This library controls a ST TDA7419 audio control IC. This is part of a project to implement an mbed controlled car stereo. The TDA7419 will take in stereo and output four channels of audio plus a subwoofer channel.
PreampTDA7419.cpp
00001 /** PreampTDA7419 Library 00002 * 00003 * @Author: Dan Cohen 00004 * 00005 */ 00006 00007 #include "mbed.h" 00008 #include "PreampTDA7419.h" 00009 #include <stdio.h> 00010 #include <string.h> 00011 00012 PreampTDA7419::PreampTDA7419(PinName sda, PinName scl): 00013 _device(sda, scl) 00014 { 00015 _volume = 6; 00016 _input = 1; 00017 00018 _mute = 0; 00019 _mix = 0; 00020 00021 // for register 4 Treble Filter 00022 _referenceInE = 0; 00023 _trebleCenterFreq = 0; 00024 _treble = 0; 00025 00026 // for middle frequecy filter 00027 _middleSoftStep = 0; 00028 _middleQ = 0; 00029 _middle = 0; 00030 00031 _atten_lf = 9; 00032 _atten_rf = 9; 00033 _atten_lr = 9; 00034 _atten_rr = 9; 00035 _atten_mix = 9; 00036 _atten_sub = 9; 00037 00038 } 00039 00040 void PreampTDA7419::writeToTDA7419 (int command, int value) 00041 { 00042 int transmissionSuccessful; 00043 _device.start(); 00044 transmissionSuccessful = _device.write(TDA7419_ADDRESS<<1); 00045 transmissionSuccessful |= _device.write(command); 00046 transmissionSuccessful |= _device.write(value); 00047 _device.stop(); 00048 // return (transmissionSuccessful); 00049 } 00050 00051 ///////////////////////////////// 00052 // set the speaker attenuators // 00053 ///////////////////////////////// 00054 // attenuation can be set from 0 to 11 and this is mapped to the 00055 // values that the TDA7419 uses for it's attenuation (0->h60) 00056 00057 // 00058 // (FL/FR/RL/RR/SWL/SWR) (13-18) 00059 void PreampTDA7419::setAttenuationReg(int regAddr, int attenuation) 00060 { 00061 int regAtten; 00062 if (attenuation == 11) { 00063 regAtten = 13; 00064 } else if (attenuation == 10) { 00065 regAtten = 6; 00066 } else { 00067 regAtten = (99-(attenuation*9)); 00068 } 00069 writeToTDA7419(regAddr, regAtten); 00070 } 00071 00072 int PreampTDA7419::calcToneAttenuationReg(int attenuation) 00073 { 00074 int regAtten; 00075 if (attenuation > 0) { 00076 regAtten = 16 + (attenuation * 3); 00077 } else if (attenuation == 0) { 00078 regAtten = 0; 00079 } else if (attenuation < 0) { 00080 regAtten = 0 - (attenuation * 3); 00081 } 00082 return (regAtten); 00083 } 00084 00085 // update all of the registers in the TDA7419 00086 void PreampTDA7419::updateTDA7419Reg() 00087 { 00088 00089 int s_main_source = 0; 00090 int s_main_loud = 1 | 0x40; 00091 int s_softmute = 2 | 0x40; 00092 int s_volume = 3 | 0x40; 00093 int s_treble = 4 | 0x40; 00094 int s_middle = 5 | 0x40; 00095 int s_bass = 6 | 0x40; 00096 int s_second_source = 7 | 0x40; 00097 int s_sub_mid_bass = 8 | 0x40; 00098 int s_mix_gain = 9 | 0x40; 00099 int s_atten_lf = 10 | 0x40; 00100 int s_atten_rf = 11 | 0x40; 00101 int s_atten_lr = 12 | 0x40; 00102 int s_atten_rr = 13 | 0x40; 00103 int s_atten_mix = 14 | 0x40; 00104 int s_atten_sub = 15 | 0x40; 00105 int s_spectrum = 16 | 0x40; 00106 // int s_test = 17 | 0x40; 00107 00108 ////////////////////////////////////////////////////////////////// 00109 // Calculate actual register values from the variables that the // 