
A program to read out the FTSE100 in real time using a TLV320 CODEC and Ethernet connexion
Dependencies: EthernetNetIf I2SSlave mbed TLV320
FTSE100.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "HTTPClient.h" 00004 #include "SDHCFileSystem.h" 00005 #include "TLV320.h" 00006 00007 EthernetNetIf eth; //Ethernet object 00008 HTTPClient http; //HTTP object 00009 TLV320 audio(p9, p10, 52, p5, p6, p7, p8, p29); //TLV320 object 00010 SDFileSystem sd(p11, p12, p13, p14, "sd"); //SD Card object 00011 InterruptIn volumeSet(p17); //Pin to set volume 00012 AnalogIn aIn(p19); //Volume control 00013 FILE *infp; //File pointer object 00014 /* Useful buffers and pointers*/ 00015 int loadBufferA[512]; 00016 volatile int readPointer = 0; 00017 volatile int theta = 0; 00018 /* Function to control volume */ 00019 void setVolume(void){ 00020 audio.outputVolume(aIn, aIn); //this way we can forget about ADC fluctuations 00021 } 00022 /* Function to send data to TLV320 */ 00023 void play(void){ 00024 audio.write(loadBufferA, readPointer, 8); 00025 /* Some pointers */ 00026 readPointer += 8; 00027 readPointer &= 0x1ff; 00028 theta -= 8; 00029 } 00030 /* Function to load buffer from SD Card */ 00031 void fillBuffer(FILE *file){ 00032 fseek(file, 44, SEEK_SET); //skip the header file, to where the data starts 00033 while(!feof(file)){ 00034 static volatile int writePointer = 0; 00035 if(theta < 512){ 00036 loadBufferA[writePointer] = ((char)(fgetc(file))|((char)fgetc(file)<<8)|((char)fgetc(file)<<16)|((char)fgetc(file)<<24)); 00037 /* More pointer fun */ 00038 ++theta; 00039 ++writePointer; 00040 writePointer &= 0x1ff; 00041 } 00042 } 00043 } 00044 int main() { 00045 /* Set up audio */ 00046 for(int j = 0; j < 512; ++j){ 00047 loadBufferA[j] = 0; 00048 } 00049 volumeSet.rise(&setVolume); 00050 audio.power(0x07); 00051 audio.attach(&play); 00052 /* Setup ethernet connection */ 00053 EthernetErr ethErr = eth.setup(); 00054 if(ethErr) return -1; 00055 /* Instantiate HTTPStream object */ 00056 HTTPText txt; 00057 while(1){ 00058 char buf[10]; 00059 HTTPResult r = http.get("http://www.srcf.ucam.org/~dew35/mbed/v1/dispBoBApp.php", &txt); //load page into buffer 00060 if(r==HTTP_OK){ 00061 string str = txt.gets(); //load web page into string 00062 str.copy(buf,9,0); //load buffer with chunk of web page 00063 for(int k = 0; k < 10; ++k){ //transform data such that the number corresponds to the correctly labelled file 00064 if(buf[k] == 0x2E) buf[k] = 10; 00065 else buf[k] -= 0x30; 00066 } 00067 } 00068 /* Play intro */ 00069 infp = fopen("/sd/11.wav", "r"); 00070 audio.start(TRANSMIT); 00071 fillBuffer(infp); 00072 audio.stop(); 00073 fclose(infp); 00074 /* Play a file for each number in FTSE100 index */ 00075 for(int j = 0; j < 7; ++j){ 00076 char strBuf[20]; 00077 sprintf(strBuf, "/sd/%d.wav", buf[j]); //create filename based on index 00078 infp = fopen(strBuf, "r"); //open said file 00079 audio.start(TRANSMIT); //play said file 00080 fillBuffer(infp); 00081 audio.stop(); 00082 fclose(infp); 00083 00084 } 00085 } 00086 } 00087
Generated on Thu Jul 14 2022 10:21:58 by
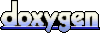