
A Port of TI's Webserver for the CC3000
Embed:
(wiki syntax)
Show/hide line numbers
evnt_handler.h
00001 /***************************************************************************** 00002 * 00003 * evnt_handler.h - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 #ifndef __EVENT_HANDLER_H__ 00036 #define __EVENT_HANDLER_H__ 00037 #include "hci.h" 00038 #include "socket.h" 00039 00040 //***************************************************************************** 00041 // 00042 // If building with a C++ compiler, make all of the definitions in this header 00043 // have a C binding. 00044 // 00045 //***************************************************************************** 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 //***************************************************************************** 00051 // 00052 // Prototypes for the APIs. 00053 // 00054 //***************************************************************************** 00055 00056 //***************************************************************************** 00057 // 00058 //! hci_event_handler 00059 //! 00060 //! @param pRetParams incoming data buffer 00061 //! @param from from information (in case of data received) 00062 //! @param fromlen from information length (in case of data received) 00063 //! 00064 //! @return none 00065 //! 00066 //! @brief Parse the incoming events packets and issues corresponding 00067 //! event handler from global array of handlers pointers 00068 // 00069 //***************************************************************************** 00070 extern unsigned char *hci_event_handler(void *pRetParams, unsigned char *from, unsigned char *fromlen); 00071 00072 //***************************************************************************** 00073 // 00074 //! hci_unsol_event_handler 00075 //! 00076 //! @param event_hdr event header 00077 //! 00078 //! @return 1 if event supported and handled 00079 //! 0 if event is not supported 00080 //! 00081 //! @brief Handle unsolicited events 00082 // 00083 //***************************************************************************** 00084 extern long hci_unsol_event_handler(char *event_hdr); 00085 00086 //***************************************************************************** 00087 // 00088 //! hci_unsolicited_event_handler 00089 //! 00090 //! @param None 00091 //! 00092 //! @return ESUCCESS if successful, EFAIL if an error occurred 00093 //! 00094 //! @brief Parse the incoming unsolicited event packets and issues 00095 //! corresponding event handler. 00096 // 00097 //***************************************************************************** 00098 extern long hci_unsolicited_event_handler(void); 00099 00100 #define M_BSD_RESP_PARAMS_OFFSET(hci_event_hdr)((char *)(hci_event_hdr) + HCI_EVENT_HEADER_SIZE) 00101 00102 #define SOCKET_STATUS_ACTIVE 0 00103 #define SOCKET_STATUS_INACTIVE 1 00104 /* Init socket_active_status = 'all ones': init all sockets with SOCKET_STATUS_INACTIVE. 00105 Will be changed by 'set_socket_active_status' upon 'connect' and 'accept' calls */ 00106 #define SOCKET_STATUS_INIT_VAL 0xFFFF 00107 #define M_IS_VALID_SD(sd) ((0 <= (sd)) && ((sd) <= 7)) 00108 #define M_IS_VALID_STATUS(status) (((status) == SOCKET_STATUS_ACTIVE)||((status) == SOCKET_STATUS_INACTIVE)) 00109 00110 extern unsigned long socket_active_status; 00111 00112 extern void set_socket_active_status(long Sd, long Status); 00113 extern long get_socket_active_status(long Sd); 00114 00115 typedef struct _bsd_accept_return_t 00116 { 00117 long iSocketDescriptor; 00118 long iStatus; 00119 sockaddr tSocketAddress; 00120 00121 } tBsdReturnParams; 00122 00123 00124 typedef struct _bsd_read_return_t 00125 { 00126 long iSocketDescriptor; 00127 long iNumberOfBytes; 00128 unsigned long uiFlags; 00129 } tBsdReadReturnParams; 00130 00131 #define BSD_RECV_FROM_FROMLEN_OFFSET (4) 00132 #define BSD_RECV_FROM_FROM_OFFSET (16) 00133 00134 00135 typedef struct _bsd_select_return_t 00136 { 00137 long iStatus; 00138 unsigned long uiRdfd; 00139 unsigned long uiWrfd; 00140 unsigned long uiExfd; 00141 } tBsdSelectRecvParams; 00142 00143 00144 typedef struct _bsd_getsockopt_return_t 00145 { 00146 unsigned char ucOptValue[4]; 00147 char iStatus; 00148 } tBsdGetSockOptReturnParams; 00149 00150 typedef struct _bsd_gethostbyname_return_t 00151 { 00152 long retVal; 00153 long outputAddress; 00154 } tBsdGethostbynameParams; 00155 00156 //***************************************************************************** 00157 // 00158 // Mark the end of the C bindings section for C++ compilers. 00159 // 00160 //***************************************************************************** 00161 #ifdef __cplusplus 00162 } 00163 #endif // __cplusplus 00164 00165 #endif // __EVENT_HANDLER_H__ 00166 00167
Generated on Wed Jul 13 2022 13:30:51 by
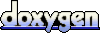