6 x 7 segment display library for PCA9637 driven breakout board
Dependents: FTSE100 InternetDispBoB digitalThermometer Counter ... more
dispBoB.cpp
00001 //NXP PCA9635 library 00002 //mbed Team - 28th June 2011 00003 //Daniel Worrall 00004 00005 #include "mbed.h" 00006 #include "PCA9635.h" 00007 #include "dispBoB.h" 00008 00009 00010 static const char loc[] = {0x02, 0x02, 0x04, 0x04, 0x10, 0x10, 0x12, 0x12}; 00011 static const short dispL[] = { 00012 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00013 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00014 0x0000, 0x1008, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0002, 0xC006, 0x600C, 0x0000, 0x0000, 0x0000, 0x0001, 0x1000, 0x0000, 00015 0xE00E, 0x2008, 0xC00D, 0x600D, 0x200B, 0x6007, 0xE007, 0x200E, 0xE00F, 0x600F, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00016 00017 0x0000, 0xE00D, 0xE003, 0xC001, 0xE009, 0xC007, 0x8007, 0x600F, 0xA003, 0x8000, 0x6008, 0xA007, 0xC002, 0xA005, 0xA001, 0xE001, 00018 0x800F, 0x200F, 0x8001, 0x6007, 0xC003, 0xE000, 0xE000, 0xE004, 0xA00B, 0x600B, 0xC00D, 0xC006, 0x0000, 0x600C, 0x0000, 0x4000, 00019 0x1000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00020 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0xC006, 0x0000, 0x600C, 0x0000, 0x0000 00021 }; 00022 static const short dispR[] = { 00023 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00024 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00025 0x0000, 0x0180, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0020, 0x0C60, 0x06C0, 0x0000, 0x0000, 0x0000, 0x0010, 0x0100, 0x0000, 00026 0x0EE0, 0x0280, 0x0CD0, 0x06D0, 0x02B0, 0x0670, 0x0E70, 0x02E0, 0x0EF0, 0x06F0, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00027 00028 0x0000, 0x0ED0, 0x0E30, 0x0C10, 0x0E90, 0x0C70, 0x0870, 0x06F0, 0x0A30, 0x0800, 0x0680, 0x0A70, 0x0C20, 0x0A50, 0x0A10, 0x0E10, 00029 0x08F0, 0x02F0, 0x0810, 0x0670, 0x0C30, 0x0E00, 0x0E00, 0x0E40, 0x0AB0, 0x06B0, 0x0CD0, 0x0C60, 0x0000, 0x06C0, 0x0000, 0x0400, 00030 0x0100, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 00031 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0C60, 0x0000, 0x06C0, 0x0000, 0x0000 00032 }; 00033 short element[8]; 00034 short ppair[4]; 00035 string nullArray = " "; 00036 00037 dispBoB::dispBoB(PinName sda, PinName scl, PinName en): _pca(sda, scl), _en(en) { 00038 init(); 00039 } 00040 void dispBoB::init(void){ //initiate - call this whenever the system needs to be reset 00041 _en = 0; 00042 _pca.init(loc[0]); 00043 _pca.init(loc[2]); 00044 _pca.init(loc[4]); 00045 _pca.init(loc[6]); 00046 } 00047 00048 void dispBoB::cls(void){ //clear screen and screen buffers 00049 for(int j = 0; j < 8; j++){ 00050 locate(j); 00051 element[j] = 0x0000; 00052 _pca.bus(0x0000); 00053 } 00054 locate(0); 00055 } 00056 00057 void dispBoB::locate(char pos){ 00058 _pca.setAddress(loc[pos]); 00059 _cursor = pos; 00060 } 00061 00062 int dispBoB::_putc(int c){ 00063 if(_cursor>6){ 00064 _cursor++; 00065 return -1; //skip if exceeds display size allow extra '.' in 6th >> to place in 5th 00066 } 00067 00068 if(c=='.'){ //choose not to print a '.' outside the display bounds 00069 if(_cursor>6){ 00070 _cursor++; 00071 return -1; 00072 } 00073 if((_cursor==0)&&((element[_cursor]&0x1000)==0x1000)) element[_cursor] = 0x0000; 00074 if(_cursor!=0) _cursor--; 00075 if(((element[_cursor]&0x1000)==0x1000)||((element[_cursor]&0x0100)==0x0100)){ 00076 _cursor++; 00077 element[_cursor]=0x0000; 00078 } 00079 } else { 00080 if(_cursor>5){ //for non '.' chars at end of line, flag up overflow and don't print 00081 _cursor++; 00082 return -1; 00083 } 00084 element[_cursor]=0x0000; //reset an element before pasting a char other than '.' 00085 } 00086 00087 if(_cursor & 1){ //look up char >> element conversion and paste to display 00088 element[_cursor] |= dispR[toupper(c)]; 00089 } else { 00090 element[_cursor] |= dispL[toupper(c)]; 00091 } 00092 00093 int i = _cursor/2; 00094 ppair[i] = element[(2*i)] + element[(2*i)+1]; //combine left and right elements into a form readable by the PCA9635 00095 00096 _pca.setAddress(loc[_cursor]); //set location to paste to 00097 bus(ppair[i]); //sned data tp PCA9635 00098 00099 locate(_cursor+1); //allow cursor to be in element[6], decision of what to do 00100 //is made at the start of the putc() algo. depending on char. 00101 return c; 00102 } 00103 00104 int dispBoB::_getc(){ 00105 return -1; 00106 } 00107 00108 void dispBoB::scroll(string str, float speed){ 00109 char buffer[12]; //instantiate a 12 element buffer array 00110 str.insert(0, nullArray); //paste 6 zeros at either end of string 00111 str.insert(str.length(), nullArray); 00112 00113 for(int k = 0; k < str.length(); k++){ 00114 str.copy(buffer, 12, k); //mask a 12 element buffer starting at k 00115 locate(0); //locate to position 0 00116 printf("%s", buffer); //print buffer to display 00117 if((element[0]&0x1000)==0x1000){ //if position zero has two characters i.e. char + '.' 00118 if(element[0]!=0x1000)k++; //then treat as one 00119 else; 00120 } 00121 wait(speed); //hold frame for duration of speed x milliseconds 00122 } 00123 } 00124 00125 //supply PCA9635 functionality to dispBoB class 00126 void dispBoB::bus(short leds){ 00127 _pca.bus(leds); 00128 } 00129 00130 00131 00132 00133 00134 00135 00136 00137
Generated on Wed Jul 13 2022 07:10:58 by
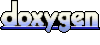