
Dependencies: keypad SDHCFileSystem TextLCD mbed FPointer wave_player
main.cpp
00001 /* 00002 Final Year Project for Ngee Ann Polytechnic Electrical Engineering Year 3 00003 Project Title: Bus Assistance for the Visually Impaired 00004 */ 00005 #include "mbed.h" 00006 #include "scmRTOS.h" 00007 #include "wave_player.h" 00008 #include "SDHCFileSystem.h" 00009 #include "keypad.h" 00010 #include "TextLCD.h" 00011 00012 Serial xbee(p9, p10); 00013 DigitalOut rst1(p11); 00014 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00015 AnalogOut AudioOut(p18); 00016 wave_player waver(&AudioOut); 00017 //Keypad keypad( p24, p23, p22, p21, p28, p27, p26, p25); 00018 TextLCD lcd(p15, p16, p17, p18, p19, p20); 00019 DigitalOut myled(LED1);//Create variable for Led 1 on the mbed 00020 DigitalOut myled1(LED2);//Create variable for Led 2 on the mbed 00021 //BusOut seven(p27, p26, p25, p24, p23, p22, p21); 00022 //BusOut leds(p28, p29, p30); 00023 00024 DigitalOut led(LED1); 00025 DigitalOut led1(LED2); 00026 00027 //OS Timer/interrupt 00028 OS::TEventFlag XBee; 00029 00030 // process types 00031 //typedef OS::process<OS::pr1, 5000> TProc1; //7 Segment LED 00032 typedef OS::process<OS::pr0, 4000> TProc2; // XBee Communication 00033 //typedef OS::process<OS::pr0, 8000> TProc3; // Playing of Audio 00034 //typedef OS::process<OS::pr0 1000> TProc4; // Interrupt of Keypad 00035 00036 // process objects 00037 //TProc1 Proc1; 00038 TProc2 Proc2; 00039 //TProc3 Proc3; 00040 //TProc4 Proc4 00041 00042 #define MAXBUS 10 00043 #define MAXLEN 4 00044 #define ENDKEY 14 00045 int req; 00046 char Bus[MAXBUS][MAXLEN]; 00047 char NumIx = 0; // Point to current index of Bus number 00048 char BusIx = 0; // Point to current bus 00049 bool BusFull = false; // Bus number buffer is full 00050 char KeyCh; 00051 00052 00053 char Keytable[] = { '1', '2', '3', 'A', 00054 '4', '5', '6', 'B', 00055 '7', '8', '9', 'C', 00056 '*', '0', '#', 'D' 00057 }; 00058 00059 uint32_t cbAfterInput(uint32_t key) { 00060 KeyCh = Keytable[key]; 00061 00062 if (BusFull) { 00063 printf("Bus number is already full\n"); 00064 return 2; 00065 } 00066 00067 00068 if (NumIx < MAXLEN - 1) { 00069 if (key != ENDKEY) { // Terminating key 00070 lcd.putc(KeyCh); 00071 printf("%c", KeyCh); 00072 00073 00074 } else { // Terminating key is entered 00075 printf("\nBus(%d,%d)=%c\n", BusIx, NumIx, KeyCh); 00076 BusIx++; 00077 NumIx = 0; 00078 } 00079 } 00080 return 0; 00081 } 00082 00083 //--------------------------------------------------------------------------- 00084 long long count = 0; 00085 00086 int main() { 00087 printf("\nInitialising ...\n"); 00088 rst1 = 0; //Set reset pin to 0 00089 myled = 0;//Set LED1 to 0 00090 myled1 = 0;//Set LED2 to 0 00091 wait_ms(1);//Wait at least one millisecond 00092 rst1 = 1;//Set reset pin to 1 00093 wait_ms(1);//Wait another millisecond 00094 OS::Run(); 00095 memset(&Bus, 0, MAXBUS * MAXLEN); 00096 //seven = Bus[BusIx][NumIx]; 00097 00098 } 00099 00100 //--------------------------------------------------------------------------- 00101 template<> OS_PROCESS void TProc1::Exec() { 00102 int i; 00103 int n = 1; 00104 for (;;) { // toggling between 7 seg led 00105 for (int i=0; i<3; i++) { 00106 leds = 0x01 << n; 00107 n = (++n) % 3; 00108 seven = Bus[BusIx][NumIx]; 00109 00110 } 00111 } 00112 } 00113 00114 //--------------------------------------------------------------------------- 00115 template<> OS_PROCESS void TProc2::Exec() { 00116 00117 for (;;) { 00118 if (xbee.readable()) { 00119 req = xbee.getc(); 00120 printf("req = %c\n", req); 00121 //XBee.SignalISR(); 00122 Sleep(2000); 00123 } 00124 } 00125 } 00126 00127 00128 //--------------------------------------------------------------------------- 00129 template<> OS_PROCESS void TProc3::Exec() { 00130 00131 for (;;) { 00132 00133 if (req == 'r') { 00134 printf("Came here 2 \n"); 00135 FILE *fp = fopen("/sd/wf/Bus.wav","r"); 00136 waver.play(fp); 00137 printf("playing"); 00138 fseek(fp, 0, SEEK_SET); 00139 fclose(fp); 00140 00141 } 00142 } 00143 00144 //--------------------------------------------------------------------------- 00145 template<> OS_PROCESS void TProc4::Exec() { 00146 00147 keypad.CallAfterInput(&cbAfterInput); 00148 keypad.Start(); 00149 while (1) { 00150 wait_ms(100); 00151 } 00152 } 00153 00154 //--------------------------------------------------------------------------- 00155 void OS::SystemTimerUserHook() { 00156 ++count; 00157 if (count % 2000 == 0) { 00158 printf("\n%lld\n", count); 00159 } 00160 } 00161 } 00162 //--------------------------------------------------------------------------- 00163 void OS::IdleProcessUserHook() { 00164 __WFI(); 00165 }
Generated on Tue Jul 12 2022 17:55:58 by
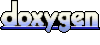