I changed one line of code in the file with path name: USBDeviceHT/targets/TARGET_Maxim
Fork of USBDeviceHT by
USBSerialBuffered Class Reference
This class is a wrapper around USBSerial such that sending of serial data over USB is supported in (and outside) of interrupt context. More...
#include <USBSerialBuffered.h>
Inherits USBSerial.
Public Member Functions | |
void | flush () |
sends internally queued but not yet sent data. | |
int | printf_irqsafe (const char *fmt,...) __attribute__((format(printf |
Writes a formatted string into an internal buffer for later sending. | |
int int | vprintf_irqsafe (const char *fmt, std::va_list ap) |
the varargs variant of printf_ | |
virtual int | _getc () |
Read a character: blocking. | |
uint8_t | available () |
Check the number of bytes available. | |
bool | connected () |
Check if the terminal is connected. | |
int | readable () |
Determine if there is a character available to read. | |
int | writeable () |
Determine if there is space available to write a character. | |
bool | writeBlock (uint8_t *buf, uint16_t size) |
Write a block of data. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void)) |
Attach a member function to call when a packet is received. | |
void | attach (void(*fptr)(void)) |
Attach a callback called when a packet is received. | |
void | attach (Callback< void()> &cb) |
Attach a Callback called when a packet is received. | |
void | attach (void(*fptr)(int baud, int bits, int parity, int stop)) |
Attach a callback to call when serial's settings are changed. | |
Protected Member Functions | |
virtual int | _putc (int c) |
Called from Stream::printf and other stdio-like methods from the base class Stream . |
Detailed Description
This class is a wrapper around USBSerial such that sending of serial data over USB is supported in (and outside) of interrupt context.
In addition it buffers characters (similiar to the I/O buffering of stdio) before starting a USB data transmit. Silently discards data if the USBSerial object is not connected to the USB host.
Definition at line 17 of file USBSerialBuffered.h.
Member Function Documentation
int _getc | ( | ) | [virtual, inherited] |
int _putc | ( | int | c ) | [protected, virtual] |
Called from Stream::printf and other stdio-like methods from the base class Stream .
Reimplemented from USBSerial.
Definition at line 176 of file USBSerialBuffered.cpp.
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) | [inherited] |
Attach a member function to call when a packet is received.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called
Definition at line 128 of file USBSerial.h.
void attach | ( | void(*)(void) | fptr ) | [inherited] |
Attach a callback called when a packet is received.
- Parameters:
-
fptr function pointer
Definition at line 139 of file USBSerial.h.
void attach | ( | Callback< void()> & | cb ) | [inherited] |
Attach a Callback called when a packet is received.
- Parameters:
-
cb Callback to attach
Definition at line 150 of file USBSerial.h.
void attach | ( | void(*)(int baud, int bits, int parity, int stop) | fptr ) | [inherited] |
Attach a callback to call when serial's settings are changed.
- Parameters:
-
fptr function pointer
Definition at line 159 of file USBSerial.h.
uint8_t available | ( | ) | [inherited] |
Check the number of bytes available.
- Returns:
- the number of bytes available
Definition at line 71 of file USBSerial.cpp.
bool connected | ( | ) | [inherited] |
Check if the terminal is connected.
- Returns:
- connection status
Definition at line 75 of file USBSerial.cpp.
void flush | ( | ) |
sends internally queued but not yet sent data.
Is blocking. Must not be called from interrupt context.
Definition at line 132 of file USBSerialBuffered.cpp.
int printf_irqsafe | ( | const char * | fmt, |
... | |||
) |
Writes a formatted string into an internal buffer for later sending.
with e.g. flush . Explicitly designed to be called from interrupt context. If the string does not fit into the internal buffer it is silently truncated.
Definition at line 103 of file USBSerialBuffered.cpp.
int readable | ( | ) | [inherited] |
Determine if there is a character available to read.
- Returns:
- 1 if there is a character available to read, 0 otherwise
Definition at line 99 of file USBSerial.h.
int vprintf_irqsafe | ( | const char * | fmt, |
std::va_list | ap | ||
) |
the varargs variant of printf_
Definition at line 111 of file USBSerialBuffered.cpp.
int writeable | ( | ) | [inherited] |
Determine if there is space available to write a character.
- Returns:
- 1 if there is space to write a character, 0 otherwise
Definition at line 107 of file USBSerial.h.
bool writeBlock | ( | uint8_t * | buf, |
uint16_t | size | ||
) | [inherited] |
Write a block of data.
For more efficiency, a block of size 64 (maximum size of a bulk endpoint) has to be written.
- Parameters:
-
buf pointer on data which will be written size size of the buffer. The maximum size of a block is limited by the size of the endpoint (64 bytes)
- Returns:
- true if successfull
Definition at line 42 of file USBSerial.cpp.
Generated on Fri Jul 15 2022 06:18:38 by
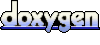