
TI's CC3100. A test demo with very little testing done!
Embed:
(wiki syntax)
Show/hide line numbers
cc3100_netapp.cpp
00001 /* 00002 * netapp.c - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 00039 /*****************************************************************************/ 00040 /* Include files */ 00041 /*****************************************************************************/ 00042 #include "cc3100_simplelink.h" 00043 #include "cc3100_protocol.h" 00044 #include "cc3100_driver.h" 00045 00046 #include "cc3100_netapp.h" 00047 #include "fPtr_func.h" 00048 00049 namespace mbed_cc3100 { 00050 00051 /*****************************************************************************/ 00052 /* Macro declarations */ 00053 /*****************************************************************************/ 00054 const uint32_t NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT = ((uint32_t)0x1 << 31); 00055 00056 #ifdef SL_TINY 00057 const uint8_t NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH = 63; 00058 #else 00059 const uint8_t NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH = 255; 00060 #endif 00061 00062 cc3100_netapp::cc3100_netapp(cc3100_driver &driver, cc3100_nonos &nonos) 00063 : _driver(driver), _nonos(nonos) 00064 { 00065 00066 } 00067 00068 cc3100_netapp::~cc3100_netapp() 00069 { 00070 00071 } 00072 00073 00074 /*****************************************************************************/ 00075 /* API functions */ 00076 /*****************************************************************************/ 00077 00078 /***************************************************************************** 00079 sl_NetAppStart 00080 *****************************************************************************/ 00081 typedef union { 00082 _NetAppStartStopCommand_t Cmd; 00083 _NetAppStartStopResponse_t Rsp; 00084 } _SlNetAppStartStopMsg_u; 00085 00086 #if _SL_INCLUDE_FUNC(sl_NetAppStart) 00087 const _SlCmdCtrl_t _SlNetAppStartCtrl = { 00088 SL_OPCODE_NETAPP_START_COMMAND, 00089 sizeof(_NetAppStartStopCommand_t), 00090 sizeof(_NetAppStartStopResponse_t) 00091 }; 00092 00093 int16_t cc3100_netapp::sl_NetAppStart(const uint32_t AppBitMap) 00094 { 00095 _SlNetAppStartStopMsg_u Msg; 00096 Msg.Cmd.appId = AppBitMap; 00097 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppStartCtrl, &Msg, NULL)); 00098 00099 return Msg.Rsp.status; 00100 } 00101 #endif 00102 00103 /***************************************************************************** 00104 sl_NetAppStop 00105 *****************************************************************************/ 00106 #if _SL_INCLUDE_FUNC(sl_NetAppStop) 00107 const _SlCmdCtrl_t _SlNetAppStopCtrl = 00108 { 00109 SL_OPCODE_NETAPP_STOP_COMMAND, 00110 sizeof(_NetAppStartStopCommand_t), 00111 sizeof(_NetAppStartStopResponse_t) 00112 }; 00113 int16_t cc3100_netapp::sl_NetAppStop(const uint32_t AppBitMap) 00114 { 00115 _SlNetAppStartStopMsg_u Msg; 00116 Msg.Cmd.appId = AppBitMap; 00117 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppStopCtrl, &Msg, NULL)); 00118 00119 return Msg.Rsp.status; 00120 } 00121 #endif 00122 00123 00124 /******************************************************************************/ 00125 /* sl_NetAppGetServiceList */ 00126 /******************************************************************************/ 00127 typedef struct { 00128 uint8_t IndexOffest; 00129 uint8_t MaxServiceCount; 00130 uint8_t Flags; 00131 int8_t Padding; 00132 } NetappGetServiceListCMD_t; 00133 00134 typedef union { 00135 NetappGetServiceListCMD_t Cmd; 00136 _BasicResponse_t Rsp; 00137 } _SlNetappGetServiceListMsg_u; 00138 00139 #if _SL_INCLUDE_FUNC(sl_NetAppGetServiceList) 00140 const _SlCmdCtrl_t _SlGetServiceListeCtrl = { 00141 SL_OPCODE_NETAPP_NETAPP_MDNS_LOOKUP_SERVICE, 00142 sizeof(NetappGetServiceListCMD_t), 00143 sizeof(_BasicResponse_t) 00144 }; 00145 00146 int16_t cc3100_netapp::sl_NetAppGetServiceList(const uint8_t IndexOffest, 00147 const uint8_t MaxServiceCount, 00148 const uint8_t Flags, 00149 int8_t *pBuffer, 00150 const uint32_t RxBufferLength 00151 ) 00152 { 00153 int32_t retVal= 0; 00154 _SlNetappGetServiceListMsg_u Msg; 00155 _SlCmdExt_t CmdExt; 00156 uint16_t ServiceSize = 0; 00157 uint16_t BufferSize = 0; 00158 00159 /* 00160 Calculate RX pBuffer size 00161 WARNING: 00162 if this size is BufferSize than 1480 error should be returned because there 00163 is no place in the RX packet. 