
Bible eBook Prototype More details at: http://mbed.org/users/davervw/notebook/ebible-abstract/
Dependencies: SDHCFileSystem TextLCD mbed BibleIO
BibleUI.h
00001 /* Bible UI Class Definition - KJV Bible eBook Browser 00002 * 00003 * Copyright (c) 2011 David R. Van Wagner davervw@yahoo.com 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #include <mbed.h> 00025 #include "BibleIO.h" 00026 #include "TextLCD.h" 00027 00028 class BibleUI 00029 { 00030 private: 00031 short book; 00032 short chapter; 00033 short verse; 00034 short offset; 00035 short disp_len; 00036 BibleIO &HolyBible; 00037 TextLCD &lcd; 00038 DigitalIn& lb; 00039 DigitalIn& rb; 00040 DigitalOut led1; 00041 DigitalOut led2; 00042 DigitalOut led3; 00043 DigitalOut led4; 00044 00045 public: 00046 BibleUI(BibleIO &bible, TextLCD &textlcd, DigitalIn& left, DigitalIn& right); 00047 void start(); 00048 static void indexing(int progress, void* context); 00049 00050 private: 00051 void display_nav(); 00052 void display_verse(); 00053 void show_title(); 00054 void main(); 00055 bool append_next_verse(char*& text, short len, short book, short chapter, short verse); 00056 void remove_string(char* &text, char* find); 00057 int word_wrap(char* &text); 00058 };
Generated on Sat Jul 16 2022 05:22:41 by
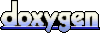