
WappAutomation 2 : - WITH RESET - WITHOUT Switch Cable Tested on TEL50
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "MFRC522.h" 00003 #include "EthernetInterface.h" 00004 00005 00006 //***************************** Change this for each new prober installation ******************************** 00007 #define PROBER_NAME "tl50" 00008 #define IP "10.129.97.130" 00009 /*Please update the list below: 00010 TEL12 : 10.129.97.100 00011 TEL20 : 10.129.97.101 00012 TEL23 : 10.129.97.102 00013 TEL36 : 10.129.97.103 00014 TEL01 : 10.129.97.105 00015 TEL19 : 10.129.97.106 00016 TEL27 : 10.129.97.107 00017 TEL37 : 10.129.97.108 00018 TEL38 : 10.129.97.109 00019 TEL39 : 10.129.97.110 00020 TEL40 : 10.129.97.111 00021 TEL16 : 10.129.97.112 00022 TEL21 : 10.129.97.113 00023 TEL22 : 10.129.97.114 00024 TEL34 : 10.129.97.115 00025 TEL30 : 10.129.97.116 00026 TEL32 : 10.129.97.117 00027 TEL26 : 10.129.97.118 00028 TEL25 : 10.129.97.119 00029 TEL28 : 10.129.97.120 00030 TEL29 : 10.129.97.121 00031 TEL31 : 10.129.97.122 00032 TEL33 : 10.129.97.123 00033 TEL42 : 10.129.97.124 00034 TEL43 : 10.129.97.125 00035 TEL44 : 10.129.97.126 00036 TEL45 : 10.129.97.127 00037 TEL46 : 10.129.97.128 00038 TEL47 : 10.129.97.129 00039 TEL48 : 10.129.97.104 00040 TEL50 : 10.129.97.130 00041 ************************************************************************************************************/ 00042 00043 00044 //******************************************* RFID Antenna ************************************************** 00045 //Cheap 13.56 Mhz RFID-RC522 module (This code is based on Martin Olejar's MFRC522 library. Minimal changes) 00046 //On Nucleo-F429ZI, SPI1 and SPI2 could not be used in the same time with EthernetInterface -> SPI4 used 00047 //RFID IRQ=pin5 -> Not used. Leave open 00048 //RFID MISO=pin4 -> Nucleo SPI_MISO -> White -> PE_5 00049 //RFID MOSI=pin3 -> Nucleo SPI_MOSI -> Green -> PE_6 00050 //RFID SCK=pin2 -> Nucleo SPI_SCK -> Yellow -> PE_2 00051 //RFID SDA=pin1 -> Nucleo SPI_CS -> Blue -> PE_4 00052 //RFID RST=pin7 -> Nucleo DigitalOut -> Brown -> D8 00053 //*********************************************************************************************************** 00054 00055 00056 //********************************************* Antenna LED ************************************************* 00057 //Anode -> +3.3v pin 00058 //Cathode -> D3 pin 00059 //Use LED_ON and LED_OFF to use the LED 00060 //*********************************************************************************************************** 00061 00062 00063 //****************************************** Interlock Relay ************************************************ 00064 //Power + -> +5v pin 00065 //Power - -> GND pin 00066 //Command -> D4 pin 00067 //Use RELAY_OPEN and RELAY_CLOSE to use the relay 00068 //*********************************************************************************************************** 00069 00070 00071 #define MF_RESET D8 // Nucleo Pin for MFRC522 reset 00072 #define LED_ON 0 00073 #define LED_OFF 1 00074 #define RELAY_CLOSED 0 00075 #define RELAY_OPEN 1 00076 00077 #define