LCD TFT for ssd0139 driver 8 bit mode - using portout for max speed
Dependents: TFT_CJS_ssd01399_portver_prettyfont TFT_CJS_ssd01399_portver_prettyfont snake_main_game ServerLatest
Fork of LCDTFT_ssd01399 by
LCDTFT.h
00001 /** 00002 @file LCDTFT.h 00003 @version: 1.0 00004 00005 @web www.micros-designs.com.ar 00006 @date 30/01/11 00007 00008 *- Version Log --------------------------------------------------------------* 00009 * Fecha Autor Comentarios * 00010 *----------------------------------------------------------------------------* 00011 * 30/01/11 Suky Original * 00012 * june / 2015 Chris Stevens Update 00013 *----------------------------------------------------------------------------*/ 00014 /////////////////////////////////////////////////////////////////////////// 00015 //// //// 00016 //// //// 00017 //// (C) Copyright 2011 www.micros-designs.com.ar //// 00018 //// Este c�digo puede ser usado, modificado y distribuido libremente //// 00019 //// sin eliminar esta cabecera y sin garant�a de ning�n tipo. //// 00020 //// //// 00021 //// //// 00022 /////////////////////////////////////////////////////////////////////////// 00023 00024 /** Library for SSD01399 controller using port rather than bus to speed up screen updates 00025 * 00026 * 00027 * @code 00028 * #include "mbed.h" 00029 * #include "LCDTFT.h" 00030 * 00031 *PortOut MyPort(p13,p14,p15,p16,p17,p18,p19,p20,p30,p29,p28,p27,p26,p25,p24,p23); 00032 *LCDTFT MyLCD(p5,p6,p7,p22,p21,&MyPort); 00033 * 00034 *int main(){ 00035 * MyLCD.vLCDTFTInit(); 00036 * MyLCD.vLCDTFTFillScreen(ColorWhite); 00037 * MyLCD.printf("Hola mbed!!!"); 00038 * 00039 * MyLCD.vDrawImageBMP24Bits("IMG0.BMP"); 00040 * 00041 * while(1){ 00042 * } 00043 *} 00044 * @endcode 00045 */ 00046 00047 #include "mbed.h" 00048 #include "Fuentes.h" 00049 00050 #define ColorRed 0xf800 00051 #define ColorGreen 0x400 00052 #define ColorBlue 0x001f 00053 #define ColorMarron 0x8208 00054 #define ColorBlack 0x0 00055 #define ColorWhite 0xffff 00056 #define ColorMaroon 0x8000 00057 #define ColorFuchsia 0xf81f 00058 #define ColorViolet 0x801f 00059 #define ColorSeaBlue 0x14 00060 #define ColorGray 0x8410 00061 #define ColorOlive 0x8400 00062 #define ColorOrange 0xfc08 00063 #define ColorYellow 0xffe0 00064 #define ColorCyan 0x87ff 00065 #define ColorPink 0xf810 00066 #define ColorNavy 0x10 00067 #define ColorPurple 0x8010 00068 #define ColorTeal 0x410 00069 #define ColorLime 0x7e0 00070 #define ColorAqua 0x7ff 00071 00072 #define LCD_X_MAX 240 // these swapped 00073 #define LCD_Y_MAX 320 00074 00075 class LCDTFT: public Stream { 00076 public: 00077 /** Crea LCDTFT interface 00078 * 00079 * @param RD 00080 * @param WR 00081 * @param RS 00082 * @param CS 00083 * @param RESET 00084 * @param PORTLCD (8-bits) 00085 */ 00086 LCDTFT(PinName PIN_RD,PinName PIN_WR,PinName PIN_RS,PinName PIN_CS,PinName PIN_RESET, PortOut *PORTLCD); 00087 /** Inicializa LCD 00088 * format =0 portrait and 1 for landscape 00089 */ 00090 void vLCDTFTInit(bool format); 00091 /** Fija parametros para escritura mediante printf 00092 * 00093 * @param Xo X inicial 00094 * @param Yo Y inicial 00095 * @param Xmin X minimo 00096 * @param Xmax X maximo 00097 * @param Alto Alto de letra (1,2,3..) 