j
Fork of ds3231 by
Embed:
(wiki syntax)
Show/hide line numbers
ds3231.h
Go to the documentation of this file.
00001 /******************************************************************//** 00002 * @file ds3231.h 00003 * 00004 * @author Justin Jordan 00005 * 00006 * @version 1.0 00007 * 00008 * Started: 11NOV14 00009 * 00010 * Updated: 00011 * 00012 * @brief Header file for DS3231 class 00013 * 00014 *********************************************************************** 00015 * 00016 * @copyright 00017 * Copyright (C) 2015 Maxim Integrated Products, Inc., All Rights Reserved. 00018 * 00019 * Permission is hereby granted, free of charge, to any person obtaining a 00020 * copy of this software and associated documentation files (the "Software"), 00021 * to deal in the Software without restriction, including without limitation 00022 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00023 * and/or sell copies of the Software, and to permit persons to whom the 00024 * Software is furnished to do so, subject to the following conditions: 00025 * 00026 * The above copyright notice and this permission notice shall be included 00027 * in all copies or substantial portions of the Software. 00028 * 00029 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00030 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00031 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00032 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00033 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00034 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00035 * OTHER DEALINGS IN THE SOFTWARE. 00036 * 00037 * Except as contained in this notice, the name of Maxim Integrated 00038 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00039 * Products, Inc. Branding Policy. 00040 * 00041 * The mere transfer of this software does not imply any licenses 00042 * of trade secrets, proprietary technology, copyrights, patents, 00043 * trademarks, maskwork rights, or any other form of intellectual 00044 * property whatsoever. Maxim Integrated Products, Inc. retains all 00045 * ownership rights. 00046 **********************************************************************/ 00047 00048 00049 #ifndef DS3231_H 00050 #define DS3231_H 00051 00052 00053 #include "mbed.h" 00054 00055 00056 #define DS3231_I2C_ADRS 0x68 00057 #define I2C_WRITE 0 00058 #define I2C_READ 1 00059 00060 #define AM_PM (1 << 5) 00061 #define MODE (1 << 6) 00062 #define DY_DT (1 << 6) 00063 #define ALRM_MASK (1 << 7) 00064 00065 //control register bit masks 00066 #define A1IE (1 << 0) 00067 #define A2IE (1 << 1) 00068 #define INTCN (1 << 2) 00069 #define RS1 (1 << 3) 00070 #define RS2 (1 << 4) 00071 #define CONV (1 << 5) 00072 #define BBSQW (1 << 6) 00073 #define EOSC (1 << 7) 00074 00075 //status register bit masks 00076 #define A1F (1 << 0) 00077 #define A2F (1 << 1) 00078 #define BSY (1 << 2) 00079 #define EN32KHZ (1 << 3) 00080 #define OSF (1 << 7) 00081 00082 00083 /** 00084 * ds3231_time_t - Struct for containing time data. 00085 * 00086 * Members: 00087 * 00088 * - uint32_t seconds - Use decimal value. Member fx's convert to BCD 00089 * 00090 * - uint32_t minutes - Use decimal value. Member fx's convert to BCD 00091 * 00092 * - uint32_t hours - Use decimal value. Member fx's convert to BCD 00093 * 00094 * - bool am_pm - TRUE for PM, same logic as datasheet 00095 * 00096 * - bool mode - TRUE for 12 hour, same logic as datasheet 00097 */ 00098 typedef struct 00099 { 00100 uint32_t seconds; 00101 uint32_t minutes; 00102 uint32_t hours; 00103 bool am_pm; 00104 bool mode; 00105 }ds3231_time_t; 00106 00107 00108 /** 00109 * ds3231_calendar_t - Struct for containing calendar data. 00110 * 00111 * Members: 00112 * 00113 * - uint32_t day - Use decimal value. Member fx's convert to BCD 00114 * 00115 * - uint32_t date - Use decimal value. Member fx's convert to BCD 00116 * 00117 * - uint32_t month - Use decimal value. Member fx's