
A Port of TI's Webserver for the CC3000
Embed:
(wiki syntax)
Show/hide line numbers
cc3000.h
00001 /***************************************************************************** 00002 * 00003 * cc3000.h - CC3000 Function Definitions 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 #ifndef CC3000_H 00037 #define CC3000_H 00038 00039 #include "netapp.h" 00040 00041 00042 #define NUM_STATES 6 00043 #define FIRST_STATE_LED_NUM 1 00044 #define MAX_SSID_LEN 32 00045 00046 // CC3000 State Machine Definitions 00047 enum cc3000StateEnum 00048 { 00049 CC3000_UNINIT = 0x01, // CC3000 Driver Uninitialized 00050 CC3000_INIT = 0x02, // CC3000 Driver Initialized 00051 CC3000_ASSOC = 0x04, // CC3000 Associated to AP 00052 CC3000_IP_ALLOC = 0x08, // CC3000 has IP Address 00053 CC3000_SERVER_INIT = 0x10, // CC3000 Server Initialized 00054 }; 00055 00056 00057 00058 int ConnectUsingSSID(char * ssidName); 00059 void setupLocalSocket(void); 00060 void ConnectToServer(void); 00061 void ConnectToServer(void); 00062 00063 00064 char *sendDriverPatch(unsigned long *Length); 00065 char *sendBootLoaderPatch(unsigned long *Length); 00066 char *sendWLFWPatch(unsigned long *Length); 00067 00068 void CC3000_UsynchCallback(long lEventType, char * data, unsigned char length); 00069 00070 int initDriver(void); 00071 void StartSmartConfig(void); 00072 void closeLocalSocket(void); 00073 void disconnectAll(); 00074 00075 char isFTCSet(); 00076 void setFTCFlag(); 00077 00078 00079 // Machine State 00080 char currentCC3000State(); 00081 void setCC3000MachineState(char stat); 00082 void unsetCC3000MachineState(char stat); 00083 void resetCC3000StateMachine(); 00084 char highestCC3000State(); 00085 //static void StartUnsolicitedEventTimer(void); 00086 static void DemoInitSpi(void); 00087 00088 tNetappIpconfigRetArgs * getCC3000Info(); 00089 00090 #endif 00091
Generated on Wed Jul 13 2022 13:30:51 by
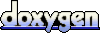