- Added setBaud() function - Added CheckNetworkStatus() function - Improved messaging system
Dependents: IoT_Ex BatteryModelTester BatteryModelTester
Fork of WiflyInterface by
WiflyInterface.cpp
00001 #include "WiflyInterface.h" 00002 00003 #define DEBUG 00004 #define INFOMESSAGES 00005 #define WARNMESSAGES 00006 #define ERRMESSAGES 00007 #define FUNCNAME "WiflyInterface" 00008 #include "messages.h" 00009 00010 00011 WiflyInterface::WiflyInterface( PinName tx, PinName rx, PinName reset, PinName tcp_status, 00012 const char * ssid, const char * phrase, Security sec) : 00013 Wifly(tx, rx, reset, tcp_status, ssid, phrase, sec) 00014 { 00015 ip_set = false; 00016 DBG("WiflyInterface Constructed"); 00017 } 00018 00019 int WiflyInterface::init() 00020 { 00021 state.dhcp = true; 00022 reset(); 00023 INFO("WiflyInterface Initialized."); 00024 return 0; 00025 } 00026 00027 int WiflyInterface::init(const char* ip, const char* mask, const char* gateway) 00028 { 00029 state.dhcp = false; 00030 this->ip = ip; 00031 strcpy(ip_string, ip); 00032 ip_set = true; 00033 this->netmask = mask; 00034 this->gateway = gateway; 00035 reset(); 00036 00037 return 0; 00038 } 00039 00040 int WiflyInterface::connect() 00041 { 00042 // join() returns a boolean, it does not like it all the time, thus casting it as int 00043 int ii = (int) join(); 00044 INFO("join() complete, return value: %d",ii); 00045 return ii; 00046 } 00047 00048 int WiflyInterface::disconnect() 00049 { 00050 return Wifly::disconnect(); 00051 } 00052 00053 char * WiflyInterface::getIPAddress() 00054 { 00055 char * match = 0; 00056 if (!ip_set) { 00057 if (!sendCommand("get ip a\r", NULL, ip_string)) 00058 return NULL; 00059 exit(); 00060 flush(); 00061 match = strstr(ip_string, "<"); 00062 if (match != NULL) { 00063 *match = '\0'; 00064 } 00065 if (strlen(ip_string) < 6) { 00066 match = strstr(ip_string, ">"); 00067 if (match != NULL) { 00068 int len = strlen(match + 1); 00069 memcpy(ip_string, match + 1, len); 00070 } 00071 } 00072 ip_set = true; 00073 } 00074 return ip_string; 00075 }
Generated on Sat Jul 16 2022 22:55:16 by
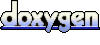