convert to BCD 00118 * 00119 * - uint32_t year - Use decimal value. Member fx's convert to BCD 00120 */ 00121 typedef struct 00122 { 00123 uint32_t day; 00124 uint32_t date; 00125 uint32_t month; 00126 uint32_t year; 00127 }ds3231_calendar_t; 00128 00129 00130 /** 00131 * ds3231_alrm_t - Struct for containing alarm data. 00132 * 00133 * Members: 00134 * 00135 * - uint32_t seconds - Use decimal value. Member fx's convert to BCD 00136 * 00137 * - uint32_t minutes - Use decimal value. Member fx's convert to BCD 00138 * 00139 * - uint32_t hours - Use decimal value. Member fx's convert to BCD 00140 * 00141 * - uint32_t day - Use decimal value. Member fx's convert to BCD 00142 * 00143 * - uint32_t date - Use decimal value. Member fx's convert to BCD 00144 * 00145 * - bool am1 - Flag for setting alarm rate 00146 * 00147 * - bool am2 - Flag for setting alarm rate 00148 * 00149 * - bool am3 - Flag for setting alarm rate 00150 * 00151 * - bool am4 - Flag for setting alarm rate 00152 * 00153 * - bool am_pm - TRUE for PM, same logic as datasheet 00154 * 00155 * - bool mode - TRUE for 12 hour, same logic as datasheet 00156 * 00157 * - bool dy_dt - TRUE for Day, same logic as datasheet 00158 */ 00159 typedef struct 00160 { 00161 //Seconds and am1 not used for alarm2 00162 uint32_t seconds; 00163 uint32_t minutes; 00164 uint32_t hours; 00165 uint32_t day; 00166 uint32_t date; 00167 bool am1; 00168 bool am2; 00169 bool am3; 00170 bool am4; 00171 bool am_pm; 00172 bool mode; 00173 bool dy_dt; 00174 }ds3231_alrm_t; 00175 00176 00177 /** 00178 * ds3231_cntl_stat_t - Struct for containing control and status 00179 * register data. 00180 * 00181 * Members: 00182 * 00183 * - uint8_t control - Binary data for read/write of control register 00184 * 00185 * - uint8_t status - Binary data for read/write of status register 00186 */ 00187 typedef struct 00188 { 00189 uint8_t control; 00190 uint8_t status; 00191 }ds3231_cntl_stat_t; 00192 00193 00194 /******************************************************************//** 00195 * Ds3231 Class 00196 **********************************************************************/ 00197 class Ds3231 : public I2C 00198 { 00199 uint8_t w_adrs, r_adrs; 00200 00201 /**********************************************************//** 00202 * Private mmber fx, converts unsigned char to BCD 00203 * 00204 * On Entry: 00205 * @param[in] data - 0-255 00206 * 00207 * On Exit: 00208 * @return bcd_result = BCD representation of data 00209 * 00210 **************************************************************/ 00211 uint16_t uchar_2_bcd(uint8_t data); 00212 00213 00214 /**********************************************************//** 00215 * Private mmber fx, converts BCD to a uint8_t 00216 * 00217 * On Entry: 00218 * @param[in] bcd - 0-99 00219 * 00220 * On Exit: 00221 * @return rtn_val = integer rep. of BCD 00222 * 00223 **************************************************************/ 00224 uint8_t bcd_2_uchar(uint8_t bcd); 00225 00226 public: 00227 00228 /** 00229 * ds3231_regs_t - enumerated DS3231 registers 00230 */ 00231 typedef enum 00232 { 00233 SECONDS, 00234 MINUTES, 00235 HOURS, 00236 DAY, 00237 DATE, 00238 MONTH, 00239 YEAR, 00240 ALRM1_SECONDS, 00241 ALRM1_MINUTES, 00242 ALRM1_HOURS, 00243 ALRM1_DAY_DATE, 00244 ALRM2_MINUTES, 00245 ALRM2_HOURS, 00246 ALRM2_DAY_DATE, 00247 CONTROL, 00248 STATUS, 00249 AGING_OFFSET, //don't touch this register 00250 MSB_TEMP, 00251 LSB_TEMP 00252 }ds3231_regs_t; 00253 00254 00255 /**********************************************************//** 00256 * Constructor for Ds3231 Class 00257 * 00258 * On Entry: 00259 * @param[in] sda - sda pin of I2C bus 00260 * @param[in] scl - scl pin of I2C bus 00261 * 00262 * On Exit: 00263 * @return none 00264 * 00265 * Example: 00266 * @code 00267 * 00268 * //instantiate rtc object 00269 * Ds3231 rtc(D14, D15); 00270 * 00271 * @endcode 00272 **************************************************************/ 