
TI's CC3100. A test demo with very little testing done!
Embed:
(wiki syntax)
Show/hide line numbers
cc3100.h
00001 /* 00002 * device.h - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 #ifndef DEVICE_H_ 00038 #define DEVICE_H_ 00039 00040 /*****************************************************************************/ 00041 /* Include files */ 00042 /*****************************************************************************/ 00043 #include "mbed.h" 00044 #include "cc3100_simplelink.h" 00045 #include "cc3100_driver.h" 00046 #include "cc3100_wlan_rx_filters.h" 00047 00048 #include "cc3100_spi.h" 00049 #include "cc3100_netcfg.h" 00050 00051 namespace mbed_cc3100 { 00052 00053 /*! 00054 00055 \addtogroup device 00056 @{ 00057 00058 */ 00059 const int16_t ROLE_UNKNOWN_ERR = -1; 00060 00061 const uint16_t MAX_BUFF_SIZE = 1460; 00062 extern uint32_t g_PingPacketsRecv; 00063 extern uint32_t g_GatewayIP; 00064 extern uint32_t g_StationIP; 00065 extern uint32_t g_DestinationIP; 00066 extern uint32_t g_BytesReceived; // variable to store the file size 00067 extern uint32_t g_Status; 00068 extern uint8_t g_buff[MAX_BUFF_SIZE+1]; 00069 extern int32_t g_SockID; 00070 00071 00072 /* File on the serial flash */ 00073 #define FILE_NAME "cc3000_module.pdf" 00074 #define HOST_NAME "www.ti.com" 00075 00076 #define HTTP_FILE_NOT_FOUND "404 Not Found" /* HTTP file not found response */ 00077 #define HTTP_STATUS_OK "200 OK" /* HTTP status ok response */ 00078 #define HTTP_CONTENT_LENGTH "Content-Length:" /* HTTP content length header */ 00079 #define HTTP_TRANSFER_ENCODING "Transfer-Encoding:" /* HTTP transfer encoding header */ 00080 #define HTTP_ENCODING_CHUNKED "chunked" /* HTTP transfer encoding header value */ 00081 #define HTTP_CONNECTION "Connection:" /* HTTP Connection header */ 00082 #define HTTP_CONNECTION_CLOSE "close" /* HTTP Connection header value */ 00083 #define HTTP_END_OF_HEADER "\r\n\r\n" /* string marking the end of headers in response */ 00084 00085 /*****************************************************************************/ 00086 /* Macro declarations */ 00087 /*****************************************************************************/ 00088 00089 const uint16_t IP_LEASE_TIME = 3600; 00090 00091 const uint16_t SIZE_45K = 46080; /* Serial flash file size 45 KB */ 00092 const uint16_t READ_SIZE = 1450; 00093 const uint8_t SPACE = 32; 00094 00095 const uint16_t PING_INTERVAL = 1000; 00096 const uint8_t PING_SIZE = 20; 00097 const uint16_t PING_TIMEOUT = 3000; 00098 const uint8_t PING_ATTEMPTS = 3; 00099 const uint8_t PING_PKT_SIZE = 20; 00100 00101 const uint8_t SL_STOP_TIMEOUT = 0xFF; 00102 00103 /* SL internal Error codes */ 00104 00105 /* Receive this error in case there are no resources to issue the command 00106 If possible, increase the number of MAX_CUNCURENT_ACTIONS (result in memory increase) 00107 If not, try again later */ 00108 const int16_t SL_POOL_IS_EMPTY = (-2000); 00109 00110 /* Receive this error in case a given length for RX buffer was too small. 00111 Receive payload was bigger than the given buffer size. Therefore, payload is cut according to receive size 00112 Recommend to increase buffer size */ 00113 const int16_t SL_ESMALLBUF = (-2001); 00114 00115 /* Receive this error in case zero length is supplied to a "get" API 00116 Recommend to supply length according to requested information (view options defines for help) */ 00117 const int16_t SL_EZEROLEN = (-2002); 00118 00119 /* User supplied invalid parameter */ 00120 const int16_t SL_INVALPARAM = (-2003); 00121 00122 /* Failed to open interface */ 00123 const int16_t SL_BAD_INTERFACE = (-2004); 00124 00125 /* End of SL internal Error codes */ 00126 00127 /*****************************************************************************/ 00128 /* Errors returned from the general