00110 // buttons control // 00111 ////////////////////////////////////////////////////////////////// 00112 00113 ////////////////////////// 00114 // update the registers // 00115 ////////////////////////// 00116 writeToTDA7419(s_main_source, ( (0x78) | (_input & 0x3) ) ); 00117 writeToTDA7419(s_main_loud, (0xc0)); 00118 writeToTDA7419(s_softmute, (0xa7)); 00119 setAttenuationReg(s_volume, _volume); 00120 00121 00122 // tone register attenuation isn't simple so moving that 00123 // calculation to a separate function 00124 // locking softwtep as '0' because that is on and I think we 00125 // want soft step! 00126 writeToTDA7419(s_treble, 00127 ( (0 & 0x1 ) << 7 ) | 00128 ( (1 & 0x3 ) << 5 ) | 00129 ( (calcToneAttenuationReg(_treble) & 0x1f ) ) ); 00130 00131 writeToTDA7419(s_middle, 00132 ( (0 & 0x1 ) << 7 ) | 00133 ( (1 & 0x3 ) << 5 ) | 00134 ( (calcToneAttenuationReg(_middle) & 0x1f ) ) ); 00135 00136 writeToTDA7419(s_bass, 00137 ( (0 & 0x1 ) << 7 ) | 00138 ( (1 & 0x3 ) << 5 ) | 00139 ( (calcToneAttenuationReg(_bass) & 0x1f ) ) ); 00140 00141 00142 // this register allows the second source to be routed to the rear speakers 00143 // not useful in the context of this project 00144 writeToTDA7419(s_second_source, (0x07)); 00145 00146 // this is the subwoofer cut-off frequency 00147 // 11 which is 160Khz) 00148 writeToTDA7419(s_sub_mid_bass, (0x63)); 00149 00150 // mix to the front speakers, enable the sub, no gain 00151 if (_mix == 1) { 00152 writeToTDA7419(s_mix_gain, (0xf7)); 00153 } else { 00154 writeToTDA7419(s_mix_gain, (0xf0)); 00155 } 00156 00157 setAttenuationReg(s_atten_lf , _atten_lf ); 00158 setAttenuationReg(s_atten_rf , _atten_rf ); 00159 setAttenuationReg(s_atten_lr , _atten_lr ); 00160 setAttenuationReg(s_atten_rr , _atten_rr ); 00161 00162 setAttenuationReg(s_atten_mix , _atten_mix ); 00163 setAttenuationReg(s_atten_sub , _atten_sub ); 00164 00165 writeToTDA7419 (s_spectrum, (0x09)); 00166 00167 } 00168 00169 /* setVolume: This sets the volume within the valid range of 0->11 00170 return indicates it was successfully set */ 00171 void PreampTDA7419::setVolume(int volume) 00172 { 00173 if (volume > 11) { 00174 _volume = 11; 00175 } else if (volume < 0) { 00176 volume = 0; 00177 } else { 00178 _volume = volume; 00179 } 00180 updateTDA7419Reg(); 00181 } 00182 00183 /* readVolume: return the volume level that is currently set */ 00184 int PreampTDA7419::readVolume() 00185 { 00186 return (_volume); 00187 } 00188 /* readVolume: return the volume level that is currently set */ 00189 int PreampTDA7419::increaseVolume() 00190 { 00191 _volume++; 00192 setVolume(_volume); 00193 return (_volume); 00194 } 00195 /* readVolume: return the volume level that is currently set */ 00196 int PreampTDA7419::decreaseVolume() 00197 { 00198 _volume--; 00199 setVolume(_volume); 00200 return (_volume); 00201 } 00202 00203 void PreampTDA7419::setInput(int input) 00204 { 00205 if (input > 3) { 00206 _input = 3; 00207 } else if (input < 0) { 00208 input = 0; 00209 } else { 00210 _input = input; 00211 } 00212 updateTDA7419Reg(); 00213 } 00214 00215 int PreampTDA7419::readInput() 00216 { 00217 return (_input); 00218 } 00219 00220 int PreampTDA7419::increaseTreble() 00221 { 00222 