INTERLOCK_DELAY 5000 // Delay (in milliseconds) between alarm received and relay off to simulate open interlock 00078 #define APCD_READ_DELAY 500 // Delay (in milliseconds) before read the APCD data 00079 #define SOCKETCONNECTION_ATTEMPT 5 // Number of attempts for socket connection 00080 #define TIMEOUT_WAITTAG 20 // Timeout in case of New Tag Present detected 00081 00082 #define GATEWAY "10.129.97.254" //The gateway to go out the prober VLAN 00083 #define MASK "255.255.255.0" 00084 #define SERVER "10.18.47.90" //Automation server IP Address - { 164, 129, 103, 88 } for Dev Automation server 00085 #define PORT 7778 //Automation port 00086 00087 #define MBX_OPEN "MBX_OPEN srvpat" //"MBX_OPEN srv_pat" 00088 #define MBX_CLOSE "MBX_DISCONNECT" 00089 #define MBX_COUNT "MBX_COUNT STM32Insrv_" PROBER_NAME 00090 #define MBX_GET "MBX_GET 5,STM32Insrv_" PROBER_NAME 00091 #define MBX_PUT_BEGIN "MBX_PUT STM32Outsrv, CMD/A=\"WAPP\" MID/A=\"gnb3t" PROBER_NAME "\" MTY/A=\"E\" WAPPID/A=\"" 00092 #define MBX_PUT_END "\"" 00093 00094 00095 Serial PC(USBTX, USBRX); 00096 MFRC522 Antenna(PE_6, PE_5, PE_2, PE_4, MF_RESET); 00097 EthernetInterface eth; 00098 TCPSocket apcd; 00099 DigitalIn WappExchangeSwitch(D2); 00100 DigitalOut TagReadLED(D3); 00101 DigitalOut Interlock(D4); 00102 DigitalOut red(LED3); 00103 DigitalOut blue(LED2); 00104 DigitalOut green(LED1); 00105 00106 char bufTagID[256]; 00107 int APCDsendData (char *tcpCmd); 00108 char *APCDgetData (); 00109 00110 00111 //IsTagPresent(): function to read the Tag ID 00112 // Return false if no Tag present 00113 // Return true if a Tag ID was read (Tag ID is saved int the bufTagID buffer) 00114 bool IsTagPresent() 00115 { 00116 /* 00117 if (Antenna.PICC_IsNewCardPresent() && WappExchangeSwitch == 1){ 00118 PC.printf("TAG detected ... "); 00119 00120 //Select one of the cards 00121 int count = 0; 00122 while (!Antenna.PICC_ReadCardSerial() && WappExchangeSwitch == 1 && count < TIMEOUT_WAITTAG){ 00123 count++; 00124 wait_ms(100); 00125 } 00126 if (count == TIMEOUT_WAITTAG){ 00127 PC.printf("Timeout occured -> no Tag present\r\n"); 00128 return false; 00129 } 00130 if (WappExchangeSwitch == 0) return false; 00131 00132 //Light during 1s Tag LED to indicate that a tag was read 00133 TagReadLED = LED_ON; 00134 wait_ms(500); 00135 TagReadLED = LED_OFF; 00136 00137 //Save Tag ID into buffer 00138 PC.printf("ID = "); 00139 int pos = 0; 00140 char hexbuf[2]; 00141 for (int i = 0; i < 256; i++) bufTagID[i] = '\0'; 00142 for (uint8_t i = 0; i < Antenna.uid.size; i++) { 00143 sprintf(hexbuf, "%02x", Antenna.uid.uidByte[i]); 00144 PC.putc(hexbuf[0]); 00145 PC.putc(hexbuf[1]); 00146 bufTagID[pos] = hexbuf[0]; 00147 pos++; 00148 bufTagID[pos] = hexbuf[1]; 00149 pos++; 00150 } 00151 PC.printf("\r\n"); 00152 return true; 00153 } 00154 else return false; 00155 */ 00156 00157 //Look for new cards during 5s 00158 int timeout = 0; 00159 while ( ! Antenna.PICC_IsNewCardPresent() == 1 && timeout < TIMEOUT_WAITTAG) { 00160 wait_ms(100); 00161 timeout++; 00162 } 00163 if (timeout >= TIMEOUT_WAITTAG) { 00164 PC.printf("Timeout occured -> no Tag present\r\n"); 