error async event */ 00129 /*****************************************************************************/ 00130 00131 /* Use bit 32: Lower bits of status variable are used for NWP events 00132 * 1 in a 'status_variable', the device has completed the ping operation 00133 * 0 in a 'status_variable', the device has not completed the ping operation 00134 */ 00135 //const uint32_t STATUS_BIT_PING_DONE = 31; 00136 00137 /* Status bits - These are used to set/reset the corresponding bits in a 'status_variable' */ 00138 typedef enum { 00139 STATUS_BIT_CONNECTION = 0, /* If this bit is: 00140 * 1 in a 'status_variable', the device is connected to the AP 00141 * 0 in a 'status_variable', the device is not connected to the AP 00142 */ 00143 00144 STATUS_BIT_STA_CONNECTED, /* If this bit is: 00145 * 1 in a 'status_variable', client is connected to device 00146 * 0 in a 'status_variable', client is not connected to device 00147 */ 00148 00149 STATUS_BIT_IP_ACQUIRED, /* If this bit is: 00150 * 1 in a 'status_variable', the device has acquired an IP 00151 * 0 in a 'status_variable', the device has not acquired an IP 00152 */ 00153 00154 STATUS_BIT_IP_LEASED, /* If this bit is: 00155 * 1 in a 'status_variable', the device has leased an IP 00156 * 0 in a 'status_variable', the device has not leased an IP 00157 */ 00158 00159 STATUS_BIT_CONNECTION_FAILED, /* If this bit is: 00160 * 1 in a 'status_variable', failed to connect to device 00161 * 0 in a 'status_variable' 00162 */ 00163 00164 STATUS_BIT_P2P_NEG_REQ_RECEIVED,/* If this bit is: 00165 * 1 in a 'status_variable', connection requested by remote wifi-direct device 00166 * 0 in a 'status_variable', 00167 */ 00168 STATUS_BIT_SMARTCONFIG_DONE, /* If this bit is: 00169 * 1 in a 'status_variable', smartconfig completed 00170 * 0 in a 'status_variable', smartconfig event couldn't complete 00171 */ 00172 00173 STATUS_BIT_SMARTCONFIG_STOPPED, /* If this bit is: 00174 * 1 in a 'status_variable', smartconfig process stopped 00175 * 0 in a 'status_variable', smartconfig process running 00176 */ 00177 00178 STATUS_BIT_PING_DONE = 31 00179 /* Use bit 32: Lower bits of status variable are used for NWP events 00180 * 1 in a 'status_variable', the device has completed the ping operation 00181 * 0 in a 'status_variable', the device has not completed the ping operation 00182 */ 00183 00184 } e_StatusBits; 00185 00186 /* Application specific status/error codes */ 00187 typedef enum { 00188 LAN_CONNECTION_FAILED = -0x7D0, /* Choosing this number to avoid overlap with host-driver's error codes */ 00189 INTERNET_CONNECTION_FAILED = LAN_CONNECTION_FAILED - 1, 00190 DEVICE_NOT_IN_STATION_MODE = INTERNET_CONNECTION_FAILED - 1, 00191 HTTP_SEND_ERROR = DEVICE_NOT_IN_STATION_MODE - 1, 00192 HTTP_RECV_ERROR = HTTP_SEND_ERROR - 1, 00193 HTTP_INVALID_RESPONSE = HTTP_RECV_ERROR -1, 00194 SNTP_SEND_ERROR = DEVICE_NOT_IN_STATION_MODE - 1, 00195 SNTP_RECV_ERROR = SNTP_SEND_ERROR - 1, 00196 SNTP_SERVER_RESPONSE_ERROR = SNTP_RECV_ERROR - 1, 00197 INVALID_HEX_STRING = DEVICE_NOT_IN_STATION_MODE - 1, 00198 TCP_RECV_ERROR = INVALID_HEX_STRING - 1, 00199 TCP_SEND_ERROR = TCP_RECV_ERROR - 1, 00200 FILE_NOT_FOUND_ERROR = TCP_SEND_ERROR - 1, 00201 INVALID_SERVER_RESPONSE = FILE_NOT_FOUND_ERROR - 1, 00202 FORMAT_NOT_SUPPORTED = INVALID_SERVER_RESPONSE - 1, 00203 FILE_WRITE_ERROR = FORMAT_NOT_SUPPORTED - 1, 00204 INVALID_FILE = FILE_WRITE_ERROR - 1, 00205 00206 STATUS_CODE_MAX = -0xBB8 00207 } e_AppStatusCodes; 00208 00209 /* Send types */ 00210 typedef enum { 00211 SL_ERR_SENDER_HEALTH_MON, 00212 SL_ERR_SENDER_CLI_UART, 00213 SL_ERR_SENDER_SUPPLICANT, 00214 SL_ERR_SENDER_NETWORK_STACK, 00215 SL_ERR_SENDER_WLAN_DRV_IF, 00216 SL_ERR_SENDER_WILINK, 00217 SL_ERR_SENDER_INIT_APP, 00218 SL_ERR_SENDER_NETX, 00219 SL_ERR_SENDER_HOST_APD, 00220 SL_ERR_SENDER_MDNS, 