if (_treble < 5) { 00223 _treble++; 00224 } 00225 updateTDA7419Reg(); 00226 return(_treble); 00227 } 00228 00229 int PreampTDA7419::decreaseTreble() 00230 { 00231 if (_treble > -5) { 00232 _treble--; 00233 } 00234 updateTDA7419Reg(); 00235 return(_treble); 00236 } 00237 00238 int PreampTDA7419::readTreble() 00239 { 00240 return (_treble); 00241 } 00242 00243 int PreampTDA7419::increaseMiddle() 00244 { 00245 if (_middle < 5) { 00246 _middle++; 00247 } 00248 updateTDA7419Reg(); 00249 return(_middle); 00250 } 00251 00252 int PreampTDA7419::decreaseMiddle() 00253 { 00254 if (_middle > -5) { 00255 _middle--; 00256 } 00257 updateTDA7419Reg(); 00258 return(_middle); 00259 } 00260 00261 int PreampTDA7419::readMiddle() 00262 { 00263 return (_middle); 00264 } 00265 00266 int PreampTDA7419::increaseBass() 00267 { 00268 if (_bass < 5) { 00269 _bass++; 00270 } 00271 updateTDA7419Reg(); 00272 return(_bass); 00273 } 00274 00275 int PreampTDA7419::decreaseBass() 00276 { 00277 if (_bass > -5) { 00278 _bass--; 00279 } 00280 updateTDA7419Reg(); 00281 return(_bass); 00282 } 00283 00284 int PreampTDA7419::readBass() 00285 { 00286 return (_bass); 00287 } 00288 00289 int PreampTDA7419::increaseSpeaker (int speakerNumber) { 00290 switch (speakerNumber) { 00291 case (1): if (_atten_lf < 11) { _atten_lf++; }; updateTDA7419Reg(); return( _atten_lf ); 00292 case (2): if (_atten_rf < 11) { _atten_rf++; }; updateTDA7419Reg(); return( _atten_rf ); 00293 case (3): if (_atten_lr < 11) { _atten_lr++; }; updateTDA7419Reg(); return( _atten_lr ); 00294 case (4): if (_atten_rr < 11) { _atten_rr++; }; updateTDA7419Reg(); return( _atten_rr ); 00295 case (5): if (_atten_sub < 11) { _atten_sub++; }; updateTDA7419Reg(); return( _atten_sub ); 00296 case (6): if (_atten_mix < 11) { _atten_mix++; }; updateTDA7419Reg(); return( _atten_mix ); 00297 } 00298 return (_atten_lf ); 00299 } 00300 00301 int PreampTDA7419::decreaseSpeaker (int speakerNumber) { 00302 switch (speakerNumber) { 00303 case (1): if (_atten_lf > 0) { _atten_lf--; }; updateTDA7419Reg(); return( _atten_lf ); 00304 case (2): if (_atten_rf > 0) { _atten_rf--; }; updateTDA7419Reg(); return( _atten_rf ); 00305 case (3): if (_atten_lr > 0) { _atten_lr--; }; updateTDA7419Reg(); return( _atten_lr ); 00306 case (4): if (_atten_rr > 0) { _atten_rr--; }; updateTDA7419Reg(); return( _atten_rr ); 00307 case (5): if (_atten_sub > 0) { _atten_sub--; }; updateTDA7419Reg(); return( _atten_sub ); 00308 case (6): if (_atten_mix > 0) { _atten_mix--; }; updateTDA7419Reg(); return( _atten_mix ); 00309 } 00310 return (_atten_lf ); 00311 } 00312 00313 int PreampTDA7419::readSpeaker (int speakerNumber) { 00314 switch (speakerNumber) { 00315 case (1): return( _atten_lf ); 00316 case (2): return( _atten_rf ); 00317 case (3): return( _atten_lr ); 00318 case (4): return( _atten_rr ); 00319 case (5): return( _atten_sub ); 00320 case (6): return( _atten_mix ); 00321 } 00322 return (_atten_lf ); 00323 } 00324 00325 int PreampTDA7419::toggleMix() { 00326 _mix = !_mix; 00327 updateTDA7419Reg(); 00328 return (_mix); 00329 } 00330 00331 int PreampTDA7419::readMix() { 00332 return (_mix); 00333 }
Generated on Thu Jul 14 2022 04:32:30 by
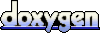