
Use the TLV320 with in-built I2S object to stream audio data from an SD Card and send it to the TLV320 CODEC for audio playback
Dependencies: I2SSlave mbed TLV320
main.cpp
00001 #include "mbed.h" 00002 #include "SDHCFileSystem.h" 00003 #include "TLV320.h" 00004 00005 TLV320 audio(p9, p10, 52, p5, p6, p7, p8, p29); //TLV320 object 00006 SDFileSystem sd(p11, p12, p13, p14, "sd"); //SD Card object 00007 InterruptIn volumeSet(p17); 00008 AnalogIn aIn(p19); 00009 FILE *infp; //File pointer object 00010 /* Buffers */ 00011 int circularBuffer[4096]; 00012 volatile int readPointer = 0; 00013 volatile int theta = 0; 00014 /* Wav file header data, for setting up the transfer protocol */ 00015 short channels; 00016 long sampleRate; 00017 short wordWidth; 00018 /* Function to set volume*/ 00019 void setVolume(void){ 00020 audio.outputVolume(aIn, aIn); 00021 } 00022 /* Function to read from circular buffer and send data to TLV320 */ 00023 void play(void){ 00024 audio.write(circularBuffer, readPointer, 8); 00025 //Pointer fun :-) 00026 readPointer += 8; 00027 readPointer &= 0xfff; 00028 theta -= 8; 00029 } 00030 /* Function to load circular buffer from SD Card */ 00031 void fillBuffer(void){ 00032 while(!feof(infp)){ //fill the circular buffer until the end of the file 00033 static volatile int writePointer = 0; 00034 if(theta < 4096){ 00035 fread(&circularBuffer[writePointer], 4, 4, infp); //read 4 integers into the circular buffer at a time 00036 //More pointer fun :D 00037 theta+=4; 00038 writePointer+=4; 00039 writePointer &= 0xfff; 00040 } 00041 } 00042 } 00043 /* main */ 00044 int main(){ 00045 infp = fopen("/sd/test.wav", "r"); //open file 00046 if(infp == NULL){ //make sure it's been opened 00047 perror("Error opening file!"); 00048 exit(1); 00049 } 00050 /* Parse wav file header */ 00051 fseek(infp, 22, SEEK_SET); 00052 fread(&channels, 2, 1, infp); 00053 fseek(infp, 24, SEEK_SET); 00054 fread(&sampleRate, 4, 1, infp); 00055 fseek(infp, 34, SEEK_SET); 00056 fread(&wordWidth, 2, 1, infp); 00057 00058 volumeSet.rise(&setVolume); //attach set volume function to digital input 00059 audio.power(0x07); //power up TLV apart from analogue input 00060 audio.frequency(sampleRate); //set sample frequency 00061 audio.format(wordWidth, (2-channels)); //set transfer protocol 00062 audio.attach(&play); //attach interrupt handler to send data to TLV320 00063 for(int j = 0; j < 4096; ++j){ //upon interrupt generation 00064 circularBuffer[j] = 0; //clear circular buffer 00065 } 00066 audio.start(TRANSMIT); //interrupt come from the I2STXFIFO only 00067 fillBuffer(); //continually fill circular buffer 00068 }
Generated on Tue Jul 12 2022 23:46:06 by
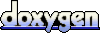