00221 SL_ERR_SENDER_HTTP_SERVER, 00222 SL_ERR_SENDER_DHCP_SERVER, 00223 SL_ERR_SENDER_DHCP_CLIENT, 00224 SL_ERR_DISPATCHER, 00225 SL_ERR_NUM_SENDER_LAST=0xFF 00226 } SlErrorSender_e; 00227 00228 /* Error codes */ 00229 const int8_t SL_ERROR_STATIC_ADDR_SUBNET_ERROR = (-60); /* network stack error*/ 00230 const int8_t SL_ERROR_ILLEGAL_CHANNEL = (-61); /* supplicant error */ 00231 const int8_t SL_ERROR_SUPPLICANT_ERROR = (-72); /* init error code */ 00232 const int8_t SL_ERROR_HOSTAPD_INIT_FAIL = (-73); /* init error code */ 00233 const int8_t SL_ERROR_HOSTAPD_INIT_IF_FAIL = (-74); /* init error code */ 00234 const int8_t SL_ERROR_WLAN_DRV_INIT_FAIL = (-75); /* init error code */ 00235 const int8_t SL_ERROR_WLAN_DRV_START_FAIL = (-76); /* wlan start error */ 00236 const int8_t SL_ERROR_FS_FILE_TABLE_LOAD_FAILED = (-77); /* init file system failed */ 00237 const int8_t SL_ERROR_PREFERRED_NETWORKS_FILE_LOAD_FAILED = (-78); /* init file system failed */ 00238 const int8_t SL_ERROR_HOSTAPD_BSSID_VALIDATION_ERROR = (-79); /* Ap configurations BSSID error */ 00239 const int8_t SL_ERROR_HOSTAPD_FAILED_TO_SETUP_INTERFACE = (-80); /* Ap configurations interface error */ 00240 const int8_t SL_ERROR_MDNS_ENABLE_FAIL = (-81); /* mDNS enable failed */ 00241 const int8_t SL_ERROR_HTTP_SERVER_ENABLE_FAILED = (-82); /* HTTP server enable failed */ 00242 const int8_t SL_ERROR_DHCP_SERVER_ENABLE_FAILED = (-83); /* DHCP server enable failed */ 00243 const int8_t SL_ERROR_PREFERRED_NETWORK_LIST_FULL = (-93); /* supplicant error */ 00244 const int8_t SL_ERROR_PREFERRED_NETWORKS_FILE_WRITE_FAILED = (-94); /* supplicant error */ 00245 const int8_t SL_ERROR_DHCP_CLIENT_RENEW_FAILED = (-100); /* DHCP client error */ 00246 /* WLAN Connection management status */ 00247 const int8_t SL_ERROR_CON_MGMT_STATUS_UNSPECIFIED = (-102); 00248 const int8_t SL_ERROR_CON_MGMT_STATUS_AUTH_REJECT = (-103); 00249 const int8_t SL_ERROR_CON_MGMT_STATUS_ASSOC_REJECT = (-104); 00250 const int8_t SL_ERROR_CON_MGMT_STATUS_SECURITY_FAILURE = (-105); 00251 const int8_t SL_ERROR_CON_MGMT_STATUS_AP_DEAUTHENTICATE = (-106); 00252 const int8_t SL_ERROR_CON_MGMT_STATUS_AP_DISASSOCIATE = (-107); 00253 const int8_t SL_ERROR_CON_MGMT_STATUS_ROAMING_TRIGGER = (-108); 00254 const int8_t SL_ERROR_CON_MGMT_STATUS_DISCONNECT_DURING_CONNECT = (-109); 00255 const int8_t SL_ERROR_CON_MGMT_STATUS_SG_RESELECT = (-110); 00256 const int8_t SL_ERROR_CON_MGMT_STATUS_ROC_FAILURE = (-111); 00257 const int8_t SL_ERROR_CON_MGMT_STATUS_MIC_FAILURE = (-112); 00258 /* end of WLAN connection management error statuses */ 00259 const int8_t SL_ERROR_WAKELOCK_ERROR_PREFIX = (-115); /* Wake lock expired */ 00260 const int8_t SL_ERROR_LENGTH_ERROR_PREFIX = (-116); /* Uart header length error */ 00261 const int8_t SL_ERROR_MDNS_CREATE_FAIL = (-121); /* mDNS create failed */ 00262 const int8_t SL_ERROR_GENERAL_ERROR = (-127); 00263 00264 00265 00266 const int8_t SL_DEVICE_GENERAL_CONFIGURATION = (1); 00267 const int8_t SL_DEVICE_GENERAL_CONFIGURATION_DATE_TIME = (11); 00268 const int8_t SL_DEVICE_GENERAL_VERSION = (12); 00269 const int8_t SL_DEVICE_STATUS = (2); 00270 00271 /* 00272 Declare the different event group classifications 00273 The SimpleLink device send asynchronous events. Each event has a group 00274 classification according to its nature. 