00273 Ds3231(PinName sda, PinName scl); 00274 00275 00276 /**********************************************************//** 00277 * Sets the time on DS3231 00278 * Struct data is in integrer format, not BCD. Fx will convert 00279 * to BCD for you. 00280 * 00281 * On Entry: 00282 * @param[in] time - struct cotaining time data; 00283 * 00284 * On Exit: 00285 * @return return value = 0 on success, non-0 on failure 00286 * 00287 * Example: 00288 * @code 00289 * 00290 * //instantiate rtc object 00291 * Ds3231 rtc(D14, D15); 00292 * 00293 * //time = 12:00:00 AM 12hr mode 00294 * ds3231_time_t time = {12, 0, 0, 0, 1} 00295 * uint16_t rtn_val; 00296 * 00297 * rtn_val = rtc.set_time(time); 00298 * 00299 * @endcode 00300 **************************************************************/ 00301 uint16_t set_time(ds3231_time_t time); 00302 00303 00304 /**********************************************************//** 00305 * Sets the calendar on DS3231 00306 * 00307 * On Entry: 00308 * @param[in] calendar - struct cotaining calendar data 00309 * 00310 * On Exit: 00311 * @return return value = 0 on success, non-0 on failure 00312 * 00313 * Example: 00314 * @code 00315 * 00316 * //instantiate rtc object 00317 * Ds3231 rtc(D14, D15); 00318 * 00319 * //see datasheet for calendar format 00320 * ds3231_calendar_t calendar = {1, 1, 1, 0}; 00321 * uint16_t rtn_val; 00322 * 00323 * rtn_val = rtc.set_calendar(calendar); 00324 * 00325 * @endcode 00326 **************************************************************/ 00327 uint16_t set_calendar(ds3231_calendar_t calendar); 00328 00329 00330 /**********************************************************//** 00331 * Set either Alarm1 or Alarm2 of DS3231 00332 * 00333 * On Entry: 00334 * @param[in] alarm - struct cotaining alarm data 00335 * 00336 * @param[in] one_r_two - TRUE for Alarm1 and FALSE for 00337 * Alarm2 00338 * 00339 * On Exit: 00340 * @return return value = 0 on success, non-0 on failure 00341 * 00342 * Example: 00343 * @code 00344 * 00345 * //instantiate rtc object 00346 * Ds3231 rtc(D14, D15); 00347 * 00348 * //see ds3231.h for .members and datasheet for alarm format 00349 * ds3231_alrm_t alarm; 00350 * uint16_t rtn_val; 00351 * 00352 * rtn_val = rtc.set_alarm(alarm, FALSE); 00353 * 00354 * @endcode 00355 **************************************************************/ 00356 uint16_t set_alarm(ds3231_alrm_t alarm, bool one_r_two); 00357 00358 00359 /**********************************************************//** 00360 * Set control and status registers of DS3231 00361 * 00362 * On Entry: 00363 * @param[in] data - Struct containing control and status 00364 * register data 00365 * 00366 * On Exit: 00367 * @return return value = 0 on success, non-0 on failure 00368 * 00369 * Example: 00370 * @code 00371 * 00372 * //instantiate rtc object 00373 * Ds3231 rtc(D14, D15); 00374 * 00375 * //do not use 0xAA, see datasheet for appropriate data 00376 * ds3231_cntl_stat_t data = {0xAA, 0xAA}; 00377 * 00378 * rtn_val = rtc.set_cntl_stat_reg(data); 00379 * 00380 * @endcode 00381 **************************************************************/ 00382 uint16_t set_cntl_stat_reg(ds3231_cntl_stat_t data); 00383 00384 00385 /**********************************************************//** 00386 * Gets the time on DS3231 00387 * 00388 * On Entry: 00389 * @param[in] time - pointer to struct for storing time data 00390 * 00391 * On Exit: 00392 * @param[out] time - contains current integrer rtc time 00393 * data 00394 * @return return value = 0 on success, non-0 on failure 00395 * 00396 * Example: 00397 * @code 00398 * 00399 * //instantiate rtc object 00400 * Ds3231 rtc(D14, D15); 00401 * 00402 * //time = 12:00:00 AM 12hr mode 00403 * ds3231_time_t time = {12, 0, 0, 0, 1} 00404 * uint16_t rtn_val; 00405 * 00406 * rtn_val = rtc.get_time(&time); 00407 * 00408 * @endcode 00409 **************************************************************/ 00410 uint16_t