00098 * @param Color Color de letra 16-bits 00099 * and the background color for clearing 00100 */ 00101 void vLCDTFTSetParametersPrintf(unsigned short Xo,unsigned short Yo,unsigned short Xmin,unsigned short Xmax,unsigned char Alto, unsigned short Color, unsigned short BackColor); 00102 /** Pinta pantalla completa de color 00103 * @param Color Color de 16-bits 00104 */ 00105 void vLCDTFTFillScreen(unsigned short Color); 00106 /** Dibuja punto en pantalla 00107 * @param x Posicion x 00108 * @param y Posicion y 00109 * @param Color Color 16-bits 00110 */ 00111 void vLCDTFTPoint(unsigned short x,unsigned short y,unsigned short Color); 00112 /** Escribe string en LCD 00113 * @param x X inicial 00114 * @param y Y inicial 00115 * @param *PtrText Texto a escribir 00116 * @param *Fuente Fuente de letra a escribir (ARIAL) 00117 * @param Alto Alto de letra (1,2,3) 00118 * @param Color Color de 16-bits 00119 */ 00120 void vLCDTFTText(unsigned short x,unsigned short y,const char *PtrText,const char (*Fuente)[5],unsigned char Alto,unsigned short Color,unsigned short BackColor); 00121 00122 void vLCDTFTLine(unsigned short x1,unsigned short y1,unsigned short x2,unsigned short y2,unsigned short Color); 00123 void vLCDTFTRectangle(unsigned short x1,unsigned short y1,unsigned short x2,unsigned short y2,bool Filled,unsigned short Color); 00124 void vLCDTFTCircle(unsigned short x,unsigned short y,unsigned short Radius,bool Filled,unsigned short Color); 00125 /** Dibuja imagen ubicada en memoria de mbed (2Mbytes) centrada en patalla 00126 * @param NameImagen nombre de imagen (ejm: IMAGEN.BMP) 00127 */ 00128 void vDrawImageBMP24Bits(const char *NameImagen); 00129 void vLCDTFTDrawImage(unsigned short x,unsigned short y, unsigned short Width, unsigned short Heigh, unsigned int Lenght, const unsigned short *Imagen); 00130 #if DOXYGEN_ONLY 00131 /** Escribe un caracter en LCD 00132 * 00133 * @param c El caracter a escribir en LCD 00134 */ 00135 int putc(int c); 00136 00137 /** Escribe un string formateado en LCD 00138 * 00139 * @param format A printf-style format string, followed by the 00140 * variables to use in formating the string. 00141 */ 00142 int printf(const char* format, ...); 00143 #endif 00144 00145 private: 00146 DigitalOut LCD_PIN_RD,LCD_PIN_WR,LCD_PIN_RS,LCD_PIN_CS,LCD_PIN_RESET; 00147 PortOut *LCD_PORT; 00148 unsigned short X,Y,X_min,X_max,_Alto,_Color,_Background; 00149 // Stream implementation functions 00150 virtual int _putc(int value); 00151 virtual int _getc(); 00152 00153 virtual void vLCDTFTWriteCommand(unsigned short Data); 00154 virtual void vLCDTFTWriteData(unsigned short Data); 00155 virtual void vLCDTFTWriteCommandData(unsigned short CMD,unsigned short Data); 00156 virtual void vLCDTFTAddressSet(unsigned short x1,unsigned short y1,unsigned short x2,unsigned short y2); 00157 virtual void vLCDTFTAddressSetPoint(unsigned short x,unsigned short y); 00158 }; 00159 // **************************************************************************************************************************************
Generated on Wed Jul 13 2022 02:54:41 by
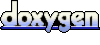