00275 */ 00276 #if 1 00277 /* SL_EVENT_CLASS_WLAN connection user events */ 00278 const int8_t SL_WLAN_CONNECT_EVENT = (1); 00279 const int8_t SL_WLAN_DISCONNECT_EVENT = (2); 00280 /* WLAN Smart Config user events */ 00281 const int8_t SL_WLAN_SMART_CONFIG_COMPLETE_EVENT = (3); 00282 const int8_t SL_WLAN_SMART_CONFIG_STOP_EVENT = (4); 00283 /* WLAN AP user events */ 00284 const int8_t SL_WLAN_STA_CONNECTED_EVENT = (5); 00285 const int8_t SL_WLAN_STA_DISCONNECTED_EVENT = (6); 00286 /* WLAN P2P user events */ 00287 const int8_t SL_WLAN_P2P_DEV_FOUND_EVENT = (7); 00288 const int8_t SL_WLAN_P2P_NEG_REQ_RECEIVED_EVENT = (8); 00289 const int8_t SL_WLAN_CONNECTION_FAILED_EVENT = (9); 00290 /* SL_EVENT_CLASS_DEVICE user events */ 00291 const int8_t SL_DEVICE_FATAL_ERROR_EVENT = (1); 00292 const int8_t SL_DEVICE_ABORT_ERROR_EVENT = (2); 00293 /* SL_EVENT_CLASS_BSD user events */ 00294 const int8_t SL_SOCKET_TX_FAILED_EVENT = (1); 00295 const int8_t SL_SOCKET_ASYNC_EVENT = (2); 00296 /* SL_EVENT_CLASS_NETAPP user events */ 00297 const int8_t SL_NETAPP_IPV4_IPACQUIRED_EVENT = (1); 00298 const int8_t SL_NETAPP_IPV6_IPACQUIRED_EVENT = (2); 00299 const int8_t SL_NETAPP_IP_LEASED_EVENT = (3); 00300 const int8_t SL_NETAPP_IP_RELEASED_EVENT = (4); 00301 00302 /* Server Events */ 00303 const int8_t SL_NETAPP_HTTPGETTOKENVALUE_EVENT = (1); 00304 const int8_t SL_NETAPP_HTTPPOSTTOKENVALUE_EVENT = (2); 00305 #endif 00306 00307 /* 00308 Declare the different event group classifications for sl_DevGet 00309 for getting status indications 00310 */ 00311 00312 /* Events list to mask/unmask*/ 00313 const int8_t SL_EVENT_CLASS_GLOBAL = (0); 00314 const int8_t SL_EVENT_CLASS_DEVICE = (1); 00315 const int8_t SL_EVENT_CLASS_WLAN = (2); 00316 const int8_t SL_EVENT_CLASS_BSD = (3); 00317 const int8_t SL_EVENT_CLASS_NETAPP = (4); 00318 const int8_t SL_EVENT_CLASS_NETCFG = (5); 00319 const int8_t SL_EVENT_CLASS_FS = (6); 00320 00321 00322 /****************** DEVICE CLASS status ****************/ 00323 const uint32_t EVENT_DROPPED_DEVICE_ASYNC_GENERAL_ERROR = (0x00000001L); 00324 const uint32_t STATUS_DEVICE_SMART_CONFIG_ACTIVE = (0x80000000L); 00325 00326 /****************** WLAN CLASS status ****************/ 00327 const uint32_t EVENT_DROPPED_WLAN_WLANASYNCONNECTEDRESPONSE = (0x00000001L); 00328 const uint32_t EVENT_DROPPED_WLAN_WLANASYNCDISCONNECTEDRESPONSE = (0x00000002L); 00329 const uint32_t EVENT_DROPPED_WLAN_STA_CONNECTED = (0x00000004L); 00330 const uint32_t EVENT_DROPPED_WLAN_STA_DISCONNECTED = (0x00000008L); 00331 const uint32_t STATUS_WLAN_STA_CONNECTED = (0x80000000L); 00332 00333 /****************** NETAPP CLASS status ****************/ 00334 const uint32_t EVENT_DROPPED_NETAPP_IPACQUIRED = (0x00000001L); 00335 const uint32_t EVENT_DROPPED_NETAPP_IPACQUIRED_V6 = (0x00000002L); 00336 const uint32_t EVENT_DROPPED_NETAPP_IP_LEASED = (0x00000004L); 00337 const uint32_t EVENT_DROPPED_NETAPP_IP_RELEASED = (0x00000008L); 00338 00339 /****************** BSD CLASS status ****************/ 00340 const uint32_t EVENT_DROPPED_SOCKET_TXFAILEDASYNCRESPONSE = (0x00000001L); 00341 00342 /****************** FS CLASS ****************/ 00343 00344 /*****************************************************************************/ 00345 /* Structure/Enum declarations */ 00346 /*****************************************************************************/ 00347 00348 #ifdef SL_IF_TYPE_UART 00349 typedef struct { 00350 uint32_t BaudRate; 00351 uint8_t FlowControlEnable; 00352 uint8_t CommPort; 00353 } SlUartIfParams_t; 00354 #endif 00355 00356 typedef struct { 00357 uint32_t ChipId; 00358 uint32_t FwVersion[4]; 00359 uint8_t PhyVersion[4]; 00360 } _SlPartialVersion; 00361 00362 typedef struct { 00363 _SlPartialVersion ChipFwAndPhyVersion; 00364 uint32_t NwpVersion[4]; 00365 uint16_t RomVersion; 00366 uint16_t Padding; 00367 } SlVersionFull; 00368 00369 typedef struct 00370 { 00371 uint32_t AbortType; 00372 uint32_t AbortData; 00373 }sl_DeviceReportAbort; 00374 00375 typedef struct { 00376 int8_t status; 00377 SlErrorSender_e sender; 00378 } sl_DeviceReport; 00379 00380 typedef union { 00381 sl_DeviceReport deviceEvent; 00382 sl_DeviceReportAbort deviceReport; 00383 } _SlDeviceEventData_u; 00384 00385 typedef struct { 00386 uint32_t Event; 00387 _SlDeviceEventData_u EventData; 00388 } SlDeviceEvent_t; 00389 00390 typedef struct { 00391 /* time */ 00392 uint32_t sl_tm_sec; 00393 uint32_t sl_tm_min; 00394 uint32_t sl_tm_hour; 00395 /* date */ 00396 uint32_t sl_tm_day; /* 1-31 */ 00397 uint32_t sl_tm_mon; /* 1-12 */ 00398 uint32_t sl_tm_year; /* YYYY 4 digits */ 00399 uint32_t sl_tm_week_day; /* not required */ 00400 uint32_t sl_tm_year_day; /* not required */ 00401 uint32_t reserved[3]; 00402 } SlDateTime_t; 00403 00404 /******************************************************************************/ 00405 /* Type declarations */ 00406 /******************************************************************************/ 00407 typedef void (*P_INIT_CALLBACK)(uint32_t Status); 00408 00409 class cc3100_netcfg; 00410 00411 class cc3100 00412 { 00413 00414 public: 00415 00416 cc3100(PinName cc3100_irq, PinName cc3100_nHIB, PinName cc3100_cs, SPI cc3100_spi); 00417 00418 ~cc3100(); 00419 00420 /*****************************************************************************/ 00421 /* Function prototypes */ 00422 /*****************************************************************************/ 00423 int32_t initializeAppVariables(); 00424 00425 int32_t establishConnectionWithAP(void); 00426 00427 int32_t checkLanConnection(void); 00428 00429 int32_t checkInternetConnection(void); 00430 00431 int32_t createUDPConnection(void); 00432 00433 int32_t createConnection(uint32_t DestinationIP); 00434 00435 int32_t getChunkSize(int32_t *len, uint8_t **p_Buff, uint32_t *chunk_size); 00436 00437 int32_t hexToi(unsigned char *ptr); 00438 00439 // int32_t getFile(void); 00440 00441 int32_t disconnectFromAP(void); 00442 00443 uint16_t itoa(int16_t cNum, uint8_t *cString); 00444 00445 int32_t configureSimpleLinkToDefaultState(void); 00446 00447 int16_t _sl_GetStartResponseConvert(uint32_t Status); 00448 00449 void _sl_HandleAsync_InitComplete(void *pVoidBuf); 00450 00451 bool IS_PING_DONE(uint32_t status_variable,const uint32_t bit); 00452 bool IS_CONNECTED(uint32_t status_variable,const uint32_t bit); 00453 bool IS_STA_CONNECTED(uint32_t status_variable,const uint32_t bit); 00454 bool IS_IP_ACQUIRED(uint32_t status_variable,const uint32_t bit); 00455 bool IS_IP_LEASED(uint32_t status_variable,const uint32_t bit); 00456 bool IS_CONNECTION_FAILED(uint32_t status_variable,const uint32_t bit); 00457 bool IS_P2P_NEG_REQ_RECEIVED(uint32_t status_variable,const uint32_t bit); 00458 bool IS_SMARTCONFIG_DONE(uint32_t status_variable,const uint32_t bit); 00459 bool IS_SMARTCONFIG_STOPPED(uint32_t status_variable,const uint32_t bit); 00460 00461 00462 00463 void CLR_STATUS_BIT(uint32_t status_variable, const uint32_t bit); 00464 void SET_STATUS_BIT(uint32_t status_variable, const uint32_t bit); 00465 00466 00467 /*! 00468 \brief Start the SimpleLink device 00469 00470 This function initialize the communication interface, set the enable pin 00471 of the device, and call to the init complete callback. 00472 00473 \param[in] pIfHdl Opened Interface Object. In case the interface 00474 must be opened outside the SimpleLink Driver, the 00475 user might give the handler to be used in \n 00476 any access of the communication interface with the 00477 device (UART/SPI). \n 00478 The SimpleLink driver will open an interface port 00479 only if this parameter is null! \n 00480 \param[in] pDevName The name of the device to open. Could be used when 00481 the pIfHdl is null, to transfer information to the 00482 open interface function \n 00483 This pointer could be used to pass additional information to 00484 sl_IfOpen in case it is required (e.g. UART com port name) 00485 \param[in] pInitCallBack Pointer to function that would be called 00486 on completion of the initialization process.\n 00487 If this parameter is NULL the function is 00488 blocked until the device initialization 00489 is completed, otherwise the function returns 00490 immediately. 