
Measure the width (time-wise) of an incoming pulse.
Dependencies: dispBoB mbed PCA9635
counter.cpp
00001 #include "mbed.h" 00002 #include "dispBoB.h" 00003 00004 dispBoB db(p28, p27, p26); //instantiate a dispBoB object 00005 InterruptIn trigger(p12); //set up the trigger as an external interrupt 00006 Timer t; 00007 00008 void up(){ //call this on rising edge 00009 t.start(); //start timer 00010 } 00011 00012 void down(){ //call this upon falling edge 00013 t.stop(); //stop timer 00014 db.locate(0); 00015 db.printf("%06d", t.read_ms()); //print counter info to dispBoB 00016 t.reset(); //reset timer 00017 } 00018 00019 int main() { 00020 trigger.mode(PullUp); //activate internal pull up (hardware specific) 00021 db.init(); //ALWAYS initialise dispBoB 00022 db.cls(); 00023 trigger.rise(&up); //attach up() to interrupt on rising edge of trigger 00024 trigger.fall(&down); //attach down() to interrupt on falling edge of trigger 00025 db.printf("%06d", t.read_ms()); //display an initial count "000000" 00026 00027 //To change the timebase just replace the read_ms() function with 00028 //read() for seconds and read_us() for microseconds. These use a 32bit 00029 //int microsecond counter, so have a max time of ~30mins 00030 }
Generated on Wed Jul 13 2022 07:33:40 by
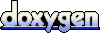