get_time(ds3231_time_t* time); 00411 00412 00413 /**********************************************************//** 00414 * Gets the calendar on DS3231 00415 * 00416 * On Entry: 00417 * @param[in] calendar - pointer to struct for storing 00418 * calendar data 00419 * 00420 * On Exit: 00421 * @param[out] calendar - contains current integer rtc 00422 * calendar data 00423 * @return return value = 0 on success, non-0 on failure 00424 * 00425 * Example: 00426 * @code 00427 * 00428 * //instantiate rtc object 00429 * Ds3231 rtc(D14, D15); 00430 * 00431 * //see datasheet for calendar format 00432 * ds3231_calendar_t calendar = {1, 1, 1, 0}; 00433 * uint16_t rtn_val; 00434 * 00435 * rtn_val = rtc.get_calendar(&calendar); 00436 * 00437 * @endcode 00438 **************************************************************/ 00439 uint16_t get_calendar(ds3231_calendar_t* calendar); 00440 00441 00442 /**********************************************************//** 00443 * Get either Alarm1 or Alarm2 of DS3231 00444 * 00445 * On Entry: 00446 * @param[in] alarm - pointer to struct for storing alarm 00447 * data; 00448 * 00449 * @param[in] one_r_two - TRUE for Alarm1 and FALSE for 00450 * Alarm2 00451 * 00452 * On Exit: 00453 * @param[out] alarm - contains integer alarm data 00454 * @return return value = 0 on success, non-0 on failure 00455 * 00456 * Example: 00457 * @code 00458 * 00459 * //instantiate rtc object 00460 * Ds3231 rtc(D14, D15); 00461 * 00462 * //see ds3231.h for .members and datasheet for alarm format 00463 * ds3231_alrm_t alarm; 00464 * uint16_t rtn_val; 00465 * 00466 * rtn_val = rtc.get_alarm(&alarm, FALSE); 00467 * 00468 * @endcode 00469 **************************************************************/ 00470 uint16_t get_alarm(ds3231_alrm_t* alarm, bool one_r_two); 00471 00472 00473 /**********************************************************//** 00474 * Get control and status registers of DS3231 00475 * 00476 * On Entry: 00477 * @param[in] data - pointer to struct for storing control 00478 * and status register data 00479 * 00480 * On Exit: 00481 * @param[out] data - contains control and status registers 00482 * data 00483 * @return return value = 0 on success, non-0 on failure 00484 * 00485 * Example: 00486 * @code 00487 * 00488 * //instantiate rtc object 00489 * Ds3231 rtc(D14, D15); 00490 * 00491 * //do not use 0xAA, see datasheet for appropriate data 00492 * ds3231_cntl_stat_t data = {0xAA, 0xAA}; 00493 * 00494 * rtn_val = rtc.get_cntl_stat_reg(&data); 00495 * 00496 * @endcode 00497 **************************************************************/ 00498 uint16_t get_cntl_stat_reg(ds3231_cntl_stat_t* data); 00499 00500 00501 /**********************************************************//** 00502 * Get temperature data of DS3231 00503 * 00504 * On Entry: 00505 * 00506 * On Exit: 00507 * @return return value = raw temperature data 00508 * 00509 * Example: 00510 * @code 00511 * 00512 * //instantiate rtc object 00513 * Ds3231 rtc(D14, D15); 00514 * 00515 * uint16_t temp; 00516 * 00517 * temp = rtc.get_temperature(); 00518 * 00519 * @endcode 00520 **************************************************************/ 00521 uint16_t get_temperature(void); 00522 00523 00524 /**********************************************************//** 00525 * Get epoch time based on current RTC time and date. 00526 * DS3231 must be configured and running before this fx is 00527 * called 00528 * 00529 * On Entry: 00530 * 00531 * On Exit: 00532 * @return return value = epoch time 00533 * 00534 * Example: 00535 * @code 00536 * 00537 * //instantiate rtc object 00538 * Ds3231 rtc(D14, D15); 00539 * 00540 * time_t epoch_time; 00541 * 00542 * epoch_time = rtc.get_epoch(); 00543 * 00544 * @endcode 00545 **************************************************************/ 00546 time_t get_epoch(void); 00547 00548 }; 00549 #endif /* DS3231_H*/
Generated on Sun Jul 24 2022 08:51:26 by
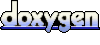