FSR
Dependents: FSR_hello_world FINAL_PROJECT Pousse_seringue_Ulg 0__Pousse_seringue ... more
FSR.h
00001 #ifndef FSR_H 00002 #define FSR_H 00003 00004 #include "mbed.h" 00005 00006 /** Force sensitive resistor class using an AnalogIn pin 00007 * 00008 * Example: 00009 * @code 00010 * #include "mbed.h" 00011 * #include "FSR.h" 00012 * FSR fsr(p20, 10); // Pin 20 is used as the AnalogIn pin and a 10k resistor is used as a voltage divider 00013 * int main(){ 00014 * while (1) 00015 * { 00016 * printf("The raw data is %f\n", fsr.readRaw()); 00017 * printf("The resistance of the FSR is %f\n", fsr.readFSRResistance()); 00018 * printf("The weight on the FSR is %f\n\n", fsr.readWeight()); 00019 * wait(0.3); //just here to slow down the output for easier reading 00020 * } 00021 * } 00022 * @endcode 00023 */ 00024 00025 class FSR 00026 { 00027 public: 00028 /** Create an FSR object 00029 * 00030 * @param Pin AnalogIn pin number 00031 * @param resistance resistance of the voltage divider resistor in k 00032 */ 00033 FSR(PinName Pin, float resistance); 00034 00035 /** Read the raw data 00036 * 00037 * @return the raw float data ranging from 0 to 1 00038 */ 00039 float readRaw(); 00040 00041 /** Read the resistance of the FSR 00042 * 00043 * @return the resistance of the FSR 00044 */ 00045 float readFSRResistance(); 00046 00047 /** Read the weight in N. 0 anyway if the weight is less than 100g 00048 * 00049 * @return the weight ranging from 100g to 10000g 00050 */ 00051 float readWeight(); 00052 protected: 00053 AnalogIn _ain; 00054 float _r; 00055 }; 00056 00057 #endif
Generated on Tue Jul 12 2022 11:35:09 by
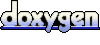