- Added setBaud() function - Added CheckNetworkStatus() function - Improved messaging system
Dependents: IoT_Ex BatteryModelTester BatteryModelTester
Fork of WiflyInterface by
Wifly.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * @section DESCRIPTION 00019 * 00020 * Wifly RN131-C, wifi module 00021 * 00022 * Datasheet: 00023 * 00024 * http://dlnmh9ip6v2uc.cloudfront.net/datasheets/Wireless/WiFi/WiFly-RN-UM.pdf 00025 */ 00026 00027 00028 00029 #ifndef WIFLY_H 00030 #define WIFLY_H 00031 00032 #include "mbed.h" 00033 #include "CBuffer.h" 00034 00035 #define DEFAULT_WAIT_RESP_TIMEOUT 500 00036 // Connection command register: 00037 #define CC_ASSOCIATION 0x0010 00038 #define CC_AUTHENTICATION 0x0020 00039 00040 extern Serial pc; 00041 00042 enum Security { 00043 NONE = 0, 00044 WEP_128 = 1, 00045 WPA = 3, 00046 WPA2 = 4, 00047 }; 00048 00049 enum Protocol { 00050 UDP = (1 << 0), 00051 TCP = (1 << 1) 00052 }; 00053 00054 /** 00055 * The Wifly class 00056 */ 00057 class Wifly 00058 { 00059 00060 public: 00061 /** 00062 * Constructor 00063 * 00064 * @param tx mbed pin to use for tx line of Serial interface 00065 * @param rx mbed pin to use for rx line of Serial interface 00066 * @param reset reset pin of the wifi module () 00067 * @param tcp_status connection status pin of the wifi module (GPIO 6) 00068 * @param ssid ssid of the network 00069 * @param phrase WEP, WPA or WPA2 key 00070 * @param sec Security type (NONE, WEP_128 or WPA, WPA2) 00071 */ 00072 Wifly( PinName tx, PinName rx, PinName reset, PinName tcp_status, const char * ssid, const char * phrase, Security sec); 00073 00074 /** 00075 * Connect the wifi module to the ssid contained in the constructor. 00076 * 00077 * @return true if connected, false otherwise 00078 */ 00079 bool join(); 00080 00081 /** Sets the baudrate of the serial port used to communicate with the ethernet/wifi adapter 00082 * @param baudrate The baudrate that the connection should be set to. Valid values are: 2400, 4800, 9600, 19200, 38400, 115200, 230400, 460800, 921600 do NOT go above 230400 00083 */ 00084 void setBaud(int baudrate); 00085 00086 /** 00087 * Disconnect the wifly module from the access point 00088 * 00089 * @return true if successful 00090 */ 00091 bool disconnect(); 00092 00093 /** 00094 * Open a tcp connection with the specified host on the specified port 00095 * 00096 * @param host host (can be either an ip address or a name. If a name is provided, a dns request will be established) 00097 * @param port port 00098 * @return true if successful 00099 */ 00100 bool connect(const char * host, int port); 00101 00102 00103 /** 00104 * Set the protocol (UDP or TCP) 00105 * 00106 * @param p protocol 00107 * @return true if successful 00108 */ 00109 bool setProtocol(Protocol p); 00110 00111 /** 00112 * Reset the wifi module 00113 */ 00114 void reset(); 00115 00116 /** 00117 * Reboot the wifi module 00118 */ 00119 bool reboot(); 00120 00121 /** 00122 * Check if characters are available 00123 * 00124 * @return number of available characters 00125 */ 00126 int readable(); 00127 00128 /** 00129 * Check if characters are available 00130 * 00131 * @return number of available characters 00132 */ 00133 int writeable(); 00134 00135 /** 00136 * Check if a tcp link is active 00137 * 00138 * @return true if successful 00139 */ 00140 bool is_connected(); 00141 00142 /** 00143 * Read a character 00144 * 00145 * @return the character read 00146 */ 00147 char getc(); 00148 00149 /** 00150 * Flush the buffer 00151 */ 00152 void flush(); 00153 00154 /** 00155 * Write a character 00156 * 00157 * @param the character which will be written 00158 */ 00159 int putc(char c); 00160 00161 00162 /** 00163 * To enter in command mode (we can configure the module) 00164 * 00165 * @return true if successful, false otherwise 00166 */ 00167 bool cmdMode(); 00168 00169 /** 00170 * To exit the command mode 00171 * 00172 * @return true if successful, false otherwise 00173 */ 00174 bool exit(); 00175 00176 /** 00177 * Close a tcp connection 00178 * 00179 * @return true if successful 00180 */ 00181 bool close(); 00182 00183 /** 00184 * Send a string to the wifi module by serial port. This function desactivates the user interrupt handler when a character is received to analyze the response from the wifi module. 00185 * Useful to send a command to the module and wait a response. 00186 * 00187 * 00188 * @param str string to be sent 00189 * @param len string length 00190 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknoledged. (default: "NO") 00191 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00192 * 00193 * @return true if ACK has been found in the response from the wifi module. False otherwise or if there is no response in 5s. 00194 */ 00195 int send(const char * str, int len, const char * ACK = NULL, char * res = NULL, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00196 00197 /** 00198 * Send a command to the wify module. Check if the module is in command mode. If not enter in command mode 00199 * 00200 * @param str string to be sent 00201 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknoledged. (default: "NO") 00202 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00203 * 00204 * @return true if successful 00205 */ 00206 bool sendCommand(const char * cmd, const char * ack = NULL, char * res = NULL, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00207 00208 /** 00209 * Check if the wifly module is still connected to the network: 00210 * 00211 * @return 0: if not connected 00212 * 1: if connected 00213 */ 00214 int checkNetworkStatus(void); 00215 00216 /** 00217 * Return true if the module is using dhcp 00218 * 00219 * @return true if the module is using dhcp 00220 */ 00221 bool isDHCP() { 00222 return state.dhcp; 00223 } 00224 00225 /** 00226 * Puts the module to sleep 00227 * 00228 */ 00229 void sleep(void); 00230 00231 bool gethostbyname(const char * host, char * ip); 00232 00233 static Wifly * getInstance() { 00234 return inst; 00235 }; 00236 00237 protected: 00238 Serial wifi; 00239 DigitalOut reset_pin; 00240 DigitalIn tcp_status; 00241 char phrase[30]; 00242 char ssid[30]; 00243 const char * ip; 00244 const char * netmask; 00245 const char * gateway; 00246 int channel; 00247 CircBuffer<char> buf_wifly; 00248 00249 static Wifly * inst; 00250 00251 void attach_rx(bool null); 00252 void handler_rx(void); 00253 00254 int serial_baudrate; 00255 00256 00257 typedef struct STATE { 00258 bool associated; 00259 bool tcp; 00260 bool dhcp; 00261 Security sec; 00262 Protocol proto; 00263 bool cmd_mode; 00264 } State; 00265 00266 State state; 00267 char * getStringSecurity(); 00268 }; 00269 00270 #endif
Generated on Sat Jul 16 2022 22:55:16 by
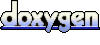