00164 */ 00165 switch(Flags) { 00166 case SL_NET_APP_FULL_SERVICE_WITH_TEXT_IPV4_TYPE: 00167 ServiceSize = sizeof(SlNetAppGetFullServiceWithTextIpv4List_t); 00168 break; 00169 00170 case SL_NET_APP_FULL_SERVICE_IPV4_TYPE: 00171 ServiceSize = sizeof(SlNetAppGetFullServiceIpv4List_t); 00172 break; 00173 00174 case SL_NET_APP_SHORT_SERVICE_IPV4_TYPE: 00175 ServiceSize = sizeof(SlNetAppGetShortServiceIpv4List_t); 00176 break; 00177 00178 default: 00179 ServiceSize = sizeof(_BasicResponse_t); 00180 break; 00181 } 00182 00183 00184 00185 BufferSize = MaxServiceCount * ServiceSize; 00186 00187 /*Check the size of the requested services is smaller than size of the user buffer. 00188 If not an error is returned in order to avoid overwriting memory. */ 00189 if(RxBufferLength <= BufferSize) { 00190 return SL_ERROR_NETAPP_RX_BUFFER_LENGTH_ERROR; 00191 } 00192 00193 _driver._SlDrvResetCmdExt(&CmdExt); 00194 CmdExt.RxPayloadLen = BufferSize; 00195 00196 CmdExt.pRxPayload = (uint8_t *)pBuffer; 00197 00198 Msg.Cmd.IndexOffest = IndexOffest; 00199 Msg.Cmd.MaxServiceCount = MaxServiceCount; 00200 Msg.Cmd.Flags = Flags; 00201 Msg.Cmd.Padding = 0; 00202 00203 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetServiceListeCtrl, &Msg, &CmdExt)); 00204 retVal = Msg.Rsp.status; 00205 00206 return (int16_t)retVal; 00207 } 00208 00209 #endif 00210 00211 /*****************************************************************************/ 00212 /* sl_mDNSRegisterService */ 00213 /*****************************************************************************/ 00214 /* 00215 * The below struct depicts the constant parameters of the command/API RegisterService. 00216 * 00217 1. ServiceLen - The length of the service should be smaller than NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00218 2. TextLen - The length of the text should be smaller than NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00219 3. port - The port on this target host. 00220 4. TTL - The TTL of the service 00221 5. Options - bitwise parameters: 00222 bit 0 - is unique (means if the service needs to be unique) 00223 bit 31 - for internal use if the service should be added or deleted (set means ADD). 00224 bit 1-30 for future. 00225 00226 NOTE: 00227 00228 1. There are another variable parameter is this API which is the service name and the text. 00229 2. According to now there is no warning and Async event to user on if the service is a unique. 00230 * 00231 */ 00232 00233 typedef struct { 00234 uint8_t ServiceNameLen; 00235 uint8_t TextLen; 00236 uint16_t Port; 00237 uint32_t TTL; 00238 uint32_t Options; 00239 } NetappMdnsSetService_t; 00240 00241 typedef union { 00242 NetappMdnsSetService_t Cmd; 00243 _BasicResponse_t Rsp; 00244 } _SlNetappMdnsRegisterServiceMsg_u; 00245 00246 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSRegisterUnregisterService) 00247 const _SlCmdCtrl_t _SlRegisterServiceCtrl = { 00248 SL_OPCODE_NETAPP_MDNSREGISTERSERVICE, 00249 sizeof(NetappMdnsSetService_t), 00250 sizeof(_BasicResponse_t) 00251 }; 00252 00253 /****************************************************************************** 00254 00255 sl_NetAppMDNSRegisterService 00256 00257 CALLER user from its host 00258 00259 00260 DESCRIPTION: 00261 Add/delete service 00262 The function manipulates the command that register the service and call 00263 to the NWP in order to add/delete the service to/from the mDNS package and to/from the DB. 00264 00265 This register service is a service offered by the application. 00266 This unregister service is a service offered by the application before. 00267 00268 The service name should be full service name according to RFC 00269 of the DNS-SD - means the value in name field in SRV answer. 