00491 00492 \return Returns the current active role (STA/AP/P2P) or an error code: 00493 - ROLE_STA, ROLE_AP, ROLE_P2P in case of success, 00494 otherwise in failure one of the following is return: 00495 - ROLE_STA_ERR (Failure to load MAC/PHY in STA role) 00496 - ROLE_AP_ERR (Failure to load MAC/PHY in AP role) 00497 - ROLE_P2P_ERR (Failure to load MAC/PHY in P2P role) 00498 00499 00500 \sa sl_Stop 00501 00502 \note belongs to \ref basic_api 00503 00504 \warning This function must be called before any other SimpleLink API is used, or after sl_Stop is called for reinit the device 00505 \par Example: 00506 \code 00507 An example for open interface without callback routine. The interface name and handler are 00508 handled by the sl_IfOpen routine: 00509 00510 if( sl_Start(NULL, NULL, NULL) < 0 ) 00511 { 00512 LOG("Error opening interface to device\n"); 00513 } 00514 \endcode 00515 */ 00516 #if _SL_INCLUDE_FUNC(sl_Start) 00517 int16_t sl_Start(const void* pIfHdl, int8_t* pDevName, const P_INIT_CALLBACK pInitCallBack); 00518 #endif 00519 00520 /*! 00521 \brief Stop the SimpleLink device 00522 00523 This function clears the enable pin of the device, closes the communication \n 00524 interface and invokes the stop complete callback 00525 00526 \param[in] timeout Stop timeout in msec. Should be used to give the device time to finish \n 00527 any transmission/reception that is not completed when the function was called. \n 00528 Additional options: 00529 - 0 Enter to hibernate immediately \n 00530 - 0xFFFF Host waits for device's response before \n 00531 hibernating, without timeout protection \n 00532 - 0 < Timeout[msec] < 0xFFFF Host waits for device's response before \n 00533 hibernating, with a defined timeout protection \n 00534 This timeout defines the max time to wait. The NWP \n 00535 response can be sent earlier than this timeout. 00536 00537 \return On success, zero is returned. On error, -1 is returned 00538 00539 \sa sl_Start 00540 00541 \note This API will shutdown the device and invoke the "i/f close" function regardless \n 00542 if it was opened implicitly or explicitly. \n 00543 It is up to the platform interface library to properly handle interface close \n 00544 routine \n 00545 belongs to \ref basic_api \n 00546 \warning 00547 */ 00548 #if _SL_INCLUDE_FUNC(sl_Stop) 00549 int16_t sl_Stop(const uint16_t timeout); 00550 #endif 00551 00552 00553 /*! 00554 \brief Internal function for setting device configurations 00555 00556 \return On success, zero is returned. On error, -1 is 00557 returned 00558 00559 \param[in] DeviceSetId configuration id 00560 \param[in] Option configurations option 00561 \param[in] ConfigLen configurations len 00562 \param[in] pValues configurations values 00563 00564 \sa 00565 \note 00566 \warning 00567 \par Examples: 00568 \code 00569 Setting device time and date example: 00570 00571 SlDateTime_t dateTime= {0}; 00572 dateTime.sl_tm_day = (uint32_t)23; // Day of month (DD format) range 1-13 00573 dateTime.sl_tm_mon = (uint32_t)6; // Month (MM format) in the range of 1-12 00574 dateTime.sl_tm_year = (uint32_t)2014; // Year (YYYY format) 00575 dateTime.sl_tm_hour = (uint32_t)17; // Hours in the range of 0-23 00576 dateTime.sl_tm_min = (uint32_t)55; // Minutes in the range of 0-59 00577 dateTime.sl_tm_sec = (uint32_t)22; // Seconds in the range of 0-59 00578 sl_DevSet(SL_DEVICE_GENERAL_CONFIGURATION, 00579 SL_DEVICE_GENERAL_CONFIGURATION_DATE_TIME, 00580 sizeof(SlDateTime_t), 00581 (uint8_t *)(&dateTime)); 00582 00583 \endcode 00584 */ 00585 #if _SL_INCLUDE_FUNC(sl_DevSet) 00586 int32_t sl_DevSet(const uint8_t DeviceSetId , const uint8_t Option, const uint8_t ConfigLen, const uint8_t *pValues); 00587 #endif 00588 00589 /*! 