
Please run it on your NUCLEO-L152
Embed:
(wiki syntax)
Show/hide line numbers
ADA_GFX_kbv.h
00001 #ifndef _ADAFRUIT_GFX_H 00002 #define _ADAFRUIT_GFX_H 00003 00004 //#define USE_VFONTS 00005 00006 #include "Arduino.h" 00007 #define boolean bool 00008 00009 #ifndef HEX 00010 #define HEX 1 00011 #endif 00012 00013 #define swap(a, b) { int16_t t = a; a = b; b = t; } 00014 00015 class Adafruit_GFX { //: public Stream { // requires an interface 00016 00017 public: 00018 00019 Adafruit_GFX(int16_t w, int16_t h); // Constructor 00020 00021 // This MUST be defined by the subclass: 00022 virtual void drawPixel(int16_t x, int16_t y, uint16_t color) = 0; 00023 00024 // These MAY be overridden by the subclass to provide device-specific 00025 // optimized code. Otherwise 'generic' versions are used. 00026 virtual void 00027 drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color), 00028 drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color), 00029 drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color), 00030 drawRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color), 00031 fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color), 00032 fillScreen(uint16_t color), 00033 invertDisplay(boolean i); 00034 00035 // These exist only with Adafruit_GFX (no subclass overrides) 00036 void 00037 drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color), 00038 drawCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, 00039 uint16_t color), 00040 fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color), 00041 fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, 00042 int16_t delta, uint16_t color), 00043 drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, 00044 int16_t x2, int16_t y2, uint16_t color), 00045 fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, 00046 int16_t x2, int16_t y2, uint16_t color), 00047 drawRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h, 00048 int16_t radius, uint16_t color), 00049 fillRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h, 00050 int16_t radius, uint16_t color), 00051 drawBitmap(int16_t x, int16_t y, const uint8_t *bitmap, 00052 int16_t w, int16_t h, uint16_t color), 00053 drawChar(int16_t x, int16_t y, unsigned char c, uint16_t color, 00054 uint16_t bg, uint8_t size), 00055 setCursor(int16_t x, int16_t y), 00056 setTextColor(uint16_t c), 00057 setTextColor(uint16_t c, uint16_t bg), 00058 setTextSize(uint8_t s), 00059 setTextWrap(boolean w), 00060 setRotation(uint8_t r); 00061 00062 #if defined(ARDUINO) && ARDUINO < 100 00063 virtual void write(uint8_t); 00064 #else 00065 virtual size_t write(uint8_t); 00066 #endif 00067 int printf(const char* format, ...); 00068 void print(const char *s) { printf("%s", s); } 00069 void print(char *s) { printf("%s", s); } 00070 void print(char c) { printf("%c", c); } 00071 void print(int16_t v) { printf("%d", v); } 00072 void print(int32_t vl) { printf("%ld", vl); } 00073 void print(double vd, int =2) { printf("%0.2f", vd); } 00074 void println(uint8_t u, int fmt=0) { if (fmt == 0) printf("%u\n", u); else printf("%04X\n", u); } 00075 void println(uint16_t u, int fmt=0) { if (fmt == 0) printf("%u\n", u); else printf("%04X\n", u); } 00076 void println(uint32_t ul, int fmt=0) { if (fmt == 0) printf("%lu\n", ul); else printf("%04lX\n", ul); } 00077 void println(const char *s="") { printf("%s\n", s); } 00078 void println(double vd, int prec=2) { printf("%0.2f\n", vd); } 00079 00080 int16_t 00081 height(void), 00082 width(void); 00083 00084 uint8_t getRotation(void); 00085 00086 protected: 00087 const int16_t 00088 WIDTH, HEIGHT; // This is the 'raw' display w/h - never changes 00089 int16_t 00090 _width, _height, // Display w/h as modified by current rotation 00091 cursor_x, cursor_y; 00092 uint16_t 00093 textcolor, textbgcolor; 00094 uint8_t 00095 textsize, 00096 rotation; 00097 boolean 00098 wrap; // If set, 'wrap' text at right edge of display 00099 00100 #if defined USE_VFONTS 00101 public: 00102 void setFont(const PROGMEM uint8_t *ads, uint8_t mode = 0); 00103 void drawFontChar(int16_t x, int16_t y, uint8_t c, uint16_t color, uint16_t bg, uint8_t size); 00104 int8_t charWidth(uint8_t c); 00105 private: 00106 uint8_t *vfont_ads, vfont_wid, vfont_ht, vfont_ofs, vfont_siz, vfont_mode, *vfont_letter_ads; 00107 #endif 00108 00109 }; 00110 00111 #endif // _ADAFRUIT_GFX_H
Generated on Sat Jul 23 2022 01:16:42 by
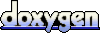