00270 00271 Example for service name: 00272 1. PC1._ipp._tcp.local 00273 2. PC2_server._ftp._tcp.local 00274 00275 If the option is_unique is set, mDNS probes the service name to make sure 00276 it is unique before starting to announce the service on the network. 00277 Instance is the instance portion of the service name. 00278 00279 00280 00281 00282 PARAMETERS: 00283 00284 The command is from constant parameters and variables parameters. 00285 00286 Constant parameters are: 00287 00288 ServiceLen - The length of the service. 00289 TextLen - The length of the service should be smaller than 64. 00290 port - The port on this target host. 00291 TTL - The TTL of the service 00292 Options - bitwise parameters: 00293 bit 0 - is unique (means if the service needs to be unique) 00294 bit 31 - for internal use if the service should be added or deleted (set means ADD). 00295 bit 1-30 for future. 00296 00297 The variables parameters are: 00298 00299 Service name(full service name) - The service name. 00300 Example for service name: 00301 1. PC1._ipp._tcp.local 00302 2. PC2_server._ftp._tcp.local 00303 00304 Text - The description of the service. 00305 should be as mentioned in the RFC 00306 (according to type of the service IPP,FTP...) 00307 00308 NOTE - pay attention 00309 00310 1. Temporary - there is an allocation on stack of internal buffer. 00311 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00312 It means that the sum of the text length and service name length cannot be bigger than 00313 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00314 If it is - An error is returned. 00315 00316 2. According to now from certain constraints the variables parameters are set in the 00317 attribute part (contain constant parameters) 00318 00319 00320 00321 RETURNS: Status - the immediate response of the command status. 00322 0 means success. 00323 00324 00325 00326 ******************************************************************************/ 00327 int16_t cc3100_netapp::sl_NetAppMDNSRegisterUnregisterService(const char* pServiceName, 00328 const uint8_t ServiceNameLen, 00329 const char* pText, 00330 const uint8_t TextLen, 00331 const uint16_t Port, 00332 const uint32_t TTL, 00333 const uint32_t Options) 00334 { 00335 00336 _SlNetappMdnsRegisterServiceMsg_u Msg; 00337 _SlCmdExt_t CmdExt ; 00338 unsigned char ServiceNameAndTextBuffer[NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH]; 00339 unsigned char *TextPtr; 00340 00341 /* 00342 00343 NOTE - pay attention 00344 00345 1. Temporary - there is an allocation on stack of internal buffer. 00346 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00347 It means that the sum of the text length and service name length cannot be bigger than 00348 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00349 If it is - An error is returned. 00350 00351 2. According to now from certain constraints the variables parameters are set in the 00352 attribute part (contain constant parameters) 00353 00354 00355 */ 00356 00357 /*build the attribute part of the command. 00358 It contains the constant parameters of the command*/ 00359 00360 Msg.Cmd.ServiceNameLen = ServiceNameLen; 00361 Msg.Cmd.Options = Options; 00362 Msg.Cmd.Port = Port; 00363 Msg.Cmd.TextLen = TextLen; 00364 Msg.Cmd.TTL = TTL; 00365 00366 /*Build the payload part of the command 00367 Copy the service name and text to one buffer. 00368 NOTE - pay attention 00369 The size of the service length + the text length should be smaller than 255, 00370 Until the simplelink drive supports to variable length through SPI command. */ 00371 if(TextLen + ServiceNameLen > (NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH - 1 )) { /*-1 is for giving a place to set null termination at the end of the text*/ 00372 return -1; 00373 } 00374 00375 _driver._SlDrvMemZero(ServiceNameAndTextBuffer, NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH); 00376 00377 00378 /*Copy the service name*/ 00379 memcpy(ServiceNameAndTextBuffer, 00380 pServiceName, 00381 ServiceNameLen); 00382 00383 if(TextLen > 0 ) { 00384 00385 TextPtr = &ServiceNameAndTextBuffer[ServiceNameLen]; 00386 /*Copy the text just after the service name*/ 00387 memcpy(TextPtr, pText, TextLen); 00388 } 00389 00390 _driver._SlDrvResetCmdExt(&CmdExt); 00391 CmdExt.TxPayloadLen = (TextLen + ServiceNameLen); 00392 CmdExt.pTxPayload = (uint8_t *)ServiceNameAndTextBuffer; 00393 00394 00395 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlRegisterServiceCtrl, &Msg, &CmdExt)); 00396 00397 return (int16_t)Msg.Rsp.status; 00398 00399 00400 } 00401 #endif 00402 00403 /**********************************************************************************************/ 00404 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSRegisterService) 00405 int16_t cc3100_netapp::sl_NetAppMDNSRegisterService(const char* pServiceName, 00406 const uint8_t ServiceNameLen, 00407 const char* pText, 00408 const uint8_t TextLen, 00409 const uint16_t Port, 00410 const uint32_t TTL, 00411 uint32_t Options) 00412 { 00413 00414 /* 00415 00416 NOTE - pay attention 00417 00418 1. Temporary - there is an allocation on stack of internal buffer. 00419 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00420 It means that the sum of the text length and service name length cannot be bigger than 00421 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00422 If it is - An error is returned. 00423 00424 2. According to now from certain constraints the variables parameters are set in the 00425 attribute part (contain constant parameters) 00426 00427 */ 00428 00429 /*Set the add service bit in the options parameter. 00430 In order not use different opcodes for the register service and unregister service 00431 bit 31 in option is taken for this purpose. if it is set it means in NWP that the service should be added 00432 if it is cleared it means that the service should be deleted and there is only meaning to pServiceName 00433 and ServiceNameLen values. */ 00434 Options |= NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT; 00435 00436 return (sl_NetAppMDNSRegisterUnregisterService( pServiceName, ServiceNameLen, pText, TextLen, Port, TTL, Options)); 00437 00438 00439 } 00440 #endif 00441 /**********************************************************************************************/ 00442 00443 00444 00445 /**********************************************************************************************/ 00446 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSUnRegisterService) 00447 int16_t cc3100_netapp::sl_NetAppMDNSUnRegisterService(const char* pServiceName, const uint8_t ServiceNameLen) 00448 { 00449 uint32_t Options = 0; 00450 00451 /* 00452 00453 NOTE - pay attention 00454 00455 The size of the service length should be smaller than 255, 00456 Until the simplelink drive supports to variable length through SPI command. 00457 00458 00459 */ 00460 00461 /*Clear the add service bit in the options parameter. 00462 In order not use different opcodes for the register service and unregister service 00463 bit 31 in option is taken for this purpose. if it is set it means in NWP that the service should be added 00464 if it is cleared it means that the service should be deleted and there is only meaning to pServiceName 00465 and ServiceNameLen values.*/ 00466 00467 Options &= (~NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT); 00468 00469 return (sl_NetAppMDNSRegisterUnregisterService(pServiceName, ServiceNameLen, NULL, 0, 0, 0, Options)); 00470 00471 00472 } 00473 #endif 00474 /**********************************************************************************************/ 00475 00476 00477 00478 /*****************************************************************************/ 00479 /* sl_DnsGetHostByService */ 00480 /*****************************************************************************/ 00481 /* 00482 * The below struct depicts the constant parameters of the command/API sl_DnsGetHostByService. 00483 * 00484 1. ServiceLen - The length of the service should be smaller than 255. 00485 2. AddrLen - TIPv4 or IPv6 (SL_AF_INET , SL_AF_INET6). 00486 * 00487 */ 00488 00489 typedef struct { 00490 uint8_t ServiceLen; 00491 uint8_t AddrLen; 00492 uint16_t Padding; 00493 } _GetHostByServiceCommand_t; 00494 00495 00496 00497 /* 00498 * The below structure depict the constant parameters that are returned in the Async event answer 00499 * according to command/API sl_DnsGetHostByService for IPv4 and IPv6. 