00590 \brief Internal function for getting device configurations 00591 \return On success, zero is returned. On error, -1 is 00592 returned 00593 \param[in] DeviceGetId configuration id - example SL_DEVICE_STATUS 00594 \param[out] pOption Get configurations option, example for get status options 00595 - SL_EVENT_CLASS_GLOBAL 00596 - SL_EVENT_CLASS_DEVICE 00597 - SL_EVENT_CLASS_WLAN 00598 - SL_EVENT_CLASS_BSD 00599 - SL_EVENT_CLASS_NETAPP 00600 - SL_EVENT_CLASS_NETCFG 00601 - SL_EVENT_CLASS_FS 00602 \param[out] pConfigLen The length of the allocated memory as input, when the 00603 function complete, the value of this parameter would be 00604 the len that actually read from the device.\n 00605 If the device return length that is longer from the input 00606 value, the function will cut the end of the returned structure 00607 and will return SL_ESMALLBUF 00608 \param[out] pValues Get configurations values 00609 \sa 00610 \note 00611 \warning 00612 \par Examples: 00613 \code 00614 Example for getting WLAN class status: 00615 uint32_t statusWlan; 00616 uint8_t pConfigOpt; 00617 uint8_t pConfigLen; 00618 pConfigLen = sizeof(_u32); 00619 pConfigOpt = SL_EVENT_CLASS_WLAN; 00620 sl_DevGet(SL_DEVICE_STATUS,&pConfigOpt,&pConfigLen,(uint8_t *)(&statusWlan)); 00621 Example for getting version: 00622 SlVersionFull ver; 00623 pConfigLen = sizeof(ver); 00624 pConfigOpt = SL_DEVICE_GENERAL_VERSION; 00625 sl_DevGet(SL_DEVICE_GENERAL_CONFIGURATION,&pConfigOpt,&pConfigLen,(uint8_t *)(&ver)); 00626 printf("CHIP %d\nMAC 31.%d.%d.%d.%d\nPHY %d.%d.%d.%d\nNWP %d.%d.%d.%d\nROM %d\nHOST %d.%d.%d.%d\n", 00627 ver.ChipFwAndPhyVersion.ChipId, 00628 ver.ChipFwAndPhyVersion.FwVersion[0],ver.ChipFwAndPhyVersion.FwVersion[1], 00629 ver.ChipFwAndPhyVersion.FwVersion[2],ver.ChipFwAndPhyVersion.FwVersion[3], 00630 ver.ChipFwAndPhyVersion.PhyVersion[0],ver.ChipFwAndPhyVersion.PhyVersion[1], 00631 ver.ChipFwAndPhyVersion.PhyVersion[2],ver.ChipFwAndPhyVersion.PhyVersion[3], 00632 ver.NwpVersion[0],ver.NwpVersion[1],ver.NwpVersion[2],ver.NwpVersion[3], 00633 ver.RomVersion, 00634 SL_MAJOR_VERSION_NUM,SL_MINOR_VERSION_NUM,SL_VERSION_NUM,SL_SUB_VERSION_NUM); 00635 00636 \endcode 00637 \code 00638 Getting Device time and date example: 00639 00640 SlDateTime_t dateTime = {0}; 00641 int8_t configLen = sizeof(SlDateTime_t); 00642 int8_t configOpt = SL_DEVICE_GENERAL_CONFIGURATION_DATE_TIME; 00643 sl_DevGet(SL_DEVICE_GENERAL_CONFIGURATION,&configOpt, &configLen,(uint8_t *)(&dateTime)); 00644 00645 printf("Day %d,Mon %d,Year %d,Hour %,Min %d,Sec %d\n",dateTime.sl_tm_day,dateTime.sl_tm_mon,dateTime.sl_tm_year 00646 dateTime.sl_tm_hour,dateTime.sl_tm_min,dateTime.sl_tm_sec); 00647 \endcode 00648 */ 00649 #if _SL_INCLUDE_FUNC(sl_DevGet) 00650 int32_t sl_DevGet(const uint8_t DeviceGetId, uint8_t *pOption,uint8_t *pConfigLen, uint8_t *pValues); 00651 #endif 00652 00653 00654 /*! 00655 \brief Set asynchronous event mask 00656 00657 Mask asynchronous events from the device. Masked events do not 00658 generate asynchronous messages from the device. 00659 By default - all events are active 00660 00661 \param[in] EventClass The classification groups that the 00662 mask is referred to. Need to be one of 00663 the following: 00664 - SL_EVENT_CLASS_GLOBAL 00665 - SL_EVENT_CLASS_DEVICE 00666 - SL_EVENT_CLASS_WLAN 00667 - SL_EVENT_CLASS_BSD 00668 - SL_EVENT_CLASS_NETAPP 00669 - SL_EVENT_CLASS_NETCFG 00670 - SL_EVENT_CLASS_FS 00671 00672 00673 \param[in] Mask Event Mask bitmap. Valid mask are (per group): 00674 - SL_EVENT_CLASS_WLAN user events 00675 - SL_WLAN_CONNECT_EVENT 00676 - SL_WLAN_DISCONNECT_EVENT 00677 - SL_EVENT_CLASS_DEVICE user events 00678 - SL_DEVICE_FATAL_ERROR_EVENT 00679 - SL_EVENT_CLASS_BSD user events 00680 - SL_SOCKET_TX_FAILED_EVENT 00681 - SL_SOCKET_ASYNC_EVENT 00682 - SL_EVENT_CLASS_NETAPP user events 00683 - SL_NETAPP_IPV4_IPACQUIRED_EVENT 