00165 return false; 00166 } 00167 //if (WappExchangeSwitch == 0) return false; 00168 PC.printf("TAG detected ... "); 00169 00170 //pc.printf("Yes, tag is present... Try to read Tag ID\r\n"); 00171 00172 //Select one of the cards 00173 timeout = 0; 00174 while ( ! Antenna.PICC_ReadCardSerial() == 1 && timeout < TIMEOUT_WAITTAG) { 00175 wait_ms(100); 00176 timeout++; 00177 } 00178 if (timeout >= TIMEOUT_WAITTAG) { 00179 PC.printf("Timeout occured during ReadCardSerial\r\n"); 00180 return false; 00181 } 00182 //if (WappExchangeSwitch == 0) return false; 00183 00184 //Light during 1s Tag LED to indicate that a tag was read 00185 TagReadLED = LED_ON; 00186 wait_ms(500); 00187 TagReadLED = LED_OFF; 00188 00189 //Save Tag ID into buffer 00190 PC.printf("ID = "); 00191 int pos = 0; 00192 char hexbuf[2]; 00193 for (int i = 0; i < 256; i++) bufTagID[i] = '\0'; 00194 for (uint8_t i = 0; i < Antenna.uid.size; i++) { 00195 sprintf(hexbuf, "%02x", Antenna.uid.uidByte[i]); 00196 PC.putc(hexbuf[0]); 00197 PC.putc(hexbuf[1]); 00198 bufTagID[pos] = hexbuf[0]; 00199 pos++; 00200 bufTagID[pos] = hexbuf[1]; 00201 pos++; 00202 } 00203 PC.printf("\r\n"); 00204 00205 return true; 00206 } 00207 00208 //APCDGetWappAlarm: function to check from APCD mailbox if a wapp alarm was sent by automation 00209 // return 1 in case of alarm 00210 // return 2 if apcd.connect failed 00211 // return 0 if there is no alarm 00212 int APCDGetWappAlarm() 00213 { 00214 int result = 2; 00215 int rtrn = 2; 00216 int attempt = 0; 00217 00218 //Open a TCP socket 00219 rtrn = apcd.open(ð); 00220 PC.printf("\r\nAPCDGetWappAlarm:\r\n"); 00221 PC.printf("\tOpen socket -> %d\r\n", rtrn); 00222 blue = 1; 00223 00224 //Try APCD connection 00225 rtrn = apcd.connect(SERVER, PORT); 00226 while (rtrn < 0 && attempt < SOCKETCONNECTION_ATTEMPT) { 00227 wait_ms(500); 00228 rtrn = apcd.connect(SERVER, PORT); 00229 attempt++; 00230 } 00231 PC.printf("\tConnection -> %d\r\n", rtrn); 00232 00233 if (rtrn == 0) { //Automation server connection successfully 00234 APCDsendData(MBX_OPEN); //Open the APCD bus 00235 APCDgetData(); 00236 00237 //Get message's number in the mailbox 00238 PC.printf("\tAPCD -> %s\r\n", MBX_COUNT); 00239 APCDsendData(MBX_COUNT); 00240 00241 //Extract the data from the string received 00242 char buf[256]; 00243 sprintf(buf,"%s", APCDgetData()); 00244 unsigned short int answerLen = strlen(buf); 00245 PC.printf("\tAnswer -> %s\r\n", buf); 00246 if (answerLen > 10) { //Answer will be MBX_COUNT,xx where xx is the number of message 00247 int nbMsg = 0; 00248 char nb[10]; 00249 int pos = 10; 00250 while (buf[pos] != '\0' && pos < 20) { 00251 nb[pos-10] = buf[pos]; 00252 pos++; 00253 } 00254 nb[pos-10] = '\0'; 00255 nbMsg = atoi(nb); 00256 PC.printf("\tMsg count -> %d\r\n", nbMsg); 00257 //If there is a message or more, get all message (=> mailbox will be empty) 00258 if (nbMsg > 0) { 00259 result = 1; 00260 while (nbMsg > 0) { //Get all VFEI message in the mailbox 00261 PC.printf("\tAPCD -> %s\r\n", MBX_GET); 00262 APCDsendData(MBX_GET); 00263 APCDgetData(); 00264 nbMsg--; 00265 } 00266 } else result = 0; 00267 } 00268 00269 //Close the APCD bus 00270 APCDsendData(MBX_CLOSE); 00271 PC.printf("\tAPCD -> %s\r\n", MBX_CLOSE); 00272 APCDgetData(); 00273 } else result = 2; 00274 00275 apcd.close(); 00276 blue = 0; 00277 return result; 00278 } 00279 00280 //APCDSendTagID: function to send the VFEI message with TagID to the automation 00281 // return 1 if data sent correctly 00282 // return 2 if apcd.connect failed 00283 // return 0 if sent data lenght doesn't match with message lenght 00284 int APCDSendTagID() 00285 { 00286 int attempt = 0; 00287 int result = 2; 00288 int rtrn = 2; 00289 PC.printf("\r\nAPCDSendTagID:\r\n"); 00290 00291 //Build VFEI message 00292 char concatBuffer[256]; 00293 sprintf(concatBuffer, "%s%s%s\0", MBX_PUT_BEGIN, bufTagID, MBX_PUT_END); 00294 PC.printf("\tVFEI message : %s\r\n", concatBuffer); 00295 00296 //Open a TCP socket 00297 rtrn = apcd.open(ð); 00298 PC.printf("\tOpen socket -> %d\r\n", rtrn); 00299 blue = 1; 00300 00301 //Try APCD connection 00302 rtrn = apcd.connect(SERVER, PORT); 00303 while (rtrn < 0 && attempt < SOCKETCONNECTION_ATTEMPT) { 00304 wait_ms(500); 00305 rtrn = apcd.connect(SERVER, PORT); 00306 attempt++; 00307 } 00308 PC.printf("\tConnection -> %d\r\n", rtrn); 00309 00310 //Send VFEI message if possible 00311 if (rtrn == 0) { 00312 PC.printf("\tAPCD -> %s\r\n", MBX_OPEN); 00313 APCDsendData(MBX_OPEN); //Open the APCD bus 00314 APCDgetData(); 00315 rtrn = APCDsendData(concatBuffer); //Send the VFEI message 00316 APCDgetData(); 00317 PC.printf("\tAPCD -> %s\r\n", MBX_CLOSE); 00318 APCDsendData(MBX_CLOSE); //Close the APCD bus 00319 APCDgetData(); 00320 result = 1; 00321 } else result = 2; 00322 00323 apcd.close(); 00324 blue = 0; 00325 return result; 00326 } 00327 00328 //APCDsendData: function to send data to APCD bus 00329 // Return 1 if data sent correctly, 0 in other case 00330 // Author: F. Frezot from Arduino code 00331 int APCDsendData (char *tcpCmd) 00332 { 00333 unsigned short int dataLength = strlen(tcpCmd); 00334 unsigned char len[2]; 00335 00336 len[0] = ((dataLength>>8)&0xFF); //MSB 00337 len[1] = ((dataLength>>0)&0xFF); //LSB 00338 00339 int rtrnLenght = apcd.send(len, sizeof(len)); 00340 rtrnLenght = apcd.send(tcpCmd, dataLength); 00341 if (rtrnLenght == dataLength) return 1; 00342 else return 0; 00343 } 00344 00345 00346 //APCDgetData: function to read data from APCD bus 00347 // Return a char array with data read 00348 // Author: F. Frezot from Arduino code 00349 char *APCDgetData () 00350 { 00351 char MSB[1]; 00352 char LSB[1]; 00353 unsigned short int dataLength; 00354 char readBuf[256] = "\0"; 00355 00356 wait_ms(APCD_READ_DELAY); 00357 00358 apcd.recv(MSB, 1); 00359 apcd.recv(LSB, 1); 00360 dataLength = ((MSB[0]<<8)&0xFF00)|(LSB[0]<<0)&0x00FF; 00361 apcd.recv(readBuf, dataLength); 00362 00363 return (readBuf); 00364 } 00365 00366 int main() 00367 { 00368 // Init Serial and nucleo board LED (off) 00369 PC.baud(9600); 00370 PC.printf("Running ...\r\n"); 00371 green = 0; 00372 red = 0; 00373 blue = 0; 00374 00375 // Init RC522 Chip 00376 PC.printf("\tInit RFID..."); 00377 wait_ms(500); 00378 Antenna.PCD_Init(); 00379 wait_ms(100); 00380 Antenna.PCD_AntennaOff(); //Turn off the RFID antenna. 