Generalized adaptation of the WiiChuk_compat library.
Fork of WiiChuk_compat by
WiiChuck.h
00001 /* 00002 * Copyright (c) 2011 Greg Brush 00003 * Portions copyright (C) 2014 Clark Scheff 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 * 00023 * This code based on: 00024 * WiiChuck 00025 * http://mbed.org/users/FrankWeissenborn/libraries/WiiChuck/lnae1a 00026 * 2011-03-14 00027 * New initialization compatible with 3rd party controllers 00028 * 00029 */ 00030 00031 #ifndef __WIICHUCK_H 00032 #define __WIICHUCK_H 00033 00034 #include "mbed.h" 00035 00036 #define NUNCHUCK_ADDR 0xA4 00037 #define NUNCHUCK_READLEN 0x06 00038 #define I2C_ACK 0 00039 #define I2C_READ_DELAY 0.0001 00040 #define I2C_DEFAULT_FREQUENCY 400000 00041 00042 #define JOY_X_IDX 0 00043 #define JOY_Y_IDX 1 00044 #define ACC_X_IDX 2 00045 #define ACC_Y_IDX 3 00046 #define ACC_Z_IDX 4 00047 #define BUTTON_IDX 5 00048 00049 /** 00050 * @struct nunchuck_data_s 00051 * @brief Data structure used for holding nunchuck data 00052 * @var joyX Single byte indicating the position of the joystick in the X axis 00053 * @var joyY Single byte indicating the position of the joystick in the Y axis 00054 * @var accX 10-bit value representing the acceleration in the X axis 00055 * @var accY 10-bit value representing the acceleration in the Y axis 00056 * @var accZ 10-bit value representing the acceleration in the Z axis 00057 * @var buttonC Pressed state of the C button 00058 * @var buttonZ Pressed state of the Z button 00059 */ 00060 typedef struct nunchuck_data_s { 00061 uint8_t joyX; 00062 uint8_t joyY; 00063 uint16_t accX; 00064 uint16_t accY; 00065 uint16_t accZ; 00066 bool buttonC; 00067 bool buttonZ; 00068 } nunchuck_data_t; 00069 00070 /** 00071 * @typedef pt2Func Callback function typedef 00072 */ 00073 typedef void(*pt2Func)(nunchuck_data_t*); 00074 00075 /** 00076 * Class for interfacing with a Wii Nunchuck (including knock offs) 00077 * 00078 * Example: 00079 * @code 00080 * // Continuously read data from the nunchuck 00081 * #include "mbed.h" 00082 * #include "WiiChuck.h" 00083 * 00084 * WiiChuck nunchuck(PB_9, PB_8); 00085 * 00086 * int main() { 00087 * nunchuck_data_t nunchuckData; 00088 * 00089 * printf("joyX\tjoyY\taccX\taccY\taccZ\tbuttC\tbuttD\r\n"); 00090 * while(1) { 00091 * nunchuck.read(&nunchuckData); 00092 * printf("%d\t%d\t%d\t%d\t%d\t%s\t%s\r\n", 00093 * nunchuckData.joyX, nunchuckData.joyY, nunchuckData.accX, nunchuckData.accY, 00094 * nunchuckData.accZ, nunchuckData.buttonC ? "X" : "", nunchuckData.buttonZ ? "X" : ""); 00095 * } 00096 * } 00097 * @endcode 00098 */ 00099 class WiiChuck { 00100 public: 00101 /** 00102 * @param data I2C data pin 00103 * @param clk I2C clock pin 00104 */ 00105 WiiChuck (PinName data, PinName clk, uint32_t i2cFrequency=I2C_DEFAULT_FREQUENCY); 00106 00107 /** 00108 * Reads a packet of data from the nunchuck and fills in the supplied data structure. 00109 * 00110 * @param data Pointer to the data that will be filled in. 00111 */ 00112 bool read(nunchuck_data_t* data); 00113 00114 /** 00115 * Attach a callback function that can be called when new data is available. 00116 * 00117 * @param callback Pointer to function to be called when data has been read 00118 * @param readInterval Time interval in seconds to perform successive reads from the nunchuck 00119 */ 00120 void attach(pt2Func callback, float readInterval); 00121 00122 /** 00123 * Detach the callback and stop polling the nunchuck 00124 */ 00125 void detach(); 00126 00127 /** 00128 * Gets the value for the x and y position of the joystick when it is in the neutral position. 00129 * 00130 * @param centerX Pointer to a uint8_t that will hold the center x value 00131 * @param centerY Pointer to a uint8_t that will hold the center y value 00132 */ 00133 bool calibrateJoystickNeutralPosition(uint8_t* centerX, uint8_t* centerY); 00134 00135 private: 00136 void getValues(); 00137 00138 I2C _i2c; 00139 pt2Func _callback; 00140 Ticker _getValues; 00141 bool _initialized; 00142 nunchuck_data_t _data; 00143 }; 00144 00145 #endif
Generated on Tue Jul 19 2022 06:33:31 by
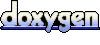