00500 * 00501 1Status - The status of the response. 00502 2.Address - Contains the IP address of the service. 00503 3.Port - Contains the port of the service. 00504 4.TextLen - Contains the max length of the text that the user wants to get. 00505 it means that if the test of service is bigger that its value than 00506 the text is cut to inout_TextLen value. 00507 Output: Contain the length of the text that is returned. Can be full text or part 00508 of the text (see above). 00509 00510 * 00511 00512 typedef struct { 00513 uint16_t Status; 00514 uint16_t TextLen; 00515 uint32_t Port; 00516 uint32_t Address; 00517 } _GetHostByServiceIPv4AsyncResponse_t; 00518 */ 00519 00520 typedef struct { 00521 uint16_t Status; 00522 uint16_t TextLen; 00523 uint32_t Port; 00524 uint32_t Address[4]; 00525 } _GetHostByServiceIPv6AsyncResponse_t; 00526 00527 00528 typedef union { 00529 _GetHostByServiceIPv4AsyncResponse_t IpV4; 00530 _GetHostByServiceIPv6AsyncResponse_t IpV6; 00531 } _GetHostByServiceAsyncResponseAttribute_u; 00532 00533 typedef union { 00534 _GetHostByServiceCommand_t Cmd; 00535 _BasicResponse_t Rsp; 00536 } _SlGetHostByServiceMsg_u; 00537 00538 #if _SL_INCLUDE_FUNC(sl_NetAppDnsGetHostByService) 00539 const _SlCmdCtrl_t _SlGetHostByServiceCtrl = { 00540 SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICE, 00541 sizeof(_GetHostByServiceCommand_t), 00542 sizeof(_BasicResponse_t) 00543 }; 00544 00545 int32_t cc3100_netapp::sl_NetAppDnsGetHostByService(unsigned char *pServiceName, /* string containing all (or only part): name + subtype + service */ 00546 const uint8_t ServiceLen, 00547 const uint8_t Family, /* 4-IPv4 , 16-IPv6 */ 00548 uint32_t pAddr[], 00549 uint32_t *pPort, 00550 uint16_t *pTextLen, /* in: max len , out: actual len */ 00551 unsigned char *pText) 00552 { 00553 00554 _SlGetHostByServiceMsg_u Msg; 00555 _SlCmdExt_t CmdExt ; 00556 _GetHostByServiceAsyncResponse_t AsyncRsp; 00557 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00558 00559 /* 00560 Note: 00561 1. The return's attributes are belonged to first service that is found. 00562 It can be other services with the same service name will response to 00563 the query. The results of these responses are saved in the peer cache of the NWP, and 00564 should be read by another API. 00565 00566 2. Text length can be 120 bytes only - not more 00567 It is because of constraints in the NWP on the buffer that is allocated for the Async event. 00568 00569 3.The API waits to Async event by blocking. It means that the API is finished only after an Async event 00570 is sent by the NWP. 00571 00572 4.No rolling option!!! - only PTR type is sent. 00573 00574 00575 */ 00576 /*build the attribute part of the command. 00577 It contains the constant parameters of the command */ 00578 00579 Msg.Cmd.ServiceLen = ServiceLen; 00580 Msg.Cmd.AddrLen = Family; 00581 00582 /*Build the payload part of the command 00583 Copy the service name and text to one buffer.*/ 00584 _driver._SlDrvResetCmdExt(&CmdExt); 00585 CmdExt.TxPayloadLen = ServiceLen; 00586 00587 CmdExt.pTxPayload = (uint8_t *)pServiceName; 00588 00589 00590 /*set pointers to the output parameters (the returned parameters). 00591 This pointers are belonged to local struct that is set to global Async response parameter. 00592 It is done in order not to run more than one sl_DnsGetHostByService at the same time. 00593 The API should be run only if global parameter is pointed to NULL. */ 00594 AsyncRsp.out_pText = pText; 00595 AsyncRsp.inout_TextLen = (uint16_t* )pTextLen; 00596 AsyncRsp.out_pPort = pPort; 00597 AsyncRsp.out_pAddr = (uint32_t *)pAddr; 00598 00599 00600 ObjIdx = _driver._SlDrvProtectAsyncRespSetting((uint8_t*)&AsyncRsp, GETHOSYBYSERVICE_ID, SL_MAX_SOCKETS); 00601 00602 if (MAX_CONCURRENT_ACTIONS == ObjIdx) 00603 { 00604 return SL_POOL_IS_EMPTY; 00605 } 00606 00607 if (SL_AF_INET6 == Family) { 00608 g_pCB->ObjPool[ObjIdx].AdditionalData |= SL_NETAPP_FAMILY_MASK; 00609 } 00610 /* Send the command */ 00611 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetHostByServiceCtrl, &Msg, &CmdExt)); 