00381 TagReadLED = LED_OFF; //Turn off the LED who indicate if a TAG was read 00382 PC.printf("DONE\r\n"); 00383 00384 //PC.printf("\tClose relay for prober Interlock\r\n"); 00385 //Interlock = RELAY_CLOSED; //Close relay for prober interlock 00386 00387 //Ethernet Init 00388 PC.printf("\tInit Ethernet..."); 00389 eth.set_network(IP, MASK, GATEWAY); 00390 int rtrn = eth.connect(); 00391 int reset_eth = 0; 00392 if (rtrn == 0) { 00393 PC.printf("DONE\r\n"); 00394 const char *ip = eth.get_ip_address(); 00395 const char *mac = eth.get_mac_address(); 00396 PC.printf("\tIP address = %s\n\r", ip ? ip : "No IP"); 00397 PC.printf("\tMAC address = %s\n\r", mac ? mac : "No MAC"); 00398 PC.printf("Running DONE\r\n\r\n"); 00399 } else { 00400 PC.printf("FAIL -> Check if Network cable is plug-in then reset the board\r\n"); 00401 red = 0; 00402 green = 0; 00403 PC.printf("Running FAIL -> Program aborted\r\n\r\n"); 00404 while (reset_eth <= 900) { 00405 blue = !blue; 00406 TagReadLED = !TagReadLED; 00407 wait_ms(200); 00408 reset_eth++; 00409 } 00410 NVIC_SystemReset(); // reset system if no ethernet connection succeeded after 180sec Added by B. Tournier 00411 00412 } 00413 00414 //Waiting Tag and WAPP alarm 00415 int count = 0; 00416 int reset_trigger = 0; 00417 while (true) { 00418 //Check if Tag read 00419 //if (true) { 00420 //Turn on the antenna 00421 Antenna.PCD_AntennaOn(); 00422 wait_ms(100); 00423 00424 //Waiting Tag ID 00425 if (IsTagPresent()) { 00426 green = 0; 00427 int rtrn = APCDSendTagID(); 00428 if (rtrn == 1) { 00429 PC.printf("\tTag ID sent correctly (Code=%d)\r\n", rtrn); 00430 red = 0; 00431 //Blink Tag LED during 3s 00432 for (int i = 0; i < 10; i++) { 00433 TagReadLED = !TagReadLED; 00434 wait_ms(500); 00435 } 00436 //Tag LED continuous ON during 1s 00437 TagReadLED = LED_ON; 00438 wait_ms(2000); 00439 00440 //Turn off Tag LED 00441 TagReadLED = LED_OFF; 00442 } else { 00443 PC.printf("\tWARNING: Fail to send Tag ID (Code=%d)\r\n", rtrn); 00444 red = 1; 00445 } 00446 } 00447 Antenna.PCD_AntennaOff(); 00448 //} 00449 00450 //Check alarm each 4s 00451 if (count >= 8) { // Count value = each xx seconds * 2. Example: Count >= 4 for check alarm each 2s 00452 green = 0; 00453 count = -1; 00454 int rtrn = APCDGetWappAlarm(); 00455 if (rtrn == 1) { 00456 red = 0; 00457 PC.printf("Wapp alarm received"); 00458 //wait_ms(INTERLOCK_DELAY); 00459 //Interlock = RELAY_OPEN; //Activate the relay on prober interlock 00460 wait_ms(2000); 00461 //Interlock = RELAY_CLOSED; 00462 //PC.printf(" -> Close interlock relay\r\n"); 00463 } else if (rtrn == 2) { 00464 PC.printf("WARNING: Fail to check if alarm is present (Code=%d)\r\n", rtrn); 00465 red = 1; 00466 } else if (rtrn == 0) { 00467 red = 0; 00468 } 00469 } 00470 00471 green = !green; 00472 count++; 00473 reset_trigger++; 00474 wait_ms(500); 00475 if (reset_trigger >= 14400) { 00476 NVIC_SystemReset(); // reset system each 2 hours 00477 } 00478 } 00479 }
Generated on Tue Jul 12 2022 18:49:52 by
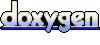