
Plays USB Audio packets through external DAC (Cirrus Logic CS4344). Simple demo code, don't expect a sophisticated I2S library. HW setup: mbed DIP 5 (I2STX_SDA) -> DAC SDIN (Serial Audio Data Input) mbed DIP 6 (I2STX_WS) -> DAC LRCK (Left Right Clock) mbed DIP 7 (I2STX_CLK) -> DAC SCLK (External Serial Clock Input) mbed DIP 30 (I2SRX_CLK) -> DAC MCLK (Master Clock) also needed an extra USB connector wired to mbed. Does not work with built-in USB. Code needs a bit of clean up.
main.cpp
00001 #include "mbed.h" 00002 #include "USBAudio.h" 00003 #include "I2S.h" 00004 00005 Serial pc(USBTX, USBRX); 00006 00007 // frequency: 48 kHz 00008 #define FREQ 48000 00009 00010 // 1 channel: mono 00011 #define NB_CHA 2 00012 00013 // length of an audio packet: each ms, we receive 48 * 16bits ->48 * 2 bytes per channel. For stereo multiply by 2 00014 #define AUDIO_LENGTH_PACKET 48 * 2 * 2 00015 00016 // USBAudio 00017 USBAudio audio(FREQ, NB_CHA,FREQ, NB_CHA,0x7bb8, 0x1112); 00018 00019 int main() { 00020 int16_t buf[AUDIO_LENGTH_PACKET/2]; 00021 00022 00023 setup(&pc); 00024 00025 while (1) { 00026 // read an audio packet 00027 audio.read((uint8_t *)buf); 00028 00029 // send to DAC 00030 play(buf,AUDIO_LENGTH_PACKET/2); 00031 } 00032 }
Generated on Sat Aug 27 2022 10:07:19 by
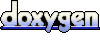