
USB Keyboard extended example This program will emulate an USB keyboard and emits predefined key sequences when the corresponding button was pressed
main.cpp
00001 00002 /* USB Keyboard extended example 00003 * This program will emulate an USB keyboard and 00004 * emits predefined key sequences when the corresponding 00005 * button was pressed 00006 * 00007 * Tested on NUCLEO-F446RE board with Arduino Multifunction Board using Mbed 2 00008 */ 00009 #include "mbed.h" 00010 #include "USBKeyboard.h" 00011 00012 DigitalIn B1(BUTTON1,PullUp); 00013 DigitalIn S1(A1,PullUp); 00014 DigitalIn S2(A2,PullUp); 00015 DigitalIn S3(A3,PullUp); 00016 USBKeyboard keyboard; 00017 00018 int B1state = 1; 00019 int S1state = 1; 00020 int S2state = 1; 00021 int S3state = 1; 00022 00023 int main(void) 00024 { 00025 while (1) { 00026 if (B1state && !B1) { 00027 keyboard.mediaControl(KEY_VOLUME_DOWN); 00028 keyboard.printf("Hello World from Mbed USBKeyboard demo\r\n"); 00029 keyboard.keyCode('s', KEY_CTRL); 00030 keyboard.keyCode(KEY_CAPS_LOCK); 00031 B1state = 0; 00032 wait(1); 00033 } else if(!B1state && B1) B1state = 1; 00034 00035 if (S1state && !S1) { 00036 keyboard.keyCode('r', KEY_LOGO); // Windows kay + R key command 00037 keyboard.printf("https://megtestesules.info\r\n "); 00038 S1state = 0; 00039 wait(1); 00040 } 00041 else if(!S1state && S1) S1state = 1; 00042 00043 if (S2state && !S2) { 00044 keyboard.keyCode('r', KEY_LOGO); // Windows key + R key command 00045 keyboard.printf("https://megtestesules.info/hobbielektronika/\r\n "); 00046 S2state = 0; 00047 wait(1); 00048 } 00049 else if(!S2state && S2) S2state = 1; 00050 00051 if (S3state && !S3) { 00052 keyboard.keyCode('r', KEY_LOGO); // Windows key + R key command 00053 keyboard.printf("https://cspista.hu/\r\n "); 00054 S3state = 0; 00055 wait(1); 00056 } 00057 else if(!S3state && S3) S3state = 1; 00058 wait(0.02); 00059 } 00060 }
Generated on Fri Aug 12 2022 07:15:38 by
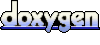