00684 - SL_NETAPP_IPV6_IPACQUIRED_EVENT 00685 00686 \return On success, zero is returned. On error, -1 is returned 00687 00688 \sa sl_EventMaskGet 00689 00690 \note belongs to \ref ext_api 00691 00692 \warning 00693 \par Example: 00694 \code 00695 00696 An example of masking connection/disconnection async events from WLAN class: 00697 sl_EventMaskSet(SL_EVENT_CLASS_WLAN, (SL_WLAN_CONNECT_EVENT | SL_WLAN_DISCONNECT_EVENT) ); 00698 00699 \endcode 00700 */ 00701 #if _SL_INCLUDE_FUNC(sl_EventMaskSet) 00702 int16_t sl_EventMaskSet(const uint8_t EventClass , const uint32_t Mask); 00703 #endif 00704 00705 /*! 00706 \brief Get current event mask of the device 00707 00708 return the events bit mask from the device. In case that event is 00709 masked, the device is not sending this event. 00710 00711 \param[in] EventClass The classification groups that the 00712 mask is referred to. Need to be one of 00713 the following: 00714 - SL_EVENT_CLASS_GLOBAL 00715 - SL_EVENT_CLASS_DEVICE 00716 - SL_EVENT_CLASS_WLAN 00717 - SL_EVENT_CLASS_BSD 00718 - SL_EVENT_CLASS_NETAPP 00719 - SL_EVENT_CLASS_NETCFG 00720 - SL_EVENT_CLASS_FS 00721 00722 \param[out] pMask Pointer to Mask bitmap where the 00723 value should be stored. Bitmasks are the same as in \ref sl_EventMaskSet 00724 00725 \return On success, zero is returned. On error, -1 is returned 00726 00727 \sa sl_EventMaskSet 00728 00729 \note belongs to \ref ext_api 00730 00731 \warning 00732 \par Example: 00733 \code 00734 00735 An example of getting an event mask for WLAN class 00736 uint32_t maskWlan; 00737 sl_StatusGet(SL_EVENT_CLASS_WLAN,&maskWlan); 00738 00739 \endcode 00740 */ 00741 #if _SL_INCLUDE_FUNC(sl_EventMaskGet) 00742 int16_t sl_EventMaskGet(const uint8_t EventClass, uint32_t *pMask); 00743 #endif 00744 00745 00746 /*! 00747 \brief the simple link task entry 00748 00749 \Param 00750 This function must be called from the main loop or from dedicated thread in 00751 the following cases: 00752 - Non-Os Platform - should be called from the mail loop 00753 - Multi Threaded Platform when the user does not implement the external spawn functions - 00754 should be called from dedicated thread allocated to the simplelink driver. 00755 In this mode the function never return. 00756 00757 \return None 00758 00759 \sa sl_Stop 00760 00761 \note belongs to \ref basic_api 00762 00763 \warning This function must be called from a thread that is start running before 00764 any call to other simple link API 00765 */ 00766 #if _SL_INCLUDE_FUNC(sl_Task) 00767 void sl_Task(void); 00768 #endif 00769 00770 00771 /*! 00772 \brief Setting the internal uart mode 00773 00774 \param[in] pUartParams Pointer to the uart configuration parameter set: 00775 baudrate - up to 711 Kbps 00776 flow control - enable/disable 00777 comm port - the comm port number 00778 00779 \return On success zero is returned, otherwise - Failed. 00780 00781 \sa sl_Stop 00782 00783 \note belongs to \ref basic_api 00784 00785 \warning This function must consider the host uart capability 00786 */ 00787 #ifdef SL_IF_TYPE_UART 00788 #if _SL_INCLUDE_FUNC(sl_UartSetMode) 00789 int16_t sl_UartSetMode(const SlUartIfParams_t* pUartParams); 00790 #endif 00791 #endif 00792 00793 public: 00794 00795 cc3100_spi _spi; 00796 cc3100_driver _driver; 00797 cc3100_nonos _nonos; 00798 cc3100_wlan _wlan; 00799 cc3100_wlan_rx_filters _wlan_filters; 00800 cc3100_netapp _netapp; 00801 cc3100_fs _fs; 00802 cc3100_netcfg _netcfg; 00803 cc3100_socket _socket; 00804 cc3100_flowcont _flowcont; 00805 00806 00807 protected: 00808 00809 00810 };//class 00811 00812 }//namespace mbed_cc3100 00813 00814 /*! 00815 00816 Close the Doxygen group. 00817 @} 00818 00819 */ 00820 00821 00822 #endif /* __DEVICE_H__ */ 00823 00824 00825
Generated on Sun Jul 17 2022 07:25:50 by
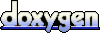