
Measure the time interval between two consecutive rising edges
Dependencies: dispBoB mbed PCA9635
intervalMeasure.cpp
00001 #include "mbed.h" 00002 #include "dispBoB.h" 00003 00004 dispBoB db(p28, p27, p26); //instantiate a dispBoB object 00005 InterruptIn trigger(p12); //set up the trigger as an external interrupt 00006 Timer t; 00007 00008 bool b = false; 00009 00010 void trig(){ //function to call upon interrupt 00011 db.locate(0); 00012 db.printf("%06d", t.read_ms()); //print current time reading 00013 if(b == false){ 00014 t.start(); //start timer 00015 b = true; 00016 } else { 00017 t.stop(); //stop timer 00018 db.printf("06d", t.read_ms()); //print out stopwatch time in milliseconds 00019 t.reset(); //reset and restart timer 00020 t.start(); 00021 } 00022 } 00023 00024 int main() { 00025 trigger.mode(PullUp); //activate internal pull up (hardware specific) 00026 db.init(); //ALWAYS initialise the dispBoB 00027 db.cls(); 00028 trigger.rise(&trig); //attach trig() to interrupt on rising edge of trigger 00029 00030 db.printf("%06d", t.read_ms()); //print initial time reading (000000) 00031 00032 //To change the timebase just replace the read_ms() function with 00033 //read() for seconds and read_us() for microseconds. These use a 32bit 00034 //int microsecond counter, so have a max time of ~30mins 00035 }
Generated on Wed Jul 13 2022 07:33:39 by
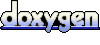