old FATFilesystem
Fork of FatFileSystem by
Embed:
(wiki syntax)
Show/hide line numbers
diskio.h
00001 /*----------------------------------------------------------------------- 00002 / Low level disk interface modlue include file R0.06 (C)ChaN, 2007 00003 /-----------------------------------------------------------------------*/ 00004 00005 #ifndef _DISKIO 00006 00007 #define _READONLY 0 /* 1: Read-only mode */ 00008 #define _USE_IOCTL 1 00009 00010 #include "integer.h" 00011 00012 #ifdef __cplusplus 00013 extern "C" { 00014 #endif 00015 00016 /* Status of Disk Functions */ 00017 typedef BYTE DSTATUS; 00018 00019 /* Results of Disk Functions */ 00020 typedef enum { 00021 RES_OK = 0, /* 0: Successful */ 00022 RES_ERROR, /* 1: R/W Error */ 00023 RES_WRPRT, /* 2: Write Protected */ 00024 RES_NOTRDY, /* 3: Not Ready */ 00025 RES_PARERR /* 4: Invalid Parameter */ 00026 } DRESULT; 00027 00028 00029 /*---------------------------------------*/ 00030 /* Prototypes for disk control functions */ 00031 00032 DSTATUS disk_initialize (BYTE); 00033 DSTATUS disk_status (BYTE); 00034 DRESULT disk_read (BYTE, BYTE*, DWORD, BYTE); 00035 #if _READONLY == 0 00036 DRESULT disk_write (BYTE, const BYTE*, DWORD, BYTE); 00037 #endif 00038 DRESULT disk_ioctl (BYTE, BYTE, void*); 00039 void disk_timerproc (void); 00040 00041 #ifdef __cplusplus 00042 }; 00043 #endif 00044 00045 00046 /* Disk Status Bits (DSTATUS) */ 00047 00048 #define STA_NOINIT 0x01 /* Drive not initialized */ 00049 #define STA_NODISK 0x02 /* No medium in the drive */ 00050 #define STA_PROTECT 0x04 /* Write protected */ 00051 00052 00053 /* Command code for disk_ioctrl() */ 00054 00055 /* Generic command */ 00056 #define CTRL_SYNC 0 /* Mandatory for read/write configuration */ 00057 #define GET_SECTOR_COUNT 1 /* Mandatory for only f_mkfs() */ 00058 #define GET_SECTOR_SIZE 2 00059 #define GET_BLOCK_SIZE 3 /* Mandatory for only f_mkfs() */ 00060 #define CTRL_POWER 4 00061 #define CTRL_LOCK 5 00062 #define CTRL_EJECT 6 00063 /* MMC/SDC command */ 00064 #define MMC_GET_TYPE 10 00065 #define MMC_GET_CSD 11 00066 #define MMC_GET_CID 12 00067 #define MMC_GET_OCR 13 00068 #define MMC_GET_SDSTAT 14 00069 /* ATA/CF command */ 00070 #define ATA_GET_REV 20 00071 #define ATA_GET_MODEL 21 00072 #define ATA_GET_SN 22 00073 00074 00075 #define _DISKIO 00076 #endif
Generated on Mon Jul 18 2022 00:47:31 by
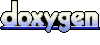