
MAX77801 Demo. The MAX77801 is a high-current, high-efficiency buck-boost Regulator and features I2C-compatible, 2-wire serial interface consisting of a bidirectional serial-data line (SDA) and a serial-clock line (SCL). Datasheet: https://datasheets.maximintegrated.com/en/ds/MAX77801.pdf Tested using MAX32630FTHR This Demo simply provides the test interface via USB Serial port. ----- [ MAX77801 DEMO ] Usage ----- i : Set Registers to POR Staus + : Increase VOUT L&H - : Dncrease VOUT L&H s : Read Status Registser e : Enable Buck Boost d : Disable Buck Boost
Dependencies: USBDevice max32630fthr max77801 mbed
main.cpp
00001 /********************************************************************** 00002 * Copyright (C) 2017 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 **********************************************************************/ 00032 00033 00034 #include "mbed.h" 00035 #include "max77801.h" 00036 #include "USBSerial.h" 00037 00038 // Virtual serial port over USB 00039 USBSerial microUSB; 00040 00041 int main() 00042 { 00043 int c; 00044 int32_t rData; 00045 double vout_l = 3.3000; 00046 double vout_h = 3.7500; 00047 00048 DigitalOut rLED(LED1, LED_OFF); 00049 DigitalOut gLED(LED2, LED_OFF); 00050 DigitalOut bLED(LED3, LED_OFF); 00051 00052 I2C i2cBus(P5_7, P6_0); 00053 i2cBus.frequency(400000); 00054 00055 MAX77801 max77801(&i2cBus); 00056 wait_ms(100); 00057 00058 rData = max77801.init(); 00059 if(rData<0) 00060 { 00061 microUSB.printf("MAX77801 Fail to Init. Stopped\r\n"); 00062 rLED = true; 00063 } 00064 else 00065 { 00066 microUSB.printf("MAX77801 Init Done\r\n"); 00067 } 00068 00069 while(1) 00070 { 00071 gLED = true;rLED = false; 00072 microUSB.printf("----- [ MAX77801 DEMO ] Usage ----- \r\n"); 00073 microUSB.printf("i : Set Registers to POR Staus \r\n"); 00074 microUSB.printf("+ : Increase VOUT L&H \r\n"); 00075 microUSB.printf("- : Dncrease VOUT L&H \r\n"); 00076 microUSB.printf("s : Read Status Registser \r\n"); 00077 microUSB.printf("e : Enable Buck Boost \r\n"); 00078 microUSB.printf("d : Disable Buck Boost \r\n"); 00079 microUSB.printf("\r\nInput >> "); 00080 00081 c = microUSB.getc(); 00082 00083 gLED = false;rLED = true; 00084 switch(c) 00085 { 00086 // ReInit 00087 case 'i': 00088 microUSB.printf("Init to POR Status\r\n"); 00089 //Set to POR Status 00090 max77801.init(); 00091 vout_l = 3.3000; 00092 rData = max77801.write_register(MAX77801::REG_VOUT_DVS_L, 0x38); 00093 if(rData < 0) 00094 microUSB.printf("Error: to access data\r\n"); 00095 vout_h = 3.7500; //WLP 00096 rData = max77801.write_register(MAX77801::REG_VOUT_DVS_H, 0x40); 00097 if(rData < 0) 00098 microUSB.printf("Error: to access data\r\n"); 00099 break; 00100 00101 //Control VOUT 00102 case '+': 00103 microUSB.printf("Increase VOUT\r\n"); 00104 vout_l += 0.0125; 00105 rData = max77801.set_vout(vout_l, MAX77801::VAL_LOW); 00106 if(rData < 0) 00107 microUSB.printf("Error: to access data\r\n"); 00108 vout_h += 0.0125; 00109 rData = max77801.set_vout(vout_h, MAX77801::VAL_HIGH); 00110 if(rData < 0) 00111 microUSB.printf("Error: to access data\r\n"); 00112 break; 00113 00114 case '-': 00115 microUSB.printf("Decrease VOUT\r\n"); 00116 vout_l -= 0.0125; 00117 rData = max77801.set_vout(vout_l, MAX77801::VAL_LOW); 00118 if(rData < 0) 00119 microUSB.printf("Error: to access data\r\n"); 00120 vout_h -= 0.0125; 00121 rData = max77801.set_vout(vout_h, MAX77801::VAL_HIGH); 00122 if(rData < 0) 00123 microUSB.printf("Error: to access data\r\n"); 00124 break; 00125 00126 //Read Status Register 00127 case 's': 00128 microUSB.printf("Read Status\r\n"); 00129 rData = max77801.get_status(); 00130 if(rData < 0) 00131 microUSB.printf("Error: to access data\r\n"); 00132 else 00133 microUSB.printf("Status 0x%2X\r\n",rData); 00134 break; 00135 00136 //Buck Boost Enable/Disable 00137 case 'e': 00138 microUSB.printf("Enable Buck Boost\r\n"); 00139 rData = max77801.config_enable(MAX77801::BUCK_BOOST_OUTPUT, 00140 MAX77801::VAL_ENABLE); 00141 if(rData < 0) 00142 microUSB.printf("Error: to access data\r\n"); 00143 break; 00144 00145 case 'd': 00146 microUSB.printf("Disable Buck Boost\r\n"); 00147 rData = max77801.config_enable(MAX77801::BUCK_BOOST_OUTPUT, 00148 MAX77801::VAL_DISABLE); 00149 if(rData < 0) 00150 microUSB.printf("Error: to access data\r\n"); 00151 break; 00152 00153 00154 default: 00155 microUSB.printf("Unknown Command\r\n"); 00156 break; 00157 } 00158 00159 wait_ms(1000); 00160 } 00161 }
Generated on Thu Jul 14 2022 17:42:09 by
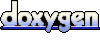