00612 00613 00614 00615 /* If the immediate reponse is O.K. than wait for aSYNC event response. */ 00616 if(SL_RET_CODE_OK == Msg.Rsp.status) { 00617 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00618 00619 /* If we are - it means that Async event was sent. 00620 The results are copied in the Async handle return functions */ 00621 00622 Msg.Rsp.status = AsyncRsp.Status; 00623 } 00624 00625 _driver._SlDrvReleasePoolObj(ObjIdx); 00626 return Msg.Rsp.status; 00627 } 00628 #endif 00629 00630 /*****************************************************************************/ 00631 /* _sl_HandleAsync_DnsGetHostByAddr */ 00632 /*****************************************************************************/ 00633 #ifndef SL_TINY_EXT 00634 void cc3100_netapp::_sl_HandleAsync_DnsGetHostByAddr(void *pVoidBuf) 00635 { 00636 SL_TRACE0(DBG_MSG, MSG_303, "STUB: _sl_HandleAsync_DnsGetHostByAddr not implemented yet!"); 00637 return; 00638 } 00639 #endif 00640 /*****************************************************************************/ 00641 /* sl_DnsGetHostByName */ 00642 /*****************************************************************************/ 00643 typedef union { 00644 _GetHostByNameIPv4AsyncResponse_t IpV4; 00645 _GetHostByNameIPv6AsyncResponse_t IpV6; 00646 } _GetHostByNameAsyncResponse_u; 00647 00648 typedef union { 00649 _GetHostByNameCommand_t Cmd; 00650 _BasicResponse_t Rsp; 00651 } _SlGetHostByNameMsg_u; 00652 00653 #if _SL_INCLUDE_FUNC(sl_NetAppDnsGetHostByName) 00654 const _SlCmdCtrl_t _SlGetHostByNameCtrl = { 00655 SL_OPCODE_NETAPP_DNSGETHOSTBYNAME, 00656 sizeof(_GetHostByNameCommand_t), 00657 sizeof(_BasicResponse_t) 00658 }; 00659 00660 int16_t cc3100_netapp::sl_NetAppDnsGetHostByName(unsigned char * hostname, const uint16_t usNameLen, uint32_t* out_ip_addr, const uint8_t family) 00661 { 00662 _SlGetHostByNameMsg_u Msg; 00663 _SlCmdExt_t ExtCtrl; 00664 _GetHostByNameAsyncResponse_u AsyncRsp; 00665 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00666 00667 _driver._SlDrvResetCmdExt(&ExtCtrl); 00668 ExtCtrl.TxPayloadLen = usNameLen; 00669 00670 ExtCtrl.pTxPayload = (unsigned char *)hostname; 00671 00672 00673 Msg.Cmd.Len = usNameLen; 00674 Msg.Cmd.family = family; 00675 00676 /*Use Obj to issue the command, if not available try later */ 00677 ObjIdx = (uint8_t)_driver._SlDrvWaitForPoolObj(GETHOSYBYNAME_ID,SL_MAX_SOCKETS); 00678 if (MAX_CONCURRENT_ACTIONS == ObjIdx) { 00679 printf("SL_POOL_IS_EMPTY \r\n"); 00680 return SL_POOL_IS_EMPTY; 00681 } 00682 00683 _driver._SlDrvProtectionObjLockWaitForever(); 00684 00685 g_pCB->ObjPool[ObjIdx].pRespArgs = (uint8_t *)&AsyncRsp; 00686 /*set bit to indicate IPv6 address is expected */ 00687 if (SL_AF_INET6 == family) { 00688 g_pCB->ObjPool[ObjIdx].AdditionalData |= SL_NETAPP_FAMILY_MASK; 00689 } 00690 00691 _driver._SlDrvProtectionObjUnLock(); 00692 00693 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetHostByNameCtrl, &Msg, &ExtCtrl)); 00694 00695 if(SL_RET_CODE_OK == Msg.Rsp.status) { 00696 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00697 Msg.Rsp.status = AsyncRsp.IpV4.status; 00698 00699 if(SL_OS_RET_CODE_OK == (int16_t)Msg.Rsp.status) { 00700 memcpy((int8_t *)out_ip_addr, (signed char *)&AsyncRsp.IpV4.ip0, (SL_AF_INET == family) ? SL_IPV4_ADDRESS_SIZE : SL_IPV6_ADDRESS_SIZE); 00701 } 00702 } 00703 _driver._SlDrvReleasePoolObj(ObjIdx); 00704 return Msg.Rsp.status; 00705 } 00706 #endif 00707 00708 #ifndef SL_TINY_EXT 00709 void cc3100_netapp::CopyPingResultsToReport(_PingReportResponse_t *pResults,SlPingReport_t *pReport) 00710 { 00711 pReport->PacketsSent = pResults->numSendsPings; 00712 pReport->PacketsReceived = pResults->numSuccsessPings; 00713 pReport->MinRoundTime = pResults->rttMin; 00714 pReport->MaxRoundTime = pResults->rttMax; 00715 pReport->AvgRoundTime = pResults->rttAvg; 00716 pReport->TestTime = pResults->testTime; 00717 } 00718 #endif 00719 /*****************************************************************************/ 00720 /* sl_PingStart */ 00721 /*****************************************************************************/ 00722 