Last version of TinyGPS library
Dependents: Nucleo_gps mbed_2018 mbed_2019_rx3 mbed_2019 ... more
TinyGPS.h
00001 /* 00002 TinyGPS - a small GPS library for Arduino providing basic NMEA parsing 00003 Based on work by and "distance_to" and "course_to" courtesy of Maarten Lamers. 00004 Suggestion to add satellites(), course_to(), and cardinal(), by Matt Monson. 00005 Precision improvements suggested by Wayne Holder. 00006 Copyright (C) 2008-2013 Mikal Hart 00007 All rights reserved. 00008 00009 This library is free software; you can redistribute it and/or 00010 modify it under the terms of the GNU Lesser General Public 00011 License as published by the Free Software Foundation; either 00012 version 2.1 of the License, or (at your option) any later version. 00013 00014 This library is distributed in the hope that it will be useful, 00015 but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00017 Lesser General Public License for more details. 00018 00019 You should have received a copy of the GNU Lesser General Public 00020 License along with this library; if not, write to the Free Software 00021 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00022 */ 00023 00024 #ifndef TinyGPS_h 00025 #define TinyGPS_h 00026 00027 /*#if defined(ARDUINO) && ARDUINO >= 100 00028 #include "Arduino.h" 00029 #else 00030 #include "WProgram.h" 00031 #endif 00032 */ 00033 00034 00035 #include "mbed.h" 00036 #include "types.h" 00037 00038 #include <stdlib.h> 00039 00040 #define _GPS_VERSION 13 // software version of this library 00041 #define _GPS_MPH_PER_KNOT 1.15077945 00042 #define _GPS_MPS_PER_KNOT 0.51444444 00043 #define _GPS_KMPH_PER_KNOT 1.852 00044 #define _GPS_MILES_PER_METER 0.00062137112 00045 #define _GPS_KM_PER_METER 0.001 00046 // #define _GPS_NO_STATS 00047 00048 #define TWO_PI 6.283185 00049 00050 //Convert from radians to degrees. 00051 #define toDegrees(x) (x * 57.2957795) 00052 //Convert from degrees to radians. 00053 #define toRadians(x) (x * 0.01745329252) 00054 00055 class TinyGPS 00056 { 00057 public: 00058 enum { 00059 GPS_INVALID_AGE = 0xFFFFFFFF, GPS_INVALID_ANGLE = 999999999, 00060 GPS_INVALID_ALTITUDE = 999999999, GPS_INVALID_DATE = 0, 00061 GPS_INVALID_TIME = 0xFFFFFFFF, GPS_INVALID_SPEED = 999999999, 00062 GPS_INVALID_FIX_TIME = 0xFFFFFFFF, GPS_INVALID_SATELLITES = 0xFF, 00063 GPS_INVALID_HDOP = 0xFFFFFFFF 00064 }; 00065 00066 static const float GPS_INVALID_F_ANGLE, GPS_INVALID_F_ALTITUDE, GPS_INVALID_F_SPEED; 00067 00068 TinyGPS(); 00069 bool encode(char c); // process one character received from GPS 00070 TinyGPS &operator << (char c) {encode(c); return *this;} 00071 00072 // lat/long in MILLIONTHs of a degree and age of fix in milliseconds 00073 // (note: versions 12 and earlier gave lat/long in 100,000ths of a degree. 00074 void get_position(long *latitude, long *longitude, unsigned long *fix_age = 0); 00075 00076 // date as ddmmyy, time as hhmmsscc, and age in milliseconds 00077 void get_datetime(unsigned long *date, unsigned long *time, unsigned long *age = 0); 00078 00079 // signed altitude in centimeters (from GPGGA sentence) 00080 inline long altitude() { return _altitude; } 00081 00082 // course in last full GPRMC sentence in 100th of a degree 00083 inline unsigned long course() { return _course; } 00084 00085 // speed in last full GPRMC sentence in 100ths of a knot 00086 inline unsigned long speed() { return _speed; } 00087 00088 // satellites used in last full GPGGA sentence 00089 inline unsigned short satellites() { return _numsats; } 00090 00091 // horizontal dilution of precision in 100ths 00092 inline unsigned long hdop() { return _hdop; } 00093 00094 void f_get_position(float *latitude, float *longitude, unsigned long *fix_age = 0); 00095 void crack_datetime(int *year, byte *month, byte *day, 00096 byte *hour, byte *minute, byte *second, byte *hundredths = 0, unsigned long *fix_age = 0); 00097 float f_altitude(); 00098 float f_course(); 00099 float f_speed_knots(); 00100 float f_speed_mph(); 00101 float f_speed_mps(); 00102 float f_speed_kmph(); 00103 00104 static int library_version() { return _GPS_VERSION; } 00105 00106 static float distance_between (float lat1, float long1, float lat2, float long2); 00107 static float course_to (float lat1, float long1, float lat2, float long2); 00108 static const char *cardinal(float course); 00109 00110 #ifndef _GPS_NO_STATS 00111 void stats(unsigned long *chars, unsigned short *good_sentences, unsigned short *failed_cs); 00112 #endif 00113 00114 private: 00115 enum {_GPS_SENTENCE_GPGGA, _GPS_SENTENCE_GPRMC, _GPS_SENTENCE_OTHER}; 00116 00117 // properties 00118 unsigned long _time, _new_time; 00119 unsigned long _date, _new_date; 00120 long _latitude, _new_latitude; 00121 long _longitude, _new_longitude; 00122 long _altitude, _new_altitude; 00123 unsigned long _speed, _new_speed; 00124 unsigned long _course, _new_course; 00125 unsigned long _hdop, _new_hdop; 00126 unsigned short _numsats, _new_numsats; 00127 00128 unsigned long _last_time_fix, _new_time_fix; 00129 unsigned long _last_position_fix, _new_position_fix; 00130 00131 // parsing state variables 00132 byte _parity; 00133 bool _is_checksum_term; 00134 char _term[15]; 00135 byte _sentence_type; 00136 byte _term_number; 00137 byte _term_offset; 00138 bool _gps_data_good; 00139 00140 #ifndef _GPS_NO_STATS 00141 // statistics 00142 unsigned long _encoded_characters; 00143 unsigned short _good_sentences; 00144 unsigned short _failed_checksum; 00145 unsigned short _passed_checksum; 00146 #endif 00147 00148 // internal utilities 00149 int from_hex(char a); 00150 unsigned long parse_decimal(); 00151 unsigned long parse_degrees(); 00152 bool term_complete(); 00153 bool gpsisdigit(char c) { return c >= '0' && c <= '9'; } 00154 long gpsatol(const char *str); 00155 int gpsstrcmp(const char *str1, const char *str2); 00156 }; 00157 00158 #if !defined(ARDUINO) 00159 // Arduino 0012 workaround 00160 #undef int 00161 #undef char 00162 #undef long 00163 #undef byte 00164 #undef float 00165 #undef abs 00166 #undef round 00167 #endif 00168 00169 #endif
Generated on Thu Jul 21 2022 10:30:43 by
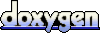