Generalized adaptation of the WiiChuk_compat library.
Fork of WiiChuk_compat by
WiiChuck Class Reference
Class for interfacing with a Wii Nunchuck (including knock offs) More...
#include <WiiChuck.h>
Public Member Functions | |
WiiChuck (PinName data, PinName clk, uint32_t i2cFrequency=I2C_DEFAULT_FREQUENCY) | |
bool | read (nunchuck_data_t *data) |
Reads a packet of data from the nunchuck and fills in the supplied data structure. | |
void | attach (pt2Func callback, float readInterval) |
Attach a callback function that can be called when new data is available. | |
void | detach () |
Detach the callback and stop polling the nunchuck. | |
bool | calibrateJoystickNeutralPosition (uint8_t *centerX, uint8_t *centerY) |
Gets the value for the x and y position of the joystick when it is in the neutral position. |
Detailed Description
Class for interfacing with a Wii Nunchuck (including knock offs)
Example:
// Continuously read data from the nunchuck #include "mbed.h" #include "WiiChuck.h" WiiChuck nunchuck(PB_9, PB_8); int main() { nunchuck_data_t nunchuckData; printf("joyX\tjoyY\taccX\taccY\taccZ\tbuttC\tbuttD\r\n"); while(1) { nunchuck.read(&nunchuckData); printf("%d\t%d\t%d\t%d\t%d\t%s\t%s\r\n", nunchuckData.joyX, nunchuckData.joyY, nunchuckData.accX, nunchuckData.accY, nunchuckData.accZ, nunchuckData.buttonC ? "X" : "", nunchuckData.buttonZ ? "X" : ""); } }
Definition at line 99 of file WiiChuck.h.
Constructor & Destructor Documentation
WiiChuck | ( | PinName | data, |
PinName | clk, | ||
uint32_t | i2cFrequency = I2C_DEFAULT_FREQUENCY |
||
) |
- Parameters:
-
data I2C data pin clk I2C clock pin
Definition at line 3 of file WiiChuck.cpp.
Member Function Documentation
void attach | ( | pt2Func | callback, |
float | readInterval | ||
) |
Attach a callback function that can be called when new data is available.
- Parameters:
-
callback Pointer to function to be called when data has been read readInterval Time interval in seconds to perform successive reads from the nunchuck
Definition at line 46 of file WiiChuck.cpp.
bool calibrateJoystickNeutralPosition | ( | uint8_t * | centerX, |
uint8_t * | centerY | ||
) |
Gets the value for the x and y position of the joystick when it is in the neutral position.
- Parameters:
-
centerX Pointer to a uint8_t that will hold the center x value centerY Pointer to a uint8_t that will hold the center y value
Definition at line 56 of file WiiChuck.cpp.
void detach | ( | ) |
Detach the callback and stop polling the nunchuck.
Definition at line 51 of file WiiChuck.cpp.
bool read | ( | nunchuck_data_t * | data ) |
Reads a packet of data from the nunchuck and fills in the supplied data structure.
- Parameters:
-
data Pointer to the data that will be filled in.
Definition at line 20 of file WiiChuck.cpp.
Generated on Tue Jul 19 2022 06:33:31 by
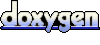