typedef union { 00723 _PingStartCommand_t Cmd; 00724 _PingReportResponse_t Rsp; 00725 } _SlPingStartMsg_u; 00726 00727 00728 typedef enum { 00729 CMD_PING_TEST_RUNNING = 0, 00730 CMD_PING_TEST_STOPPED 00731 } _SlPingStatus_e; 00732 00733 P_SL_DEV_PING_CALLBACK pPingCallBackFunc; 00734 00735 #if _SL_INCLUDE_FUNC(sl_NetAppPingStart) 00736 int16_t cc3100_netapp::sl_NetAppPingStart(const SlPingStartCommand_t* pPingParams, const uint8_t family, SlPingReport_t *pReport, const P_SL_DEV_PING_CALLBACK pPingCallback) 00737 { 00738 00739 _SlCmdCtrl_t CmdCtrl = {0, sizeof(_PingStartCommand_t), sizeof(_BasicResponse_t)}; 00740 _SlPingStartMsg_u Msg; 00741 _PingReportResponse_t PingRsp; 00742 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00743 00744 if( 0 == pPingParams->Ip ) 00745 { /* stop any ongoing ping */ 00746 return _driver._SlDrvBasicCmd(SL_OPCODE_NETAPP_PINGSTOP); 00747 } 00748 00749 if(SL_AF_INET == family) { 00750 CmdCtrl.Opcode = SL_OPCODE_NETAPP_PINGSTART; 00751 memcpy(&Msg.Cmd.ip0, &pPingParams->Ip, SL_IPV4_ADDRESS_SIZE); 00752 } else { 00753 CmdCtrl.Opcode = SL_OPCODE_NETAPP_PINGSTART_V6; 00754 memcpy(&Msg.Cmd.ip0, &pPingParams->Ip, SL_IPV6_ADDRESS_SIZE); 00755 } 00756 00757 Msg.Cmd.pingIntervalTime = pPingParams->PingIntervalTime; 00758 Msg.Cmd.PingSize = pPingParams->PingSize; 00759 Msg.Cmd.pingRequestTimeout = pPingParams->PingRequestTimeout; 00760 Msg.Cmd.totalNumberOfAttempts = pPingParams->TotalNumberOfAttempts; 00761 Msg.Cmd.flags = pPingParams->Flags; 00762 00763 if( pPingCallback ) { 00764 pPingCallBackFunc = pPingCallback; 00765 } else { 00766 /*Use Obj to issue the command, if not available try later */ 00767 ObjIdx = (uint8_t)_driver._SlDrvWaitForPoolObj(PING_ID,SL_MAX_SOCKETS); 00768 if (MAX_CONCURRENT_ACTIONS == ObjIdx) { 00769 return SL_POOL_IS_EMPTY; 00770 } 00771 OSI_RET_OK_CHECK(_nonos.sl_LockObjLock(&g_pCB->ProtectionLockObj, NON_OS_LOCK_OBJ_UNLOCK_VALUE, NON_OS_LOCK_OBJ_LOCK_VALUE, SL_OS_WAIT_FOREVER)); 00772 /* async response handler for non callback mode */ 00773 g_pCB->ObjPool[ObjIdx].pRespArgs = (uint8_t *)&PingRsp; 00774 pPingCallBackFunc = NULL; 00775 OSI_RET_OK_CHECK(_nonos.sl_LockObjUnlock(&g_pCB->ProtectionLockObj, NON_OS_LOCK_OBJ_UNLOCK_VALUE)); 00776 } 00777 00778 00779 VERIFY_RET_OK(_driver._SlDrvCmdOp(&CmdCtrl, &Msg, NULL)); 00780 /*send the command*/ 00781 if(CMD_PING_TEST_RUNNING == (int16_t)Msg.Rsp.status || CMD_PING_TEST_STOPPED == (int16_t)Msg.Rsp.status ) { 00782 /* block waiting for results if no callback function is used */ 00783 if( NULL == pPingCallback ) { 00784 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00785 if( SL_OS_RET_CODE_OK == (int16_t)PingRsp.status ) { 00786 CopyPingResultsToReport(&PingRsp,pReport); 00787 } 00788 _driver._SlDrvReleasePoolObj(ObjIdx); 00789 } 00790 } else { 00791 /* ping failure, no async response */ 00792 if( NULL == pPingCallback ) { 00793 _driver._SlDrvReleasePoolObj(ObjIdx); 00794 } 00795 } 00796 00797 return Msg.Rsp.status; 00798 } 00799 #endif 00800 00801 /*****************************************************************************/ 00802 /* sl_NetAppSet */ 00803 /*****************************************************************************/ 00804 typedef union { 00805 _NetAppSetGet_t Cmd; 00806 _BasicResponse_t Rsp; 00807 } _SlNetAppMsgSet_u; 00808 00809 #if _SL_INCLUDE_FUNC(sl_NetAppSet) 00810 const _SlCmdCtrl_t _SlNetAppSetCmdCtrl = { 00811 SL_OPCODE_NETAPP_NETAPPSET, 00812 sizeof(_NetAppSetGet_t), 00813 sizeof(_BasicResponse_t) 00814 }; 00815 00816 int32_t cc3100_netapp::sl_NetAppSet(const uint8_t AppId ,const uint8_t Option, const uint8_t OptionLen, const uint8_t *pOptionValue) 00817 { 00818 00819 _SlNetAppMsgSet_u Msg; 00820 _SlCmdExt_t CmdExt; 00821 00822 _driver._SlDrvResetCmdExt(&CmdExt); 00823 CmdExt.TxPayloadLen = (OptionLen+3) & (~3); 00824 00825 CmdExt.pTxPayload = (uint8_t *)pOptionValue; 00826 00827 Msg.Cmd.AppId = AppId; 00828 Msg.Cmd.ConfigLen = OptionLen; 00829 Msg.Cmd.ConfigOpt = Option; 00830 00831 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppSetCmdCtrl, &Msg, &CmdExt)); 00832 00833 return (int16_t)Msg.Rsp.status; 00834 } 00835 #endif 