handler function 00224 00225 \param[in] pValue - pointer to a memory strcuture that is 00226 passed to the interrupt handler. 00227 00228 \return upon successful registration, the function shall return 0. 00229 Otherwise, -1 shall be returned 00230 00231 \sa 00232 \note If there is already registered interrupt handler, the 00233 function should overwrite the old handler with the new one 00234 \warning 00235 */ 00236 int registerInterruptHandler(P_EVENT_HANDLER InterruptHdl , void* pValue); 00237 00238 /*! 00239 \brief Masks the Host IRQ 00240 00241 \param[in] none 00242 00243 \return none 00244 00245 \warning 00246 */ 00247 void MaskIntHdlr(); 00248 00249 /*! 00250 \brief Unmasks the Host IRQ 00251 00252 \param[in] none 00253 00254 \return none 00255 00256 \warning 00257 */ 00258 void UnMaskIntHdlr(); 00259 00260 00261 private: 00262 00263 InterruptIn _wlan_irq; 00264 DigitalOut _wlan_nHIB; 00265 DigitalOut _wlan_cs; 00266 SPI _wlan_spi; 00267 cc3100_driver &_driver; 00268 00269 00270 };//class 00271 }//namespace mbed_cc3100 00272 #endif 00273
Generated on Sun Jul 17 2022 07:25:50 by
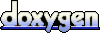