
Run a K30 CO2 sensor on a Nordic nRF52DK Board
Fork of mbed-os-example-ble-BatteryLevel by
k30.h
00001 #ifndef __K30_SERVICE_H__ 00002 #define __K30_SERVICE_H__ 00003 00004 class K30Service { 00005 public: 00006 const static uint16_t K30_SERVICE_UUID = 0xA000; 00007 const static uint16_t K30_VALUE_CHARACTERISTIC_UUID = 0xA001; 00008 00009 K30Service(BLEDevice &_ble, float k30Initial) : 00010 ble(_ble), k30Value(K30_VALUE_CHARACTERISTIC_UUID, &k30Initial, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY) 00011 { 00012 GattCharacteristic *charTable[] = {&k30Value}; 00013 GattService k30Service(K30Service::K30_SERVICE_UUID, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00014 ble.addService(k30Service); 00015 } 00016 00017 void updateK30Value(float newValue) { 00018 ble.updateCharacteristicValue(k30Value.getValueHandle(), (uint8_t *)&newValue, sizeof(float)); 00019 } 00020 00021 private: 00022 BLEDevice &ble; 00023 ReadOnlyGattCharacteristic<float> k30Value; 00024 }; 00025 00026 #endif /* #ifndef __K30_SERVICE_H__ */
Generated on Thu Jul 14 2022 19:33:10 by
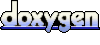