00836 00837 /*****************************************************************************/ 00838 /* sl_NetAppSendTokenValue */ 00839 /*****************************************************************************/ 00840 typedef union { 00841 sl_NetAppHttpServerSendToken_t Cmd; 00842 _BasicResponse_t Rsp; 00843 } _SlNetAppMsgSendTokenValue_u; 00844 00845 #if defined(sl_HttpServerCallback) || defined(EXT_LIB_REGISTERED_HTTP_SERVER_EVENTS) 00846 const _SlCmdCtrl_t _SlNetAppSendTokenValueCmdCtrl = { 00847 SL_OPCODE_NETAPP_HTTPSENDTOKENVALUE, 00848 sizeof(sl_NetAppHttpServerSendToken_t), 00849 sizeof(_BasicResponse_t) 00850 }; 00851 00852 uint16_t cc3100_netapp::sl_NetAppSendTokenValue(slHttpServerData_t * Token_value) 00853 { 00854 00855 _SlNetAppMsgSendTokenValue_u Msg; 00856 _SlCmdExt_t CmdExt; 00857 00858 CmdExt.TxPayloadLen = (Token_value->value_len+3) & (~3); 00859 CmdExt.RxPayloadLen = 0; 00860 CmdExt.pTxPayload = (uint8_t *) Token_value->token_value; 00861 CmdExt.pRxPayload = NULL; 00862 00863 Msg.Cmd.token_value_len = Token_value->value_len; 00864 Msg.Cmd.token_name_len = Token_value->name_len; 00865 memcpy(&Msg.Cmd.token_name[0], Token_value->token_name, Token_value->name_len); 00866 00867 00868 VERIFY_RET_OK(_driver._SlDrvCmdSend((_SlCmdCtrl_t *)&_SlNetAppSendTokenValueCmdCtrl, &Msg, &CmdExt)); 00869 00870 return Msg.Rsp.status; 00871 } 00872 #endif 00873 00874 /*****************************************************************************/ 00875 /* sl_NetAppGet */ 00876 /*****************************************************************************/ 00877 typedef union { 00878 _NetAppSetGet_t Cmd; 00879 _NetAppSetGet_t Rsp; 00880 } _SlNetAppMsgGet_u; 00881 00882 #if _SL_INCLUDE_FUNC(sl_NetAppGet) 00883 const _SlCmdCtrl_t _SlNetAppGetCmdCtrl = { 00884 SL_OPCODE_NETAPP_NETAPPGET, 00885 sizeof(_NetAppSetGet_t), 00886 sizeof(_NetAppSetGet_t) 00887 }; 00888 00889 int32_t cc3100_netapp::sl_NetAppGet(const uint8_t AppId, const uint8_t Option, uint8_t *pOptionLen, uint8_t *pOptionValue) 00890 { 00891 _SlNetAppMsgGet_u Msg; 00892 _SlCmdExt_t CmdExt; 00893 00894 if (*pOptionLen == 0) { 00895 return SL_EZEROLEN; 00896 } 00897 00898 _driver._SlDrvResetCmdExt(&CmdExt); 00899 CmdExt.RxPayloadLen = *pOptionLen; 00900 00901 CmdExt.pRxPayload = (uint8_t *)pOptionValue; 00902 00903 Msg.Cmd.AppId = AppId; 00904 Msg.Cmd.ConfigOpt = Option; 00905 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppGetCmdCtrl, &Msg, &CmdExt)); 00906 00907 00908 if (CmdExt.RxPayloadLen < CmdExt.ActualRxPayloadLen) { 00909 *pOptionLen = (uint8_t)CmdExt.RxPayloadLen; 00910 return SL_ESMALLBUF; 00911 } else { 00912 *pOptionLen = (uint8_t)CmdExt.ActualRxPayloadLen; 00913 } 00914 00915 return (int16_t)Msg.Rsp.Status; 00916 } 00917 #endif 00918 00919 cc3100_flowcont::cc3100_flowcont(cc3100_driver &driver, cc3100_nonos &nonos) 00920 : _driver(driver), _nonos(nonos) 00921 { 00922 00923 } 00924 00925 cc3100_flowcont::~cc3100_flowcont() 00926 { 00927 00928 } 00929 #if 0 00930 /*****************************************************************************/ 00931 /* _SlDrvFlowContInit */ 00932 /*****************************************************************************/ 00933 void cc3100_flowcont::_SlDrvFlowContInit(void) 00934 { 00935 g_pCB->FlowContCB.TxPoolCnt = FLOW_CONT_MIN; 00936 00937 OSI_RET_OK_CHECK(_nonos.sl_LockObjCreate(&g_pCB->FlowContCB.TxLockObj, "TxLockObj")); 00938 00939 OSI_RET_OK_CHECK(_nonos.sl_SyncObjCreate(&g_pCB->FlowContCB.TxSyncObj, "TxSyncObj")); 00940 } 00941 00942 /*****************************************************************************/ 00943 /* _SlDrvFlowContDeinit */ 00944 /*****************************************************************************/ 00945 void cc3100_flowcont::_SlDrvFlowContDeinit(void) 00946 { 00947 g_pCB->FlowContCB.TxPoolCnt = 0; 00948 00949 OSI_RET_OK_CHECK(_nonos.sl_LockObjDelete(&g_pCB->FlowContCB.TxLockObj, 0)); 00950 00951 OSI_RET_OK_CHECK(_nonos.sl_SyncObjDelete(&g_pCB->FlowContCB.TxSyncObj, 0)); 00952 } 00953 #endif 00954 }//namespace mbed_cc3100 00955 00956
Generated on Sun Jul 17 2022 07:25:50 by
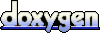